The Many Meanings
of IoC in JavaScript
Tomasz Ducin
Tomasz Ducin
21.10.2019, Katowice
The Many Meanings
of IoC in JavaScript
Tomasz Ducin
16.11.2018, Warsaw
The Many Meanings of IoC in JavaScript

Hi, I'm Tomasz
Independent Consultant & Software Architect
Trainer, Speaker, JS/TS Expert
ArchitekturaNaFroncie.pl (ANF)
Warsaw, PL
tomasz (at) ducin.dev
@tomasz_ducin
Tomasz Ducin
developer & architect
trainer @Bottega IT Minds
consultant



Title Text
INVERSION
OF CONTROL
INVERSION
OF CONTROL
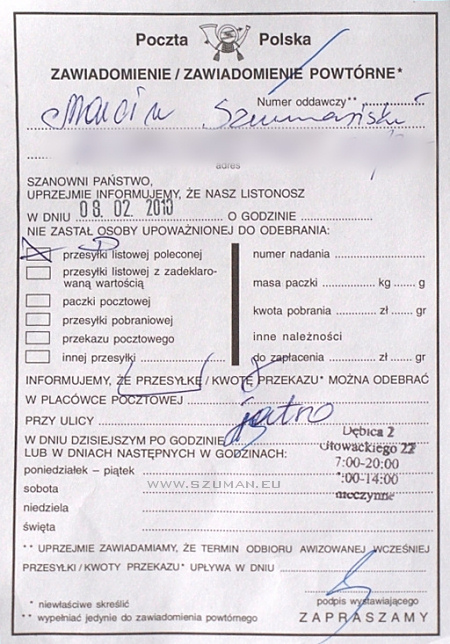


INVERSION
OF
CONTROL
taxi.takeMeTo(hotel);
LOSING CONTROL
IMPERATIVE
VS
DECLARATIVE
(NOT ONLY)
DEPENDENCY
INJECTION
(NOT ONLY)
DEPENDENCY
INJECTION


import { Component } from '@angular/core';
import { MyService } from './my-service';
@Component({
selector: 'my-component',
template: ...
})
export class MyComponent {
constructor(myService: MyService) {}
...
}
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root',
})
export class MyService {
...
}
class CoffeeMaker {
constructor(container) {
this.beanFactory = container.get(BeanFactory);
this.sugarFactory = container.get(SugarFactory);
this.waterFactory = container.get(WaterFactory);
}
make() {
this.beanFactory.create();
this.sugarFactory.create();
this.waterFactory.create();
}
}
var Container = require("typedi").Container;
var coffeeMaker = Container.get(CoffeeMaker);
coffeeMaker.make();
class CoffeeMaker {
constructor(container) {
this.beanFactory = container.get(BeanFactory);
this.sugarFactory = container.get(SugarFactory);
this.waterFactory = container.get(WaterFactory);
}
make() {
this.beanFactory.create();
this.sugarFactory.create();
this.waterFactory.create();
}
}
var Container = require("typedi").Container;
var coffeeMaker = Container.get(CoffeeMaker);
coffeeMaker.make();
DEPENDENCY INJECTION
โ
SERVICE LOCATOR
REDUX

(READ)
(WRITE)
PULL
VS
PUSH
pull vs push
Redux store API:
-
store.dispatch(action)
-
store.getState()
- pull-based -
store.subscribe(listener)
- push-based

TELL, DON'T ASK
(OOP PRINCIPLE)
getState()
dispatch()
subscribe()
๐คจ
PRINCIPLE
PUB-SUB
REACT

REACTIVE
VIEW
UPDATES
const Employee = ({ employee }) => (<div>
<span>{ employee.firstName }</span>
<span>{ employee.lastName }</span>
<span>{ employee.age}</span>
</div>)
class SomeService extends React.Component {
performSomeLogic(){
// including state mgmt, webservices, session
}
render(){ // should have NO view
return this.props.render(this.state)
}
}
const Page = () => <div>
<SomeService render={svcState =>
<>
<h1>{ svcState.header }</h1>
<p>{ svcState.content }</p>
</>
}></SomeService>
</div>
RENDER PROPS:
IoC INSIDE IoC
RxJS

REACTIVE
STREAMS
let rate = 3.94;
let amount = 1000;
let exchange = amount / rate; // 253.80
rate = 3.97;
exchange // DESYNC, sync manually!
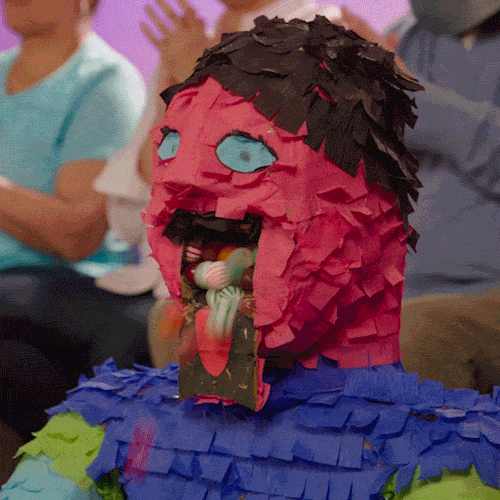
let rate$ = 3.94;
let amount$ = 1000;
let exchange$ = amount$ / rate$; // 253.80
rate$ = 3.97;
exchange$ // 251.89
slightly simplified
let rate$ = fromEvent(...) // WebSocket, 3.94
let amount$ = fromEvent(...) // input, 1000
let exchange$ = combineLatest(amount$, rate$,
(amount, rate) => amount / rate)
// AUTO -> 253.80
rate$ // external -> 3.97
exchange$ // AUTO -> 251.89
RX
REDEFINES
WHAT A VARIABLE IS
WHAT'S NEXT?
-
no Virtual DOM
-
true reactivity
<script>
const price = 9.99
let quantity = 0;
$: totalPrice = quantity * price;
function handleClick() {
quantity += 1;
}
</script>
Svelte

