Building
High Performance React Applications
slides.com/joekarlsson | @joekarlsson1

I am
Joe Karlsson

Software Engineer
How is this going to work?
Why?
My sites are fast enough...
- no one ever
40% of users abandon an article if it doesn't load after six seconds
Subtitle
+100ms delay
-1% Amazon’s sales
2015
$1,070,000,000
Optimizing
on the client
-
Simpler than optimizing on the server
-
You see immediate results
-
More "bang for your buck"
SO YOU WANNA GO FAST?
Why Is
React so
dang fast?
Virtual DOM
-
Change = entire UI re-rendered in Virtual DOM
-
DOM Diff-ing
-
Real DOM "patched"

Short Answer
ONLY RENDER WHEN YOU REALLY NEED TO
Long Answer
How To Measure Performance
Use built-in
react_perf tools
34 ms
Use
🔑 Key 🔑
Correctly
The key is used to uniquely identify an element
NEVER USE
Math.random()
or
index during a map(element, index)
class List extends Component {
render() {
const itemNode = this.props.posts.map((item, idx) => {
return <Item post={ item } key={ Math.random() } />;
})
return <div>{ itemNode }</div>;
}
};
class List extends Component {
render() {
const itemNode = this.props.posts.map((item, idx) => {
return <Item post={ item } key={ idx } />;
})
return <div>{ itemNode }</div>;
}
};
Manage
shouldComponentUpdate
Specify
exactly when a component should render
class Item extends Component {
shouldComponentUpdate(nextProps, nextState) {
if (this.props.title !== nextProps.title) {
return true;
}
return false;
}
render() {
return <h3>{ this.props.title }</h3>
}
};
Extend
✨PureComponent✨ instead of
🐢Component🐢
Does a
shallow comparison of all props/state
Will only render
if props/state change
class Item extends PureComponent {
render() {
return <h3>{ this.props.title }</h3>
}
};
✨
✨
✨
✨
✨
✨
✨
✨
✨
✨
✨
✨
✨
✨
✨
✨
✨
✨
✨
✨
✨
✨
✨
✨
Before you go
all in on PureComponent ...
Use
🔒 Immutable 🔒
Data
Fancy 🎩 word for making a new copy of a object
Immutability makes tracking changes cheap.
const x = { foo: 'bar' };
const y = x;
y.foo = 'baz';
x === y; // true
WTF???
const SomeRecord = Immutable.Record({ foo: null });
const x = new SomeRecord({ foo: 'bar' });
const y = x.set('foo', 'baz');
const z = x.set('foo', 'bar');
x === y; // false
x === z; // true
Build For
⚡️ Production ⚡️
Using
renders ~2–8x faster than the development build
NODE_ENV = 'production'
What about Stateless Components?
More thoughts on performance
gzip all the plaintext
gzip on;
gzip_min_length 1000;
gzip_proxied expired
no-cache
no-store
private
auth;
gzip_types text/plain
application/xml
application/json;
Accept-Encoding: gzip, deflate
Content-Encoding: gzip
What about images?
png, lossless, everyone uses it
Don't immediately use
progress indicators
User perception: "This is slow"
HTTP/2

Analyze your webpack bundle 🔬

Profile your app often 🏃
ONLY RENDER WHEN YOU REALLY NEED TO
Make it work,
then make it fast
-
Use key 🗝 correctly
-
Manage shouldComponentUpdate
-
Extend ✨PureComponent ✨
-
Use Immutable Data 🔒
-
Build for production ⚡️
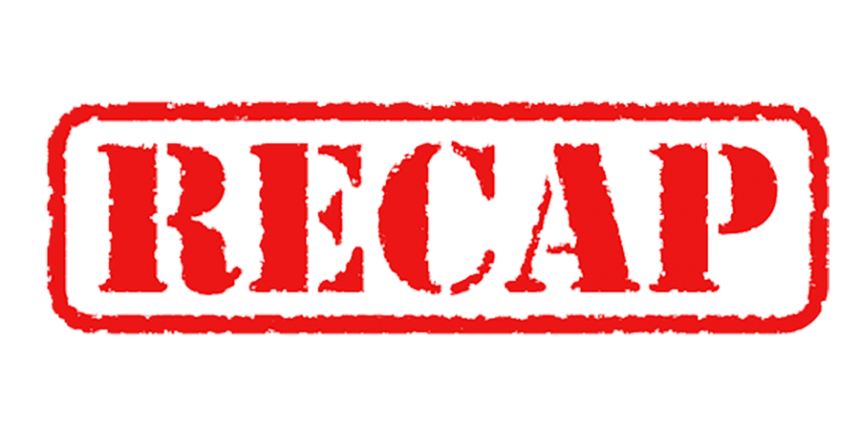
-
Analyze webpack bundle 🔬
-
Profile often 🏃💨
-
Render when really needed
-
Make it work,
then make it fast 🏃💨
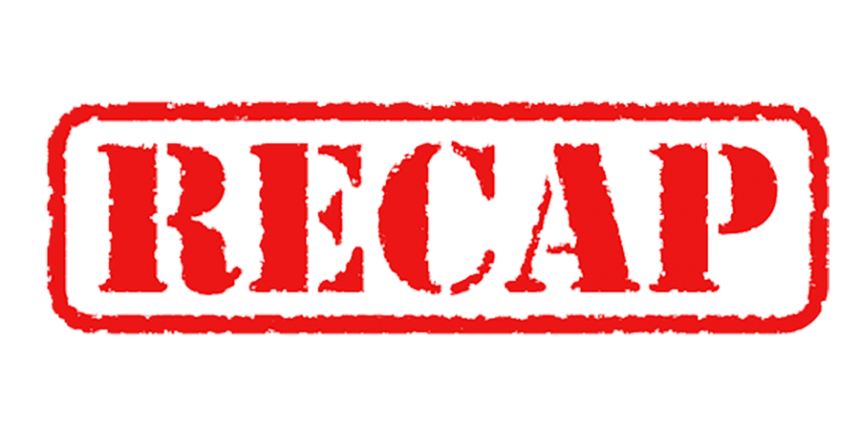

Let's Stay In Touch!
Resources:
Feedback Welcome!
Please fill out this feedback form:
https://goo.gl/forms/Hsn6oonjyIl1yxCm1