Let's Talk about
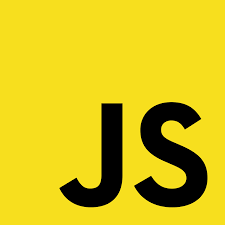
Javascript
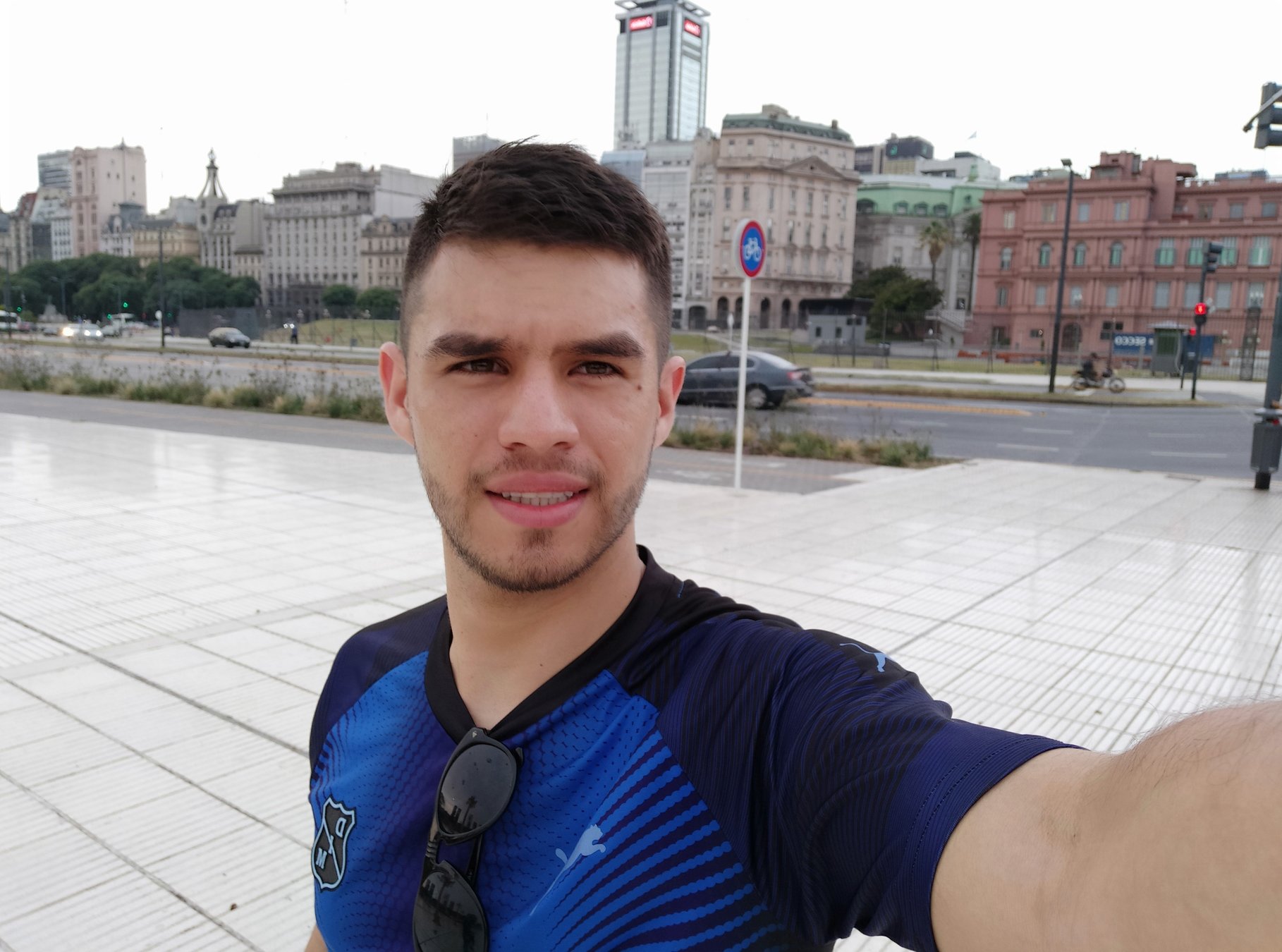
Johan Esteban Higuita
Software Developer at Talent.com
Bioengineer - Universidad de Antioquia
Session 4
Let's Talk about Javascript
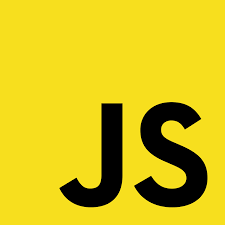
Let's Talk about Javascript
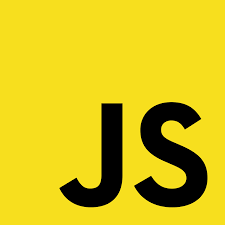
Previous session...
- Primitive vs Reference values (Immutability)
- Literal Objects
- iterables
- for - for of - for in - foreach
Let's Talk about Javascript
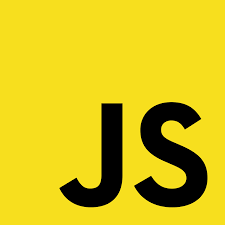
Let's Talk about Javascript
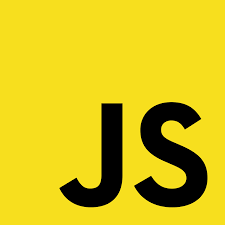
Sets & Maps
Arrays
Sets
Maps
Store data of any kind and length
Iterable, also many special array method available
Order is guaranteed
Duplicates are allowed
zero-based index to access element
Store data of any kind and length
iterable, also some special set methods available
Order is NOT guaranteed (!)
Duplicates are not allowed
No index-based access
Store key-value data of any kind and length, any key values are allowed
Order is guaranted
iterable, also some special map methods available
Duplicates are not allowed
key-based access
Let's Talk about Javascript
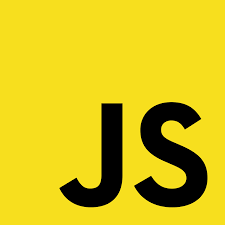
Let's Talk about Javascript
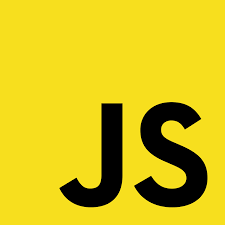
Sets
Store unique values of any type
const ids = new Set();
constructor:
Main methods:
- add(value): Appends value
- has(value): Is a value in the set?
- delete(value): Removes the element
- forEach(cb, thisArg): Iteration
Some Performance considerations:
- Accessing elements in arrays is quicker than in sets. (Array data is stored in consecutive memory).
new Set( [iterable] )
Code...
Let's Talk about Javascript
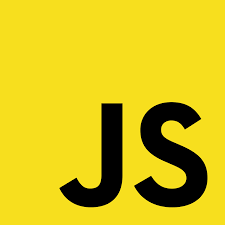
Let's Talk about Javascript
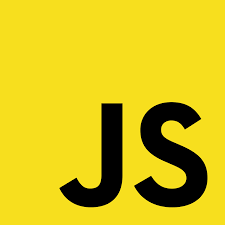
Maps
Collection of elements where each element is stored as a Key, value pair
constructor:
new Map( [iterable] )
Main methods:
- get( key ): Get the value associated to the key
- set( key, value ): Sets the value for the key
- has( key ): Is a value for the key?
- delete( key ): Removes the element
- forEach(cb, thisArg): Iteration
Some Performance considerations:
- Performs better in scenarios involving frequent additions and removals
Code...
Let's Talk about Javascript
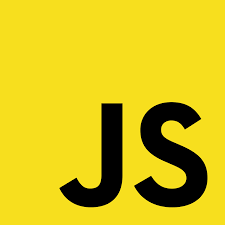
Let's Talk about Javascript
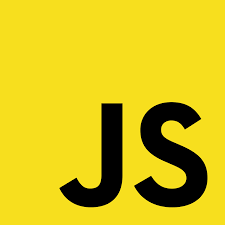
Maps vs Objects
Maps
Objects
Can use any values (and types) as keys
Better performance for large quantities of data
Better performance when adding/removing data frequently
Only may use strings, numbers or symbols as keys
Perfect for small/medium-sized sets of data
Easier / quicker to create
data is stored in consecutive memory
The number of items is easily retrieved from its size property
The number of items must be determined manually
WeakSet & WeakMap
Let's Talk about Javascript
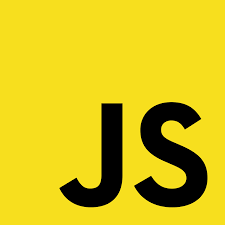
Let's Talk about Javascript
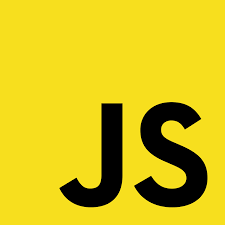
Something about functions...
A different way of defining functions
Function statement
Function Expression
function add(a, b){
return a + b;
}
const add = function(a, b){
return a + b;
}
Hoisted to top, can be declaraded anywhere un the file
Hoisted to top but not initialized.
Can't be declaraded anywhere in the file
Code...
Let's Talk about Javascript
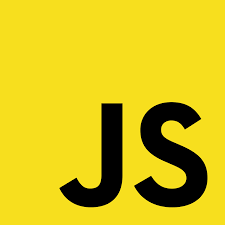
Let's Talk about Javascript
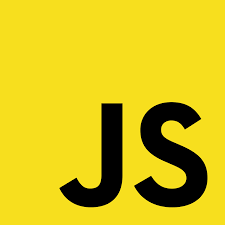
IIFE
Immediately Invoked Function Expression:
runs as soon as it is defined.
Let's Talk about Javascript
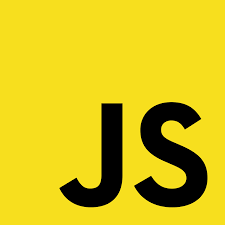
Let's Talk about Javascript
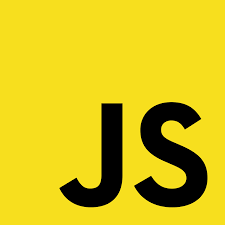
Arrow functions
- They were introduced in ES6
- Write shorter function syntax
- No 'function' keyword is required
(arg1, arg2) => { ... }
General Syntax:
No arguments
Empty pair of parentheses is requires
Exactly one argument
Exactly one expression in function body
More than one expression in function body
Parentheses can be omitted
Curly braces can be omitted, result is returned
Curly braces and return statement required
() => { ... }
arg => { ... }
(a, b) => a + b
(a, b) => {
a *= 10;
return a + b;
}
Code...
Let's Talk about Javascript
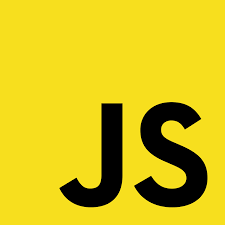
'This' keyword in functions
Inside funtions, 'this' will refer to the "thing" which called that function:
- Global context (e.g: Window Object in the browser)
- An Object (e.g: using methods)
- Some bound data (e.g: HTML element)

Using arrow functions!!!
Code...
Let's Talk about Javascript
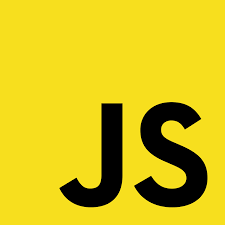
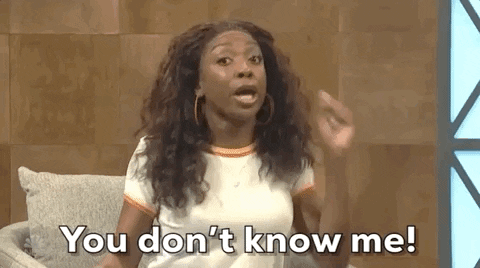
this keyword
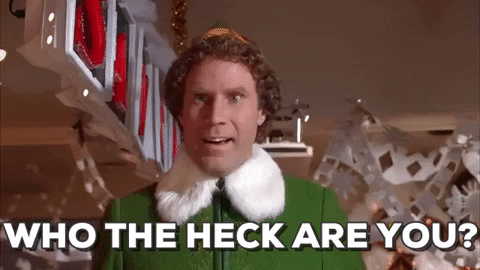
arrow function
Arrow functions do not know this shit keyword!!!
In arrow functions, this refers to the global object
Let's Talk about Javascript
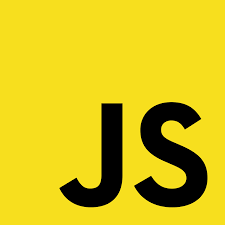
'This' keyword in functions
non-arrow function | arrow function | |
---|---|---|
Called in the global context | Global Object | Global Object |
Called on an Object | The Object | Global Object |
Let's Talk about Javascript
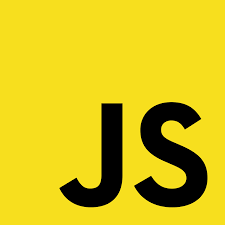
Let's Talk about Javascript
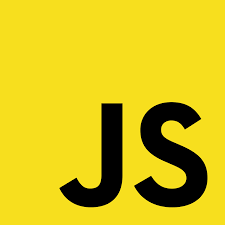
More Topics...
Main JavaScript featuresConcurrency and ParalellismAsynchronism and Non-Blockingasync vs deferES6 IntroductionScopevar let constHoistingShadowingStrict Mode
- Memory managment
- Promises & Callbacks
- Closures
- IA APIs
- bind vs call vs apply
- Node
- map, reduce, filter, find
- Prototypes vs Classes (ES6)
ImmutabilityLoopingPrimitive Referenced valuesWeb workersIteratorsfor of / for in / for / foreachMaps & Setsarrow functions (ES6)IIFEthis
Thank you!