Angular2
NgModule Overview
by @laco0416

about me

ng-sake

http://ng-sake.connpass.com/
NgModule
- Mark application entry point
- Configure platform
Module declaration
import {NgModule, ApplicationRef} from '@angular/core';
import {CommonModule} from '@angular/common';
import {FormsModule} from '@angular/forms';
import {MaterialModule} from '@angular2-material/module';
import {AppComponent} from './app.component';
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule, CommonModule, FormsModule, MaterialModule],
entryComponents: [AppComponent]
})
class AppModule {
constructor(appRef: ApplicationRef) {
appRef.bootstrap(AppComponent);
}
}
bootstrapping
import {AppModule} from './app.module';
import {platformBrowserDynamic} from '@angular/browser-platform-dynamic';
platformBrowserDynamic().bootstrapModule(AppModule);
class NgModule {
declarations: Array<ComponentType | DirectiveType | PipeType>;
imports: Array<ModuleType | ModuleWithProviders>;
exports: Array<ComponentType | DirectiveType | PipeType | ModuleType>;
providers: Array<Providers | Array<any> >;
entryComponents: Array<ComponentType>;
schemas: Array<any>;
}
@NgModule
NgModule.declarations
- List of your directives/pipes
- Can be used anywhere
- Loaded as platform stuff
NgModule.declarations
@NgModule({
declarations: [
AppComponent,
MyComponent,
MyDirective,
MyPipe,
...SOME_LIBRALIES_DIRECTIVES
],
})
NgModule.providers
- List of your providers
- Can be injected anywhere
Module providers
@NgModule({
providers: [
MyService,
SomeLibraryService,
],
})
NgModule.imports
- Load other modules
- Official modules
- BrowserModule
- HttpModule
- RouterModule
- FormsModule
- MaterialModule
NgModule.exports
- Module as a Library
- Expose module's things
Module exporting
@NgModule({
declarations: [AwesomeComponent, AwesomePipe]
exports: [AwesomeComponent, AwesomePipe],
providers: [AwesomeService]
})
export class AwesomeModule {
}
NgModule.entryComponents
- Initial components to compile
- Use cases
- AppComponent
- Lazy Loading
Migration
Legacy | New |
---|---|
bootstrap(.., [MyService]) | NgModule.providers |
Component.pipes | NgModule.declarations |
Component.directives | NgModule.declarations |
Component.providers | NgModule.providers |
RC.4
@Component({
directives: [ MyComponent ],
pipes: [ MyPipe ],
providers: [ MyService ],
})
class AppComponent {
}
bootstrap(AppComponent, [
HTTP_PROVIDERS
])
Legacy bootstrapping
with Modules
import {bootstrap} from '@angular/browser-platform-dynamic';
import {AppComponent} from './app.component';
import {FormsModule} from '@angular/forms';
bootstrap(AppComponent, {imports: [FormsModule]});
for migration
Why NgModule?
- Component.directives/pipes
- Providers inheritance
- Library architecture
- Easy to use
- Easy to migrate from angular.module()
angular.module('app', ['ui.router'])
.service('myService', MyService)
.component('myComponent', {...})
.config(() => {...});
@NgModule({
imports: [RouterModule],
delcarations: [MyComponent],
providers: [MyService]
})
class AppModule {
constructor() {
...
}
}
Angular modules
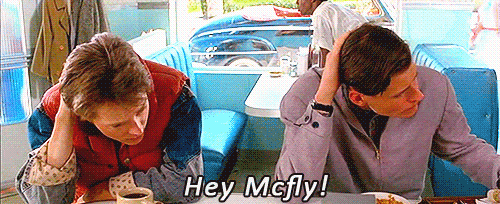
Conclusion
- Use NgModule.providers
- Remove Component.providers
- Use NgModule.declarations
- Remove Component.directives/pipes
- Keep single scope
- Use modules
- Http, Forms, Router, ...
- Make modules
- Module as a Library