Beginning Programming in Python
Fall 2019

About Myself
-
Narges Norouzi, Faculty in the Computer Science & Engineering department.
-
M.A.Sc and P.hD. in Computer Engineering from the University of Toronto, Canada.
-
Email: nanorouz@ucsc.edu
-
Research in Biometric Systems, Statistical Signal Processing, and Machine Learning.
Instructional Assistants
-
Teaching Assistants:
-
Rafael Espericueta; resperic@ucsc.edu
-
Michael Briden; mbriden@ucsc.edu
-
Hou-I Lin; hlin64@ucsc.edu
-
Evan Olds; eolds@ucsc.edu
-
Katelyn Stone; khstone@ucsc.edu
-




Instructional Assistants
-
Tutors:
- Farris Tang - Email: fktang@ucsc.edu (Labs B, C, & D)
- Bryan Tor - Email: btor@ucsc.edu (Labs A, C, & E)
- Radhika Gathwala - Email: ragathwa@ucsc.edu (Labs A & E)
- Chhavi Singal - Email: csingal@ucsc.edu (Labs F, G, & H)
- Thomas Cannon - Email: thwcanno@ucsc.edu (Labs B, D, & G)
- Sean Li - Email: sejli@ucs.edu (Labs F & G)
LSS Tutoring
- Andres Montes Clemens - Email: amontesc@ucsc.edu
- Benjamin Paulsen - Email: bjpaulse@ucsc.edu
3 tutoring sessions per week
2 drop-in hours a week
What this course is
-
An introduction to programming in Python
-
An introduction to theoretical problems in computer science

What this course is not
-
We do not talk about (in any depth):
-
Applications of computing
-
Other programming languages (Java, C, C++, Matlab, etc.)
-
History of computing (well, not much)
-
How to use Microsoft office or create a web page(!)
-
Course Objectives
-
Understand the fundamentals of programming.
-
Understand the fundamentals of object-oriented programming.
-
Gain exposure to the important topics and principles of software development.
-
Have the ability to write computer programs to solve specified problems.
-
Use software development environment to create, debug, and run programs.
Honor Policy
-
The UCSC honor Policy is in effect in this class. As a student in the course, you also agree to follow the following principles.
You are not allowed to describe problems on an exam or quiz to a student who has not taken it yet. You are not allowed to show exam papers to another student or view another student's exam papers while working on an exam.
No code or solutions are to be distributed to other students either electronically (i.e. e-mail) or on paper. If you are looking at another student's code (even in the lab), you are in violation of this honor policy.
Honor Policy
-
The UCSC honor Policy is in effect in this class. As a student in the course, you also agree to follow the following principles.
Unless otherwise specified, the only allowed collaboration for the assignments is the discussion of ideas; no collaboration is allowed on the exams and assignments.
Unless otherwise noted, exams and individual assignments are pledged: you promise that you have neither given nor received unauthorized help.
When you're in doubt regarding the honorability of an action, you MUST ask before doing it.
Grading Policy
5 bi-weekly assignments: 40% - due every other Sunday
10 readings/practices from zybooks: 10% - due every Sunday
Attendance: 5% - due ~10 minutes after each lecture
Midterm: 20%
Final exam: 25%

Assignments
-
5 programming assignments due at 11:59 pm on Sundays every other week.
-
Submission is through Stepik. You need to sign in on Canvas and navigate to Stepik course through the "Stepik submission" module.
-
Assignments will be marked out of 100 depending on the number of test cases you pass on Stepik.
-
Late policy:
-
2 free "grace days", no explanation necessary
-
You cannot use both of them on one assignment
-
No late submission after using both grace days
-
My Philosophy: Hard But Fair
-
Fairness is a challenge in a class of ~300 students
-
If you feel something is not fair, you need to let me know. I will do my best to correct it
-
If you think that this course is too easy, let me know. I will do my best to correct it
Who to contact?
-
I am not always the best person
-
I easily get flooded with emails, as I have hundreds of students (~450 this quarter)
-
The TAs can often answer a question just as easily as I can, and much quicker
-
-
Piazza!
-
If you feel like you do not want other students to see your question, you can post it privately for instructors - or post anonymously
-
Our response time is on average below 20 minutes
-

Lots of contact hours
- Narges: Tuesdays 4 to 6 PM (E2-357)
- Michael Briden (TA): Tuesdays 10 - 12 PM (BE-153A)
- Hou-i Lin (TA): Wednesday 2:30 - 3:30 PM & Thursday 12 - 1 PM (BE-153A)
- Katelyn Stone (TA): Monday 3 -5 PM (BE-153A)
- Bryan Tor (Tutor): Thursdays 4 - 5 PM (BE-318)
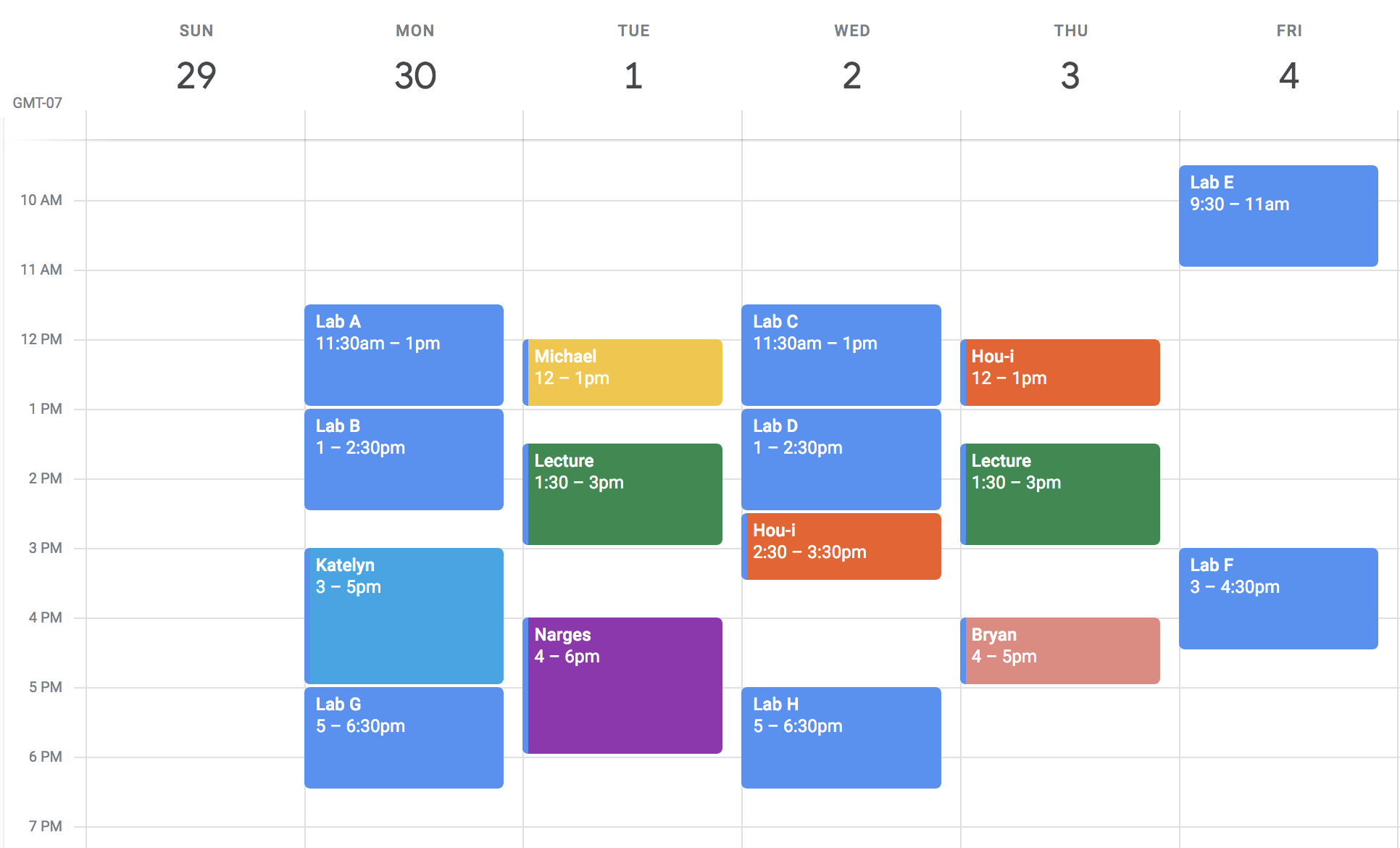
Textbook
-
We will use an online interactive book called Zybooks
-
Zybooks:
-
Sign in or create an account at learn.zybooks.com
-
Enter zyBook code: UCSCCSE20Fall2019
-
Subscribe: A subscription is $40 and will last until December 30th.
- Another book (not required):
"How to think like a computer scientist":
http://www.openbookproject.net/thinkcs/python/english3e/
5 minutes break!

Programming Notebooks
-
Each lecture has one associated IPython notebook
-
This lecture's notebook is here: http://bit.ly/2yY3ECC
-
The notebooks make it easy to poke around with the examples are also a great way to do basic exploratory analysis.
-
Collaboratory: Python notebooks can be edited and run in your web browser using Google Collaboratory
-
To make your own copy of the lecture, open up the above link and then make a copy in your Google drive
Alternatively, you can run the notebook using Jupyter (https://jupyter.org/)
Sample Code
# Hello world program - this is a comment
# (it's just to make the code readable)
print("Hello, world!")
A bigger example
## A basic program
# Inputs - let's ask the user to input two numbers and
# call them x and y (x and y are called variables)
x = float(input("Please enter a number: "))
y = float(input("Please enter another number: "))
# Doing stuff
z = x + y # Add the inputs together
# Outputs
# Let's print the result
print("The sum of your two inputs is:", z)
Interpreted vs. Compiled languages
-
Python is (basically) an interpreted language:
-
Interpreted languages are generally read line-by-line and executed line-by-line.
-
This allows you to use Python interactively
-
You can also get the interpreter to use files, which is much better when you want to save your work.
-
- Other languages, e.g. C, Java, etc. are compiled:
- The code is read in its entirety before it is executed.
- There are speed (optimization) and error checking benefits to this approach (can catch an error at the end of your program before executing any of it).
- But, more complex, and generally no interactive execution.
debugging
## Our first debugging session!
# Warning - most computer programming is debugging!
# IMO, it is the process of taking an idea and refining it
# into a precise, working program
x = 1
y = 2
## Let's try an assert - a check you can put in your code to establish that something
## is the way you think it should be
assert x + y == 4
Other ways to run python
- Interactively via interpreter
- Via a Dev environment (Pycharm demo)
comments
# This is a comment
# Code can be hard to read, and yet you
# often have to edit and maintain code over time.
# Comments let you keep notes in your code.
# Rule of thumb: Good code is often about 50% code and 50% comments
# It is amazing to read code you wrote last year - you
# likely won't remember how it works without comments!
# 80 Character limit: Because some text editors don't automatically line-wrap it is a good idea to try to keep your comments to less than 80 or so characters by spreading them across multiple lines.
# Later on, we'll see comment strings, which are another way
# of documenting code.
Basic Types
-
In general, a type is the formatting of bits to represent a given information
-
Types impose constraints, e.g. ints are whole numbers only
Integer
Float
String
Boolean
Basic Types
# What's the "type" of 1?
# For now, just understand that we can use type()
# to find out the 'type' of any object in Python
type(1)
# Outputs <class, 'int'> or prints int
# Similarly, 5 is an integer (called an 'int')
type(5)
# Okay, so what's the type of a decimal?
type(3.142)
# And the type of 1.0?
# (there is no room for indecision/ambiguity in a programming language)
type(1.0)
# What about text?
type("hello")
String type
# Strings can be expressed a bunch of other ways:
type("hello") # Double quotes
type('hello') # Single quotes
type("""hello""") # Triple double quotes
type('''hello''') # Triple single quotes
# We can embed single quotes in double quotes:
type("This is a string containing a 'single quoted substring'")
# Trying to embed single quotes
# in a single quoted string naively does not work
type('This is a string containing a 'single quoted substring'')
# And vice-versa, we can embed double quotes in single quotes:
type('This is a string containing a "double quoted substring"')
# Single and double quoted strings can't span multiple lines. This is an error
type('This is an
attempt to have a string on two lines')
Triple quotes
# Triple quotes (either single or double), allow embedding essentially anything,
# including spanning multiple lines:
type("""Boom, now
we can make crazy strings that ' nest quotes in quotes " " ' etc.
and which span multiple lines""")
# You will also see this a lot in Python code:
"""
A comment string.
These can be used to document some code
across multiple lines. We'll later see this at the top
of classes and functions as a standard way of giving
a description string.
"""
# Will print: " \nA comment string. \n\nThese can be used to document some code\n ... "
Type Conversion
# It is useful to know that int, float and string types can be cross converted
type( int('5') ) # This int() turns the string into an integer
type( str(5) ) # We can also go back the other way
# Of course, this doesn't work because int() doesn't how to interpret 'foo'
int("foo")
# We can also convert between floats and ints, doing
# rounding as we go float --> int
int(5.999)
float(5)
# Prints 5.0
putting it all together
What's due next?
- Zybooks Chapter 1 on September 29th (!)
- Not graded (but do it - seriously):
- Installing latest version of Python on your laptop
- Install Pycharm - test sample codes
- Create an iPython notebook, try running it by Google Colab
- Run different cells of the first lecture's notebook
Lecture 1 challenge
Questions?

CSE 20 - Lecture 1
By Narges Norouzi
CSE 20 - Lecture 1
- 2,928