JavaScript : Days of the Future Past

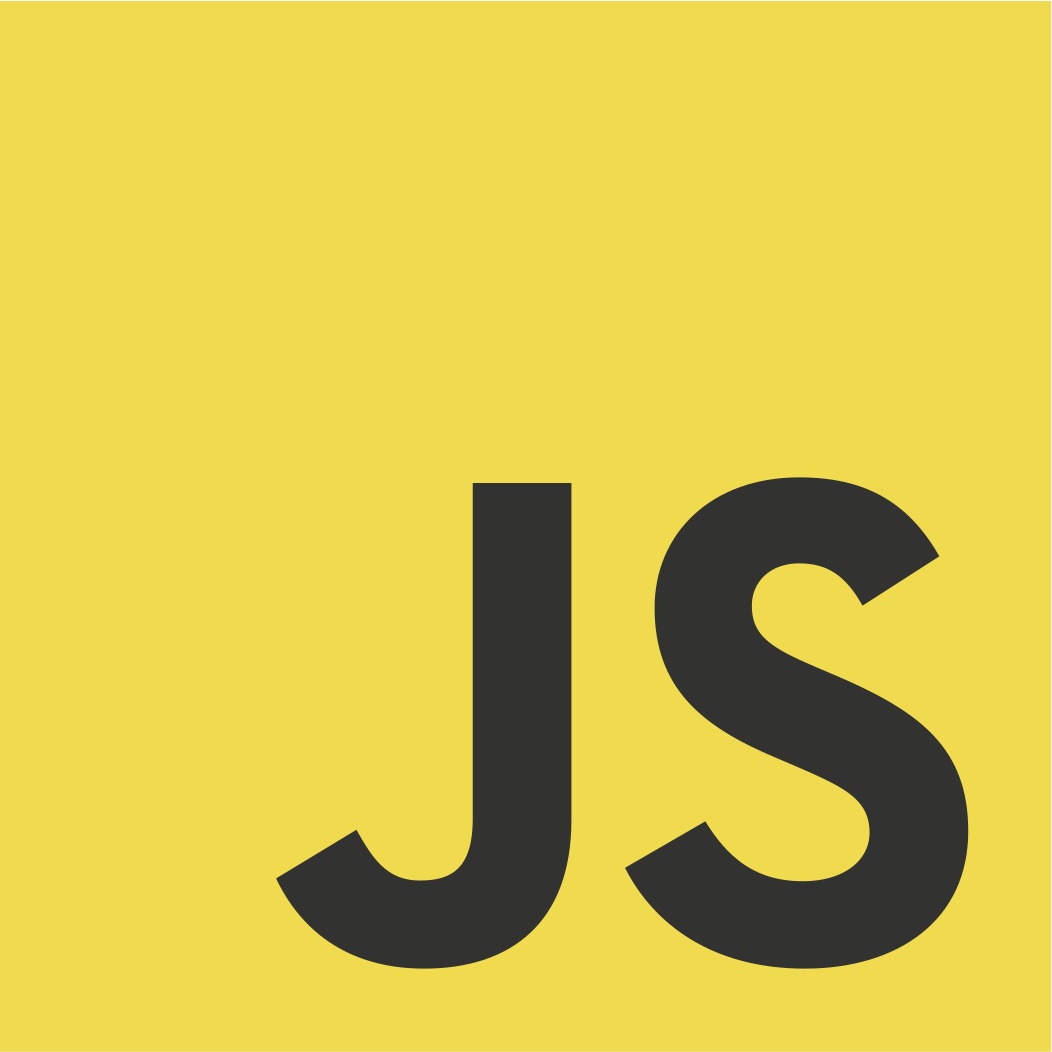
Once upon a time ...
- 'Mocha' - May 95
- LiveScript September 95
- JavaScript December 95
- MS JScript August 96
- Ecma 262 - 97
- 3rd Edition - 99
- 2007 Crossroads
- 2008 Incremental Update - 5
- Harmony and ES.next
- 2014 ES 6
How TC 39 Works
Requirements
- Concrete Demonstration
- Casual Developers
- Start small iteratively prototype
Goals
- Better for Complex apps, libraries, code generators.
- Testable spec.
- De facto Standards.
Why so long?

Radicals
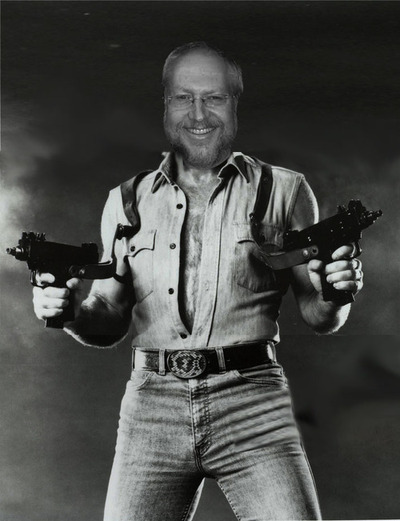
Reactionaries
Moderates

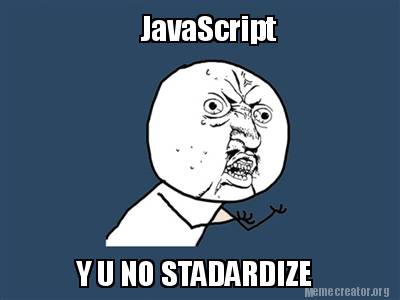
"I’ve been a member of ECMA TC39 off and on for a decade and a half, and based on what I have seen, JavaScript will catch up with Underscore in 30 to 50 years."
Libraries, Polyfills, Shims as stepping stones.
ES 6
- Object.is()
console.log(NaN == NaN); // false
console.log(NaN === NaN); // false
console.log(Object.is(NaN, NaN)); // true
- Let Keyword (Block Binding)
function x(condition) {
if (condition) {
console.log(value1); // Undefined!
var value1 = "aditya";
}
console.log(value1); // aditya
//ES6
if (condition) {
console.log(value2); // ReferenceError!
let value2 = "punjani";
}
console.log(value2); //Will also be ReferenceError!
}
x(true);
- Default Parameters
function x(value, time, callback) {
time = time || 10;
callback = callback || function() {};
//Or do some ugly hack with Arguments special object;
}
ES6
function x(value, time=10, callback=function(){}) {
//Do magic here
}
- Rest and Spread
//Rest
function sum(first, ...numbers) {
let result = first,
i = 0,
len = numbers.length;
while (i < len) {
result += numbers[i];
i++;
}
return result;
}
//Spread
let values = [25, 50, 75, 100]
// equivalent to
// console.log(Math.max(25, 50, 75, 100));
console.log(Math.max(...values)); // 100
- Arrow Functions
1. Lexical 'this' binding,
2. Not newable,
3. Cant change 'this'
4. No arguments object.
var reflect = value => value; // is the same as var reflect = function(value) { return value; };
- For of
//Lets you iternate over iterable objects - Arrays, Arguments, Nodelists,Generators.
function printArgs() {
for (let arg of arguments) {
console.log(arg);
}
}
// Iterate over all <div> elements
var divs = document.getElementsByTagName("div");
for (let div of divs) {
div.innerHTML = "Changed...";
}
- Generators
function* gen() { yield 1; yield 2; }
for (let i of gen()) console.log(i); // prints 1 and 2
- Sets
var items = new Set(); items.add(5); items.add("5"); console.log(items.size()); // 2 console.log(items.has(5)); // true items.delete(5)
console.log(items.has(5)); // false
- Maps
- Weak Maps
- Class
- Promises
var p = new Promise(function(resolve,reject){
setTimeout(function(){
resolve("Yay!");
},100);
});
p.then(function(msg){
console.log(msg); // Yay!
});
- Tail Call optimisation
- Parallel JS
- Object.observe
Thank You

JavaScript : Days of the Future Past
By Aditya Punjani
JavaScript : Days of the Future Past
- 829