Week 0 Review
Git + IntelliJ
A Java program
Printing
Variables + Types
User Input
If Statements
Git + IntelliJ
@_@
a Java program
Java
= a programming language + platform
platform
= an environment for running other programs
Java platform
- JVM
- Java language + compiler
- Java standard libraries
This is all included in the JDK
(Java Development Kit)
JVM
Java Virtual Machine
(virtual computer)
compiler
= a program that transforms source code into
machine code
in IntelliJ!
HelloWorld.java
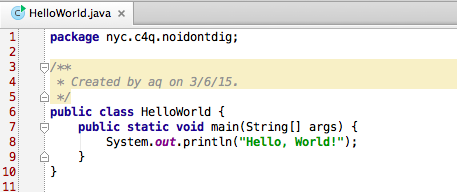
Variables + Types
variables
= names associated with values in a computer
variables allow programmers to save data and reference it later
naming variables
- case sensitive
- any length of letters and/or digits
- must begin with letter, "$", "_"
- by convention begin with a letter
- whitespace is not permitted
- by convention are camelCase
- should be descriptive!!!
ex: myAge, student9, isAlwaysOnTime
Primitive Types
every variable in Java has a type
Type
= a set of values and operations on those values
8 primitive types
- byte (8-bit integer)
- short (16-bit integer)
- int (32-bit integer)
- long (64-bit integer)
- float (32-bit floating point number)
- double (64-bit floating point number)
- boolean (true or false)
- char (a single character like '$' or 'a')
boolean
true or false
boolean operators
- >
- >=
- <
- <=
- ==
- !=
char
= represents a single character ('a', '$', '9')
denote a char by placing it between single quotes ' '
String
(not a primitive type)
= sequence of characters denoted by double quotes
concatenation
= the operation of joining strings together
ex. "Hello, " + "World!";
User Input
using Scanner
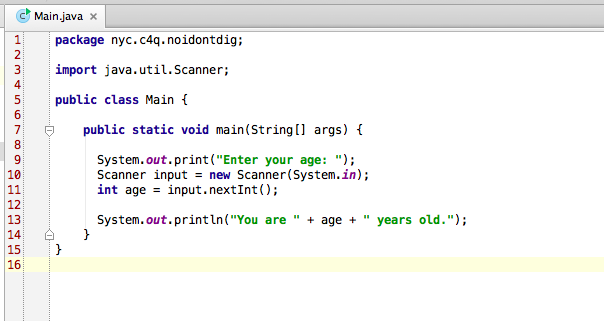
Scanner methods
- next()
- nextByte()
- nextShort()
- nextInt()
- nextLong()
- nextFloat()
- nextDouble()
- nextBoolean()
If Statements
Conditional Statements
= control structure
what are some real world examples of software using control structures?
if (7 > 3) {
System.out.println("Math is real");
}
System.out.println("This always executes");
if
if (7 > 3) {
System.out.println("Math is real");
} else {
System.out.println("Math is a lie");
}
System.out.println("This always executes");
if else
// aq is at the cinema
if (age <= 13) {
ticketPrice = 8;
} else if (age <= 64) {
ticketPrice = 13;
} else {
ticketPrice = 10;
}
else if
bio break!
practice exercises
Week 1 Review
By Alexandra Qin
Week 1 Review
- 347