ES6 Just Do It
Косинский Алексей
Gulsy Inc.

Это я
Alexey Kosinski
Gulsy Inc
Senior Frontend and Backend developer.



avkos
atriumkos
avkosinski


let
const
Computed property names
default


{ }
let
function foo() {
{
console.log(hi);
//Uncaught ReferenceError: hi is not defined
let hi = 1;
}
console.log(hi);
//Uncaught ReferenceError: hi is not defined
for(let i = 0; i < 10; i++) {
}
console.log(i);
//Uncaught ReferenceError: i is not defined
}
foo();
const
{
const PI = 3.141593;
PI = 3.14; // throws “PI” is read-only
}
{
const scorpion={name:'Scorpion',const:'object'}
scorpion.name = 'change';
scorpion = {name:'second'}; //error
}
{
const scorpion={name:'Scorpion',const:'object'}
Object.freeze(scorpion);
scorpion.name = 'change'; //error
}

Computed property names

var obj = { };
obj[prop] = 'some value';
var obj = { [prop]: 'some value' };
//this will assign to obj.foo10
var obj = { ['foo' + 10]: 'some value' };
//obj.foo10='some value'
function getFieldName(){
return 'foo15';
}
var obj = { [getFieldName()]: 'some value' };
//obj.foo15='some value'
console.log(obj)
Default values
function hello(name) {
name = name || 'Anonymous';
console.log('Hi ' + name);
}
function hello(name = 'Anonymous') {
console.log('Hi ' + name);
}
function test(one, ...twoThree) {
// twoThree is an Array
return one * twoThree.length;
}
console.log(
test(3, "14", "zdec")
)
function sum(one, two, three) {
return one + two + three;
}
// Pass each elem of array as argument
console.log(
sum(...[1,2,3])
)
String
Math



Strings
let chars = [...'abc'];
// ['a', 'b', 'c']
let str = '!!!уклобтуф енм йаД!!!';
console.log([...str].reverse().join(''));
//Secret string
'hello'.startsWith('hell')
//true
'hello'.endsWith('ello')
//true
'hello'.includes('ell')
//true
'hello'.startsWith('ello', 1)
//true
'hello'.endsWith('hell', 4)
//true
'hello'.includes('ell', 1)
//true
'hello'.includes('ell', 2)
//false
'NOBODY READS THIS ANYMORE\n'.repeat(9)

Math
Math.sign(-8) // -1
Math.sign(3) // 1
Math.trunc(3.1) // 3
Math.cbrt(8) // 2
0xFF // ES5: hexadecimal
//255
0b11 // ES6: binary
//3
0o10 // ES6: octal
//8
(255).toString(16)
//'ff'
(4).toString(2)
//'100'
(8).toString(8)
//'10'
let x = NaN;
x === NaN
//false
Number.isNaN(x)
//true
Math.clz32(0b01000000000000000000000000000000)
//1
Math.clz32(0b00100000000000000000000000000000)
//2
Math.clz32(2)
//30
Math.clz32(1)
//31
//Computes the hyperbolic sine of x.
Math.sinh(x)
//Computes the hyperbolic cosine of x.
Math.cosh(x)
//Computes the hyperbolic tangent of x.
Math.tanh(x)
//Computes the inverse hyperbolic sine of x.
Math.asinh(x)
//Computes the inverse hyperbolic cosine of x.
Math.acosh(x)
//Computes the inverse hyperbolic tangent of x.
Math.atanh(x)
//Computes the square root of the sum of squares of its arguments.
Math.hypot(...values)
Math.hypot(3,4) //5
Math.hypot(...[3,4]) //5
3
4
5
for..of
Destructor



Destructor
let user = {
name:'Scrooge',
email:'McDuck@gmail.com'
}
var name = user.name;
var email = user.email;
var { name, email } = user;
//name = 'Scrooge'
//email ='McDuck@gmail.com'
var { name: userName, email: userEmail } = user;
//userName='Scrooge'
//userEmail='McDuck@gmail.com'
function sendEmail({
email,
name:userName,
surname:userLastName='defaultLastName'
}) {
console.log(email); //simpson@email.com
console.log(userName); //Homer
console.log(userLastName); //defaultLastName
}
sendEmail({
email:'simpson@email.com',
name:'Homer'
})
let values = [22, 44];
let [first, second] = values;
//first = 22
//second = 44
let a = 10, b = 20;
a=a+b;
b=a-b;
a=a-b;
//a=20
//b=10
let a = 10, b = 20;
[a,b] = [b,a];
//a=20
//b=10
var doWork = function() {
return [1, 3, 2];
};
let [, x, y, z] = doWork();
//x==3
//y==2
//z==undefined
let employee = {
firstName: "Scott",
address: {
state: "Maryland",
country: "USA"
},
favoriteNumbers: [45,55,32,13]
};
let {
firstName,
address: {state},
favoriteNumbers: [,second]
} = employee;
//firstName="Scott"
//state="Maryland"
//second=55
for...of

let iterable = [1, 2, 3];
for (let value of iterable) {
console.log(value);
}
// 1
// 2
// 3
for (let ch of 'abc') {
console.log(ch);
}
// a
// b
// c
let iterable = [3, 5, 7];
iterable.foo = "hello";
for (let i in iterable) {
console.log(i); // logs 0, 1, 2, "foo"
}
for (let i of iterable) {
console.log(i); // logs 3, 5, 7
}
let someObj=
{
key1:'1',
key2:'2',
}
for (let [key, value] of someObj) {
console.log(key + " - " + value);
}
Uncaught TypeError: someObj[Symbol.iterator] is not a function
// Maps
var myMap = new Map(),
keyString = "a string",
keyObj = {},
keyFunc = function () {};
// setting the values
myMap.set(keyString, "value associated with 'a string'");
myMap.set(keyObj, "value associated with keyObj");
myMap.set(keyFunc, "value associated with keyFunc");
// getting the values
myMap.get(keyString); // "value associated with 'a string'"
myMap.get(keyObj); // "value associated with keyObj"
myMap.get(keyFunc); // "value associated with keyFunc"
myMap.get("a string"); // "value associated with 'a string'"
// because keyString === 'a string'
myMap.get({}); // undefined, because keyObj !== {}
myMap.get(function() {}) // undefined, because
// keyFunc !== function () {}
myMap.size; // 3
let someMap = new Map();
someMap.set('key1','1');
someMap.set('key2','2');
for (var res of someMap) {
console.log(res);
}
//["key1", "1"]
//["key2", "2"]
for (var [key,value] of someMap) {
console.log(`key=${key} - value=${value}`);
}
//key=key1 - value=1
//key=key2 - value=2
let someObj= {key1:'1', key2:'2'}
for (let [key, value] of Object.entries(someObj)) {
console.log(key + " - " + value);
}
Templates
=>



Templates
var name = "Alexey";
var text = `Hello ${name}`;
//Hello Alexey
function getName(){
return 'some text';
}
var text = `Hello ${getName()}`
//Hello Alexey
var text = `Foo
bar
baz`;
function validateEmail(email){
return `email "${email}" is not valid`;
}
function someFunc(strings, name, email) {
return strings[0]+name+strings[1]+validateEmail(email);
}
var name= 'Alexey';
var email = 'alexey@email';
//strings==['Hello World ',' some text','']
//name ='Alexey'
//email='alexey@email.com'
let result = someFunc`Hello World ${name} some text ${email}`;
//Hello World Alexey some text email "alexey@email" is not valid

=>
//ES6 style
var a1 = () => 1;
//ES5 style
function a1(){
return 1;
}
function a2(x){
return 1;
}
function a4(x,y){
return 1;
}
function a5(x){
return 1;
}
var a2 = x => 1;
var a3 = (x) => 1;
var a4 = (x, y) => x+y;
var a5 = x => { return 1; };
//ES5 style
var self = this;
el.onclick = function() {
self.doSomething();
};
//ES6 style
el.onclick = () => this.doSomething()
//ES5 style
el.onclick = function(x, y, z) {
foo();
bar();
return 'baz';
}.bind(this);
//ES6 style
el.onclick = (x, y, z) => {
foo();
bar();
return 'baz';
}
//ES5 style
var names = users.map(function(u) { return u.name; });
//ES6 style
var names = users.map(u => u.name);
Symbol
Promise
Generator




Promise

img1
img2
img3
img4
t
img1()
.then(img2)
.then(img3)
.then(img4);
img1(function(){
img2(function(){
img3(function(){
img4(function(){
....
});
});
});
});
let img1 = ()=>{ return new Promise((resolve,reject)=>{
console.log(`image - loaded`)
return resolve();
//console.log(`image - failed`)
//return reject();
})}
img1
img2
img3
img4
t
img1()
.then(Promise.all([img2(), img3()]))
.then(img4);
let img3 = ()=>{
return new Promise((resolve,reject)=>{
console.log(`image 3 - error`)
return reject('some error');
})}
img1()
.then(Promise.all([img2(), img3()])
.catch((e)=>{
console.log(e)
}))
.then(img4);
Uncaught (in promise) some error
Symbol

let s = Symbol();
let s = new Symbol();
let s1 = Symbol();
let s2 = Symbol();
//s1 != s2
let s1 = Symbol("some description");
let s2 = Symbol("some description");
//s1!=s2
let firstName = Symbol();
let person = {
lastName: "Alexey",
[firstName]: "Kosinski",
};
//person.lastName == "Alexey";
//person[firstName] == "Kosinski";
Object.getOwnPropertyNames(person) // [“lastName”]
JSON.stringify(person)) //{"lastName":"Kosinski"}
Object.getOwnPropertySymbols(person) //[“firstName”]
let symbol0 = Object.getOwnPropertySymbols(person)[0];
console.log(person[symbol0]) // "Kosinski";
Symbol.iterator
class Classroom {
constructor() {
this.students = ["Tim", "Joy", "Sue"];
}
}
class Classroom {
constructor() {
this.students = ["Tim", "Joy", "Sue"];
}
[Symbol.iterator]() {
var index = 0;
return {
next: () => {
}
}
}
}
class Classroom {
constructor() {
this.students = ["Tim", "Joy", "Sue"];
}
[Symbol.iterator]() {
var index = 0;
return {
next: () => {
if(index < this.students.length){
index += 1;
}
}
}
}
}
class Classroom {
constructor() {
this.students = ["Tim", "Joy", "Sue"];
}
[Symbol.iterator]() {
var index = 0;
return {
next: () => {
if(index < this.students.length){
index += 1;
return {
done: false,
value: this.students[index-1]
};
}
}
}
}
}
class Classroom {
constructor() {
this.students = ["Tim", "Joy", "Sue"];
}
[Symbol.iterator]() {
var index = 0;
return {
next: () => {
if(index < this.students.length){
index += 1;
return {
done: false,
value: this.students[index-1]
};
}
return { value: undefined, done: true };
}
}
}
}
let scienceClass = new Classroom();
let result = [];
for(let name of scienceClass) {
result.push(name);
}
//result == ["Tim", "Joy", "Sue"]
Generator

let numbers = function*() {
yield 1;
yield 2;
yield 3;
};
let gen = numbers();
gen.next() // return object {done: false, value: 1}
gen.next().value; //2
gen.next().value; //3
let sum = 0;
for(let n of numbers()) {
sum += n;
}
//sum == 6
function* powGenerator() {
var result = Math.pow( yield "a" , yield "b" );
return result;
}
var g = powGenerator();
console.log(g.next().value); // "a", from the first yield
console.log(g.next(10).value); // "b", from the second
console.log(g.next(2).value); // 100, the result
10
2
- let && const
- Computed property names
- Default values
- Strings
- Math
- Destructor
- for...of
- Templates
- =>
- Symbol
- Promise
- Generator
How to use?
Babel
Traceur

VS

Preset 2015
- check-es2015-constants
- transform-es2015-arrow-functions
- transform-es2015-block-scoped-functions
- transform-es2015-block-scoping
- transform-es2015-classes
- transform-es2015-computed-properties
- transform-es2015-destructuring
- transform-es2015-duplicate-keys
- transform-es2015-for-of
- transform-es2015-function-name
- transform-es2015-literals
- transform-es2015-modules-commonjs
- transform-es2015-object-super
- transform-es2015-parameters
- transform-es2015-shorthand-properties
- transform-es2015-spread
- transform-es2015-sticky-regex
- transform-es2015-template-literals
- transform-es2015-typeof-symbol
- transform-es2015-unicode-regex
- transform-regenerator
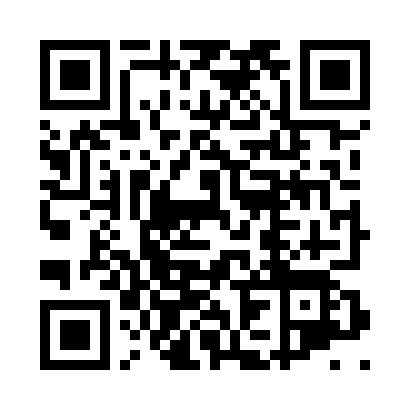
https://slides.com/alexeykosinski/just-do-it
https://goo.gl/npb2t1


?
Just Do It EcmaScript 6
By Alexey Kosinski
Just Do It EcmaScript 6
ES6
- 2,781