Monads
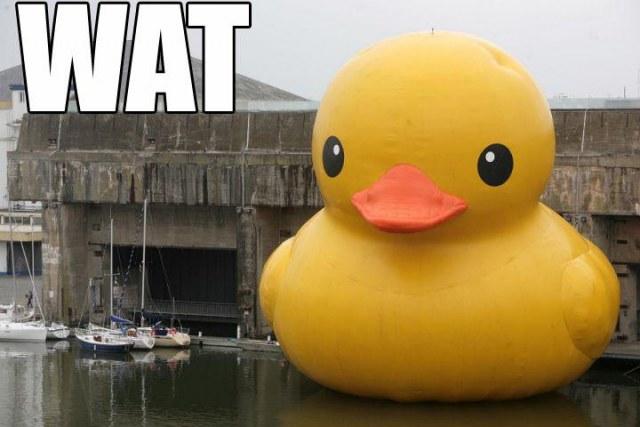
Prerequisites
-
Some kind of idea of Haskell syntax
-
Haskell Platform installed
- Platform requires Xcode on Mac
-
Clone: git@github.com:paasar/Haskell-intro.git
Solution to a problem that was not
1990 - A committee formed by Simon Peyton-Jones, Paul Hudak, Philip Wadler, Ashton Kutcher, and People for the Ethical Treatment of Animals creates Haskell, a pure, non-strict, functional language. Haskell gets some resistance due to the complexity of using monads to control side effects. Wadler tries to appease critics by explaining that "a monad is a monoid in the category of endofunctors, what's the problem?"
-
Monads were and are a solution to a problem that didn't exist before pure code was introduced.
- With monads you can manage unpure operations like IO in otherwise pure code.
-
However, monads are useful in other things and also outside functional programming.
You may have used monads already
Java (QueryDSL)
List result = query.from(customer) //customer: Domain object
.where(customer.lastName.like("A%"), customer.active.eq(true)) //BooleanExpression x2
.orderBy(customer.lastName.asc(), customer.firstName.desc()) //OrderSpecifier x2
.list(customer);
JPAQuery qFrom = query.from(nn);
JPAQuery qFromWhere = qFrom.where(mm);
JPAQuery qFromWhereOrder = qFromWhere.orderBy(oo);
List result = qFromWhereOrder.list(customer);
JavaScript (jQuery)
$("span").fadeIn("slow").text("Alert!");
Monad is...
-
Context
-
Wrapper
-
A way to chain functions in a context
- Very abstract
- State
- Maybe
- Future
- IO
- you name it
Monadic laws
1.
The first monadic law is that a monad is a wrapper around another type. In Haskell, one has the IO String type, which is returned from functions that read from files, console input, or system calls – IO is a monad that wraps the String data type.
2.
The second monadic law is just as simple: all monads must have a function to wrap themselves around other data types.Haskell refers to this as a type constructor – a function that takes some data and wraps that data inside a new type. (jQuery’s type constructor is its parentheses.)
3.
The third monadic law, and the only one that’s even remotely complicated, is that all monads must be able to feed the value or values that they wrap into another function, as long as that function eventually returns a monad.
fadeIn(), text(), and all the other chainable functions are examples of this – they take the elements given inside the jQuery object, perform their function on them, then rewraps them back into the jQuery object and returns them.
Haskell syntax
module Main where main :: IO () main = putStrLn "Hello, World!" -- Type annotation (optional) factorial :: Integer -> Integer -- Using recursion factorial 0 = 1 factorial n | n > 0 = n * factorial (n - 1)
| otherwise = error "No negs plz." -- Using fold (implements product) factorial' n = foldl1 (*) [1..n]
data TrafficLight = Red | Yellow | Green
type Name = String
data Person = Person { firstName :: Name , lastName :: Name , age :: Int } deriving Show
More Haskell syntax
-- class is kind of like interface in Java
class YesNo a where
yesno :: a -> Bool
-- instances are the implementing part for some type
instance YesNo Int where
yesno 0 = False
yesno _ = True
instance YesNo [a] where
yesno [] = False
yesno _ = True
Monad in Haskell code
class Monad m where -- chain, bind (>>=) :: m a -> (a -> m b) -> m b -- inject, wrapper, pure return :: a -> m a
foo :: (Monad m) => Int -> m Int
bar :: (Monad m) => Int -> m Bool
return 1 >>= foo >>= bar
continued
-- With 'concrete' State monad
foo' :: Int -> MyState Int
foo' x = do
put x
return x + x
bar' :: Int -> MyState Bool
bar' y = do
xFromState <- get
return y * xFromState > 10
stuff :: MyState Bool
stuff = return 1 >>= foo' >>= bar'
doStuff :: MyState Bool
doStuff = do
x <- foo' 1
z <- bar' x
return z
-- foo' 1 --> MyState 2 (1) --> bar' 2 --> MyState False (1)
References & links
- http://learnyouahaskell.com/
-
http://importantshock.wordpress.com/2009/01/18/jquery-is-a-monad/
-
http://strongtyped.blogspot.fi/2010/01/monad-non-tutorial.html
-
Crockford on Monads (~1 h)
http://www.youtube.com/watch?v=dkZFtimgAcM
- http://www.querydsl.com
- http://james-iry.blogspot.co.uk/2009/05/brief-incomplete-and-mostly-wrong.html
Monads
By Ari Paasonen
Monads
- 2,629