Arrays
The Humble Array
The most powerful datastructure
Array.prototype
♥'s you
Where shared methods live.
- [forEach, map, reduce, filter, etc. ]
Array.prototype is extensible
Let's make a method
Array.prototype.flatten
COol
So if we need more stuff, we can add it!
But please don't.
Because that is called A Monkey Patch
And is generally frowned upon in most communities.

But we get some rad stuff
for Free!
- Array.prototype.concat()
- Array.prototype.every()
- Array.prototype.filter()
- Array.prototype.find()
- Array.prototype.forEach()
- Array.prototype.indexOf()
- Array.prototype.join()
- Array.prototype.lastIndexOf()
- Array.prototype.map()
Array.prototype.pop()Array.prototype.push()
- Array.prototype.reduce()
- Array.prototype.reduceRight()
- Array.prototype.reverse()
Array.prototype.shift()- Array.prototype.slice()
- Array.prototype.some()
Array.prototype.sort()Array.prototype.splice()- Array.prototype.toString()
Array.prototype.unshift()
var a = new array();
var a = [];
THINK SMART, THINK JSMART.
var a = new array();
new Array(5, 4, 3, 2, 1);
Y?
new Array(5);
- JavaScript is dynamic, so memory isn't allocated by the author.
- Array literals are more performant than constructors.
- You can initialize with a single value. The one initialization case you can't do with the constructor.
YOu don't gain anything
The Array reference can be overridden.
YOu Could Lose Everything

oh noes!
Anything you can do with a single thing...
...You can easily do with lots of them
Arrays are Super usefull
You only really need to know a handful of methods
forEach map filter
reduce
includes find concat
slice
Slice
Sweeny Todd Would Be Proud
const bitOHoney = aSweetArray.slice(1, 4)
Create a copy of a subset of an array
the index of the beginning of the slice (inclusive)
returns a new array that contains a the subset
the index of the end of the slice (exclusive)
const bitOHoney = aSweetArray.slice(-1)
negative numbers start from the end of the array
leave off the second argument to slice through the last item in the array
Slice
Playing with knives

Concat
As in Dog or just anti-pet generally?
const longer = someArray.concat(a, [b, c], [d], ...z)
Append items or arrays of items to an array.
append single items
returns a new array composed of all the arguments
and/or arrays
Concat
Dog Park
includes
Are you "with it"
isInArray = someArray.includes(value)
returns true if the item exists in the array, false otherwise
Looks for the existence of a particular element in an array.
the particular value to look for
Includes
Fornever Alone
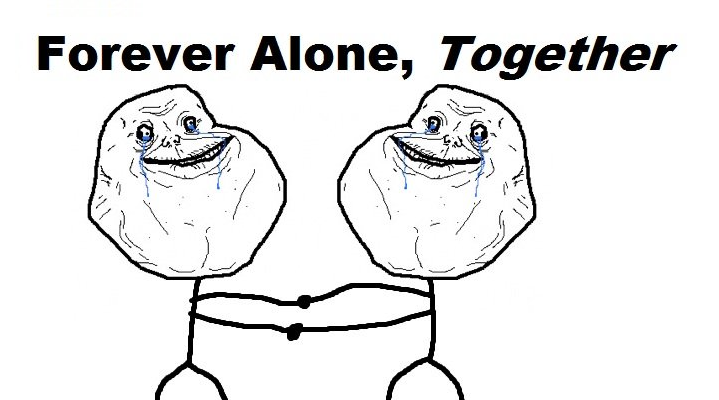
Find
I have spoke with the tongue of angels
I have held the hand of a devil
foundItem = someArray.find((item, index, array) =>
some_condition ? true : false
return whether the item meets the condition we care about
Returns the first element to match the given condition
where have we seen this signature before?
returns the first item found, or null if no items match.
Find
I finally found what I'm Looking For
Filter
Hey Man, Nice Shot
newArray = someArray
.filter((item, index, arr ) => true || false)
return `true` to include in the new array
`filter` an array to only those items that match a given condition.
`false` ta-not-to
Filter
forEach
Don't even bother with for loops
someArray.forEach(( item, index, arr ) => {
//do stuff;
return item; //Nope!
});
A function executed for every element in the array
The function takes three arguments:
- the current item
- the index of that item
- the whole array
forEach ignores any return values.
The itterables of the array are fixed at execution of the forEach
ForEach
Ah, skip it.

Map
Map it like you're Ptolemy
newArray = someArray.map(( item, index, arr ) => {
//do stuff;
return item; //Huzzah!
});
return the item that will be mapped to the new array
The `map` method creates a new array. Each item in the new array is derived from the corresponding item in the original array.
MAP
a -> b
Reduce
out of many, one.
value = someArray.reduce((incoming, current ) => {
return outgoing;
}, optionalInitialValue);
return the value that will used as the first argument in the next iteration.
Take an array and `reduce` it to a single value.
An optional initial value can be passed into the reduce function as the incoming argument to the iterator function. If not included, the first element is used.
The return value from the previous iteration
The current item in the iteration
Reduce
array -> number
Reduce
array -> object
Reduce
Remake me
Armed with these tools, you are now prepared to enter the world of Functional Programing.
dataIn => dataOut
Pure Functions
Arrays: Part 1
By Cory Brown
Arrays: Part 1
Arrays in JavaScript are incredibly powerful. Almost any data structure can be emulated by a JavaScript Array. We'll look at some of the really great things you can do with an Array.
- 1,577