getting git
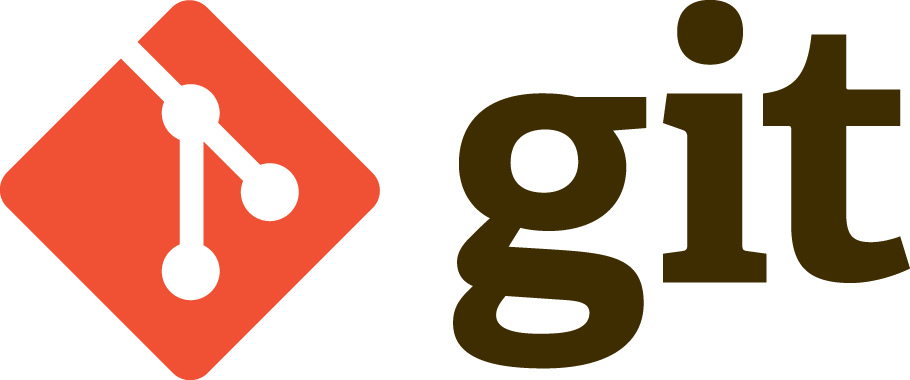
Basics
• Decentralized
• Fast
• Flexible
• Fast
• Flexible
Local VCS
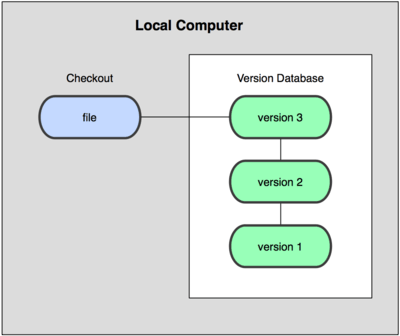
(rcs)
Centralized VCS
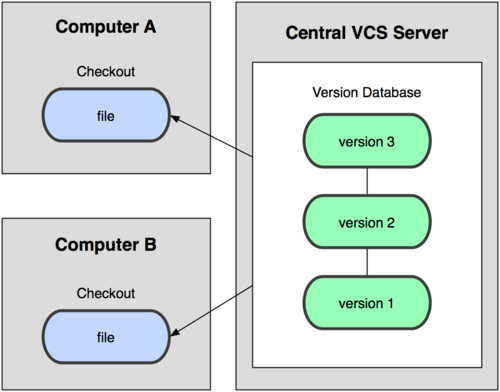
(cvs, svn)
Yay
• Access control• Central Backup
Nay
• Single point of failure• Painful merges
Decentralized VCS (git)
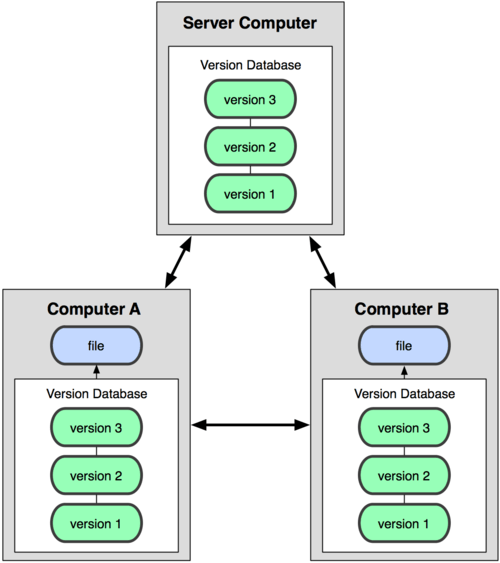
(git, mercurial, bazaar, bitkeeper, darcs)
Yay
• Every clone is a fork
• Every clone is a backup
• No connectivity issues
• Cheap branching
• Fast. Really!
• Rewriting history is possible and easy
• Flexible workflows for development, review & deployment
Nay
• Not good for large binary files (workaround: LFS)
• File permissions are not tracked (except +x)
Git design goals
• Speed
• Simple design
• Strong support for thousands of parallel branches
• Fully distributed
• Able to handle larges projects like Linux kernel effectively
• Ensure integrity
https://youtu.be/4XpnKHJAok8
Tracking Changes
(most VCS)

Changes to files are tracked.
Tracking Snapshots
(git)

Conceptually, git tracks snapshots.
(On the storage level, deduplicated deltas are used.)
Git is a file system
Git thinks of its data more like a set of snapshots of a mini filesystem.
Git has integrity
• Everything is checksummed
• References are SHA-1
• If you check out the same commit ID,
you have the same content.
24b9da6552252987aa493b52f8696cd6d3b00373
Git doesn't delete
• Git generally only adds data
• If you mess up, you can usually recover your stuff
• Recovery can be tricky though
The three states
- Modified
- Staged
- Committed
Workflow

The git directory
• Located at .git/
• Contains entire history
The working directory
• A single checkout from a Git repository
The Staging Area
• Contained in a file
• Tracks what will go into the next commit
• AKA "the index"
File Status Lifecycle

Configuration
Identity
$ git config --global user.name "John Doe"
$ git config --global user.email johndoe@example.com
Editor
$ git config --global core.editor vim
Colors
$ git config --global color.ui true
Creating a Repository
$ git init
Cloning a Repository
$ git clone https://github.com/dbrgn/fahrplan
Creating a Commit
Specific changes:
$ git add *.py
$ git add README.rst
$ git commit -m 'First commit'
All tracked changes:
$ git commit -am 'First commit'
Removing files
From staging area
$ git rm --cached file.py
From index and file system
$ git rm file.py
Moving files
Git tracks content, not files. Although there is a move command...
$ git mv file1 file2
...this is the same as...
$ mv file1 file2
$ git rm file1
$ git add file2
Show Status
$ git status
Show log
Entire (paged)
$ git log
Date filtering
$ git log --since=2.weeks
$ git log --since="2 years 1 day 3 minutes ago"
SHOW Diffs
Unstaged changes
$
git diff
Staged changes
$
git diff --cached
Relative to specific revision
$
git diff 1776f5
$
git diff
HEAD^
$ git diff awesomebranch
Show Commits
Last commit
$ git show
Specific commit
$ git show 1776f5
$ git show HEAD^
Undoing Things
Overwrite last commit
$ git commit --amend
Unstage staged file
$ git reset HEAD file.py
Unmodify modified file
$ git checkout file.py
.gitignore
$ cat .gitignore
*.pyc
*.swp
/build/ /doc/[abc]*.txt
.pypirc
*.egg-info
• Blank lines or lines starting with # are ignored
• Standard glob patterns work
• End pattern with slash (/) to specify a directory
• Negate pattern with exclamation point (!)
• Subdirectories can have their own .gitignore files
Remotes
• Other clones of the same repository
• Can be local (another checkout) or remote (coworker, central server)
• There are default remotes for push and pull
$ git remote -v
origin git://github.com/schacon/ticgit.git (fetch)
origin git://github.com/schacon/ticgit.git (push)
Push to remote
Without default
$ git push <remote> <rbranch>
Setting A default
$ git push -u <remote> <rbranch>
Then...
$ git push
Pull from Remote
Fetch & Merge
$ git pull [<remote> <rbranch>]
Fetch & Rebase
$ git pull --rebase [<remote> <rbranch>]
-> Rebasing modifies commits!
Create Tags
Lightweight tags
$ git tag v0.1.0
Annotated tags
$ git tag -a v0.1.0 -m 'Version 0.1.0'
GPG-Signed tags
$ git tag -s v0.1.0 -m 'Signed version 0.1.0'
$ git tag -v v0.1.0
Branches
Branches are "Pointers" to commits.
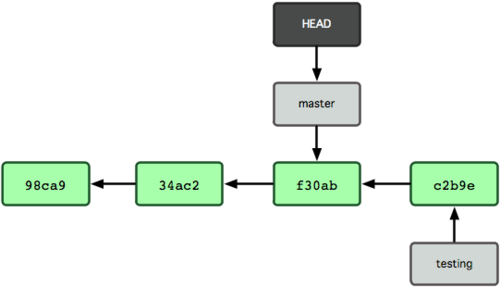
Diverging Branches
Branches can diverge.
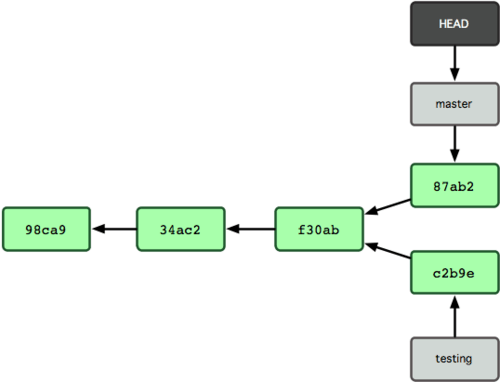
Merging Branches
Branches can be merged.

Merge conflicts
• Different auto-merge strategies
(fast-forward, 3 way, etc...)
• If it fails, fix by hand
$ git merge iss53 Auto-merging index.html CONFLICT (content): Merge conflict in index.html Automatic merge failed; fix conflicts and then commit the result.
• Then mark as resolved and trigger merge commit
$ git add index.html
$ git commit
Usually painless

Rebasing
• Linear alternative to merging
• Rewrites tree! Never rebase published code!
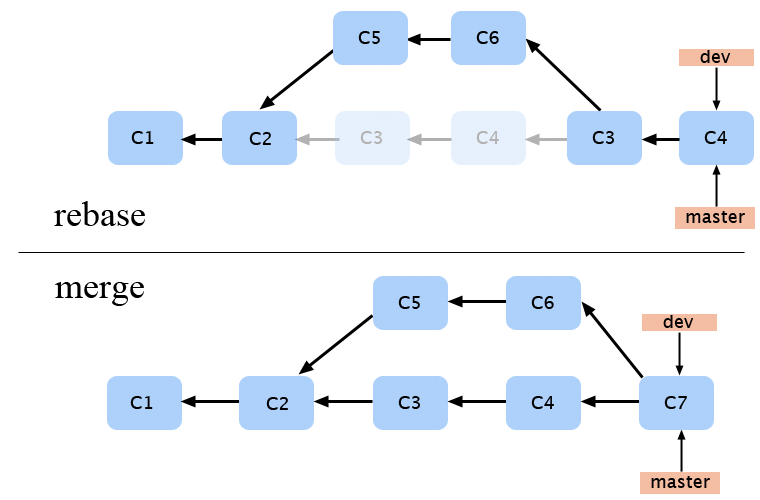
Branch Management
Create new branch
$ git branch iss53
Switch Branch
$ git checkout iss53
Create & Switch
$ git checkout -b iss53
Delete Branch
$ git branch -d iss53
Branch Management
Show local Branches
$ git branch
iss53
* master
testing
SHOW LOCAL + remote BRANCHES
$ git branch
iss53
* master
testing remotes/origin/master remotes/origin/testing remotes/origin/iss32 remotes/origin/iss48
Branch Management
Merged branches
$ git branch --merged
iss53
* master
Unmerged branches
$ git branch --no-merged
testing
Stashing
Move changes to a separate "stash".
$ git stash
$ git stash pop
$ git stash list
$ git stash apply
$ git stash drop
$ git stash clear
Common Workflow
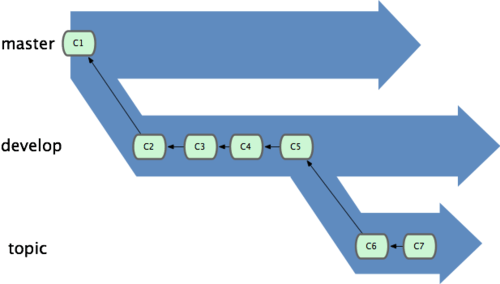
Workflow 1
• Only commit stable code to master
• Commit unstable to develop
• Deploy hotfixes to master
Workflow 2
• Commit development code to master
• Create tags for stable releases
• Branch from stable tag for hotfixes
Topic Branches
• AKA feature branches
• For each feature, create a topic branch
• Merge early, merge often
• If desired, squash commits
Legit
Easier workflow for Git. Looks legit.

Advanced Git
Autocompletion
$ echo "source ~/.git-completion.bash" >> ~/.bashrc
Aliases
$ git config --global alias.co checkout
$ git co HEAD
Advanced Git
Partial add
$ git add -p file.py
Bisecting
$ git bisect start
$ git bisect bad
$ git bisect good v1.0
Advanced Git
Rewrite commits
$ git filter-branch --env-filter
'if [ $GIT_AUTHOR_EMAIL = gezuru@gmail.com ]; then GIT_AUTHOR_EMAIL=db@threema.ch; fi; export GIT_AUTHOR_EMAIL'
Resources
• "Pro Git" Book http://git-scm.com/book
• Git reference http://git-scm.com/docs
• Cheat sheet http://wbrp.li/14Dqrgh
• Use git-svn-bridge http://wbrp.li/XnpaUp
• Commands git vs svn http://wbrp.li/YCw4bb
Now Git to work!

getting git
By Danilo Bargen
getting git
- 3,643