Basic Data Structure
C++ Standard Template Library
NCTU_Valkyrie
Linked List
[lɪŋkt lɪst]
-
分散式記憶體空間
-
search O(n)
-
insert O(1)
-
delete O(1)
value
next
value
next
value
next
value
next
head
NULL
insert
value
next
value
next
head
NULL
value
next
new
value
next
value
next
head
NULL
value
next
new
value
next
value
next
head
NULL
value
next
new
delete
value
next
value
next
head
NULL
value
next
del
value
next
value
next
head
NULL
value
next
del
value
next
value
next
head
NULL
Doubly linked list
只差在他有指回去的箭頭
其他都和linked list一樣
value
next
head
NULL
pre
value
next
pre
value
next
pre
value
next
pre
NULL
Array
Re:Zeroから始める
Array生活
- Bullet One
- Bullet Two
- Bullet Three
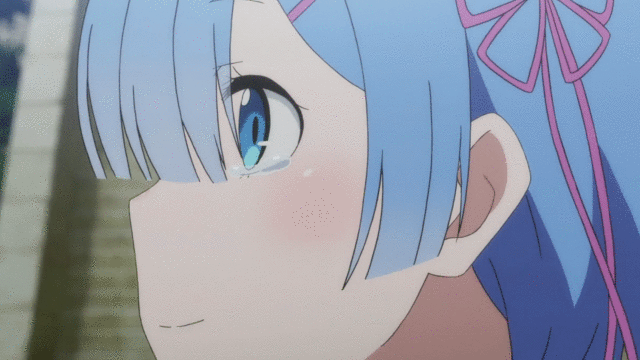
Re:Zeroから始める
Array生活
Array 是從0開始
Array 是從0開始
Array 是從0開始
因為很重要所以要說三次
-
連續記憶體空間
-
random access
-
insert O(n)
-
delete O(n)
-
modify O(1)
-
access O(1)
insert & delete
insert
delete
-
search sorted O(log n)
-
search unsorted O(n)
Stack
-
FILO
-
push O(1)
-
pop O(1)
-
top O(1)
push & pop
push
stack
stack
pop
top
top
Recursive

Queue
-
FIFO
-
push O(1)
-
pop O(1)
-
front O(1)
-
back O(1)
push
push
front
front
back
back
pop
pop
back
back
front
front
Graph
定義
G : 圖
V : 點集合
E : 邊集合
G = {E U V}
種類
-
undirected vs directed
-
cyclic vs acyclic
-
simple vs multigraph


undirected vs directed
acyclic vs cyclic

simple vs multi

Tree
-
connected
-
simple
-
acyclic

特例
-
complete
-
binary

Heap
-
complete binary tree
-
insert O(log n)
-
pop O(log n)
insert
5, 4, 6, 3, 8, 7
5
root
5
root
4
5
root
4
6
6
root
4
5
6
root
4
5
4
3
6
root
4
5
4
3
8
6
root
4
5
8
3
4
8
root
4
5
6
3
4
8
root
4
5
6
3
4
7
8
root
4
7
6
3
4
5
-
max heap
-
min heap
C++ STL
using namespace std;
C++ Reference
http://www.cplusplus.com/reference/
template parameter
template<type T>
array
- Sequence
- Contiguous Storage
- Fixed-size Aggregate
C
- T a[size]
C++
- #include<array>
- array<T, size> a
- a.begin()
- a.end()
- a.at(index)
- a[index]
string
C
- char s[size]
- 字元陣列(array)
- 空字元'\0'代表字串終止
C++
- string s
- 長度可變
- 會自動補'\0'
- s.size()
- s[index]
- s + s'
- s < s'
vector
- Sequence
- Dynamic Array
- Allocator-aware
#include<vector>
- vector<T> v
- v.begin()
- v.end()
- v.size()
- v.empty()
- v[index]
- v.front()
- v.back()
- v.push_back((T) object)
- v.pop_back()
- v.clear()
- push_back()、pop_back()、front()、back()是O(1)
- 可以做到所有stack可以做到的事情,
又效率比stack高一點,所以通常會拿來取代stack。
deque
[dεk]
Double Ended Queue
#include<deque>
- deque<T> d
- d.empty()
- d.front()
- d.back()
- d.push_back((T) object)
- d.push_front((T) object)
- d.pop_back()
- d.pop_front()
- d.clear()
- front()、back()、push_back()、push_front()、pop_back()、pop_front()複雜度是O(1)
stack
- 底層實作是用deque
- 速度比vector慢,通常會直接用vector取代它
所以以下跳過不講。(幫QQ
queue
#include<queue>
- queue<T> q
- q.empty()
- q.front()
- q.back()
- q.push((T) object)
- q.pop()
priority_queue
- max heap結構
#include<queue>
- priority_queue<T> pq
- pq.empty()
- pq.top()
- pq.push((T) object)
- pq.pop()
- pq.top()複雜度O(1)
- pq.push()、pq.pop()複雜度O(log n)
iterator
more than pointer
- 疊代器
- 剛剛講到的begin(), end()都是回傳iterator

- 用法
- Container<T>::iterator it;
- *it 取出iterator所指的元素
- 支援++、--、equation
- it1 == it2 詢問 it1 和 it2 指向的元素是否相同
- it++ 指向下一個元素
- it-- 指向前一個元素
- Random Access (vector, deque):
- it + 5 指向it後面第五個元素(不檢查邊界)
- last - first 兩者的index差
用iterator走完整個Container
vector<int> v;
vector<int>::iterator it;
//用iterator走完整個vector
for(it = v.begin(); it != v.end(); it++){
cout << *it << endl;
}
//用index走完整個vector
for(int i = 0; i < v.size(); i++){
cout << v[i] << endl;
}
- 和指標的差別:
- iterator的順序和記憶體位址順序無關
- 就算STL Container元素記憶體位置是分散的,iterator還是能夠輕易地指向下一個的元素
begin() : 指向 Container 中的第一個元素
end() : 指向 Container 的結尾(並不是最後一個元素)
stl_function(first, last)
前閉後開區間 [first,last)
需要區間資料的 STL 函數都是

list
#include <list>
- 就是 Linked List
#include <list>
- list<T> l;
- l.begin()
- l.end()
- l.push_front((T) object)
- l.push_back((T) object)
- l.pop_front()
- l.pop_back()
- l.size()
- l.insert(iterator, (T) object)
- l.erase(iterator)
- l.erase(first, last)
pair
將兩個元素綁在一起的資料結構
- pair<T1, T2> p
- p.first Type:T1
- p.second Type:T2
#include <utility>
make_pair
- make_pair(var1, var2)
- 自動生出與參數相符的pair型別
- make_pair("", 0)的型別是pair<string, int>
Pair 比大小
- pair<T1, T2> p1, p2;
- p1 < p2;
- T1 和 T2 的 operator<() 要定義好
- 先比 first 再比 second
map
鍵值對(Key-Value pair)
用Key來尋找對應的Value
將Key map 到 Value
Key唯一,不會重複存在
實作:
一個神奇的自平衡二元搜索樹(通常以紅黑樹實作)
高度為O(log N)
#include <map>
- map<Key, Value> m;
- m[Key] = Value;
- m.insert(pair<Key, Value>)
- m.begin()
- m.end()
- m.erase(Key)
- m.erase(first, last)
- m.find(Key)
- m.size()
- m.clear()
- m.empty()
m[Key] = Value 、 m.insert() 複雜度O(log N)
m.find() 複雜度O(log N)
About Map iterator
- *it 的Type是pair<Key, Value>
- map的底層會以Key的大小排序
- 迭代iterator只是從樹的最左邊走到最右邊
- 所以迭代iterator時Key和插入順序無關,並以大小排序
- m.find(Key) 回傳該Key所代表元素的iterator
- 如果m.find(Key) == m.end()
- 代表 Key 沒有存於 map 中
- map 的 for loop
map<int, int> m;
map<int, int>::iterator it;
for(it = m.begin(); it != m.end(); it++)
{
cout << (*it).first << (*it).second << endl;
}
尋找 map 中的元素
map<int, int> m;
- if(m[key] != 0) // bad
m[key]會在沒有key自動生出新的元素
導致map內的元素個數增加
- if(m.find(key) != m.end()) // good
m.find並不會增加map內的元素個數
map 的使用時機
- Key範圍太大或有負值時
- Ex. -2147483648 <= Key <= 2147483647
- Key不是整數型別時
- Ex. 紀錄 String 出現的次數
- 需要多次刪除元素時
set
沒有Value的map
僅插入、刪除Key 檢查Key是否存在
Key唯一,不會重複存在
#include <set>
set<T> s;
s.insert((T) object)
s.erase((T) object)
s.erase(first, last)
s.begin()
s.end()
s.size()
s.empty()
s.clear()
- s.insert() 複雜度 O(log N)
- s.erase() 複雜度 O(log N)
- 和 map 一樣,迭代iterator時
- *it和插入順序無關,Key由小到大排序
Set 的使用時機
- 需檢查元素是否存在時
- 刪除非頭尾端元素時
Unordered map/set
- 操作和 map/set 幾乎一樣
- insert/erase O(1)
- iterator 順序跟插入順序、Key大小無關
#include <algorithm>
sort
- 可使用 sort 的 Container : vector、deque
- sort(first, last);
- 排序整個vector<T> v
- sort(v.begin(), v.end())
Compared-Based Sort
複雜度 O(N log N)
reverse
反轉 Container 內的所有元素
可使用 reverse 的 Container : vector, deque, string, list
reverse(first, last);
反轉字串string str
reverse(str.begin(), str.end());
複雜度 O(N)
lower_bound
lower_bound(first, last, (T) object)
限制:Container 內的元素必須有序,使用才有意義
回傳第一個大於等於輸入元素的iterator
如果沒有,則回傳end()
vector<int> v;
sort(v.begin(), v.end());
auto it = lower_bound(v.begin(), v.end(), 10);
複雜度 O(log N)
upper_bound
回傳第一個大於輸入元素的iterator
如果沒有,則回傳end()
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
int main()
{
cin.tie(0);
cin.sync_with_stdio(0);
int n, q, now;
cin >> n;
vector<int> v;
for(int i = 0; i < n; ++i){
cin >> now;
v.push_back(now);
}
sort(v.begin(), v.end());
cin >> q;
while(q--){
cin >> now;
cout << upper_bound(v.begin(), v.end(), now) - v.begin() << endl;
}
}
Advanced
Array Representation of Binary Tree

General Tree Representation
template<class T>
struct Node<T>
{
T value;
struct Node<T>* parent;
vector<struct Node<T>*> children;
};
Graph Representation
Adjacency Matrix

Adjacency List

Depth First Search
1
2
3
4
5
6
7
Stack
1
2
3
4
5
6
7
1
Stack
1(1)
2
3
4
5
6
7
2
Stack
3
1(1)
2
3(2)
4
5
6
7
2
Stack
6
7
1(1)
2
3(2)
4
5
6
7(3)
2
Stack
6
1(1)
2
3(2)
4
5
6(4)
7(3)
2
Stack
1(1)
2(5)
3(2)
4
5
6(4)
7(3)
4
Stack
5
1(1)
2(5)
3(2)
4
5(6)
6(4)
7(3)
4
Stack
1(1)
2(5)
3(2)
4(7)
5(6)
6(4)
7(3)
Stack
Breadth First Search
1
2
3
4
5
6
7
Queue
1
1(1)
2
3
4
5
6
7
Queue
2
3
1(1)
2(2)
3
4
5
6
7
Queue
3
4
5
1(1)
2(2)
3(3)
4
5
6
7
Queue
4
5
6
7
1(1)
2(2)
3(3)
4(4)
5
6
7
Queue
5
6
7
1(1)
2(2)
3(3)
4(4)
5(5)
6
7
Queue
6
7
1(1)
2(2)
3(3)
4(4)
5(5)
6(6)
7
Queue
7
1(1)
2(2)
3(3)
4(4)
5(5)
6(6)
7(7)
Queue
PCCA Winter Camp 2018 Day 2
By deror1869107
PCCA Winter Camp 2018 Day 2
- 1,010