Angular 2
+
TypeScript
=
Title Text
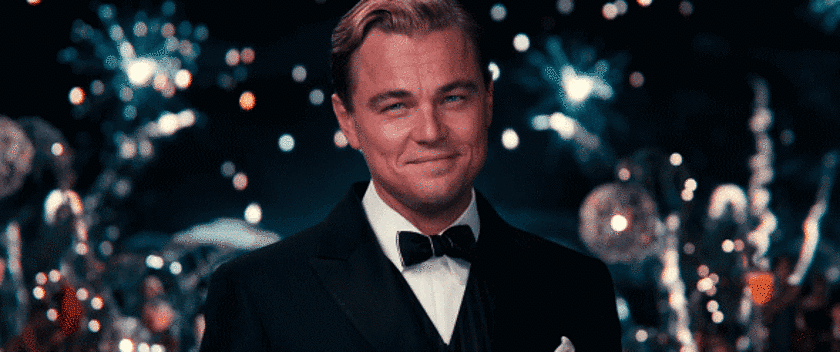
Building Blocks
ng2

OUR PARTNERS
Agenda:
- What is Angular 2
- Advantages of Angular 2
- Main Building Blocks
- Simple Code Snippets
- Recap :)

mobile and desktop
mobile and
desktop
Advantages :
- SPEED AND PERFORMANCE
- SIMPLE AND EXPRESSIVE
- LEGACY BROWSER SUPPORT
- CROSS PLATFORM SUPPORT
- FLEXIBLE DEVELOPMENT
- EASY MIGRATION FROM 1 TO 2
- NEW COOL ROUTER
- DEPENDENCY INJECTION
- OTHERS [UNDER DEVELOPMENT]
Quick ng2 Architecture Overview
Component
- Main building block
- Top level app components
- Containing many children sub-components
- Containing encapsulated View + Class
- Event handling and updates to the View
hello-world.ts
import {Component} from 'angular2/core';
@Component({
selector: 'hello-world',
template: `<h1>Hello, {{target}}!</h1>`,
styles: [`
h1 {
font-size: 1.2em;
}
`]
})
export class HelloWorldCmp {
target: string;
constructor() {
this.target = 'World';
}
}
ES2015 Modules & Typescript
- Building modular applications
- A file containing js code
- No more IIFEs & callbacks for modules
- import
- export
Directives
- Visualisation instructions
- Adding dynamic template content
- Structural - add or remove existing DOM element
- Attribute - change the visualisation of already existing DOM element
- Built-in directives - *ngFor, *ngIf, *ngSwitch, etc.
highlight.directive.ts
import {Renderer, Directive, ElementRef, Input} from 'angular2/core';
@Directive({
selector: '[myHighlight]'
})
export class HighlightDirective {
constructor(el: ElementRef, renderer: Renderer) {
renderer.setElementStyle(el.nativeElement, 'backgroundColor', 'yellow');
}
}
Services
- A class with exact purpose
- Contains business and application logic
- HTTP requests and server communication
- Components consume services
- Components depend on services they consume
name_lists.service.ts
export class NameList {
names = ['Dijkstra', 'Knuth', 'Turing', 'Hopper'];
get(): string[] {
return this.names;
}
add(value: string): void {
this.names.push(value);
}
}
Dependecy Injection
- It's a coding pattern in which a class receives its dependencies from external sources rather than creating them itself.
- An isolated component
- Decoupled code and testing boost
- Injector - The injector object that exposes APIs to us to create instances of dependencies.
- Provider - A provider is like a recipe that tells the injector how to create an instance of a dependency. A provider takes a token and maps that to a factory function that creates an object.
- Dependency - A dependency is the type of the created object
di.ts
import {provide, Injector, Injectable} from 'angular2/core';
class Shampoo {}
@Injectable()
class CosmeticShop {
constructor(private shampoo: Shampoo) {
}
}
let injector = Injector.resolveAndCreate([
provide(Shampoo, {useValue: new Shampoo()}),
CosmeticShop
]);
injector.get(CosmeticShop);
Pipes
- A service that filters data
- Transofrms values in wanted output for better UX
- Chaning pipes in potentially useful combinations
- New pipes that give us special functionality, async pipe
- Built-in pipes - DatePipe, UpperCasePipe, LowerCasePipe, CurrencyPipe, etc.
- Custom Pipe example
letterTransform.pipe.ts
home.ts
import {Pipe} from 'angular2/core';
@Pipe({
name: 'myCustomPipe'
})
export class myCustomPipe {
transform(): string {
// letter formatting
}
}
import {Component} from 'angular2/core';
import {myCustomPipe} from './myCustomPipe.pipe';
@Component({
selector: 'demo-app',
template: '<p>{{longText | myCustomPipe}}</p>',
pipes: [myCustomPipe]
})
export class App {
constructor() {
let longText = 'BANANA-BAANANABANANABANANA-BANANA';
}
}
Basic Routing
- Router is an optional service that is not part of ng 2 core
- <script src="node_modules/angular2/bundles/router.dev.js"></script>
- Navigation from one view to another
- Watching for user interactions with the view
- Searching for RouteDefinition for presenting certain view
- RouterOutlet directive - where to display the loaded component
app.ts
import {Component} from 'angular2/core';
import {
RouteConfig,
ROUTER_DIRECTIVES
} from 'angular2/router';
import {HomeCmp} from '../../home/components/home';
import {AboutCmp} from '../../about/components/about';
@Component({
selector: 'app',
templateUrl: './app.html',
styleUrls: ['./app.css'],
directives: [ROUTER_DIRECTIVES]
})
@RouteConfig([
{path: '/', component: HomeCmp, name: 'Home'},
{path: '/about', component: AboutCmp, name: 'About'},
])
export class AppCmp {}
app.html
<section>
<nav>
<a [routerLink]="['/Home']">What?</a>
<a [routerLink]="['/About']">About</a>
</nav>
<router-outlet></router-outlet>
</section>

Recap :)
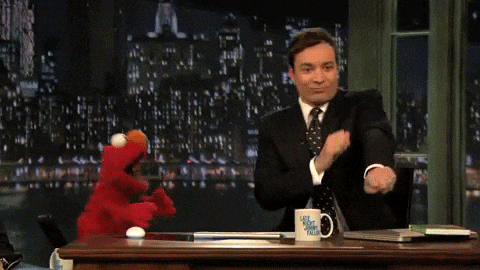
Thank you :)
Angular 2 - Building Blocks
By Elena Gancheva
Angular 2 - Building Blocks
- 3,140