Why Functional Programming Matters
Baishampayan "BG" Ghose
CTO/Co-founder - Helpshift, Inc.

Why Functional Programming Matters
(today)
Baishampayan "BG" Ghose
CTO/Co-founder - Helpshift, Inc.
A Quick Poll
Which programming language do you use at work?
- Java
- Ruby
- Python
- JavaScript
- Any functional language?
Does your code run perfectly the first time?
Yes/No
Why not?
How do we get there?
About Me
- Started my career hacking Lisp
- Want to write correct code that runs fast
- Career polyglot programmer with focus on Functional Programming
- CTO/Co-founder at Helpshift, Inc.
A Brief Interlude

von Neumann's Core Ideas
- Stored program model
- Computation through mutation of memory cells
- Assumes single thread of execution
- Abstractions to distort reality in other cases

And then, reality strikes back...
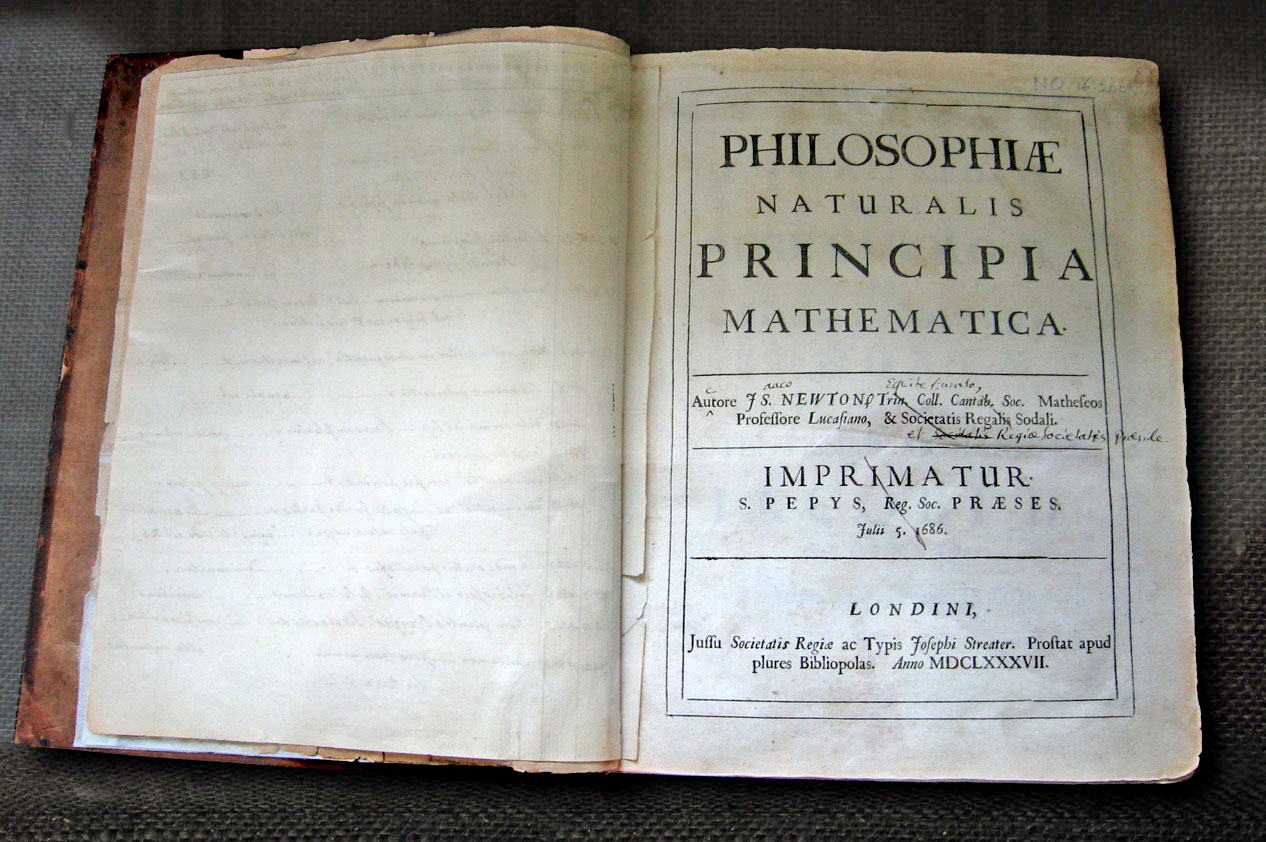
Clearly, we have run out of options
Functional Programming
A Primer
History
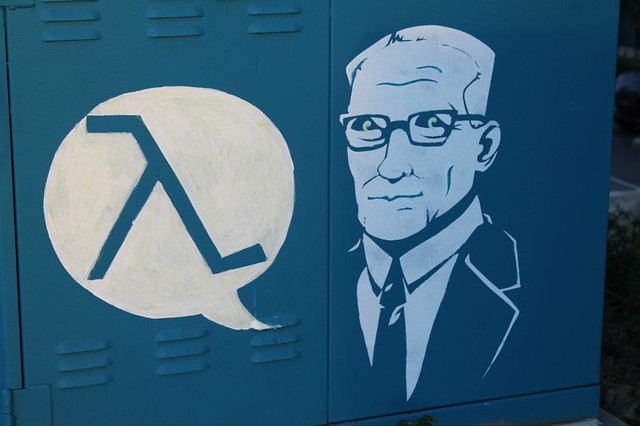
Core Ideas
- Computation based on evaluation of λ-expressions
- Immutability
- First class, and hence higher-order functions
- Sequence transformations instead of mutation of memory cells
- Referential transparency
Immutability
x = [1, 2, 3, 4]; print x; // [1, 2, 3, 4]
y = x.append(5);
print y; // [1, 2, 3, 4, 5] print x; // [1, 2, 3, 4]
Because: Maths!
First Class Functions
def make_adder(x): # close over x return lambda y: x + y add5 = make_adder(5) # I am returning a function here add5(10) # 15
###
["Church","Turing","Gödel"].sort(key=lower)
Sequence Transformation
nums = [77, 45, 2, 98, -12] # square all numbers nums2 = map(square, nums) # [5929, 2025, 4, 9604, 144] # keep only > 1000 print filter(lambda n: n > 1000, nums2)
[5929, 2025, 9604]
Referential Transparency
square(5) == 25 # a pure function
square(5) + square(5) + square(5)
# compiler can optimize this as
25 + 25 + 25
Because: square is "referentially transparent"
Benefits
- Higher levels of abstractions
- Increased modularity
- Lazy evaluation
- Concurrency
- Parallelism
- Memoization
- Testability
- Composability
FP Languages
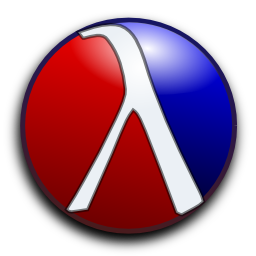
ML

FP Languages



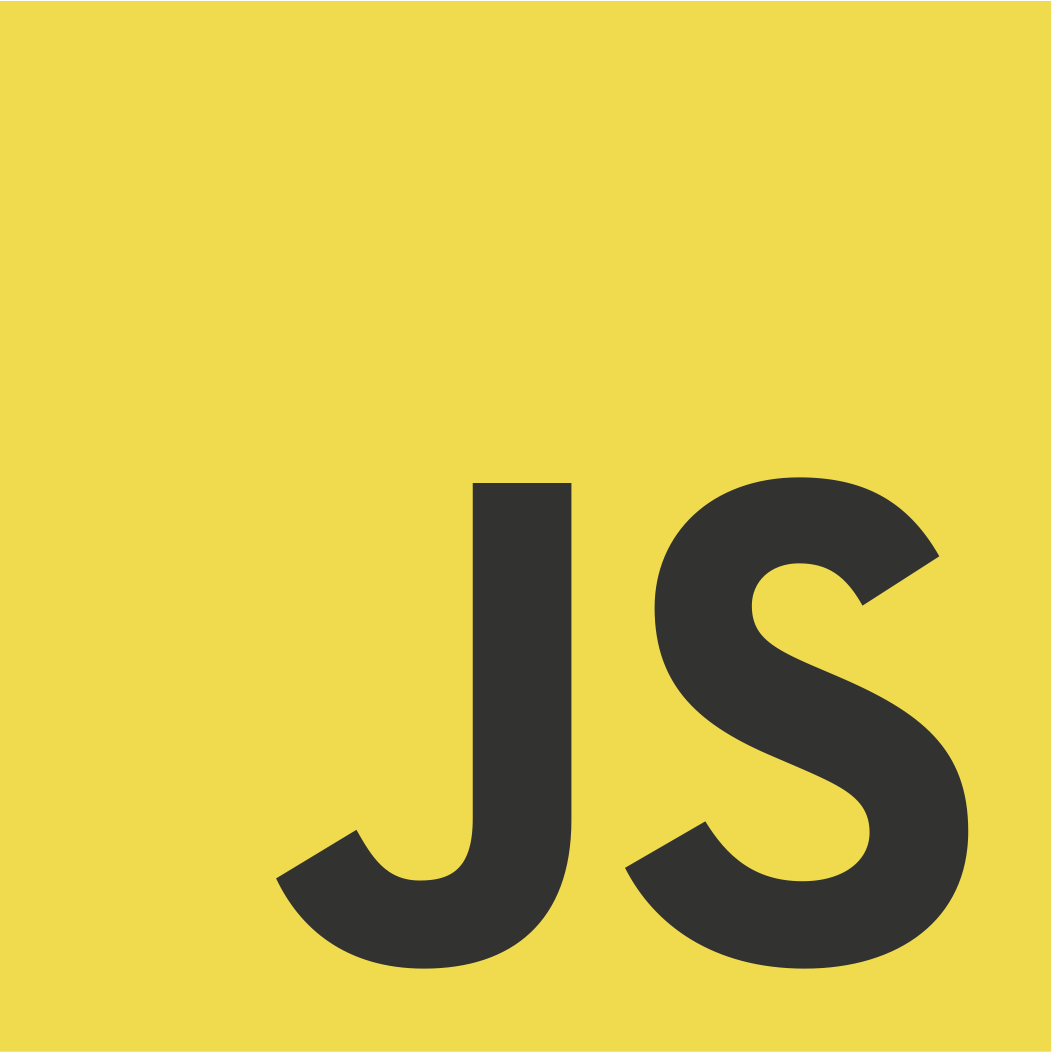
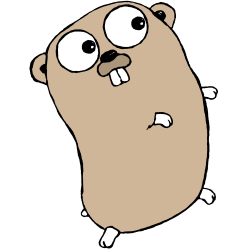
Interesting Ideas That Work Well With FP
- Communicating Sequential Processes
- Generative Testing
- Logic/Relational Programming
- Parsers

Thanks!

We're hiring: www.helpshift.com
Why Functional Programming Matters (Today)
By Baishampayan Ghose
Why Functional Programming Matters (Today)
- 3,842