ReactJS


Quiz








Text
Text
ReactJS
ReactJS in a Nutshell
- The V in MVC
- Declarative
- Everything is a Component
- 1-Way-Dataflow
Components
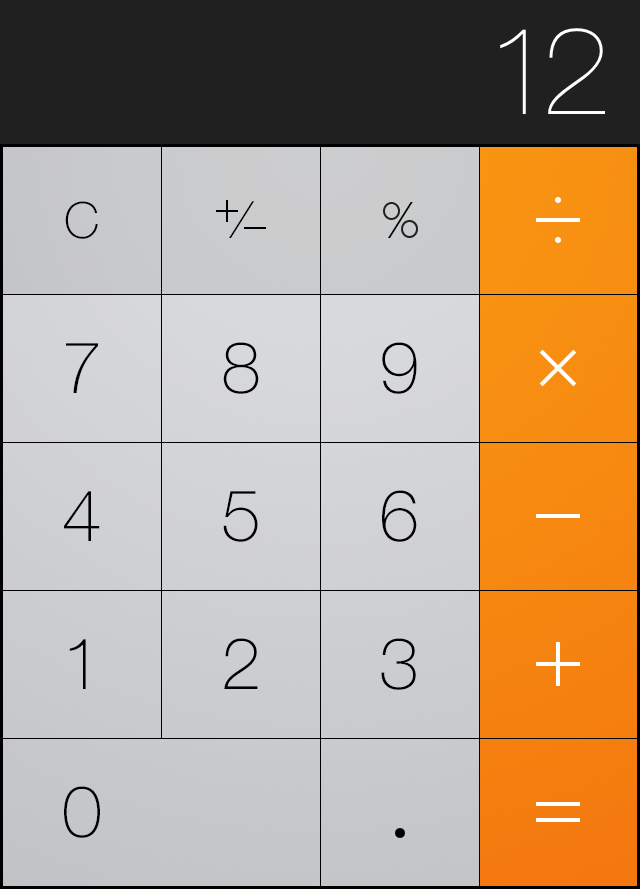
Components

Components

Components

Components
class SearchBar extends React.Component {
render() {
return (
<form>
<input type="text" placeholder="Search..." />
<p>
<input type="checkbox" />
{' '}
Only show products in stock
</p>
</form>
);
}
}
class SearchBar extends React.Component {
render() {
}
}
Components
class FilterableProductTable extends React.Component {
render() {
return (
<div>
<SearchBar />
<ProductTable products={this.props.products} />
</div>
);
}
}
JSX
- Html extension to JavaScript
- Optional
- Can be combined with JS
- Html linting
- Feels like html (but isn't)
State

State
- stuffThatShouldBeForgottenIfTheUserRefreshes
- Belongs to component
- this.state
- Try to have as little state as possible
- Don't duplicate - calculate
State
class HelloState extends React.Component {
constructor() {
this.state = {
count: 0
};
}
render() {
return (
<div>
Count: {this.state.count}
</div>
);
}
}
class HelloState extends React.Component {
constructor() {
this.state = {
count: 0
};
}
increment() {
this.setState({
count: this.state.count + 1
});
}
render() {
return (
<div>
Count: {this.state.count}
<button onClick={this.increment}>Add 1</button>
</div>
);
}
}

// index.html
<script>
function increment() {
let element = $('#count');
let current = element.text();
element.text(parseInt(current) + 1);
}
</script>
<div>
Count: <label id="count">0</label>
<button onclick="increment()">Add 1</button>
</div>
With jQuery...
Props
- stuffThatShouldStayTheSameIfTheUserRefreshes
- this.props
- read only
<todo-item title="Buy milk" color={this.state.defaultColor}>
Should I start using ReactJS?
- Easy to learn
- 1-Way-Dataflow helps to reduce complexity
- Many third party components
- Overhead
- Problematic License
Advanced State Management


Flux
React beyond the Browser
React Native

Server Side Rendering

React beyond React...





- Demo code: https://github.com/haimich/calculator
- Implementation on Codepen: http://codepen.io/mjijackson/pen/xOzyGX
- Very good demo: http://reactfordesigners.com/labs/reactjs-introduction-for-people-who-know-just-enough-jquery-to-get-by/
- https://facebook.github.io/react/docs/thinking-in-react.html
- https://github.com/facebookincubator/create-react-app
- https://medium.freecodecamp.com/is-mvc-dead-for-the-frontend-35b4d1fe39ec#.nvumb4j5m
- https://facebook.github.io/react-native/
- https://facebook.github.io/react/docs/test-utils.html
- https://daveceddia.com/visual-guide-to-state-in-react/
- http://facebook.github.io/flux/
- redux.js.org
- https://mobxjs.github.io/mobx/
Links
ReactJS
By Michael Müller
ReactJS
- 789