Software Testing Introduction
"Something that is untested is broken."
Why test?
-
Refactoring is easier
-
Avoids regression, no more deploy and pray
-
This is required when you want to do CI/CD successfully.
-
New comers can easily contribute to the team
-
Somehow an indicator of code complexity
-
When part of your code is hard to test, it means that it you have to break things up, simplify things
-
Test Pyramid
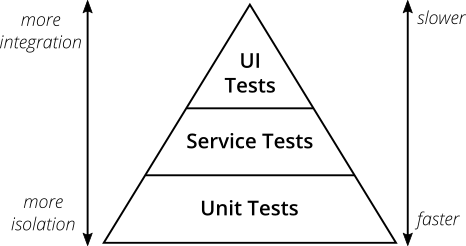
Unit tests
These are tests that tests certain "units" of you code.
- The term unit here varies, it may be a function, class or module.
- Unit tests can be sociable or solitary.
- They have the narrowest scope among the other types of tests, and we will write lots and lots of them.
Service Tests
These are tests that tests integration between services.
- This may include calls between different services.
- Application -> Database
- Application -> 3rd Party Service
- Database <- Application -> 3rd Party Service
- Will execute actual API calls instead of mocking/stubbing.
UI Tests
These are tests that tests the user interface of our application.
- Tests here includes end-to-end testing.
- This means that we have a dedicated environment, and will test everything from the front-end.
- The hardest test to do due to fragility. One change in the interface can break a lot of test cases.
- This is now easier with modern JS frameworks like React and Vue. Unit test style is possible because we are looking in to the state and methods of the component.
Methodologies
- Test-Driven Development (TDD)
- Behavior-Driven Development (BDD)
Test-Driven Development
- Add test
- Run test and see if anything fails
- Write code
- Run test
- Refactor
- Repeat
Behavior-Driven Development
- Define a test set
- Make the test fail
- Write code
- Run test
- Verify if working
- Refactor
- Repeat
Feature: Guess the word
# The first example has two steps
Scenario: Maker starts a game
When the Maker starts a game
Then the Maker waits for a Breaker to join
# The second example has three steps
Scenario: Breaker joins a game
Given the Maker has started a game with the word "silky"
When the Breaker joins the Maker's game
Then the Breaker must guess a word with 5 characters
Questions?
Thank you!
References
- https://martinfowler.com/articles/practical-test-pyramid.html
- https://www.martinfowler.com/bliki/TestDrivenDevelopment.html
- https://en.wikipedia.org/wiki/Test-driven_development
- https://en.wikipedia.org/wiki/Behavior-driven_development
Software Testing Introduction
By Jezeniel Zapanta
Software Testing Introduction
- 234