Get Started with Django Channels

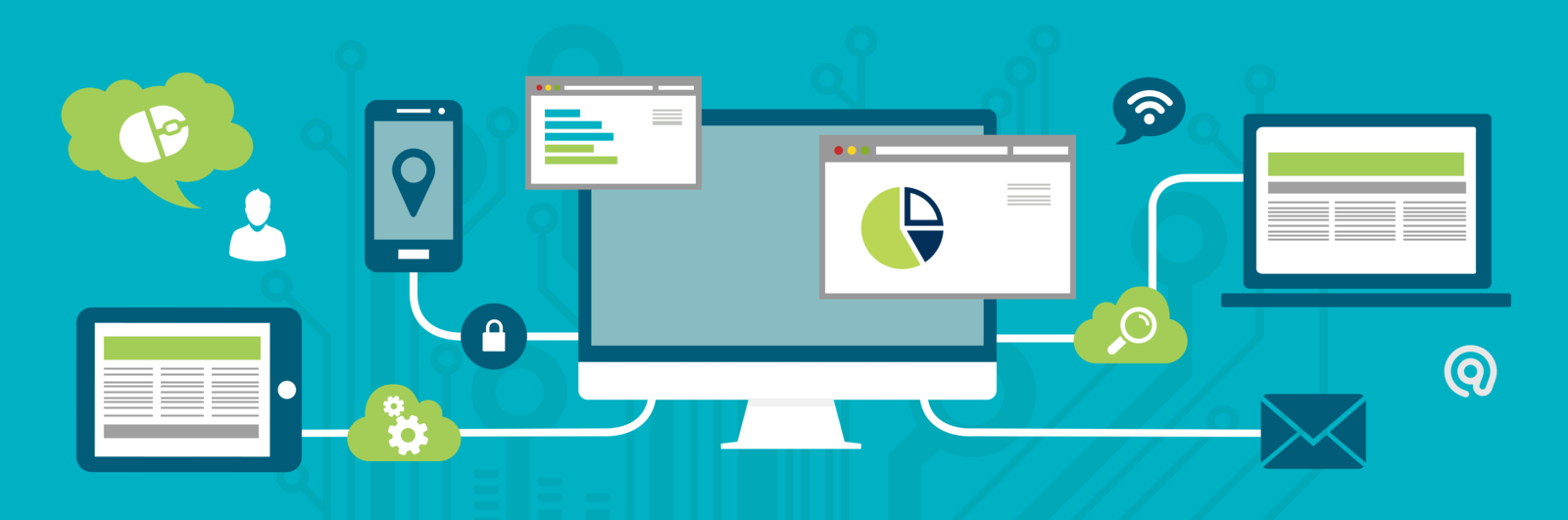
The web circa 2016 is significantly more powerful.

Real-Time Web

- Protocol that provides full-duplex communication
- Open connection between the client and the serve
- Send data at any time.

Django is built around the simple concept of requests and responses.
This doesn’t work with WebSockets!

Replaces the “guts” of Django — the request/response cycle — with messages that are sent across channels
Channels introduces some new concepts to Django
Channels are essentially task queues: messages get pushed onto the channel by producers, and then given to one of the consumers listening on that channel.
Django channels work across a network and allow producers and consumers to run transparently across many dynos and/or machines. This network layer is called the channel layer.
Channels is designed to use Redis as its preferred channel layer
Channels is available as a stand-alone app
Getting started: how to make a real-time chat app in Django
Simple real-time chat app

First steps — it’s still Django
The initial steps are the same as any Django app.
chat/models.py

First steps — it’s still Django
The initial steps are the same as any Django app.
chat/views.py

Where we’re going
To get a handle on what needed to be done on the backend, let’s look at the client code.



Installing and setting up Channels
1. Install Channels pip install channels add "channels” to your INSTALLED_APPS
2. Choose a channel layer: pip install asgi_redis

Installing and setting up Channels
3. Channel routing: Channel routing is a very similar concept to URL routing: URL routing maps URLs to view functions; channel routing maps channels to consumer functions.
4. Run with channels: Swap out Django's HTTP/WSGI-based request handler, for the one built into channels




WebSocket consumers
Channels maps WebSocket connections to three channels:
-
A message is sent to the websocket.connect channels the first time a new client (i.e. browser) connects via a WebSocket. When this happens, we’ll record that the client is now “in” a given chat room.
-
Each message the client sends on the socket gets sent across the websocket.receive channel. When a message is received, we’ll broadcast that message to all the other clients in the "room”.
-
Finally, when the client disconnects, a message is sent to websocket.disconnect. When that happens, we’ll remove the client from the “room”.

First, we need to hook each of these channels up in routing.py:

Let’s look at ws_connect first:
chat/consumers.py

Now that a client is connected, let’s take a look at ws_receive. This consumer will get called each time a message is received on the WebSocket:
After that, ws_disconnect is very simple:




(Heroku)
Thanks!
Get Started with Django Channels
By Juan David Hernández Giraldo
Get Started with Django Channels
- 1,376