React New Feature
TOC
- 버전 16
- 버전 16.3
- 새로운 버전에 쉽게 적응하는 방법
v 16
굉장히 많은 것이 바뀌기 시작하다
Fragments and strings
render() {
// No need to wrap list items in an extra element!
return [
// Don't forget the keys :)
<li key="A">First item</li>,
<li key="B">Second item</li>,
<li key="C">Third item</li>,
];
}
render() {
return 'Look ma, no spans!';
}
render() {
return <div>
<li key="A">First item</li>
<li key="B">Second item</li>
<li key="C">Third item</li>
</div>
}
Error Handling

class ErrorBoundary extends React.Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
componentDidCatch(error, info) {
this.setState({ hasError: true });
logErrorToMyService(error, info);
}
render() {
if (this.state.hasError) {
return <h1>Something went wrong.</h1>;
}
return this.props.children;
}
}
<ErrorBoundary>
<MyWidget />
</ErrorBoundary>
Portals
render() {
// React mounts a new div and renders the children into it
return (
<div>
{this.props.children}
</div>
);
}
render() {
// React does *not* create a new div. It renders the children into `domNode`.
// `domNode` is any valid DOM node, regardless of its location in the DOM.
return ReactDOM.createPortal(
this.props.children,
domNode
);
}
A typical use case for portals is when a parent component has an overflow: hidden or z-index style, but you need the child to visually “break out” of its container. For example, dialogs, hovercards, and tooltips
Server Side Suppport

DOM Attribute

etc
- Reduced file size(1/4)
- MIT Licensed
- New Core Archtecture
- fiber
- rewritten
- async rendering
- https://build-mbfootjxoo.now.sh/
- installation

v16.3
Lifecycle change & Context API
Context API
function ThemedButton(props) {
return <Button theme={props.theme} />;
}
// An intermediate component
function Toolbar(props) {
// The Toolbar component must take an extra theme prop
// and pass it to the ThemedButton
return (
<div>
<ThemedButton theme={props.theme} />
</div>
);
}
class App extends React.Component {
render() {
return <Toolbar theme="dark" />;
}
}
// Create a theme context, defaulting to light theme
const ThemeContext = React.createContext('light');
function ThemedButton(props) {
// The ThemedButton receives the theme from context
return (
<ThemeContext.Consumer>
{theme => <Button {...props} theme={theme} />}
</ThemeContext.Consumer>
);
}
// An intermediate component
function Toolbar(props) {
return (
<div>
<ThemedButton />
</div>
);
}
class App extends React.Component {
render() {
return (
<ThemeContext.Provider value="dark">
<Toolbar />
</ThemeContext.Provider>
);
}
}
https://reactjs.org/docs/context.html
Context API

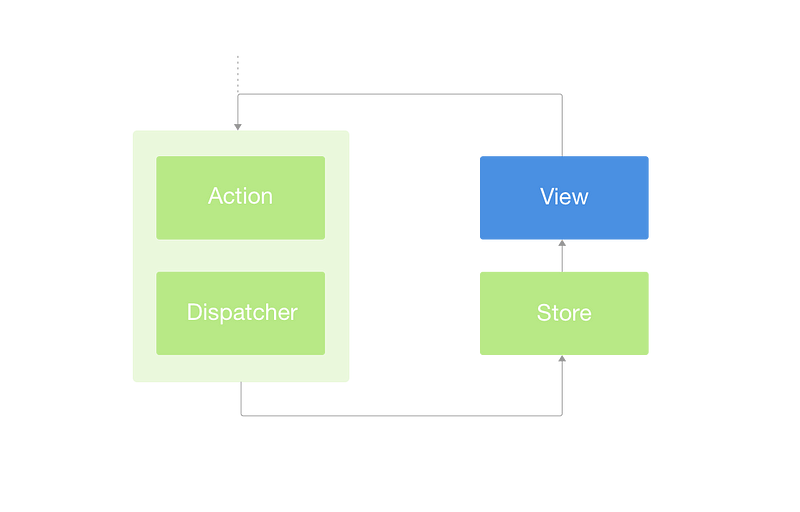
그림 출처 : https://medium.freecodecamp.org/replacing-redux-with-the-new-react-context-api-8f5d01a00e8c
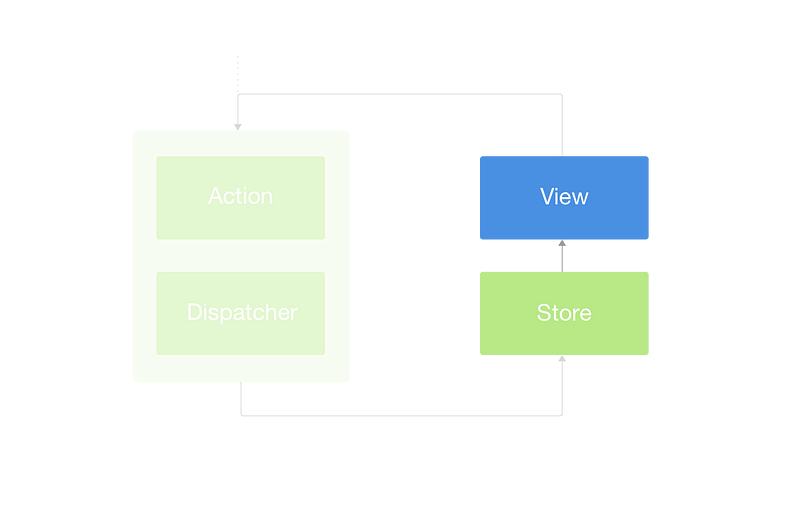
createRef API & ForwardRef API

Component Lifecycle Changes
- componentWillMount
- componentWillReceiveProps
- componentWillUpdate
~until v17
Strict Mode
import React from 'react';
function ExampleApplication() {
return (
<div>
<Header />
<React.StrictMode>
<div>
<ComponentOne />
<ComponentTwo />
</div>
</React.StrictMode>
<Footer />
</div>
);
}

새로운 버전 대응법
1. 무시한다

https://github.com/kelseyhightower/nocode
2. github

https://github.com/facebook/react/releases
3. blog
https://reactjs.org/blog/2018/03/29/react-v-16-3.html

4. devpools
http://www.devpools.kr/

감사합니다.
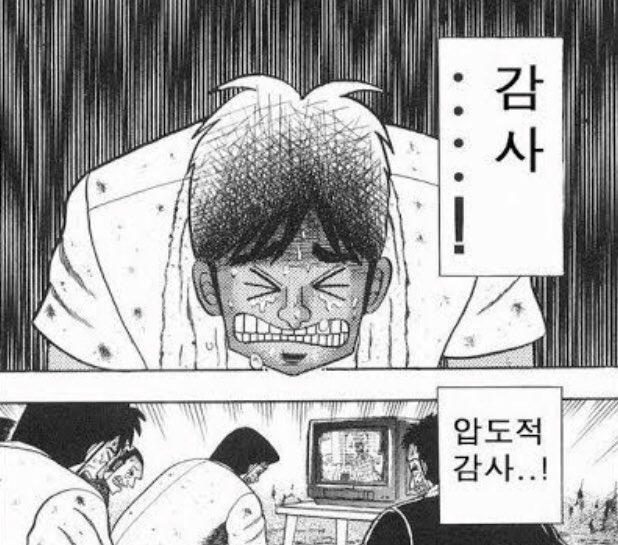
deck
By Keen Dev
deck
- 691