Why
TypeScript?
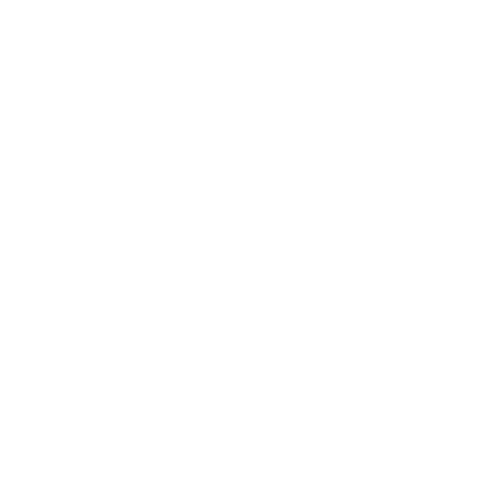
Marcell Kiss


marcellkiss@budacode.com
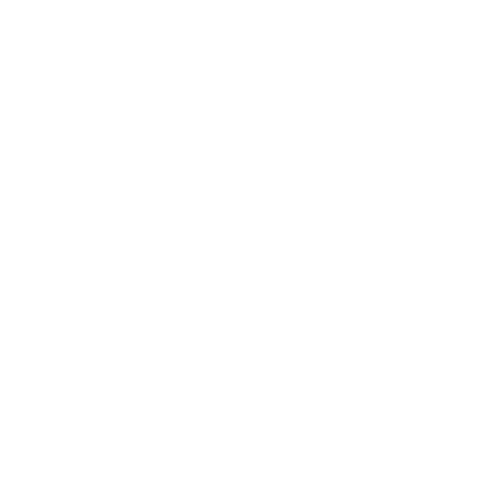



Developer
Organizer
Founder
Do we have
types
in JavaScript?
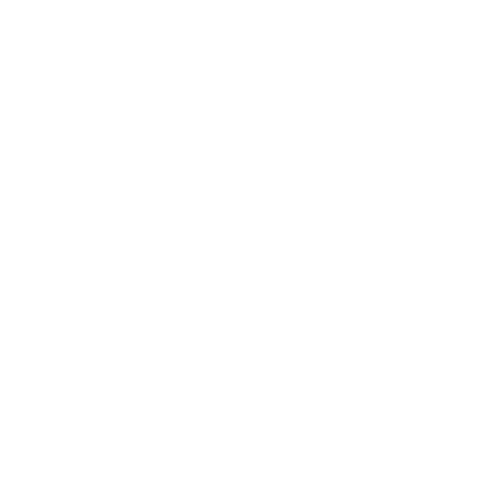
Yes, we have.
We'll cover
-
Compile-to-js languages
-
Static vs dynamic typing
-
TypeScript
- in general
- in practice
- under the hood
-
Conclusion
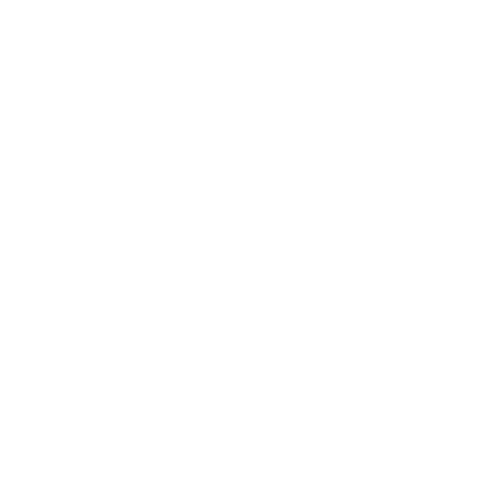
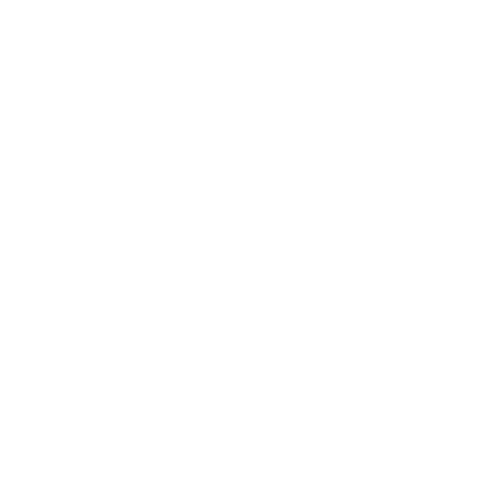
Compile-to-js languages
What do we have?
Compile-to-js languages
-
Dart
-
AtScript
-
CoffeScript
-
TypeScript
Note: there are hundreds of other solutions...
https://github.com/jashkenas/coffeescript/wiki/List-of-languages-that-compile-to-JS
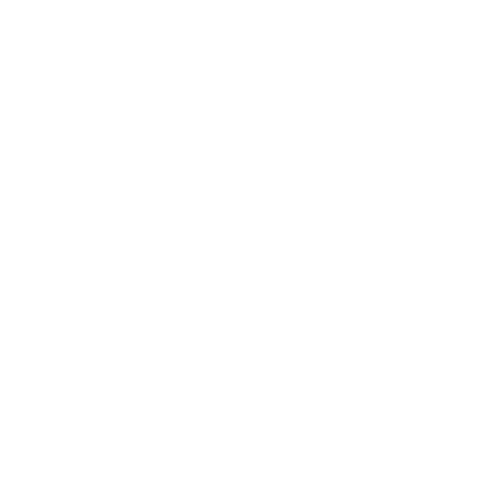
Compile-to-js languages

Good old standards...
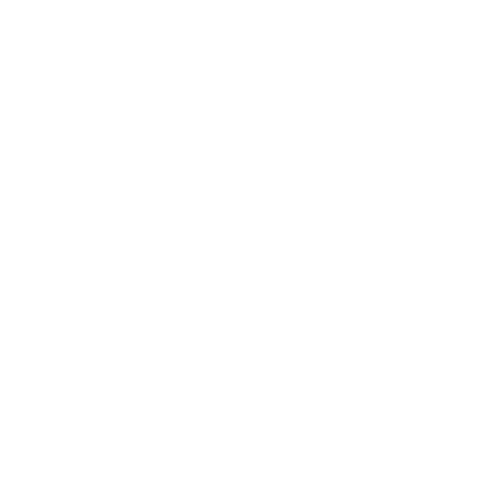
Compile-to-js languages

TypeScript is 'trending'
25.01.2016
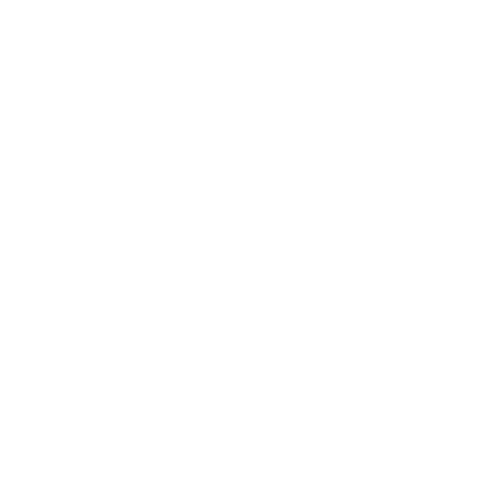
Static
vs
Dynamic typing
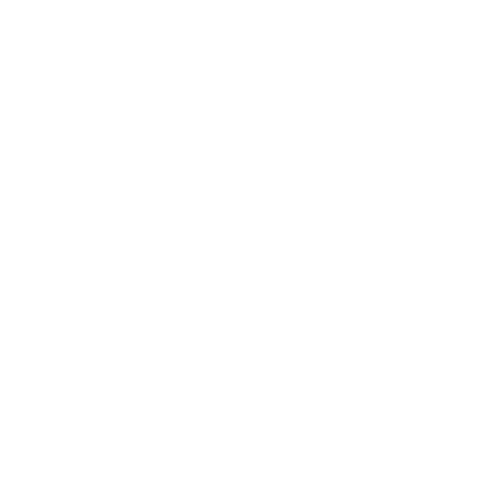
Dynamic typing
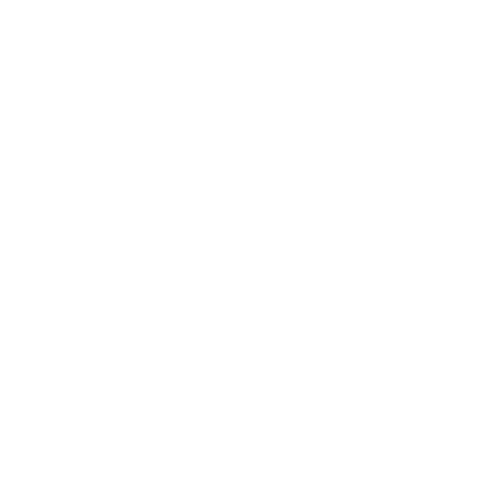
let someVar;
someVar = 'Hello World!';
someVar = 42
// ...End everything is fine...
let someVar: string;
someVar = 'Hello World!';
someVar = 42;
// Type 'number' is not assignable to type 'string'
Static typing
Static vs dynamic typing
Static typing
pros and cons
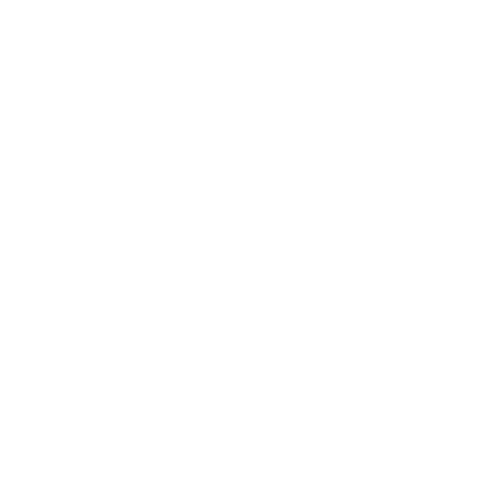
+
- Documentation
- IDE support
- Detection of mistakes
-
-
More verbose
- Less reusable code
- Not safer
Static vs dynamic typing
Static vs dynamic typing
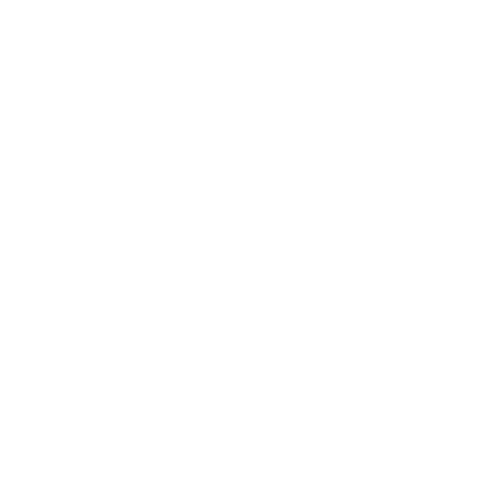
Eric Elliott @_ericelliott
Static types are overrated
Myth: it's impossible to write large applications using JavaScript
Type Correctness does not guarantee program Correctness
You need tests
TypeScript in general
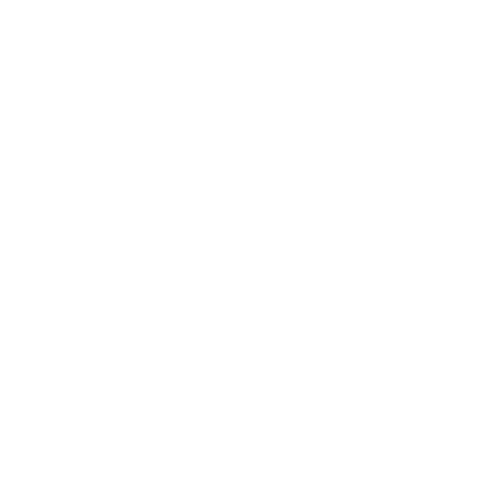
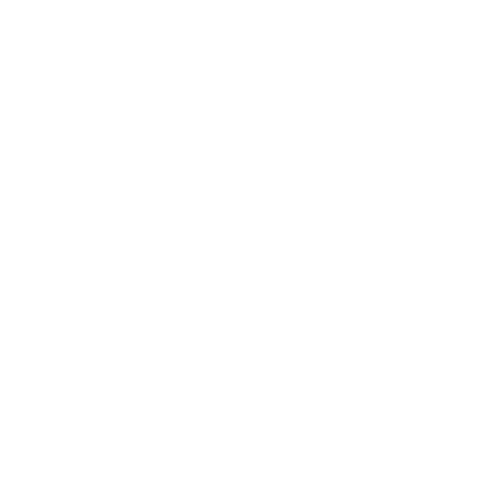
TypeScript in general
...TypeScript is a typed superset of JavaScript that compiles to plain JavaScript.
Any browser. Any host. Any OS. Open Source.
typescriptlang.org
Static vs dynamic typing
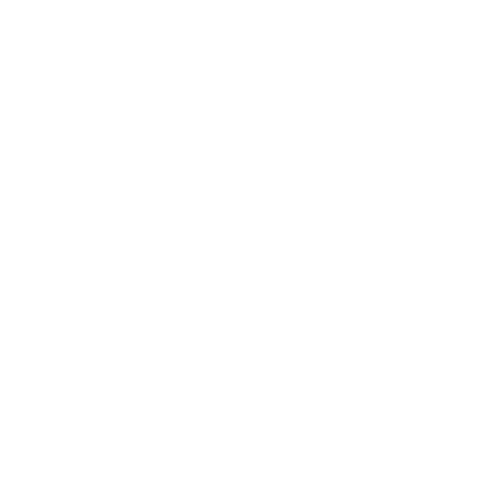
Microsoft’s TypeScript may be the best of the many JavaScript front ends.
...
I think that JavaScript’s loose typing is one of its best features and that type checking is way overrated. TypeScript adds sweetness, but at a price. It is not a price I am willing to pay.
Dougles Crockford, 2012
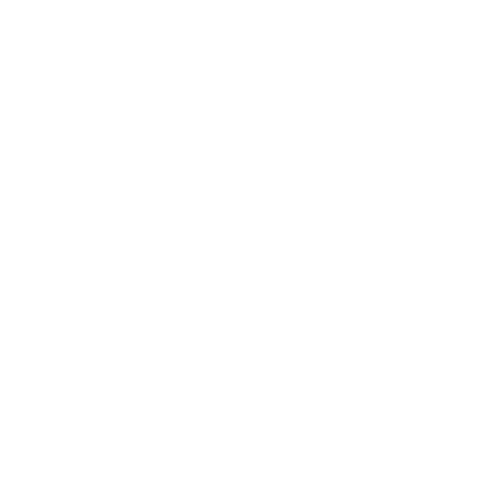
TypeScript in general
Background
-
Microsoft
TS: 2012 - Present
Anders Hejlsberg, lead architect of C# and creator of Delphi and Turbo Pascal, has worked on the development of TypeScript.
- Angular2
Changed AtScript to TypeScript
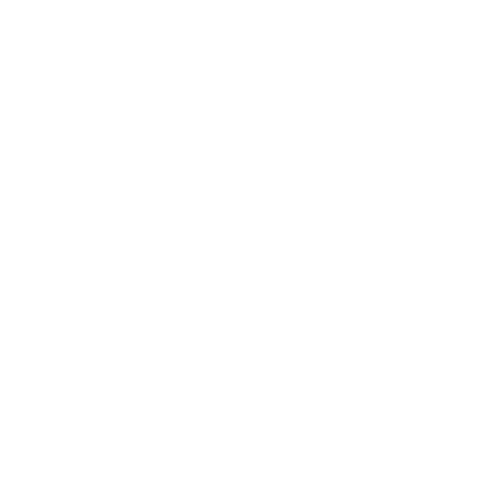
TypeScript in general
Benefits
The JavaScript community consolidates tools and frameworks about as often as Nicholas Cage makes a good movie.
@tjvantoll
- Static analysis
- ES Compatibility
- Opt-in
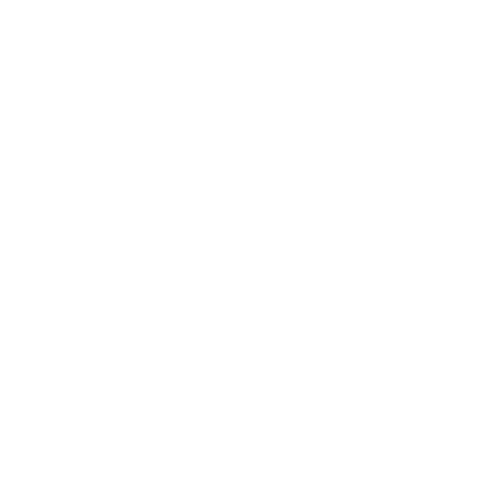
TypeScript
in practice
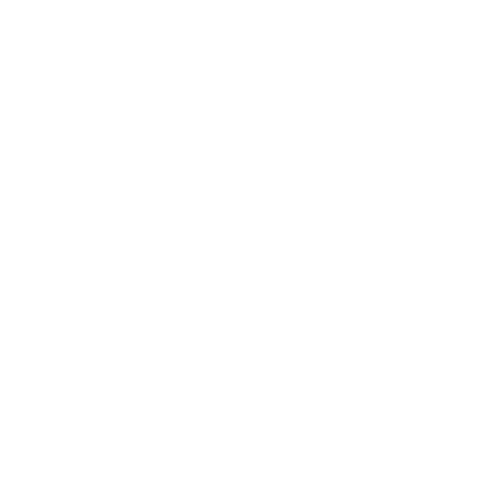
TypeScript in practice
Really quick start!
typescriptlang.org/Playground

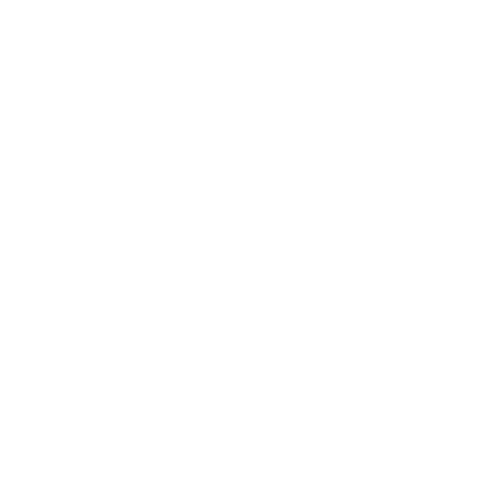
TypeScript in practice
Basics
// There are 3 basic types in TypeScript
var isDone: boolean = false;
var lines: number = 42;
var name: string = "Anders";
// When it's impossible to know, there is the "Any" type
var notSure: any = 4;
notSure = "maybe a string instead";
notSure = false; // okay, definitely a boolean
// For collections, there are typed arrays and generic arrays
var list: number[] = [1, 2, 3];
// Alternatively, using the generic array type
var list: Array<number> = [1, 2, 3];
// For enumerations:
enum Color {Red, Green, Blue};
var c: Color = Color.Green;
// Lastly, "void" is used in the special case of a function returning nothing
function bigHorribleAlert(): void {
alert("I'm a little annoying box!");
}
// Sources: learnxinyminutes.com
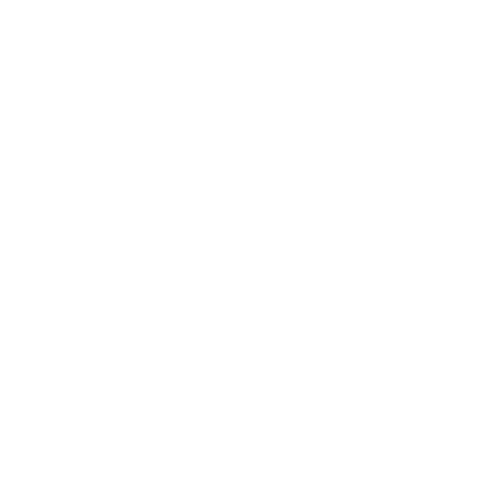
TypeScript in practice
// Interfaces are structural, anything that has the properties is compliant with
// the interface
interface Person {
name: string;
// Optional properties, marked with a "?"
age?: number;
// And of course functions
move(): void;
}
// Object that implements the "Person" interface
// Can be treated as a Person since it has the name and move properties
var p: Person = { name: "Bobby", move: () => {} };
// Objects that have the optional property:
var validPerson: Person = { name: "Bobby", age: 42, move: () => {} };
// Is not a person because age is not a number
var invalidPerson: Person = { name: "Bobby", age: true };
// Interfaces can also describe a function type
interface SearchFunc {
(source: string, subString: string): boolean;
}
// Only the parameters' types are important, names are not important.
var mySearch: SearchFunc;
mySearch = function(src: string, sub: string) {
return src.search(sub) != -1;
}
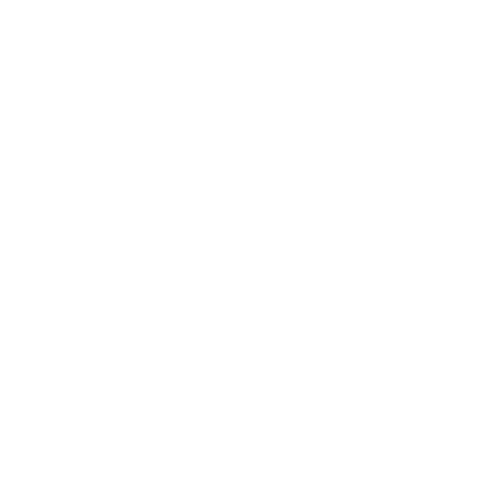
TypeScript in practice
// Classes - members are public by default
class Point {
// Properties
x: number;
// Constructor - the public/private keywords in this context will generate
// the boiler plate code for the property and the initialization in the
// constructor.
// In this example, "y" will be defined just like "x" is, but with less code
// Default values are also supported
constructor(x: number, public y: number = 0) {
this.x = x;
}
// Functions
dist() { return Math.sqrt(this.x * this.x + this.y * this.y); }
// Static members
static origin = new Point(0, 0);
}
var p1 = new Point(10 ,20);
var p2 = new Point(25); //y will be 0
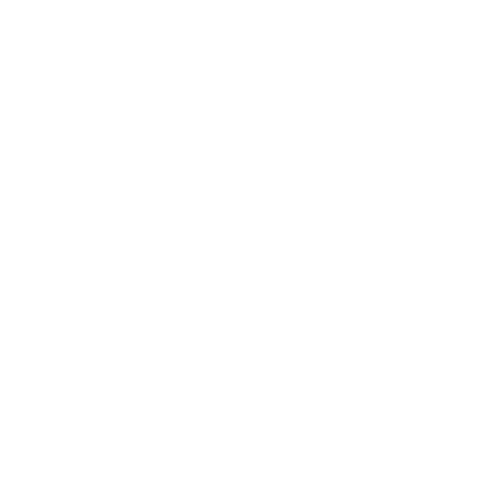
TypeScript in practice
// Inheritance
class Point3D extends Point {
constructor(x: number, y: number, public z: number = 0) {
super(x, y); // Explicit call to the super class constructor is mandatory
}
// Overwrite
dist() {
var d = super.dist();
return Math.sqrt(d * d + this.z * this.z);
}
}
Get started in local env
// Install using npm
npm install -g typescript
// Compile ts to js
tsc type.ts
// Compile more files into one and set a watcher
tsc *.ts --out app.js --watch
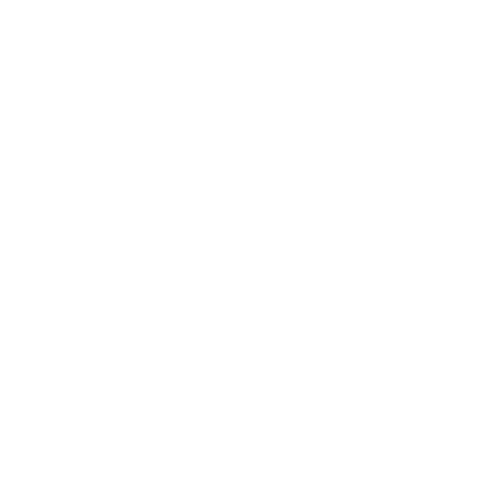
Adding it to your build process using
(grunt / gulp / webpack) is easy
TypeScript in practice
Tooling
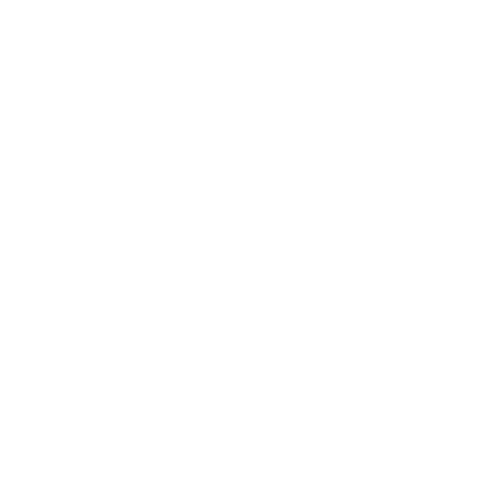
Tslint (npm)
A linter for the TypeScript language.
Typings (npm)
/// <reference path="jquery.d.ts" />
The type definition manager for TypeScript.
TypeScript in practice
Practical example
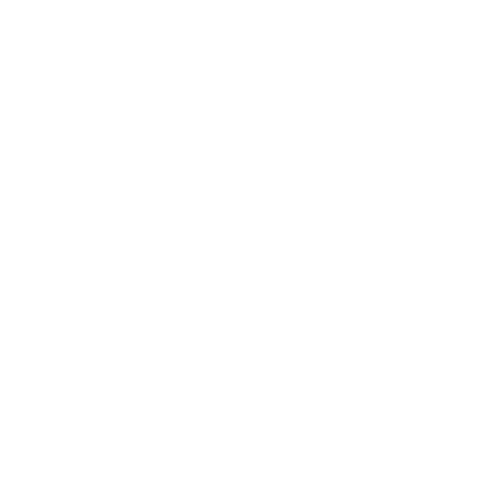
TypeScript in practice

Date range:
Aggregation type:
-
let chartFilterObject = {
startTime: null,
endTime: null,
aggregationType: null
};
<-- Create a directive
.. then, pass the results all around
We need a class, that's sure...
Practical example II.
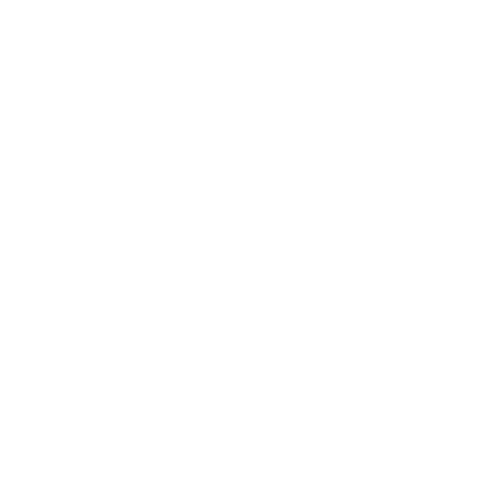
TypeScript in practice
What do we win with static typing?
- You'll always know how the object works
- The IDE will also help (autocomplete)
- Nobody will add arbitrary variables
Under the hood
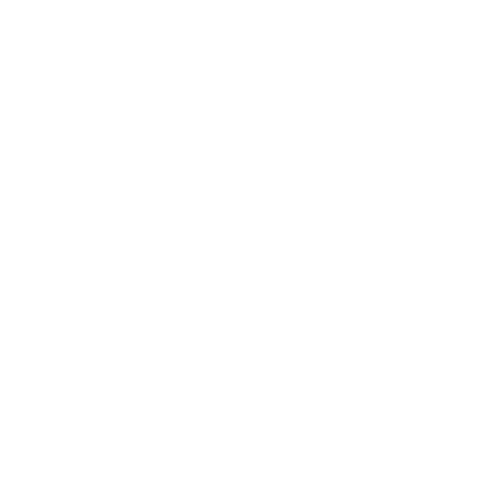
TypeScript in practice
let x: string;
x = 'Hello World!';
var x;
x = 'Hello World!';
Writing TS:
Compiled JS:
Type checking just in compile time
Under the hood II.
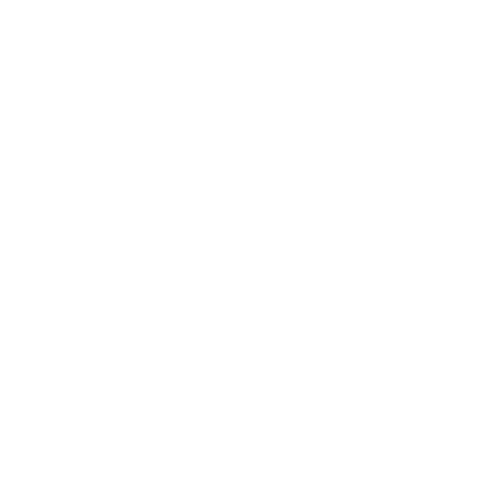
TypeScript in practice
class Greeter {
greeting: string;
constructor(message: string) {
this.greeting = message;
}
greet() {
return "Hello, " + this.greeting;
}
}
var Greeter = (function () {
function Greeter(message) {
this.greeting = message;
}
Greeter.prototype.greet = function () {
return "Hello, " + this.greeting;
};
return Greeter;
})();
Writing TS:
Compiled JS:
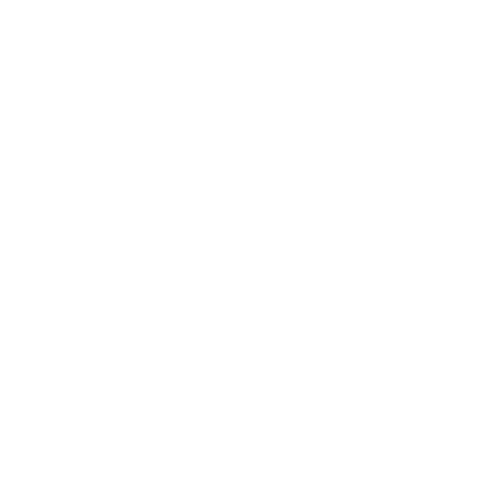
class Animal {
constructor(public name: string) { }
move(meters: number) {
alert(this.name + " moved " + meters + "m.");
}
}
class Snake extends Animal {
constructor(name: string) { super(name); }
move() {
alert("Slithering...");
super.move(5);
}
}
TypeScript in practice
Writing TS:
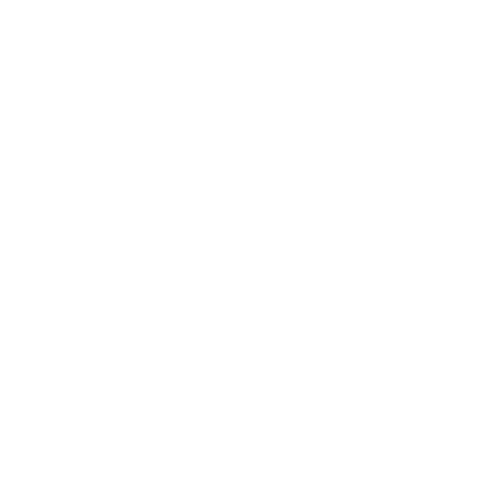
var __extends = (this && this.__extends) || function (d, b) {
for (var p in b) if (b.hasOwnProperty(p)) d[p] = b[p];
function __() { this.constructor = d; }
d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __());
};
var Animal = (function () {
function Animal(name) {
this.name = name;
}
Animal.prototype.move = function (meters) {
alert(this.name + " moved " + meters + "m.");
};
return Animal;
})();
var Snake = (function (_super) {
__extends(Snake, _super);
function Snake(name) {
_super.call(this, name);
}
Snake.prototype.move = function () {
alert("Slithering...");
_super.prototype.move.call(this, 5);
};
return Snake;
})(Animal);
TypeScript in practice
Compiled JS:
Conclusion
Matured technology
Well supported
'JavaScript safe'
Opt-in
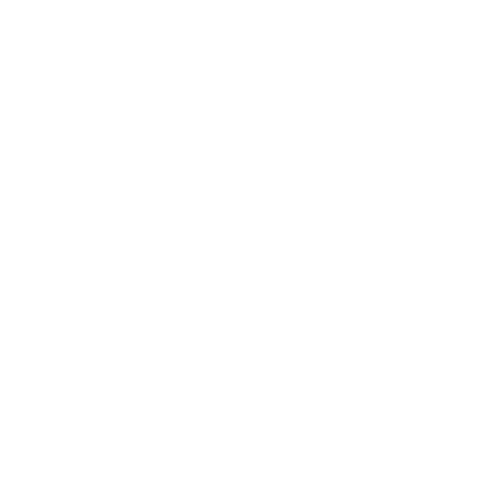
Thanks
for your attention!
Q & A
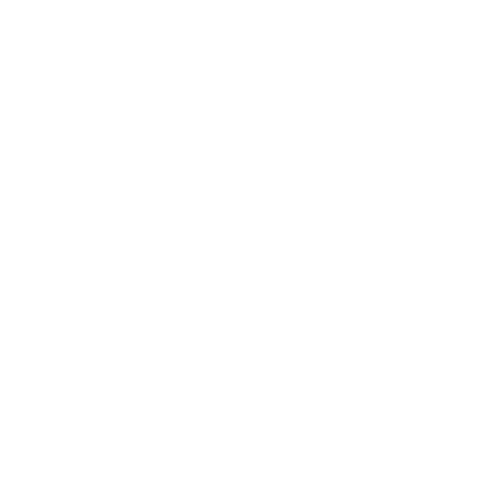
@martzellk
Sources
Original source - https://github.com/Microsoft/TypeScript
Tutorial - http://www.typescriptlang.org/Tutorial
Samples - http://www.typescriptlang.org/Samples
Handbook - http://www.typescriptlang.org/Handbook
Eric Elliott, Static types are overrated - https://www.youtube.com/watch?v=_kXiH1Yiemw
Ordog Rafael, Static vs dynamic typing - https://www.youtube.com/watch?v=K1D3IQ_L_2Q
The Rise of TypeScript? - http://developer.telerik.com/featured/the-rise-of-typescript/
Comparing to ES6 - http://kangax.github.io/compat-table/es6/
Compile to JS - https://github.com/jashkenas/coffeescript/wiki/List-of-languages-that-compile-to-JS
ES7 Proposal: typed objects - https://github.com/hemanth/es7-features
Great place to learn - http://learnxinyminutes.com
TSD - http://definitelytyped.org/tsd/
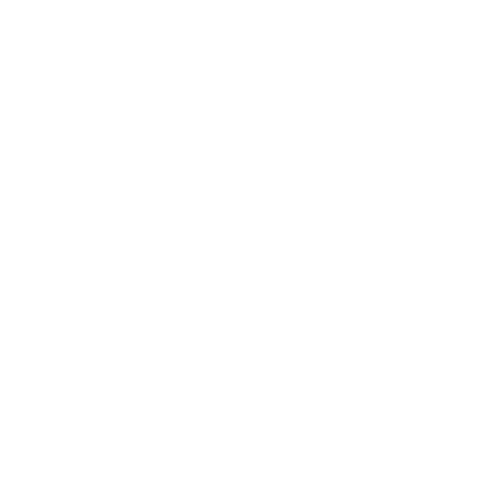
Why TypeScript - Berlin
By Marcell Kiss
Why TypeScript - Berlin
- 1,528