WHAT IS Sass?
SYNTACTICALLY
AWESOME
STYLESHEETS
MEta-Language
AND
PREPROCESSOR
FOR
CSS
FROM THE SASS DOCUMENTATION
Sass is an extension of CSS that adds power and elegance to the basic language. It allows you to use variables , nested rules , mixins , inline imports , and more, all with a fully CSS-compatible syntax. Sass helps keep large stylesheets well-organized, and get small stylesheets up and running quickly, particularly with the help of the Compass style library .
SYNTAX
.SASS
.my-element
width: 100%
overflow: hidden
color: blue
.SCSS
.my-element {
width: 100%;
overflow: hidden;
color: blue;
}
ATTRIBUTES
$VARIABLE
$width-variable: 5em;
.base {
width: $width-variable;
}
$base-height: 10px;
$default-font: 'Lucida Grande';
$default-bg-color: rgba(255, 0, 0, 0.5);
$enable-glossy-border: true;
$my-special-gradient: left, #ddebb2 0%, #ffffff 100%;
LOCAL OR GLOBAL?
$default-font: 'Verdana'
.post {
$default-font:'Lucida Grande';
font-family: $default-font;
}
.post {
$post-font: 'Lucida Grande';
font-family: $post-font;
}
.specialPost{
$post-font: 'Verdana';
font-family: $post-font;
}
OPERATIONS
.blogTitle {
font-size: $default-font-size * 3;
background: $default-background / 2;
padding: 10px;
margin: 10px;
border: {
color: #13f083 * 2;
width: $default-border-width - 3;
radius: $default-border-radius + 2;
}
}
NESTED RULES
#admin {
color: #fe0988;
p, div > .box {
font-size: 1.5em;
a {
font-weight: bold;
}
}
}
#admin {
color: #fe0988;
}
#admin p, #admin div > .box {
font-size: 1.5em;
}
#admin p a, #admin div > .box a {
font-weight: bold;
}
...REFERENCING WITH &
h3 {
font-size: 20px;
.awesome-one & {
font-size: 24px;
}
&.awesome-two {
font-size: 30px;
}
}
h3 {
font-size: 20px;
}
.awesome-one h3 {
font-size: 24px;
}
h3.awesome-two {
font-size: 30px;
}
@MIXIN
@mixin my-border {
border: {
color: #f08901;
width: 5px;
}
}
div.myBox {
@include my-border;
}
div.myBox {
border-color: #f08901;
border-width: 5px;
}
...with PARAMS
@mixin individual-border ($color, $width) {
border: {
color: $color;
width: $width;
}
}
div.individualBox {
@include individual-border($color: #07f741, $width: 10px);
}
div.individualBox {
border-color: #07f741;
border-width: 10px;
}
...AND DEFAULTS
@mixin special-border ($color: #010101, $width: 2px) {
border: {
color: $color;
width: $width;
}
}
div.specialBox {
@include special-border;
}
div.specialBox {
border-color: #010101;
border-width: 2px;
}
@EXTEND
.user {
color: #ffffff;
background: #880912;
border-style: solid;
}
.premiumUser {
@extend .user;
font-weight: bold;
}
.user, .premiumUser {
color: #ffffff;
background: #880912;
border-style: solid;
}
.premiumUser {
font-weight: bold;
}
ADvanced STYLING
@IF @ELSE
$fancy-style: false;
.myButton {
@if $fancy-style {
border-color: #d5ff00;
background: #aa00ff;
}
@else {
border-color: #f8f8f8;
background: #6f6f6f;
}
}
.myButton {
border-color: #f8f8f8;
background: #6f6f6f;
}
@FOR
$max-items: 5;
@for $i from 1 through $max-items {
.item-#{$i} {
width: 1em * $i;
}
}
.item-1 { width: 1em; }
.item-2 { width: 2em; }
.item-3 { width: 3em; }
.item-4 { width: 4em; }
.item-5 { width: 5em; }
@EACH
@each $car in viper, fiesta, golf {
.#{$car}-image {
background-image: url('../images/#{$car}.png');
}
}
.viper-image {
background-image: url("../images/viper.png");
}
.fiesta-image {
background-image: url("../images/fiesta.png");
}
.golf-image {
background-image: url("../images/golf.png");
}
@WHILE
$i: 3;
@while $i > 0 {
.while-item-#{$i} {
width: 2em * $i;
$i: $i - 1;
}
}
.while-item-3 {
width: 6em;
}
.while-item-2 {
width: 4em;
}
.while-item-1 {
width: 2em;
}
@FUNCTIOnS
$special-width: 10px;
@function double($arg) {
@return $arg * 2;
}
.fn-test {
width: double($special-width);
}
.fn-test {
width: 20px;
}
LIBRARIES
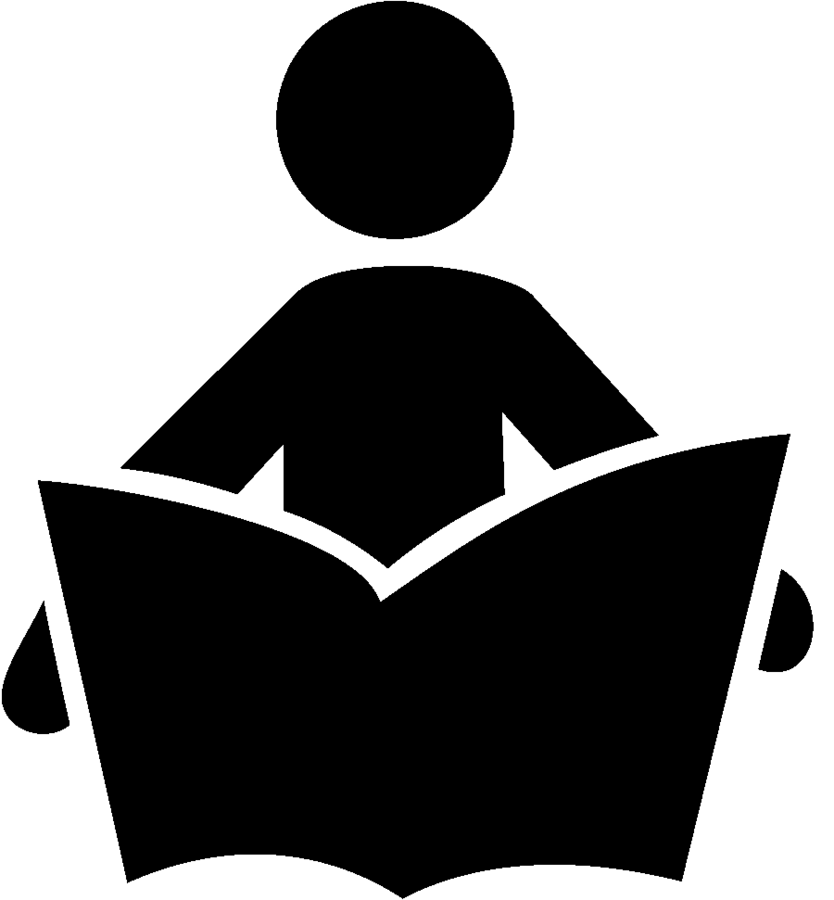
COMPASS
@import "compass/css3/border-radius";
.simpleBox {
@include border-radius (4px, 4px);
}
.simpleBox {
-webkit-border-radius: 4px 4px;
-moz-border-radius: 4px / 4px;
-khtml-border-radius: 4px / 4px;
border-radius: 4px / 4px;
}
@import "compass/css3/border-radius";
.simpleBox {
@include border-radius (4px, 4px);
}
.simpleBox {
-webkit-border-radius: 4px 4px;
-moz-border-radius: 4px / 4px;
-khtml-border-radius: 4px / 4px;
border-radius: 4px / 4px;
}
BOURBON
@import "..bourbon/bourbon";
.bourbonTest {
@include background(linear-gradient(red, green) left repeat);
}
.bourbonTest {
background: -webkit-linear-gradient( red, green) left repeat;
background: linear-gradient( red, green) left repeat;
}
Alternatives
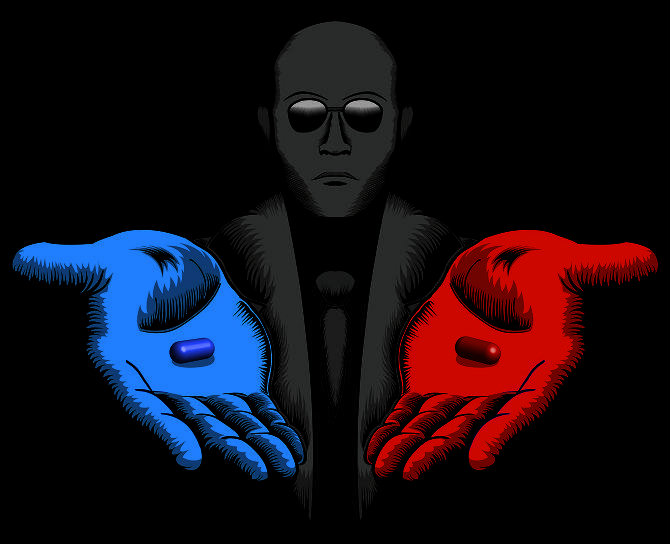
LESS
h1 {
color: #0982c1;
}
.mixin (@arg) when (lightness(@arg) >= 50%) {
background-color: black;
}
.mixin (@arg) when (lightness(@arg) < 50% {
background-color: white;
}
.mixin (@arg) {
color: @arg;
}
STYLUS
h1 {
color: #0982C1;
}
h1
color: #0982C1;
h1
color #0982C1
.logo {
width: 150px;
height: 100px;
margin-left: -(@width / 2);
margin-top: -(@height / 2);
}
QUESTIONS?
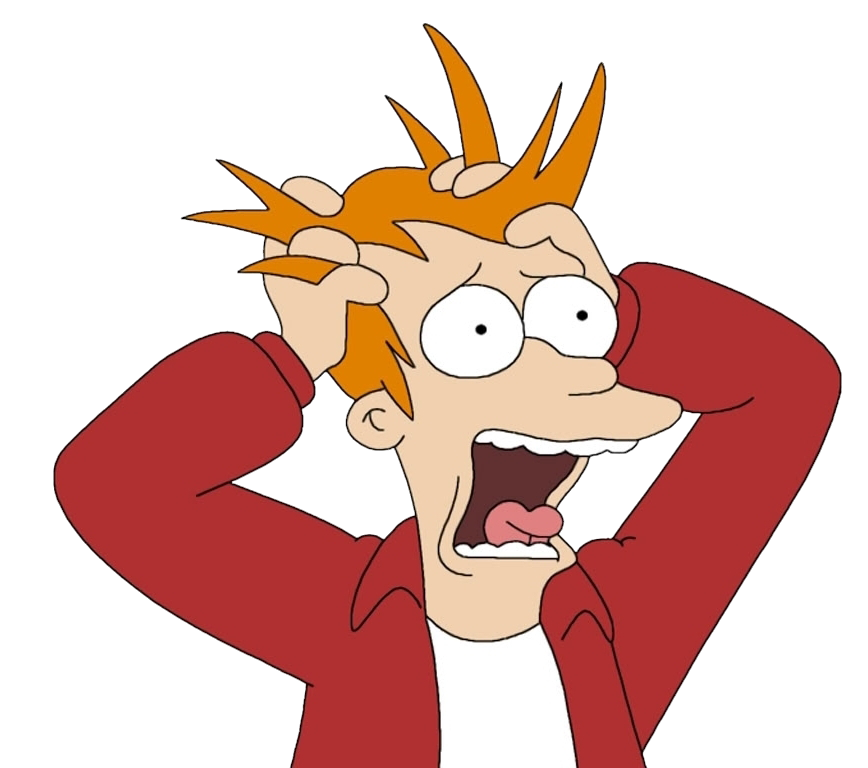
SASS
By mnowotny
SASS
A small presentation about Sass.
- 2,303