Database relationships
Database relationships
- MongoDB is schema-less (no relations)
- Mongoose provides a schema (and relations)
Database relationships
- 1 to 1
- 1 to n (One to Many)
- n to m (Many to Many)
One to One
- One item at one side has a single matching item at the other, and viceversa
- Implemented with a reference in the main one (alternatively, a reference at each end)
const countrySchema = new mongoose.Schema({
name: String,
capital: {
type: ObjectID,
ref: ''
}
});
const Country = mongoose.model('Country', countrySchema);
const capitalCitySchema = new mongoose.Schema({
name: String
});
const CapitalCity = mongoose.model('CapitalCity', capitalCitySchema);
const countrySchema
= new mongoose.Schema({
name: String,
capital: {
type: ObjectID,
ref: ''
}
});
const Country =
mongoose.model('Country', countrySchema);
const capitalCitySchema
= new mongoose.Schema({
name: String
});
const CapitalCity =
mongoose.model('CapitalCity',
capitalCitySchema);
const country = new Country({ name: 'Spain' });
const capitalCity = new CapitalCity({ name: 'Madrid' });
console.log(country.capital) // Madrid
console.log(capitalCity.country) // Spain
One to Many
- One item at side A has several matching ones at side B
- One item at side B has a single matching item at side A
- Implemented with a reference to the parent, or a list of references to the childrens (alternatively, with a junction table)
Many to Many
- One item at side A has several matching ones at side B
- One item at side B has several matching items at side A
- Usually implemented with a junction table (alternatively, a list of references at each end)
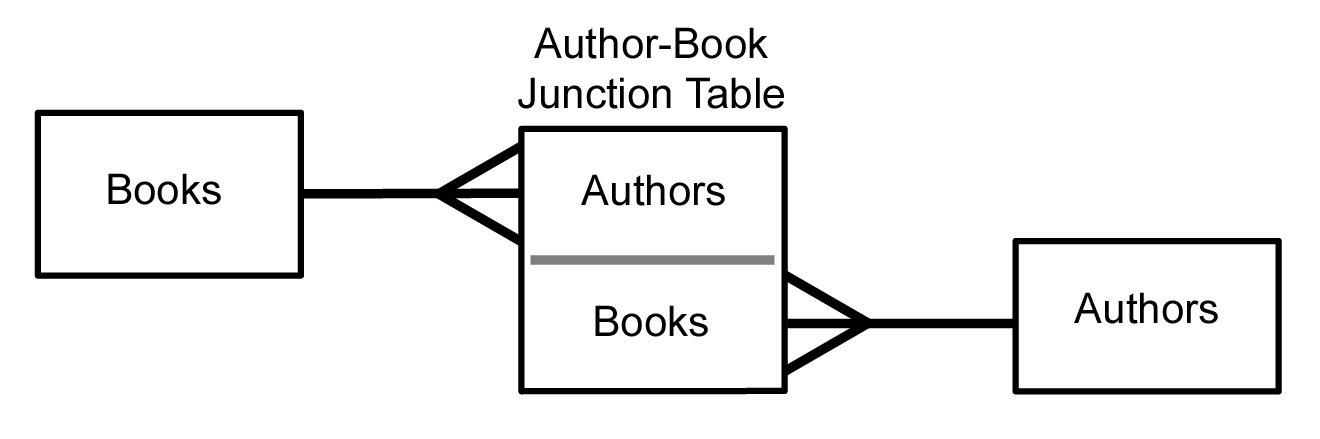
+ info
- https://en.wikipedia.org/wiki/One-to-one_(data_model)
- https://en.wikipedia.org/wiki/One-to-many_(data_model)
- https://en.wikipedia.org/wiki/Many-to-many_(data_model)
deck
By Jesús Leganés-Combarro
deck
- 390