Building
Command-Line Tools
in Ruby

What's the plan?
- Part One -- Getting Started
- Part Two -- Why Ruby?
- Part Three -- Using Ruby
- Part Four -- Power of Ruby
Part One
Getting Started
What is a command-line tool?
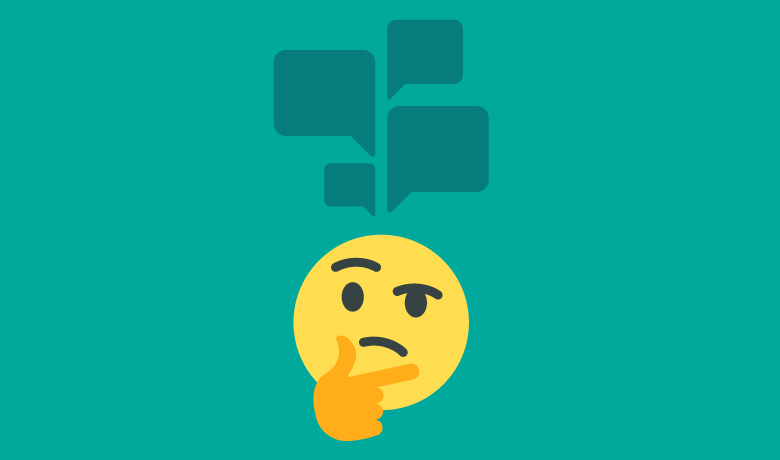
How does this help my project?
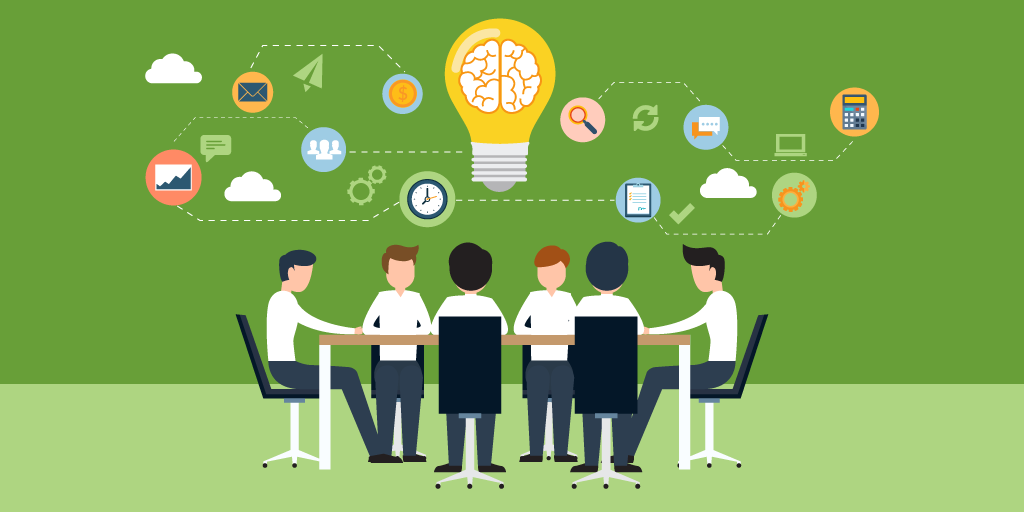
How does this help my career?

A single command

A suite of commands

Anatomy of a script interface

What makes a good script?



How to get started?

Check for name conflicts

The "hello world" script (Bash)


Can't find the script

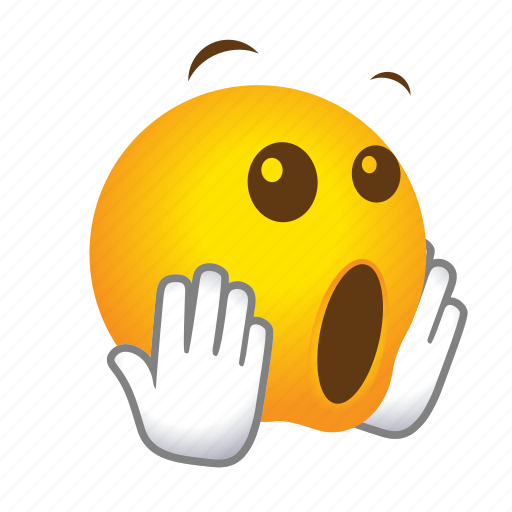
Add directory to PATH


You don't have permission

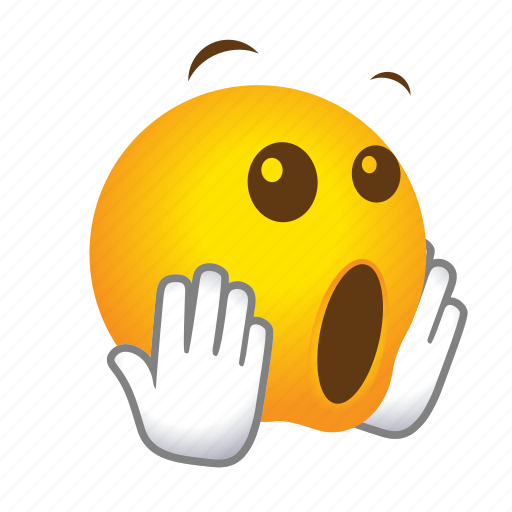
Examine the file permissions

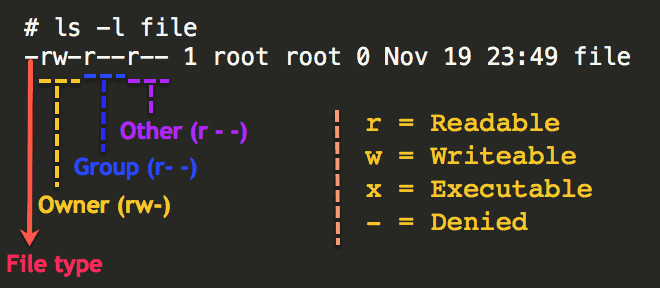
Change the file permissions
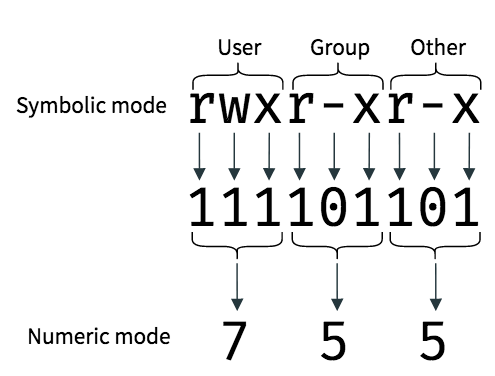

Success!

Summary
- Make your life easier by writing scripts
- Broaden your career by writing scripts
- Choose your script names carefully
- Add your script locations to your PATH
- Make your scripts executable with chmod
Part Two
Why Ruby ?
Choose the right tool for the job
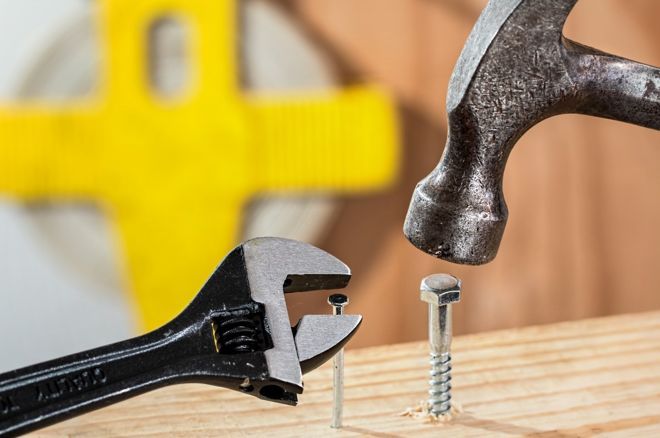
Ruby is a great choice
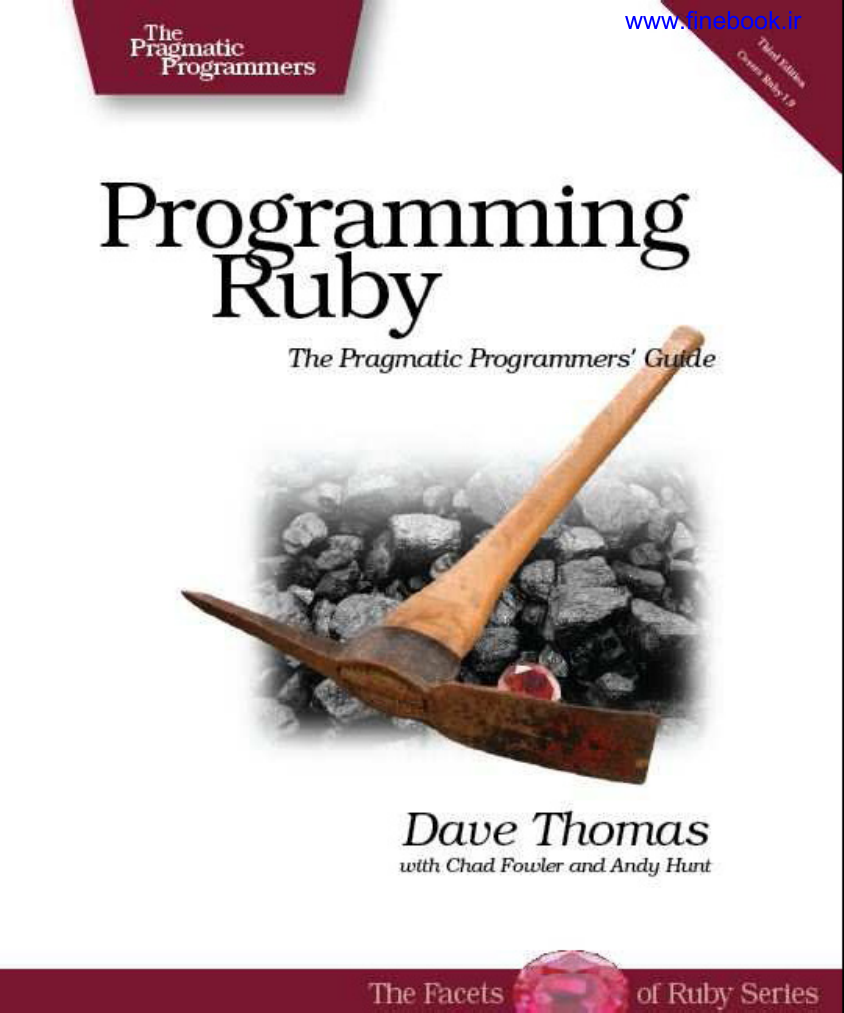
Text
Ruby is OOP

Ruby is available

Ruby is full-featured
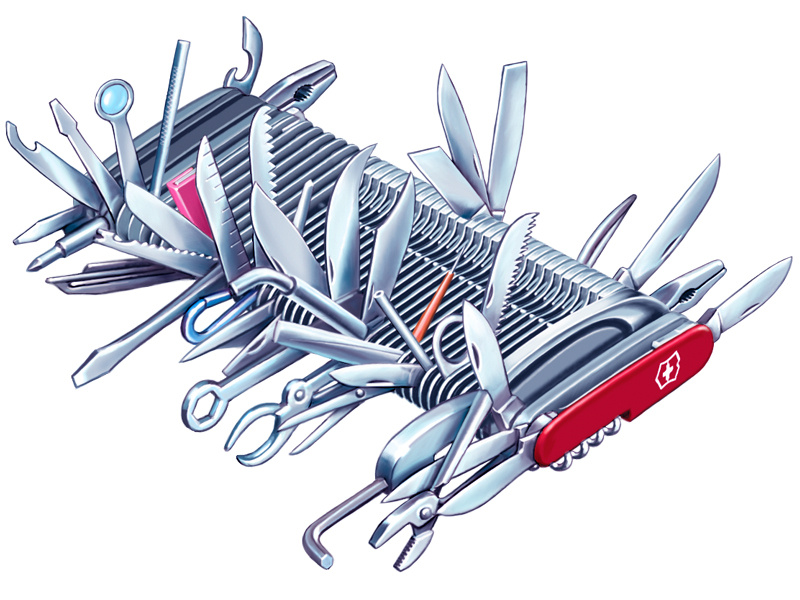
Ruby is diversivication
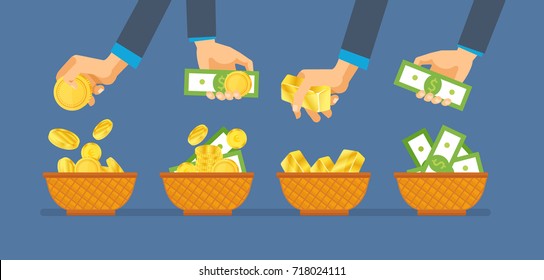
The "hello world" script (Ruby)


Can't find "puts"

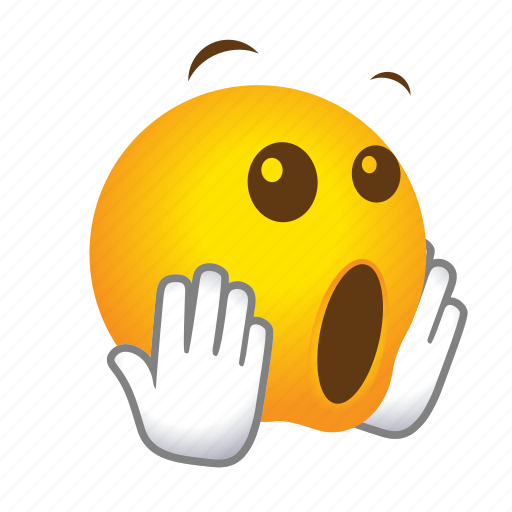
How to run a Ruby script

Start with the magic number


The "shebang" magic numberÂ


In search of Ruby



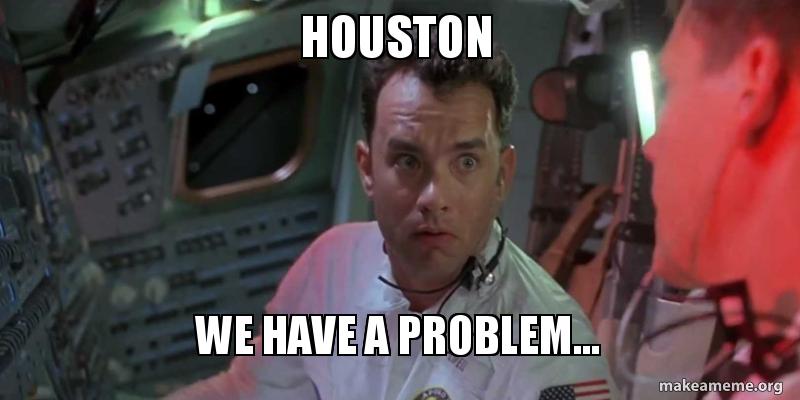
Use "env" to search for Ruby



Summary
- Always choose the right tool for the job
- Ruby is a great choice (more to come)
- Start all your scripts with #! (shebang)
- Use /usr/bin/env to locate your script interpreter
Part Three
Using Ruby
Access to the command-line

Passing arguments

Move business logic into a class


Require and use the class

More path problems

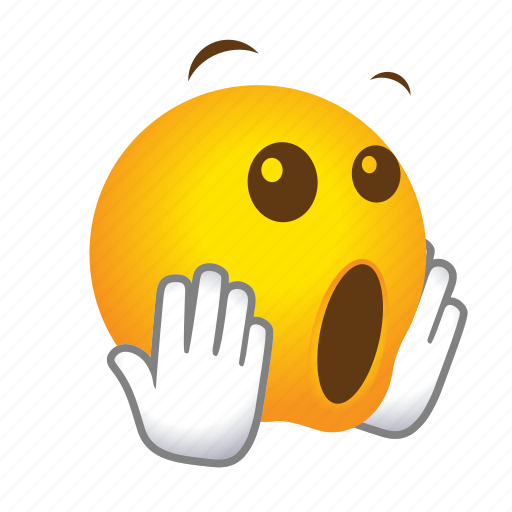


Ruby has a search path too
Move argument processing into a class

A basic argument classÂ


It still works

But what we really want is...

Parse the arguments


Use the parse results

Almost works

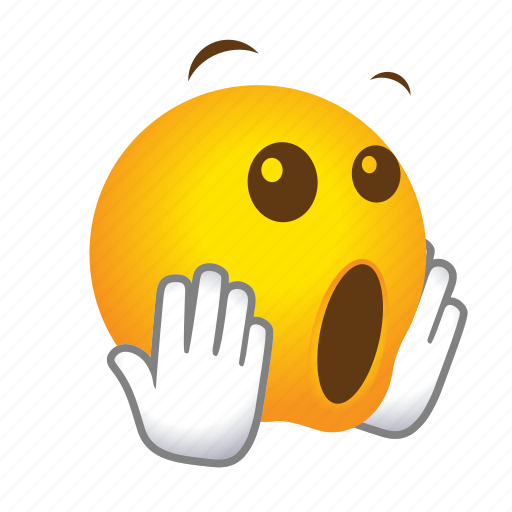
More argument parsing

It works

Just one more thing...


Summary
- Use ARGV to access command-line arguments
- Decompose your script into multiple files
- Use $LOAD_PATH to manage multiple files
- Use File.join to be more portable
- Use something like the Commander class to isolate argument processing
- Use OptionParser to provide the standard command-line interface
Part Four
Power of Ruby
What kinds of things are you going to want to do?
- Run Linux commands
- Read and Write files
- Parse and Generate file formats (e.g. JSON, YAML)
- Access the environment
3 ways to run Linux commands
- system( )
- exec( )
- ` `
#1 Run a command as a string


Check the success of each command




#2 Run a command as a string


#3 Run a command as a string
Parsing the output


Reading from a file



Writing to a file
Working with JSON


Working with JSON



Working with YAML
Working with YAML

Accessing environment variables


More access to the environment
-
FileUtils class
- pwd, mkdir, rmdir, ln, cp, mv, rm, chmod, chown, touch, ...
-
File class
- exist?, expand_path, join, open, read, write, ...
-
Dir class
- chdir, entries, exist?, ...
Summary
- Invoke external commands with system, exec, and ` `
- Read and write file contents with File methods
- Parse and generate JSON and YAML
- Access environment variables with ENV
- Access the environment with FileUtils, File, and Dir
Good resource

Questions
Building Command-Line Tools in Ruby
By Rober Kiel
Building Command-Line Tools in Ruby
- 335