Immutable Data
& Why
- Julia Gao @ryoia
Persistent Data Structure
A data structure that always returns a new value when the old one is getting modified.
Hence, the data structures are immutable.
That's it!
//mutable
var a = 10;
a = 10 * 2
//immutable
const a = 10;
const b = a * 2;
Immutable Data

Why Should You Care About Immutability?
var changeable = 1;
function bad (n, fn) {
changeable = changeable * 2;
return fn(changeable, n);
}
bad(1, multiply) // 2
bad(1, multiply) // 4
var changeable = 1;
function bad (n, fn) {
changeable = changeable * 2;
changeable = unknown(changeable);
return fn(changeable, n);
}
bad(1, multiply) //16
bad(1, multiply) // 253

Stateless/Pure Functions
- Same inputs, same output
- Every single time the function is being called
- Regardless of outside changes
Pssh, why do I need to care about that?
Why are stateless functions important
(why-is-this-important-ception)
- Guarantee the result is always what you expected
- No side effects
- Reduce #s of unit tests needed
- Fewer bugs
Declaring mutable variables in F#
let mutable name = "julia"
name <- "gulia"

Benefits of the "mutable" keyword
- Clearly indicate the variable is meant to be mutated
- Communicates the purpose of the variable
A data structure that always returns a new value when the old one is getting modified.
Hence, the data structures are immutable.
Isn't that really expensive?
Modify the data
Memory Sharing!


But isn't memory sharing bad??
Only when the data can be mutated
Benefits of using immutable data
- Encapsulation is not as important (read-only data does not need to be hidden)
- No more locks! There is no shared mutable state. No race condition!
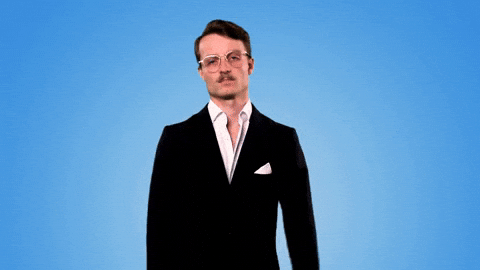
Random Numbers
function randomPlus (n) {
return n + Math.random() * 100
}

Things that worked on Friday but no longer on Monday

Thank you!
@ryoia
Get Programming With F# - Chapter 6 Immutable Data
By ryoia
Get Programming With F# - Chapter 6 Immutable Data
- 291