JavaScript
Beyond the Basics Pt II:
Revenge of the Basics


We expect cooperation from all participants to help ensure a safe environment for everybody.
We treat everyone with respect, we refrain from using offensive language and imagery, and we encourage to report any derogatory or offensive behavior to a member of the JSLeague community.
We provide a fantastic environment for everyone to learn and share skills regardless of gender, gender identity and expression, age, sexual orientation, disability, physical appearance, body size, race, ethnicity, religion (or lack thereof), or technology choices.
We value your attendance and your participation in the JSLeague community and expect everyone to accord to the community Code of Conduct at all JSLeague workshops and other events.
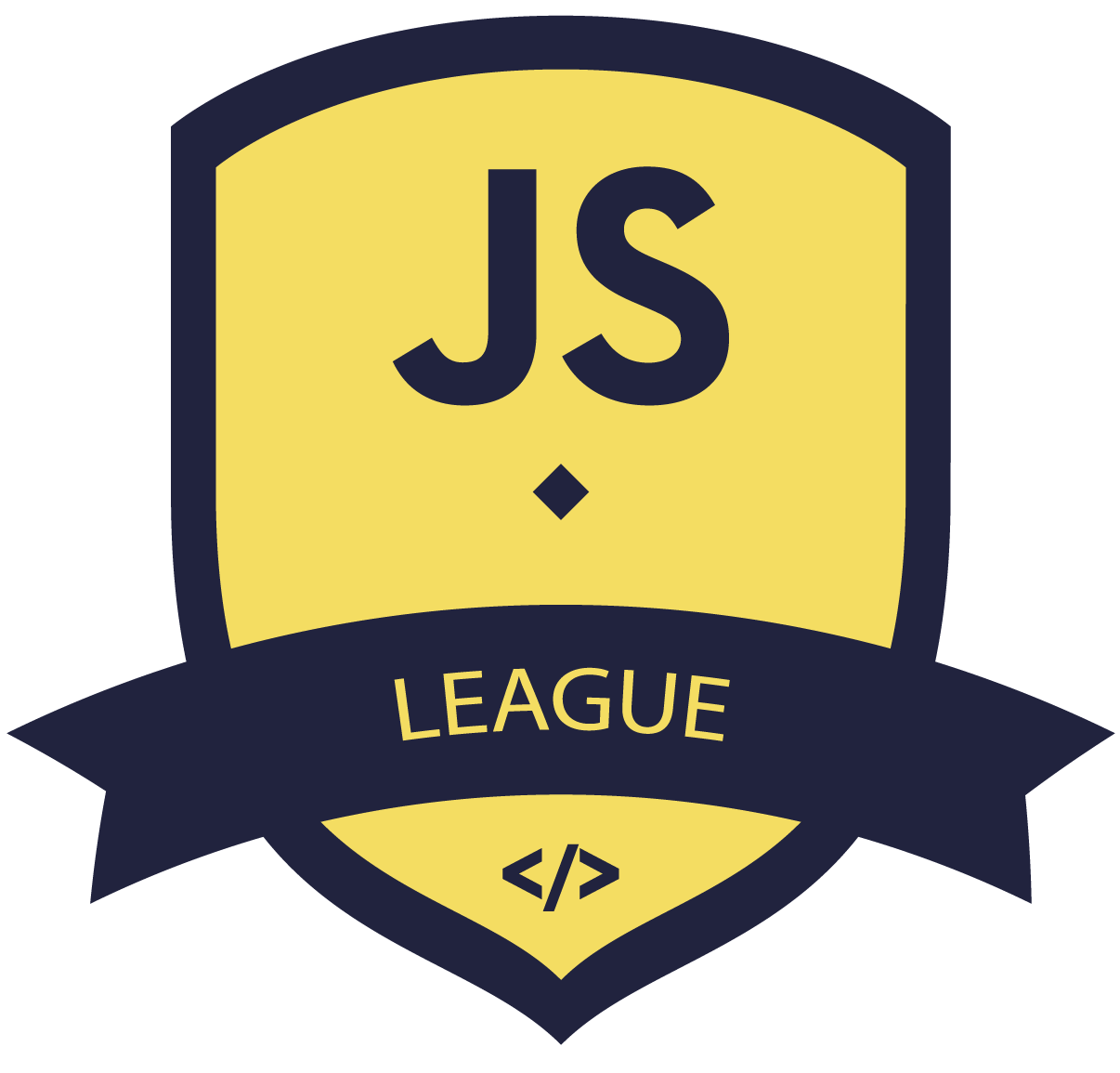
Code of conduct
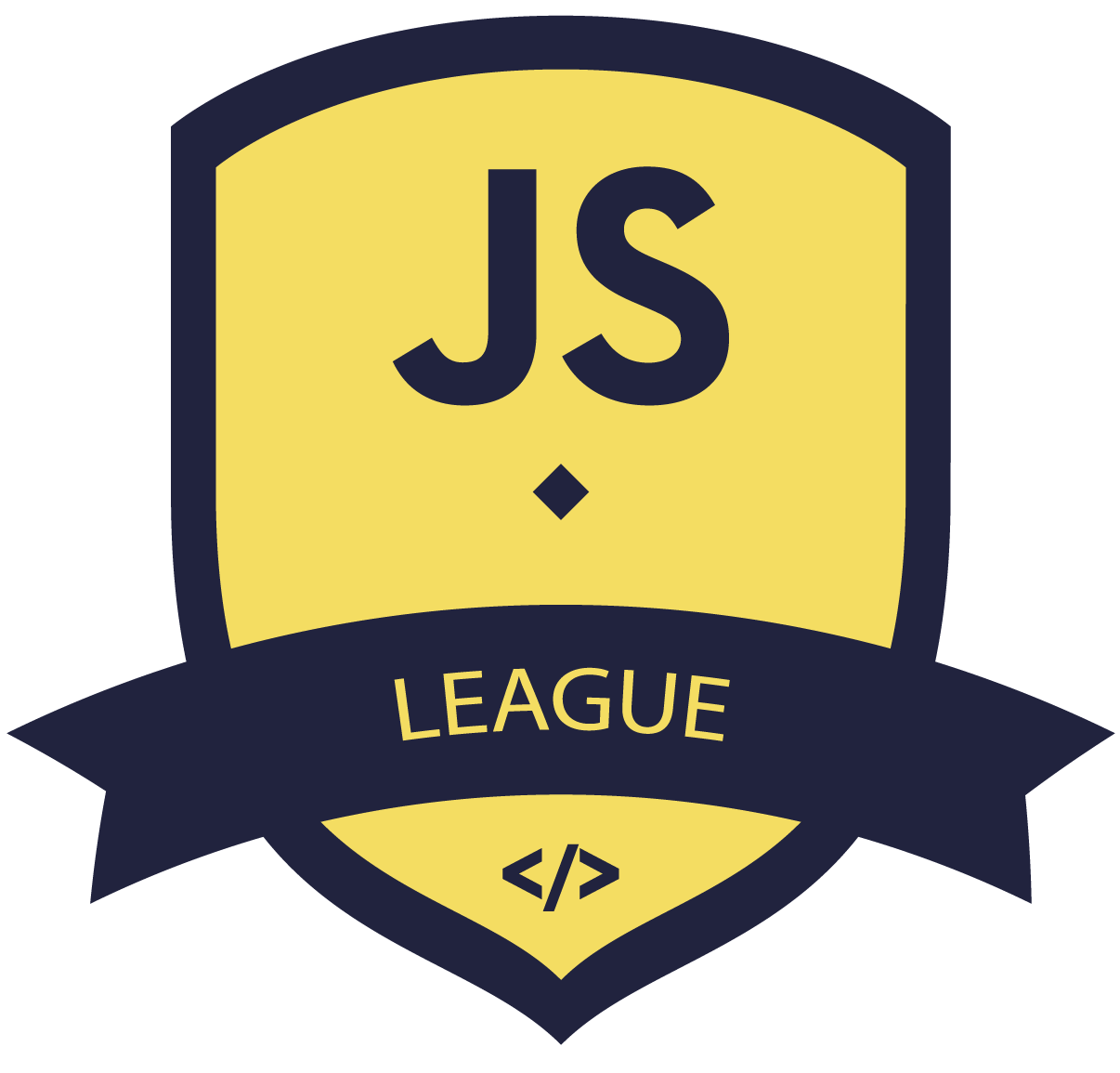
Whoami
Sabin Marcu
Senior Software Engineer @ Hootsuite
Been working in web since '09
React Dev since 2015
React, GraphQL trainer @ JSLeague

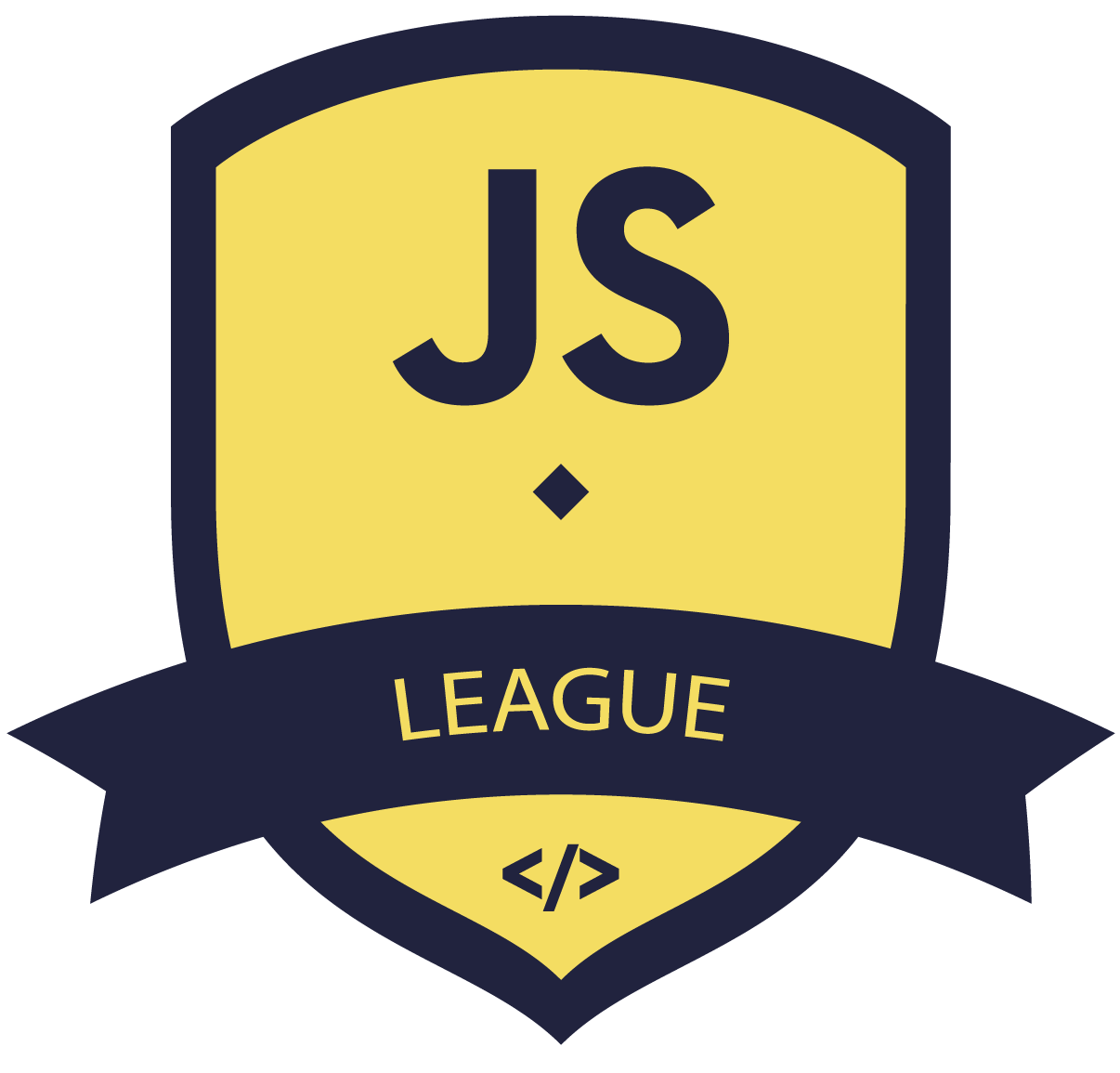
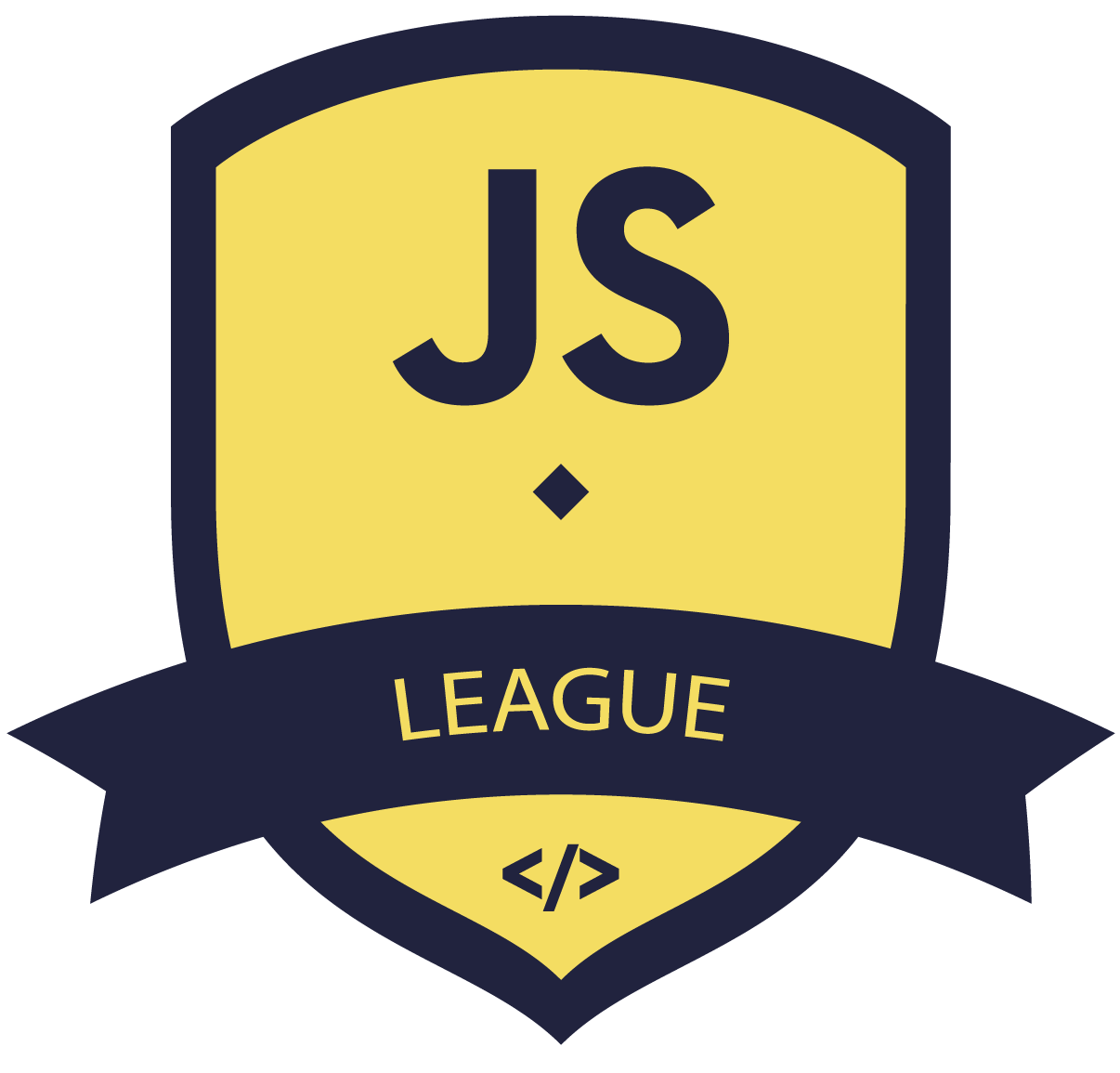
Basic concepts of JS
JavaScript Beyond the Basics
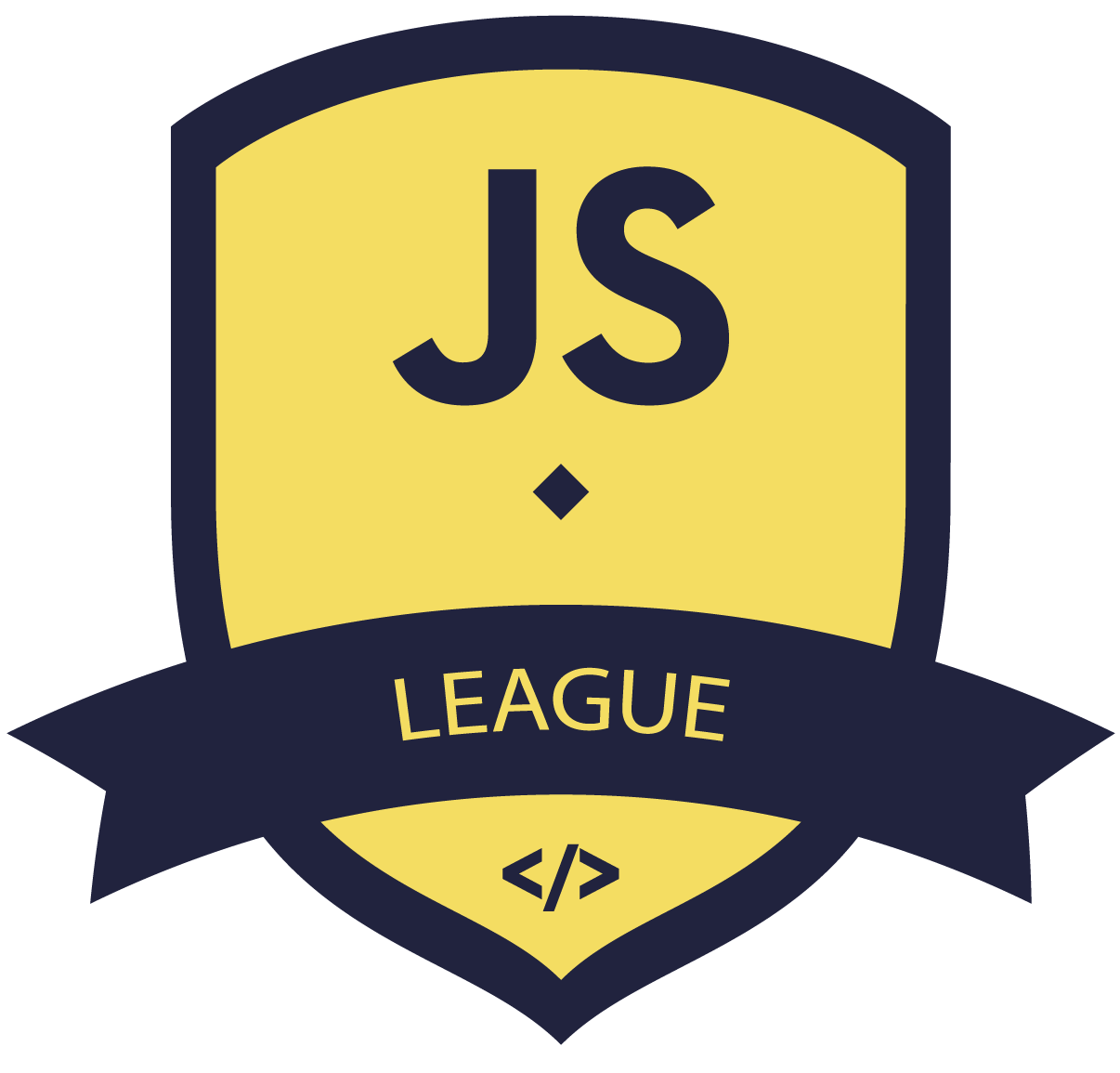
JavaScript Beyond the Basics
Data types
primitives
objects
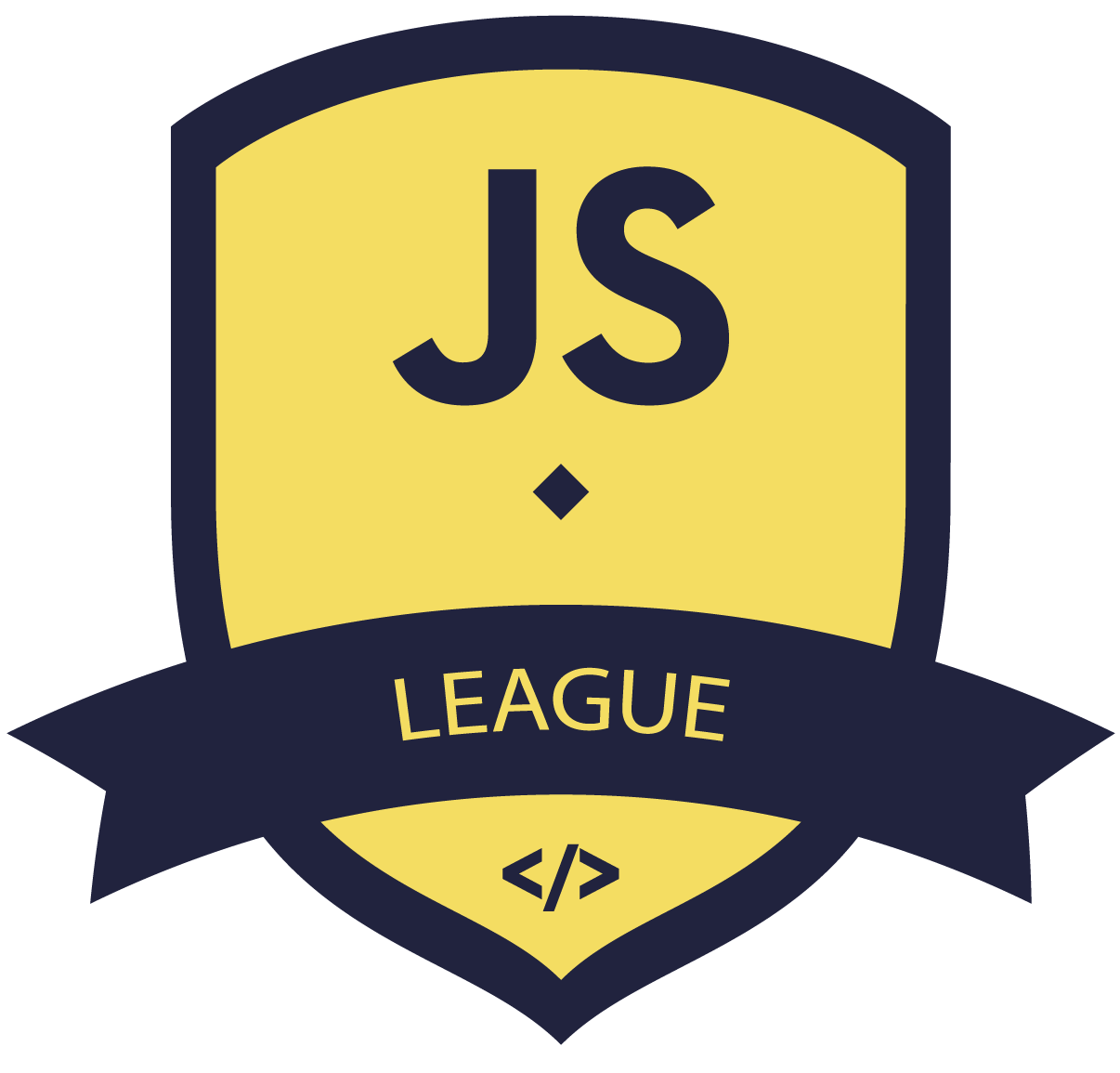
JavaScript Beyond the Basics
Type Coercion
is the process of converting value from one type to another (such as string to number, object to boolean, and so on).
End result of coercion will always be either a string, a number or a boolean value
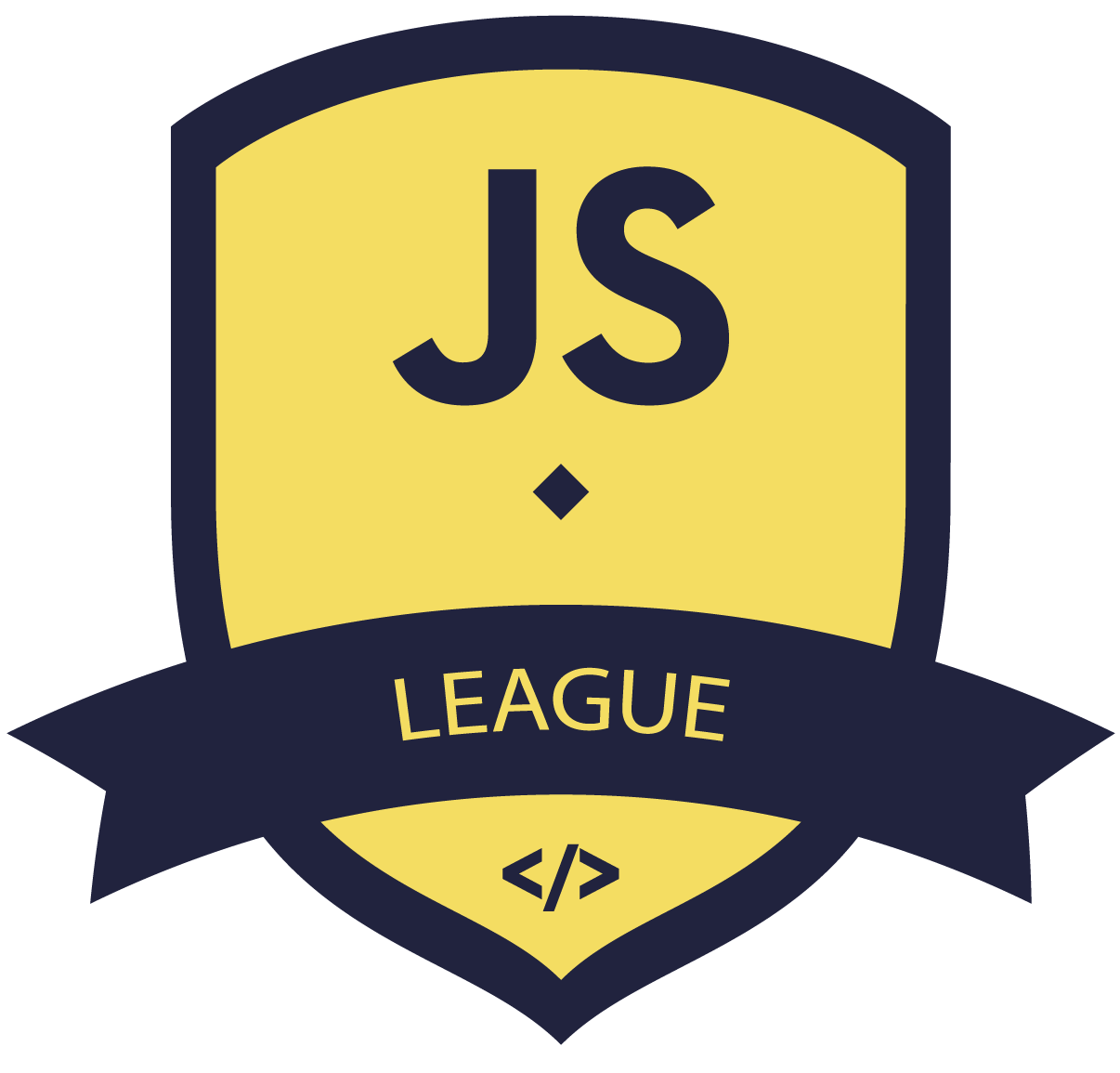
JavaScript Beyond the Basics
Implicit vs. Explicit
Number("5")
String(true)
Boolean(31)
5 + "3"
true + false
"6" - 1
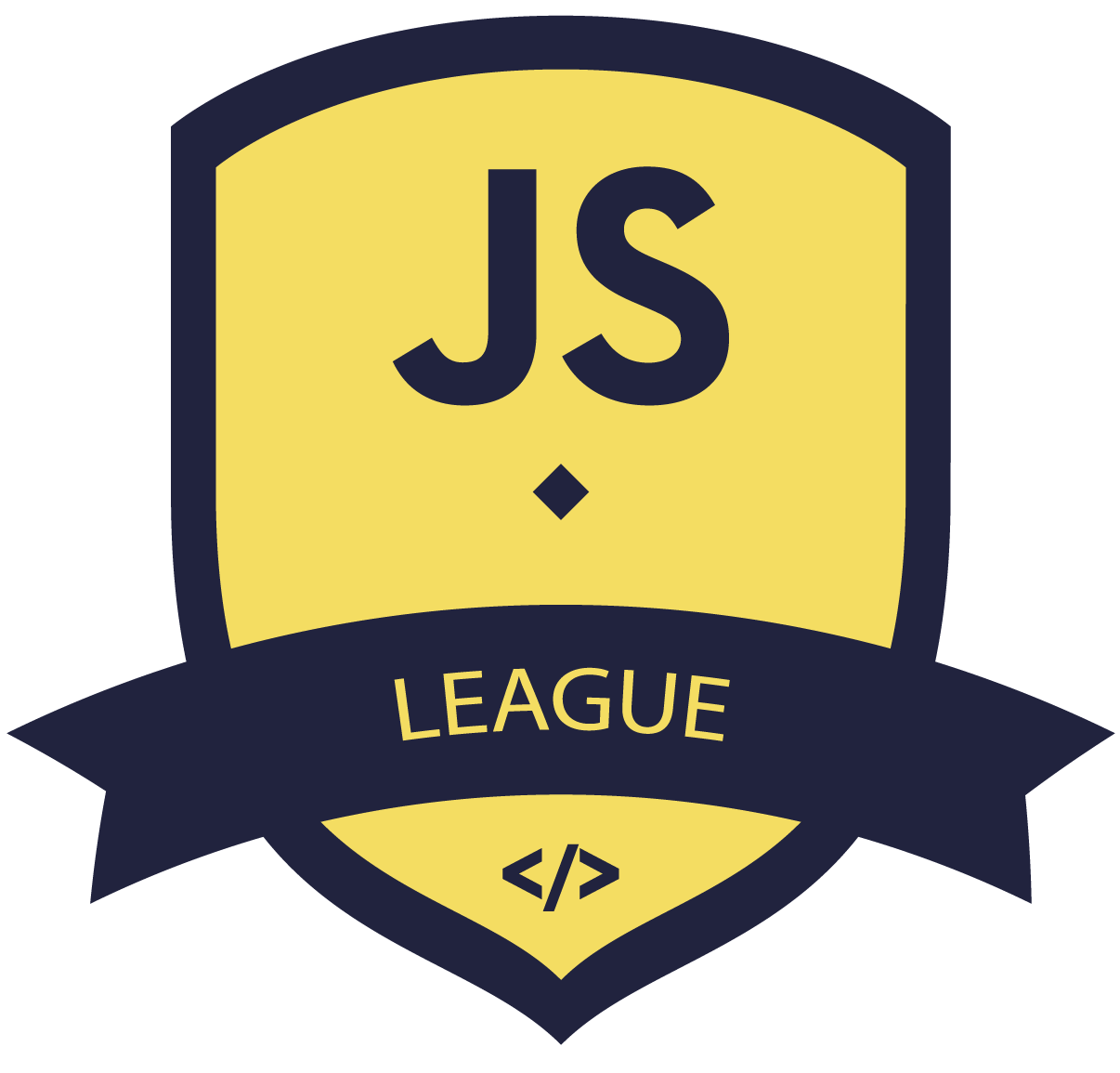
JavaScript Beyond the Basics
Loose vs Strict Equality
(== vs. ===)
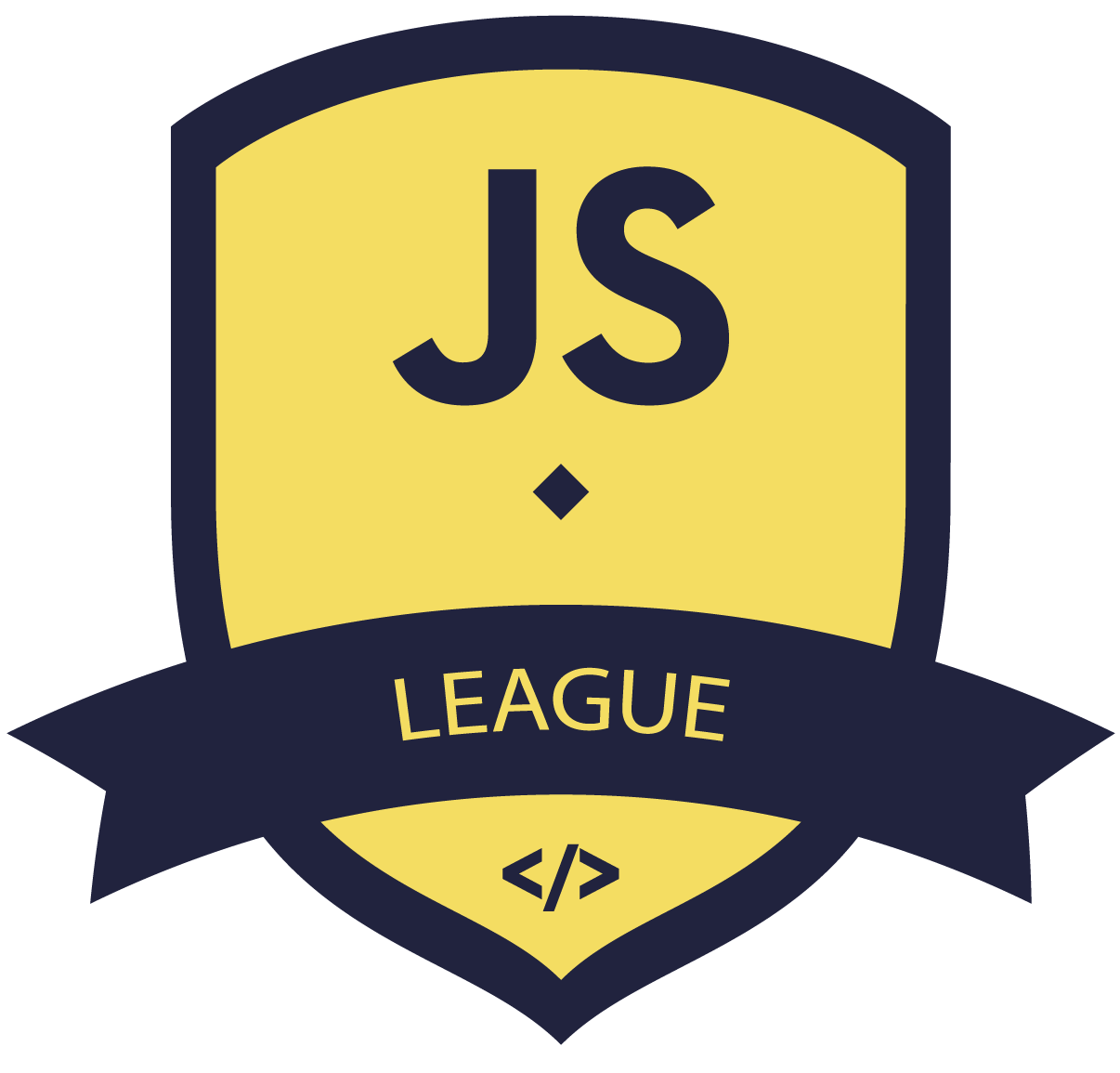
JavaScript Beyond the Basics
False positives
false, 0, "", null, undefined, NaN
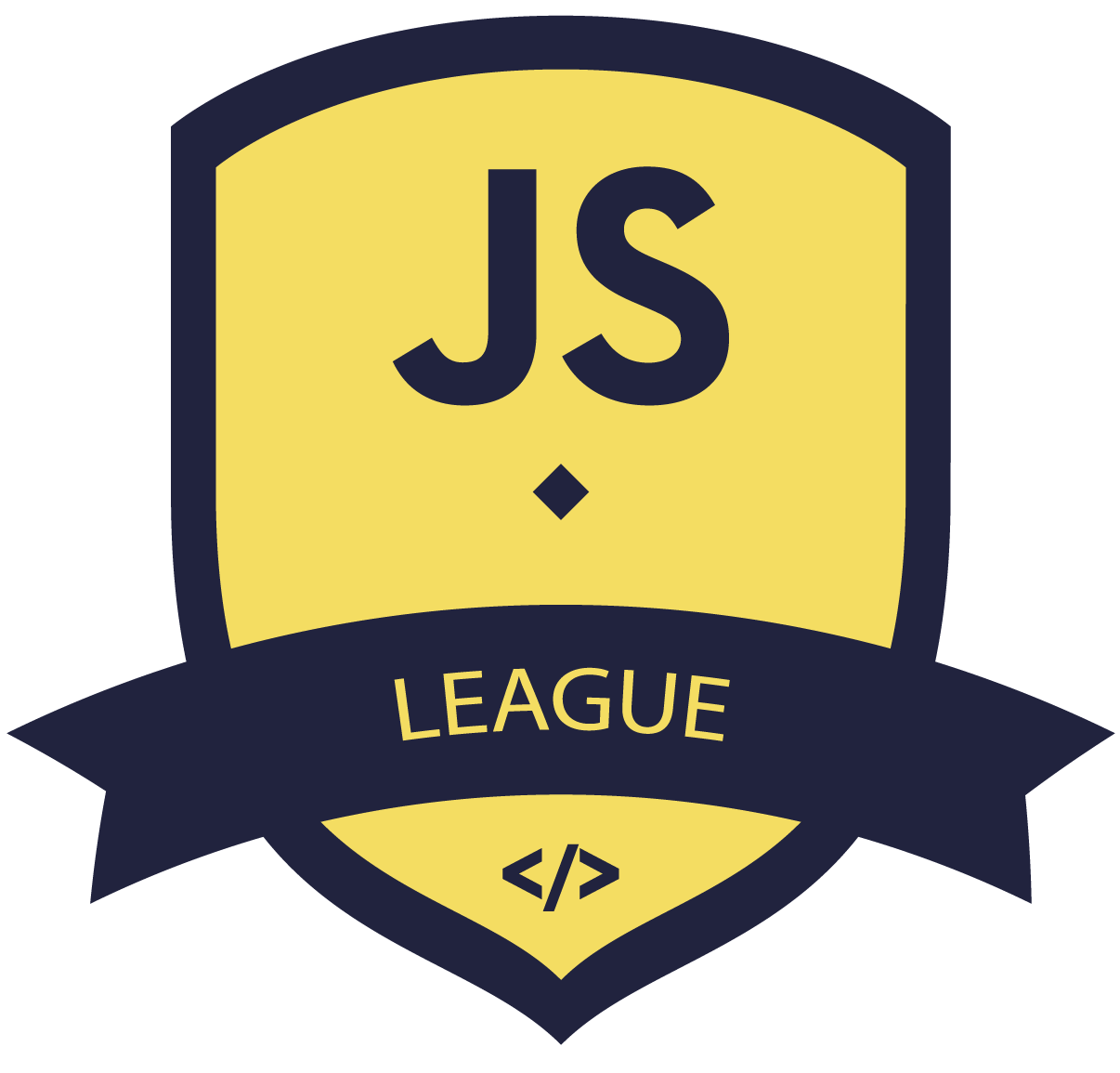
Scope and "this"
JavaScript Beyond the Basics
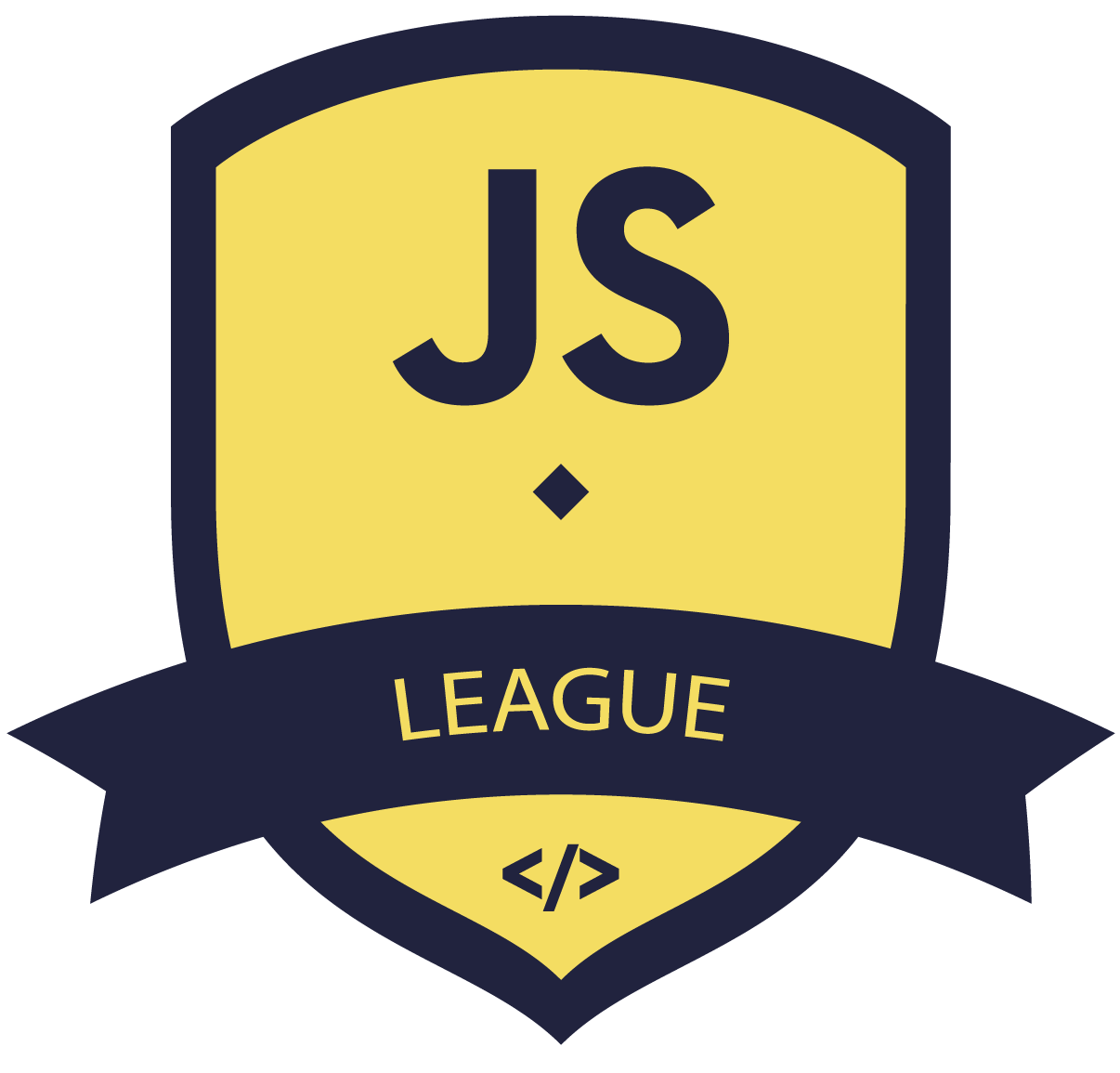
JavaScript Beyond the Basics
Scope
global
function
block
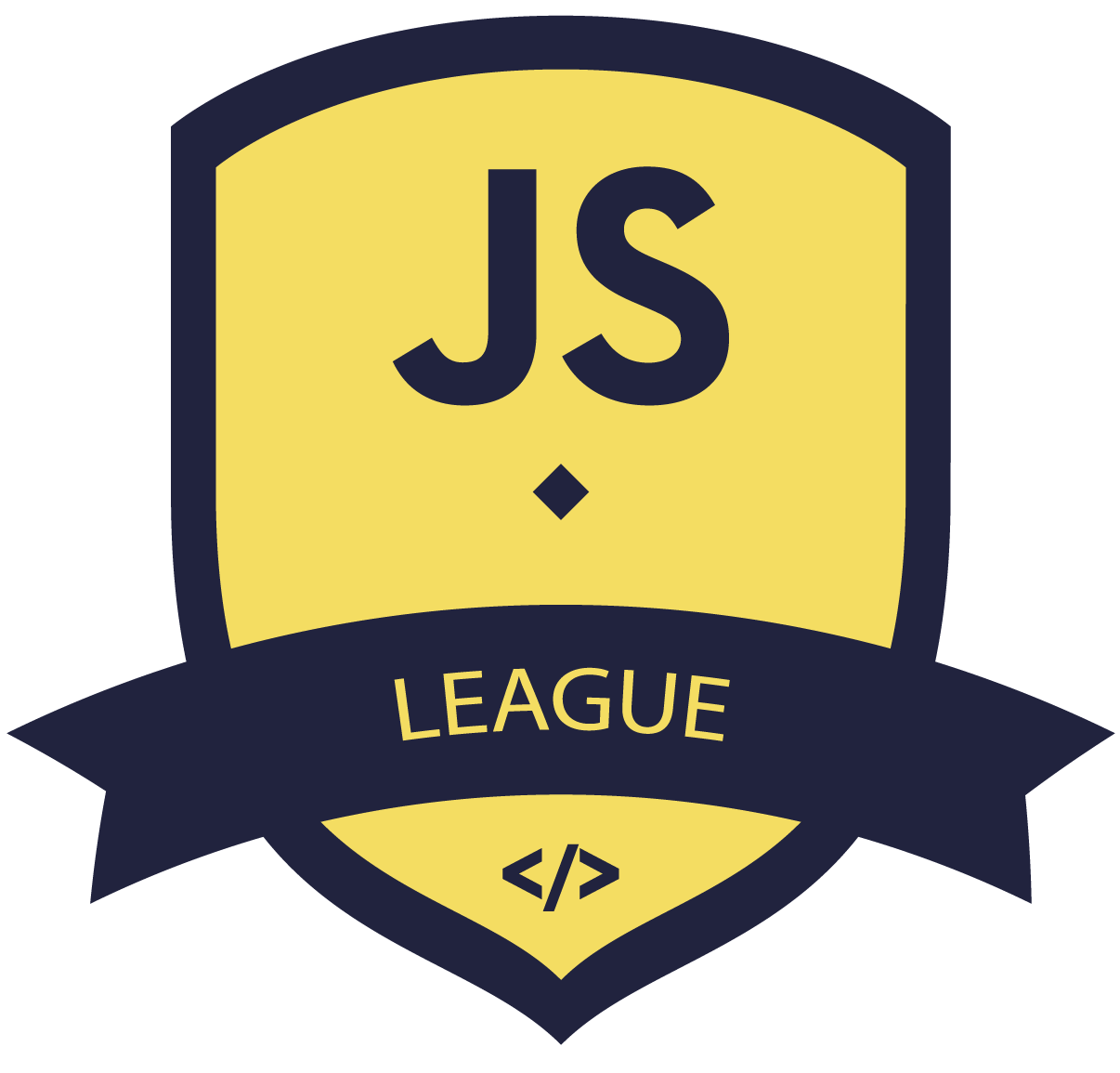
JavaScript Beyond the Basics
this
at root level
*unless strict mode
browser == window
node == global
at function level
call, apply
bind
arrow function
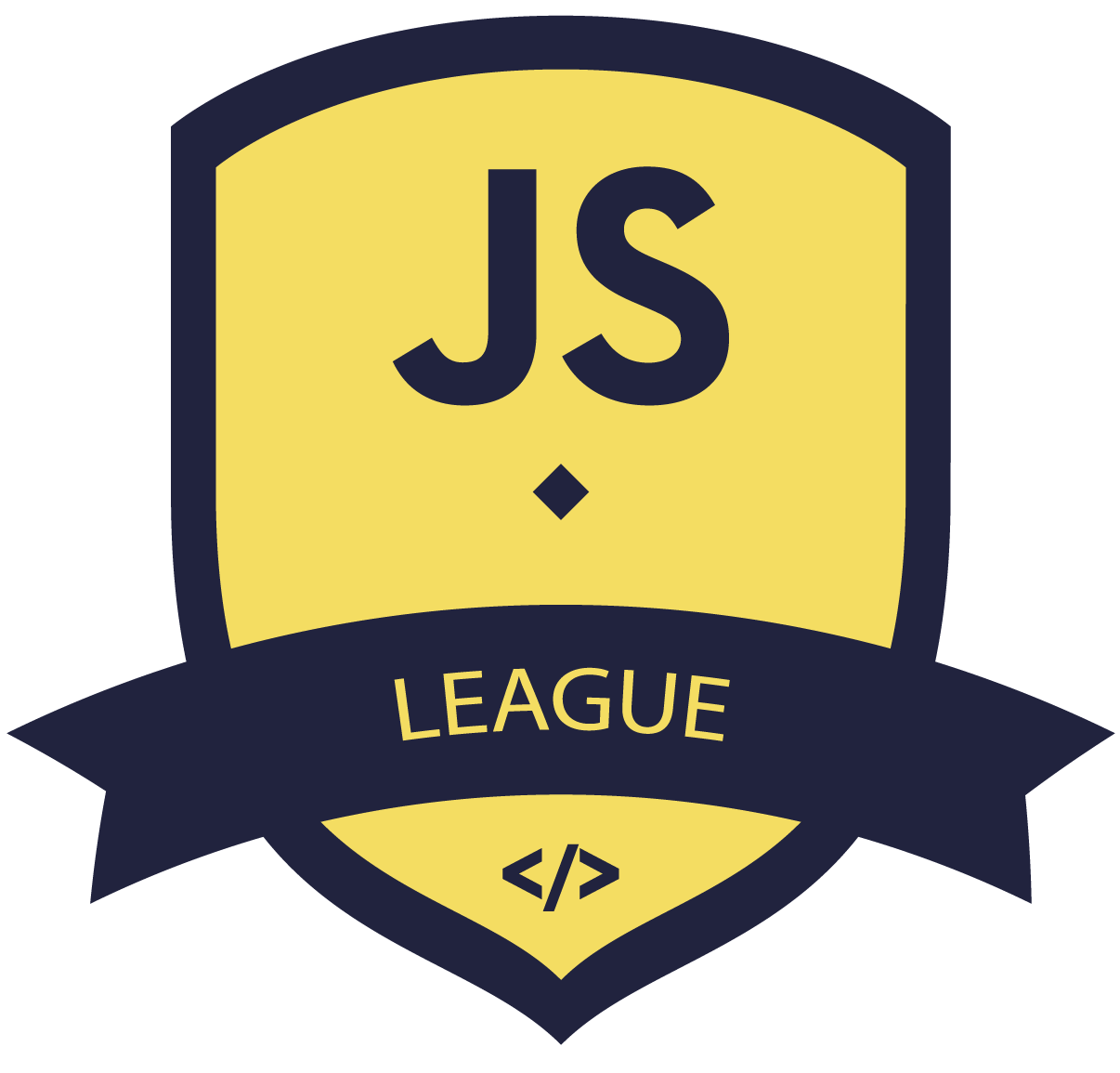
Destructuring, spread, rest
JavaScript Beyond the Basics
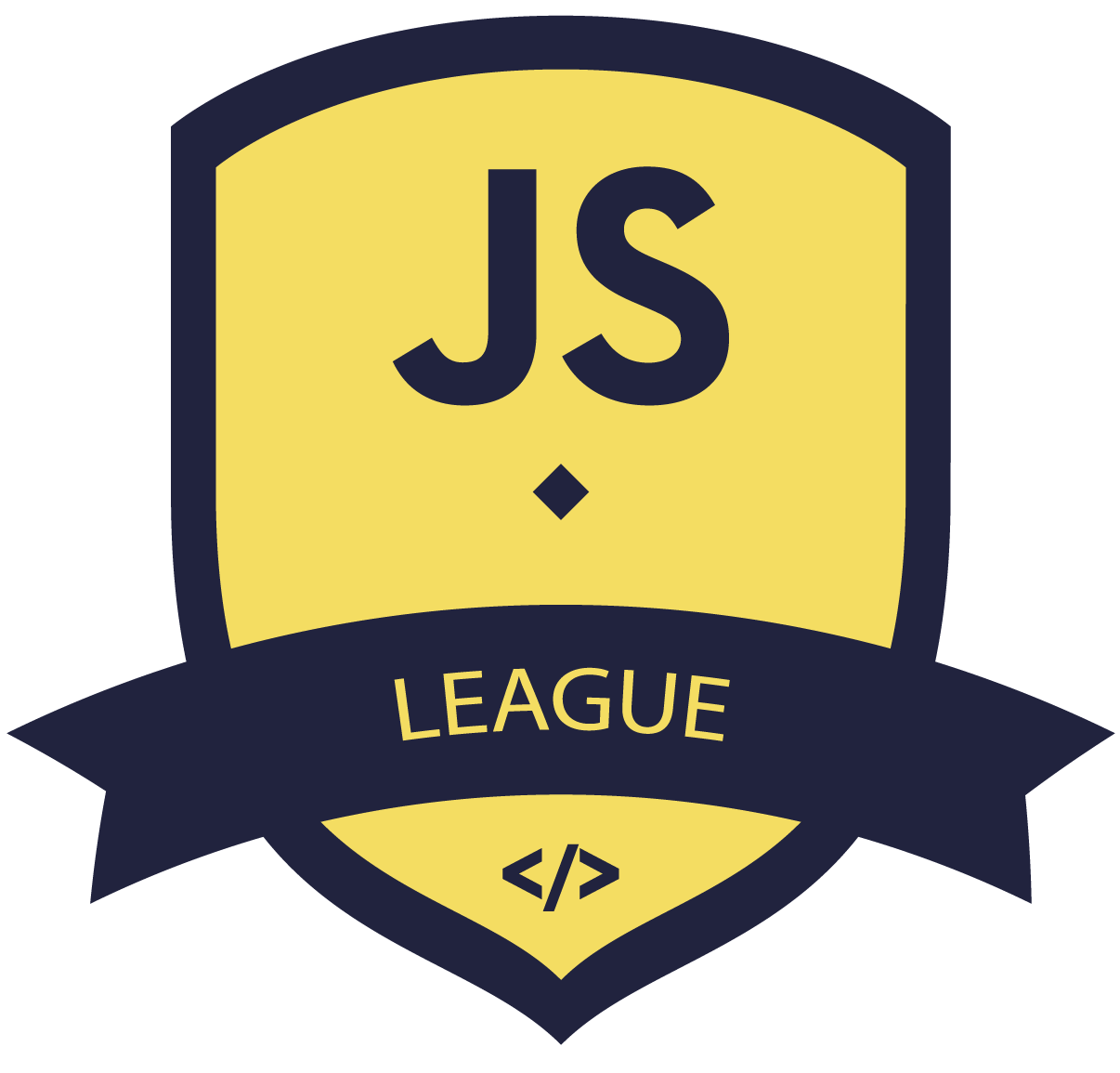
JavaScript Beyond the Basics
Destructuring
breaking down objects and arrays
into named variables
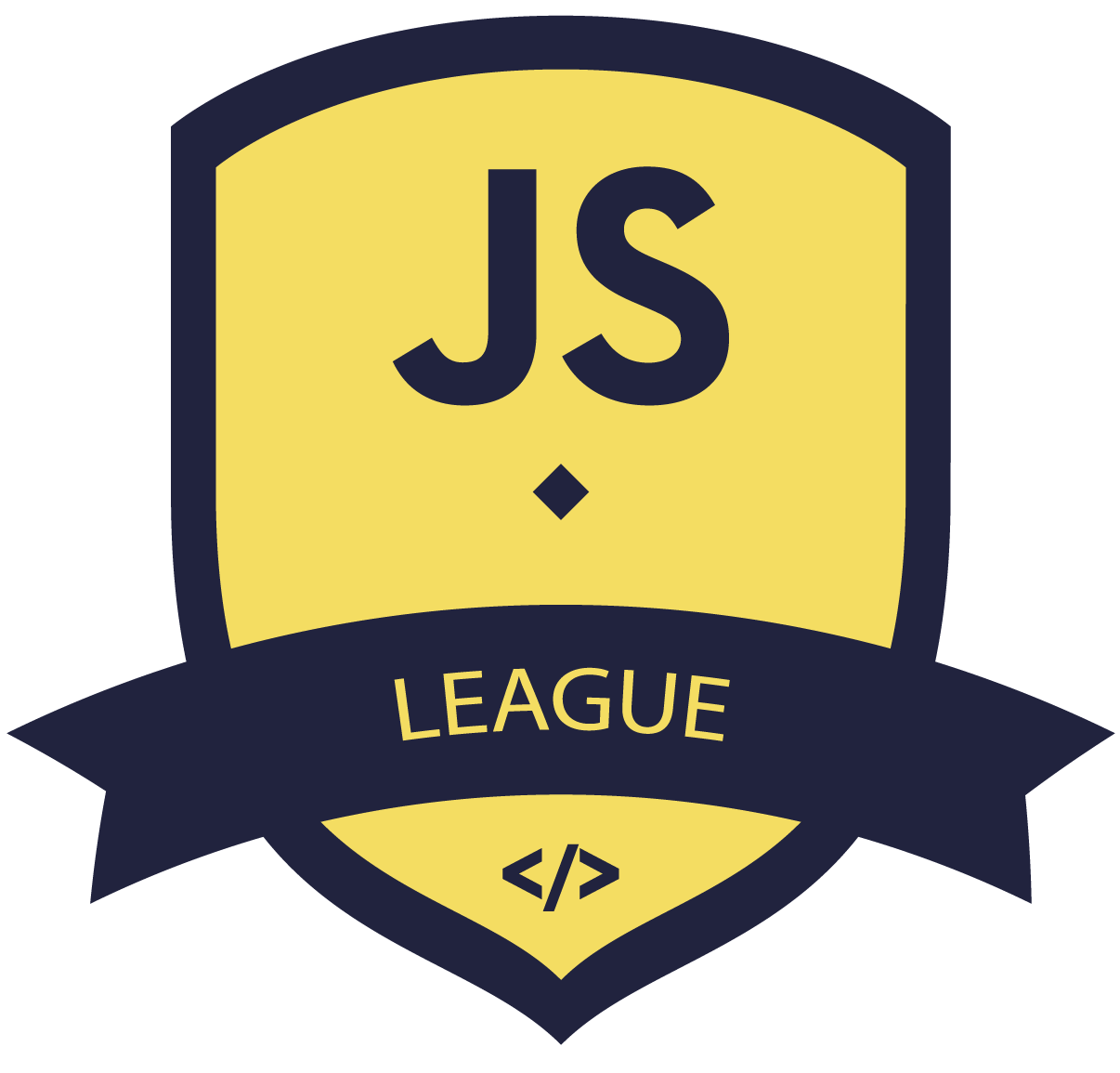
JavaScript Beyond the Basics
Rest
aggregation of remaining params
into a single variable
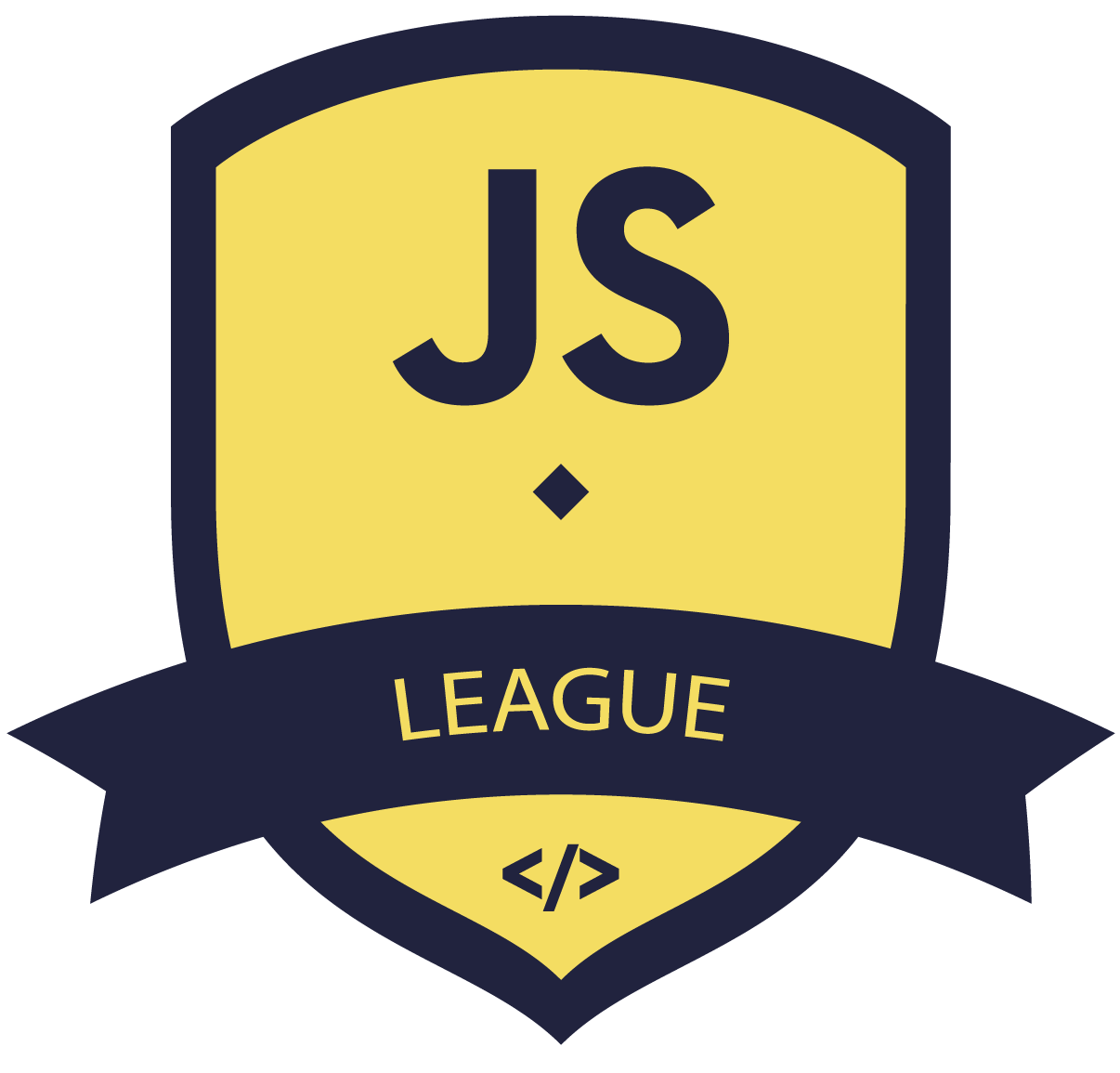
JavaScript Beyond the Basics
Spread
unpacking (or expanding)
iterable collections
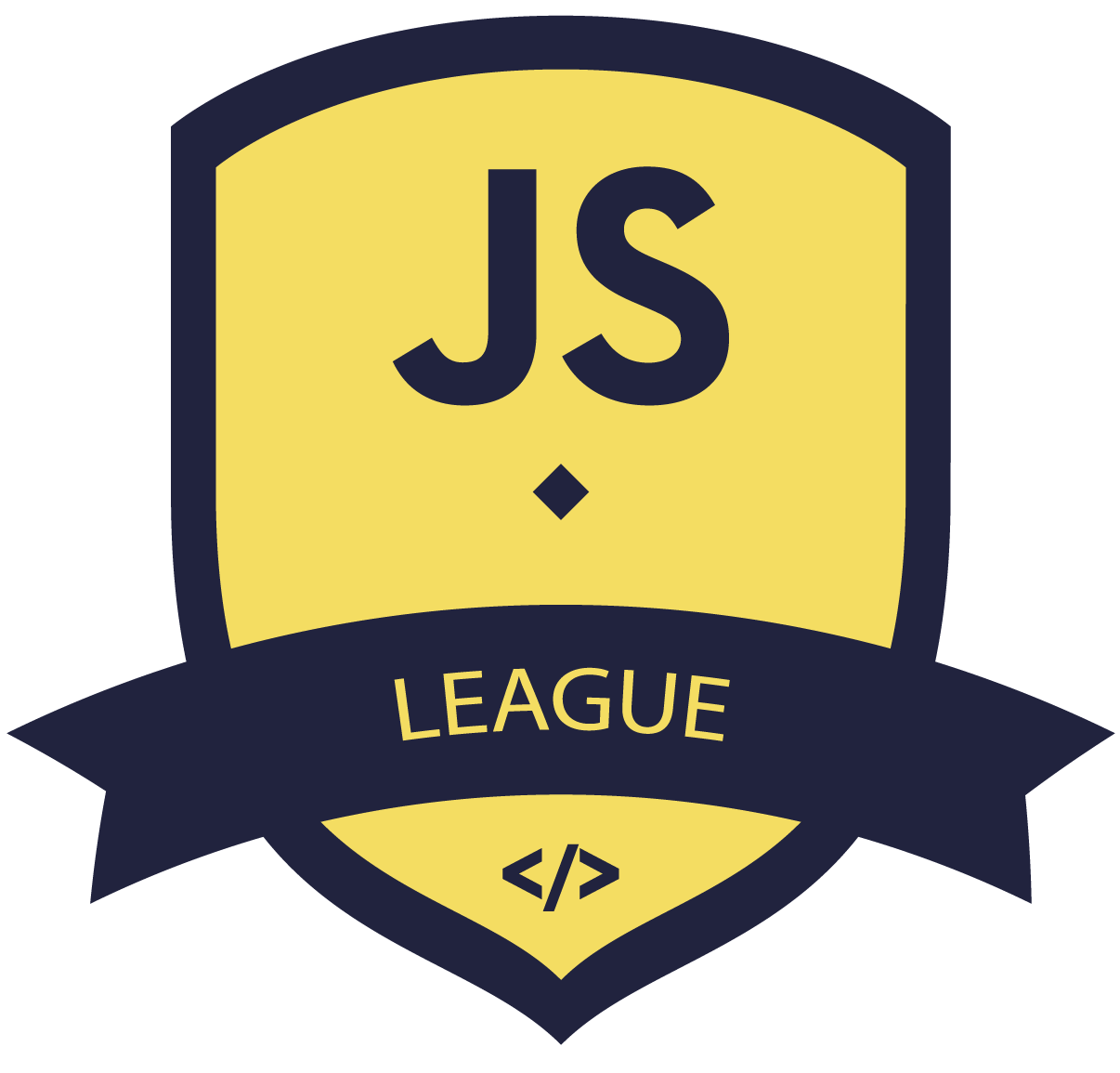
Arrow functions
JavaScript Beyond the Basics
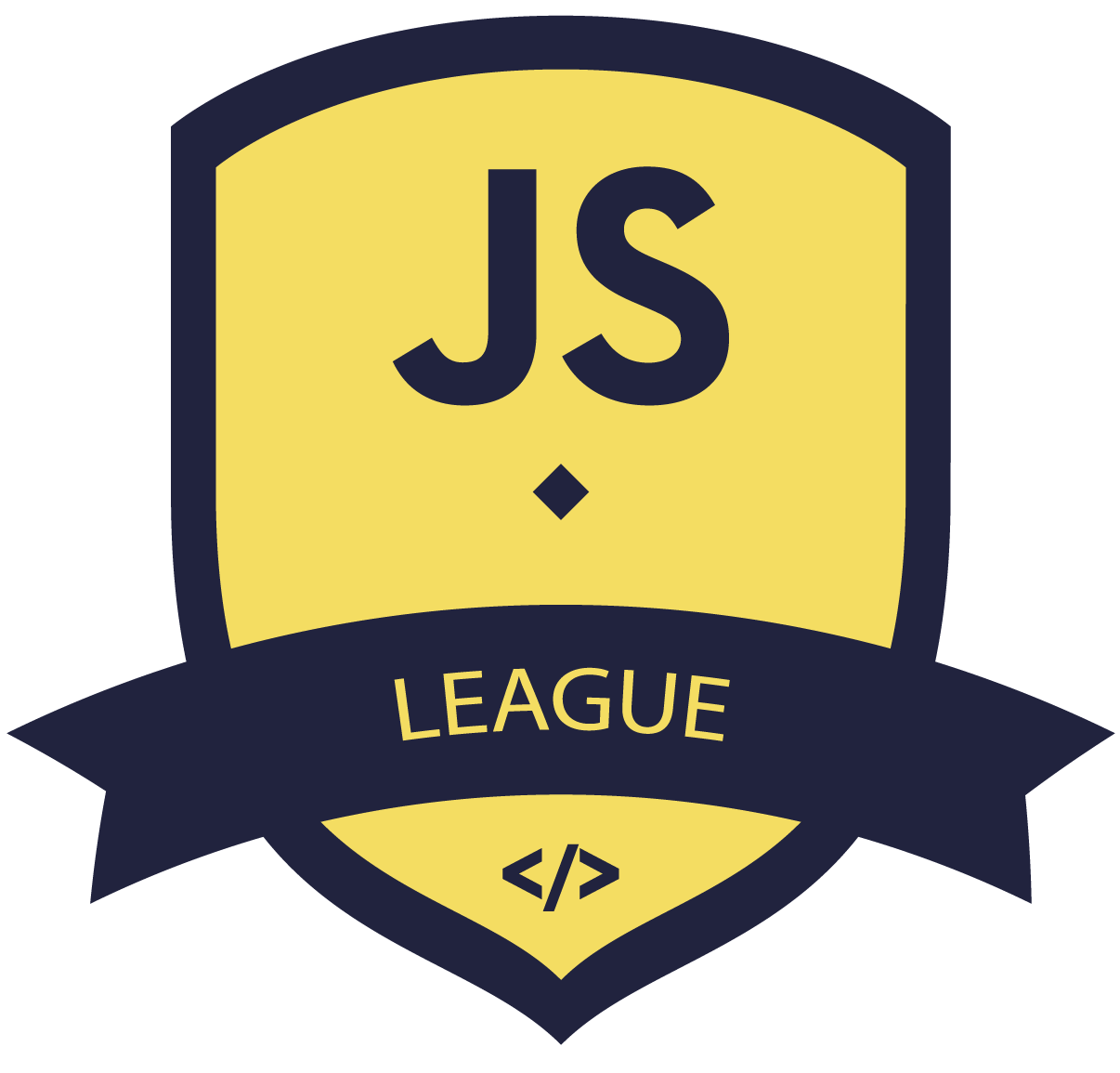
JavaScript Beyond the Basics
lexical this
cannot be used as constructors
(param1, param2, …, paramN) => { statements }
shorter syntax
no arguments
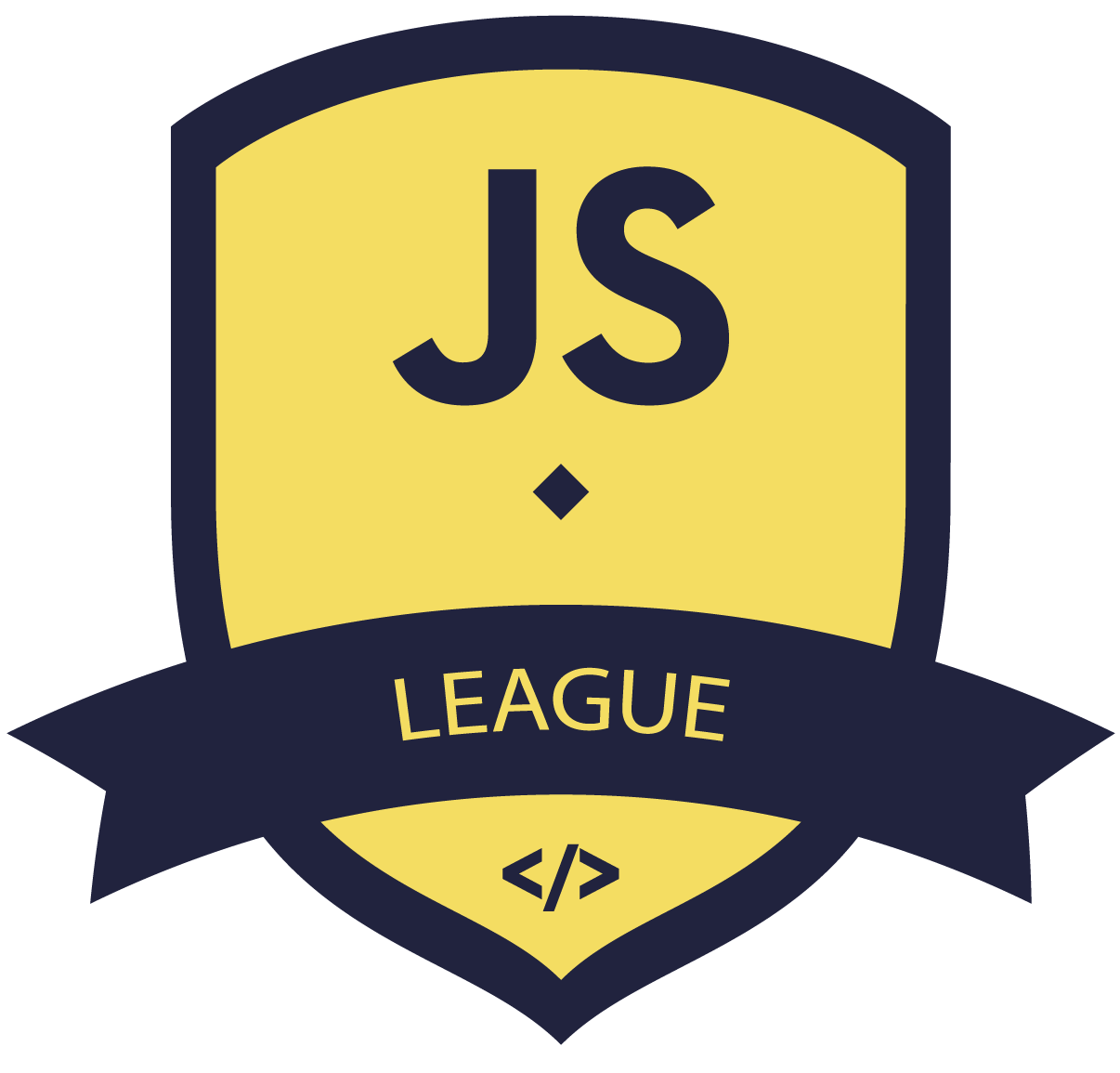
JavaScript Beyond the Basics
ARTICLE = { ID, TITLE, CONTENT }
const titles = articles.map(function (article) {
return article.title;
});
ARTICLE = { ID, TITLE, CONTENT }
const titles = articles.map(article => {
return article.title;
});
ARTICLE = { ID, TITLE, CONTENT }
const titles = articles.map(article => article.title);
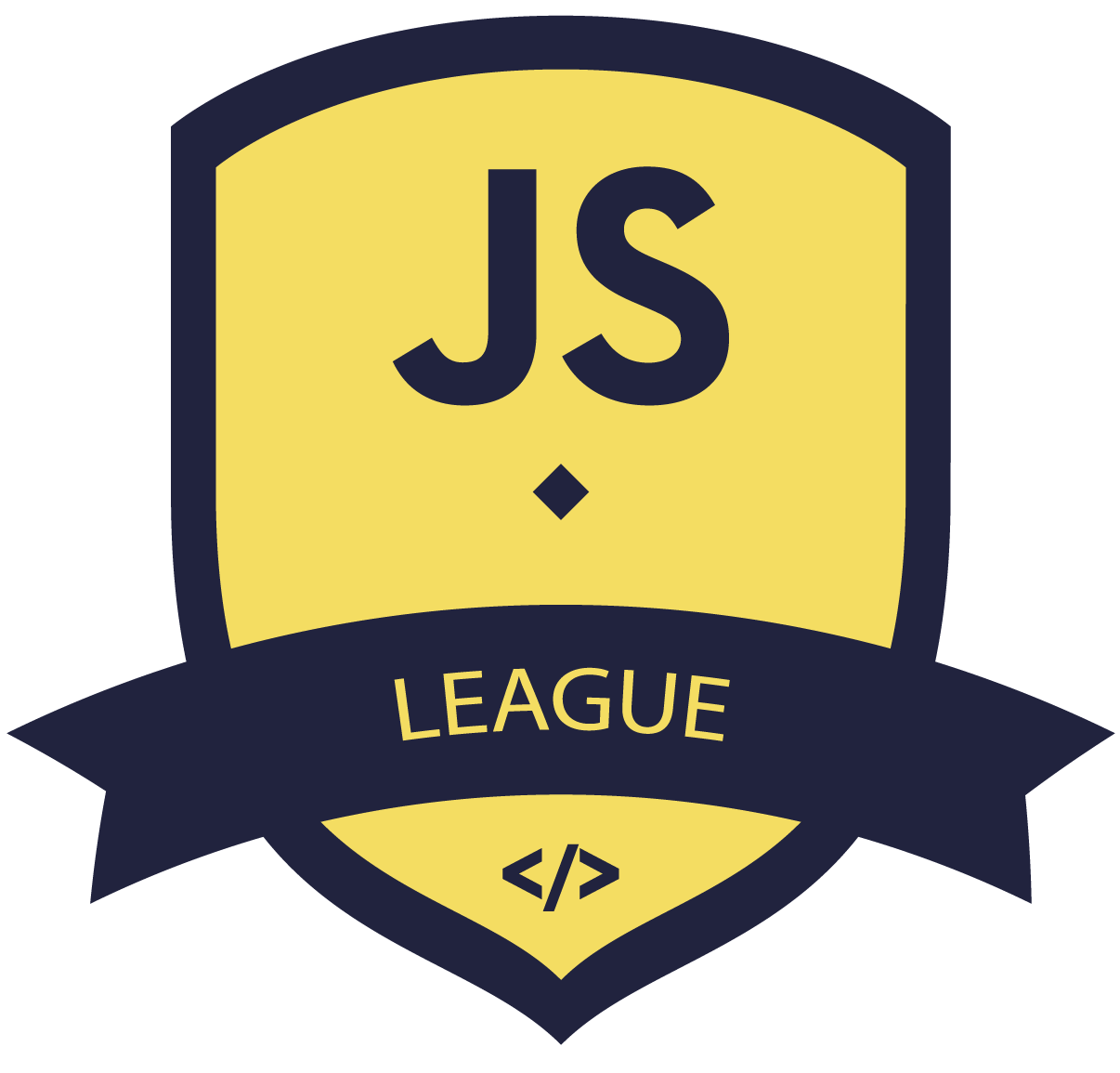
Template literals
JavaScript Beyond the Basics
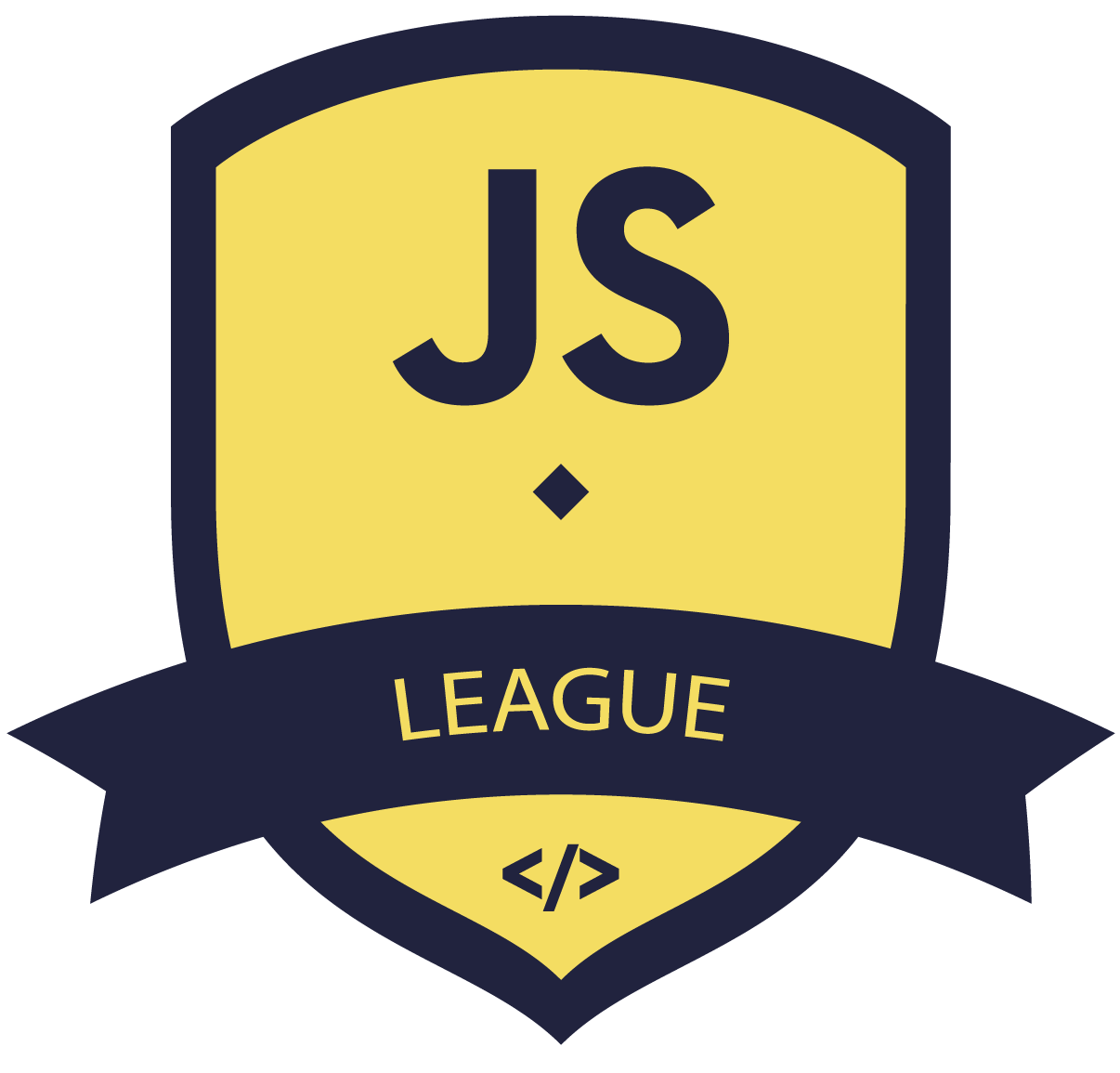
JavaScript Beyond the Basics
string interpolation
multiline
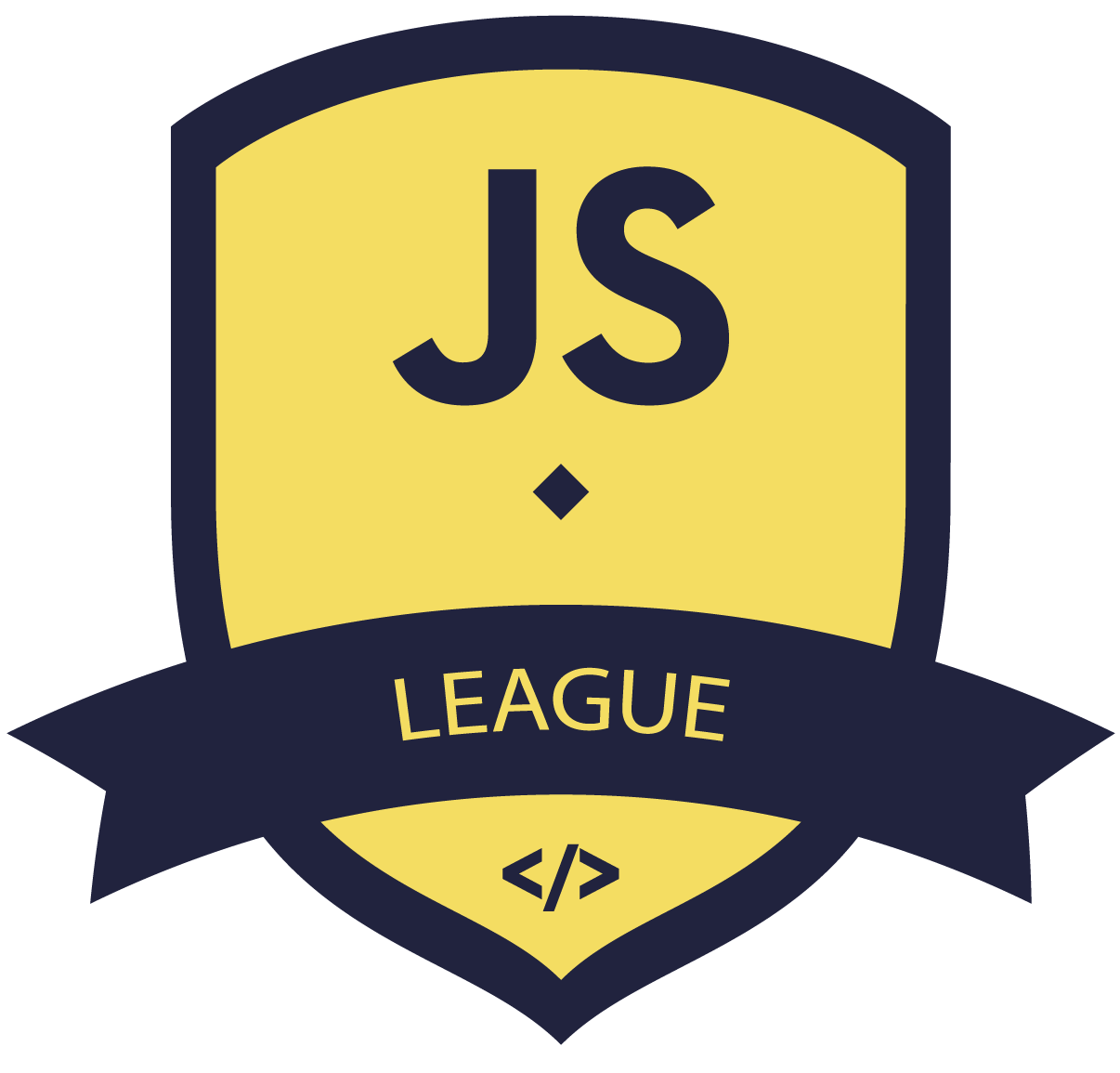
Callbacks, promises, async
JavaScript Beyond the Basics
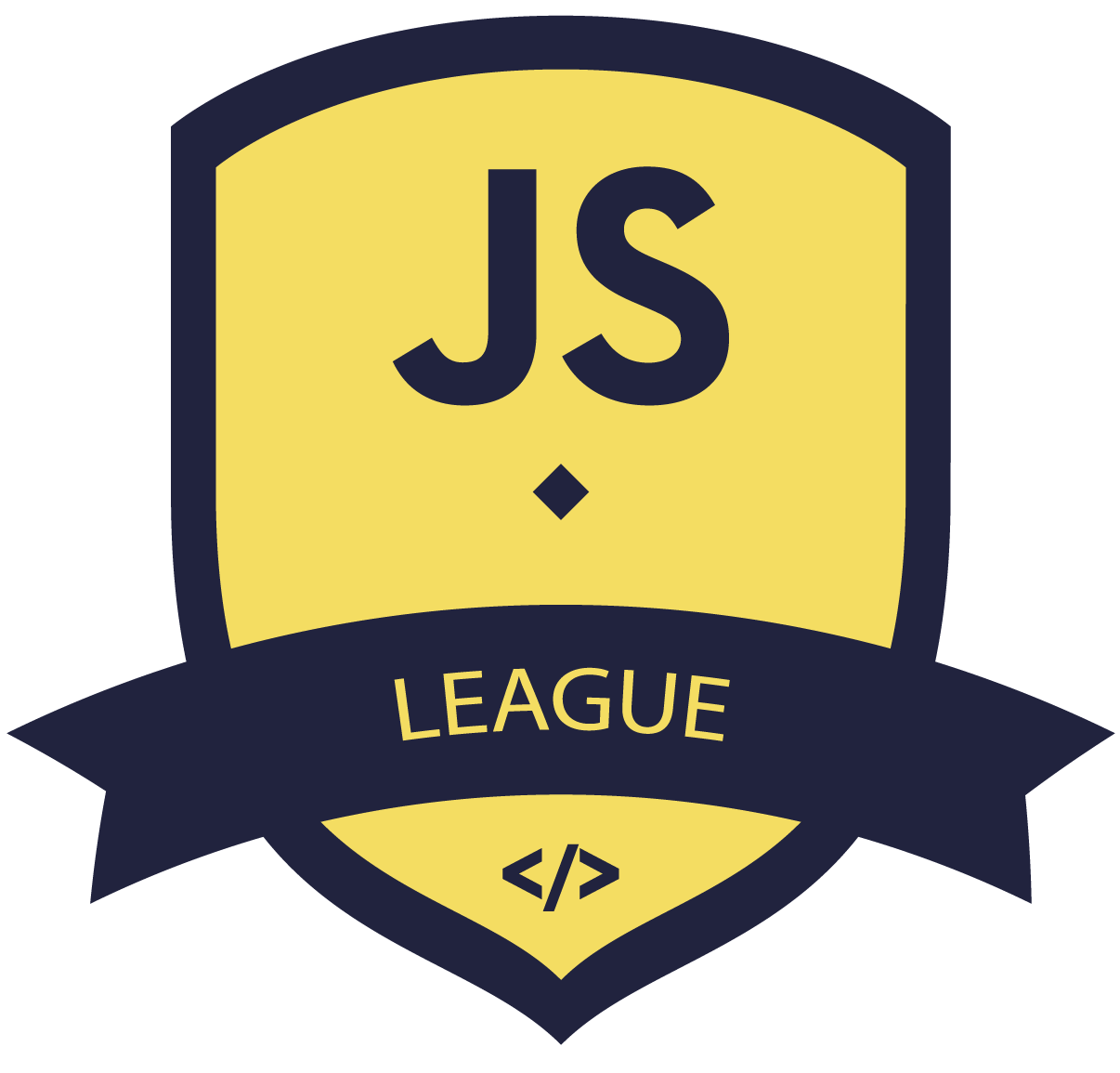
JavaScript Beyond the Basics
Callback
a function that is triggered
and resolved
at the end of another function
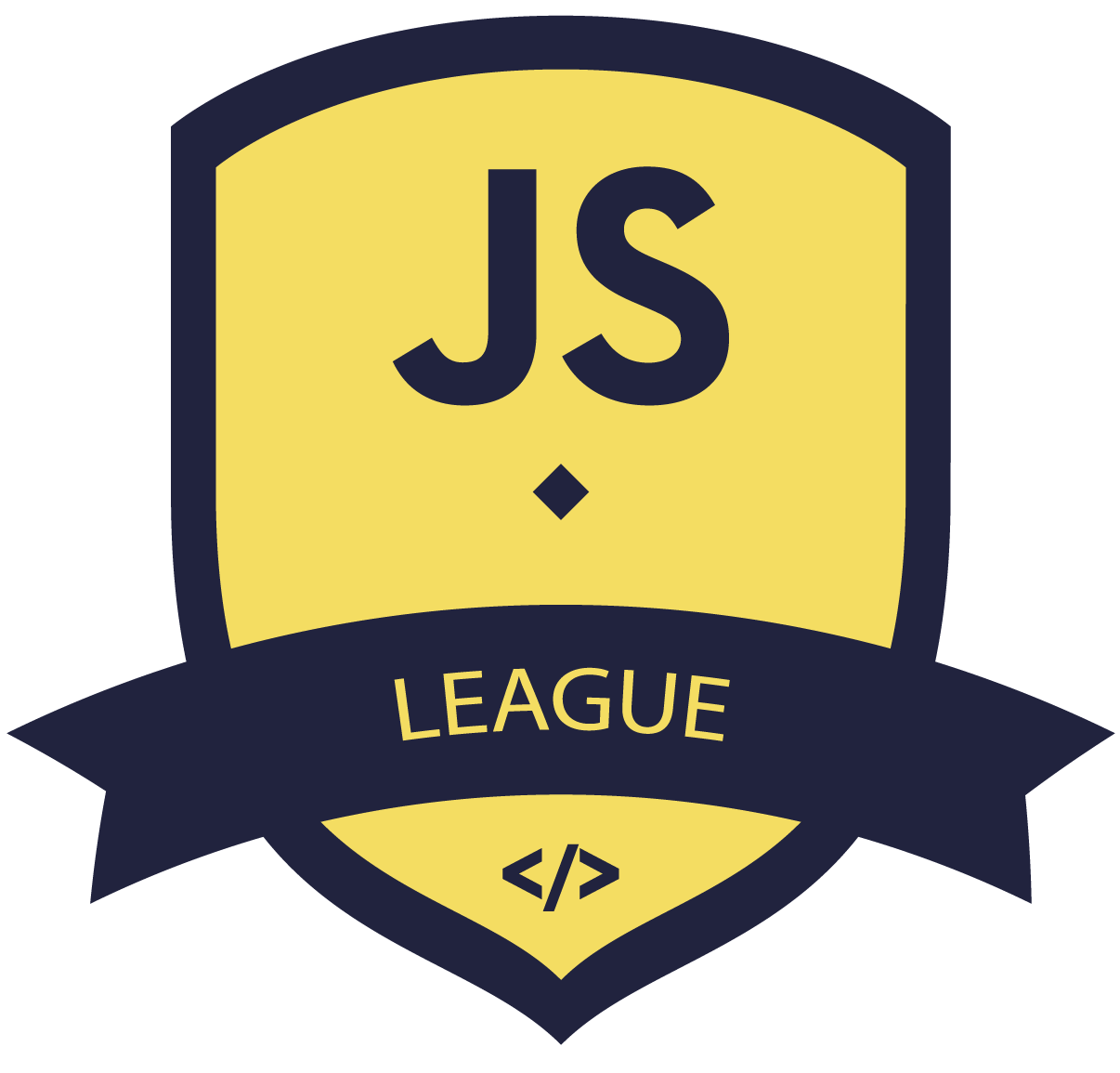
JavaScript Beyond the Basics
Promise
a fulfillment of an already started action ending in a resolve or failure
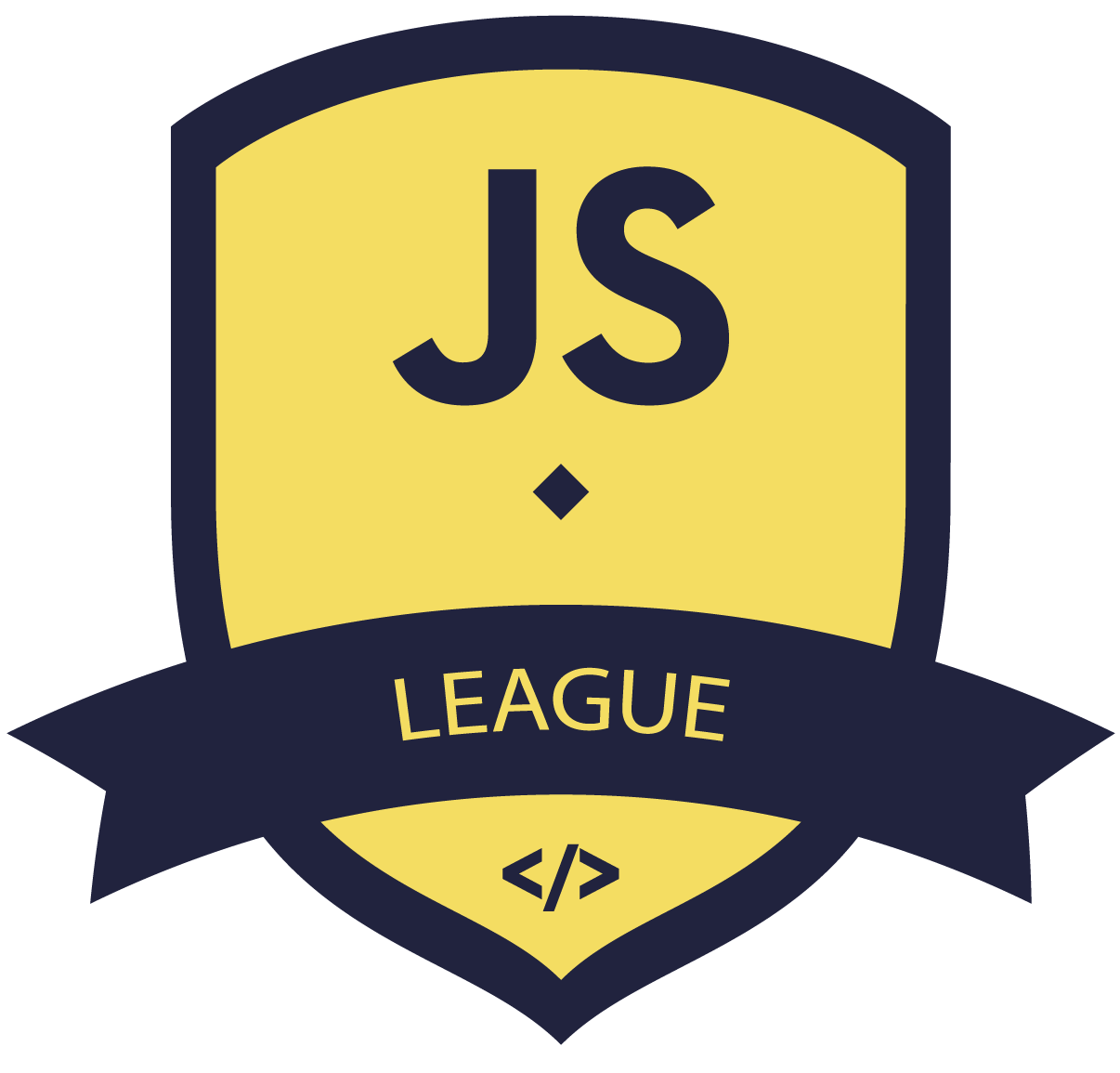
JavaScript Beyond the Basics
Async
similar to promise
but now its called async*
*also now it actually looks like js code
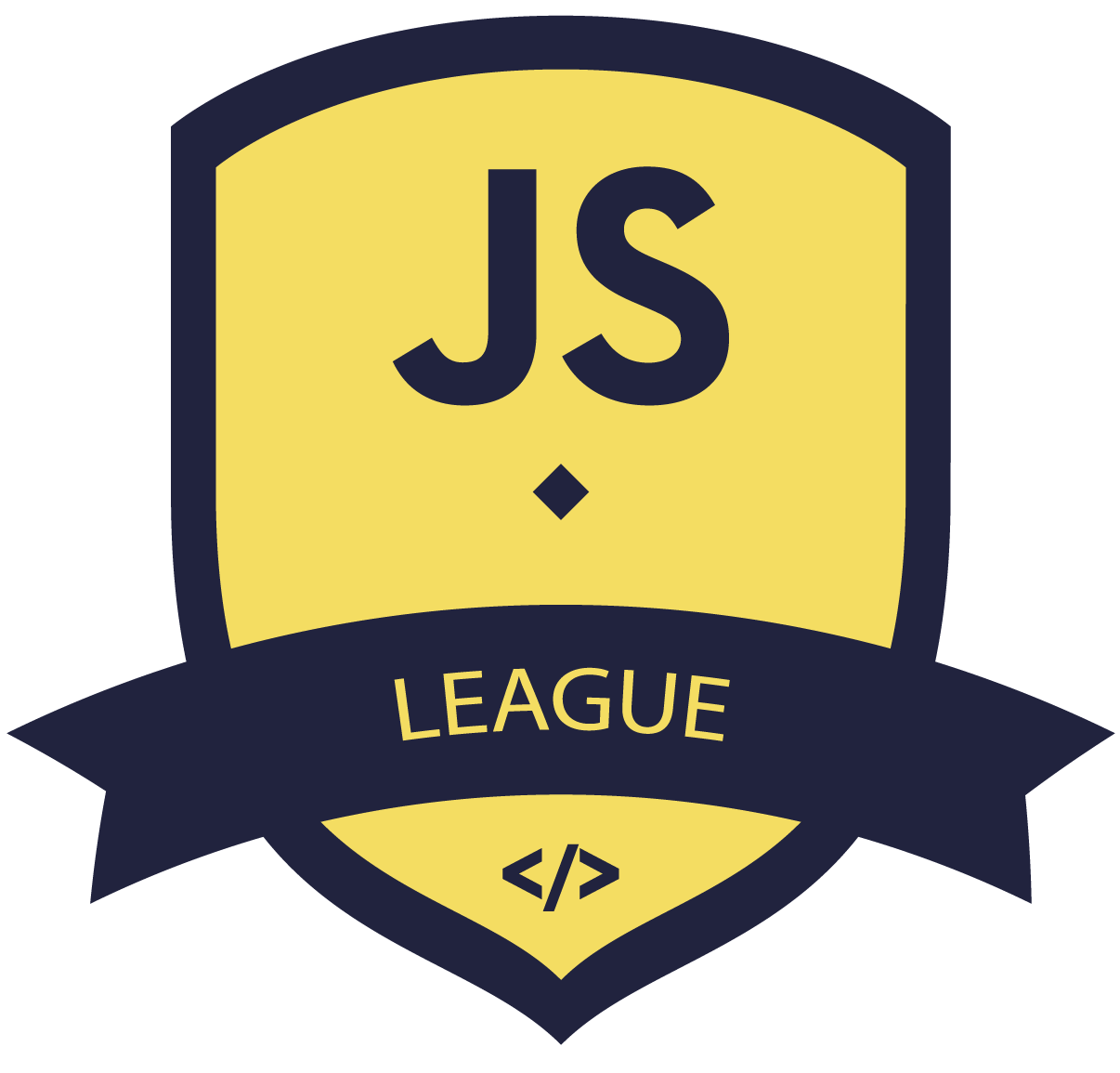
JavaScript Beyond the Basics
Closures
function inception
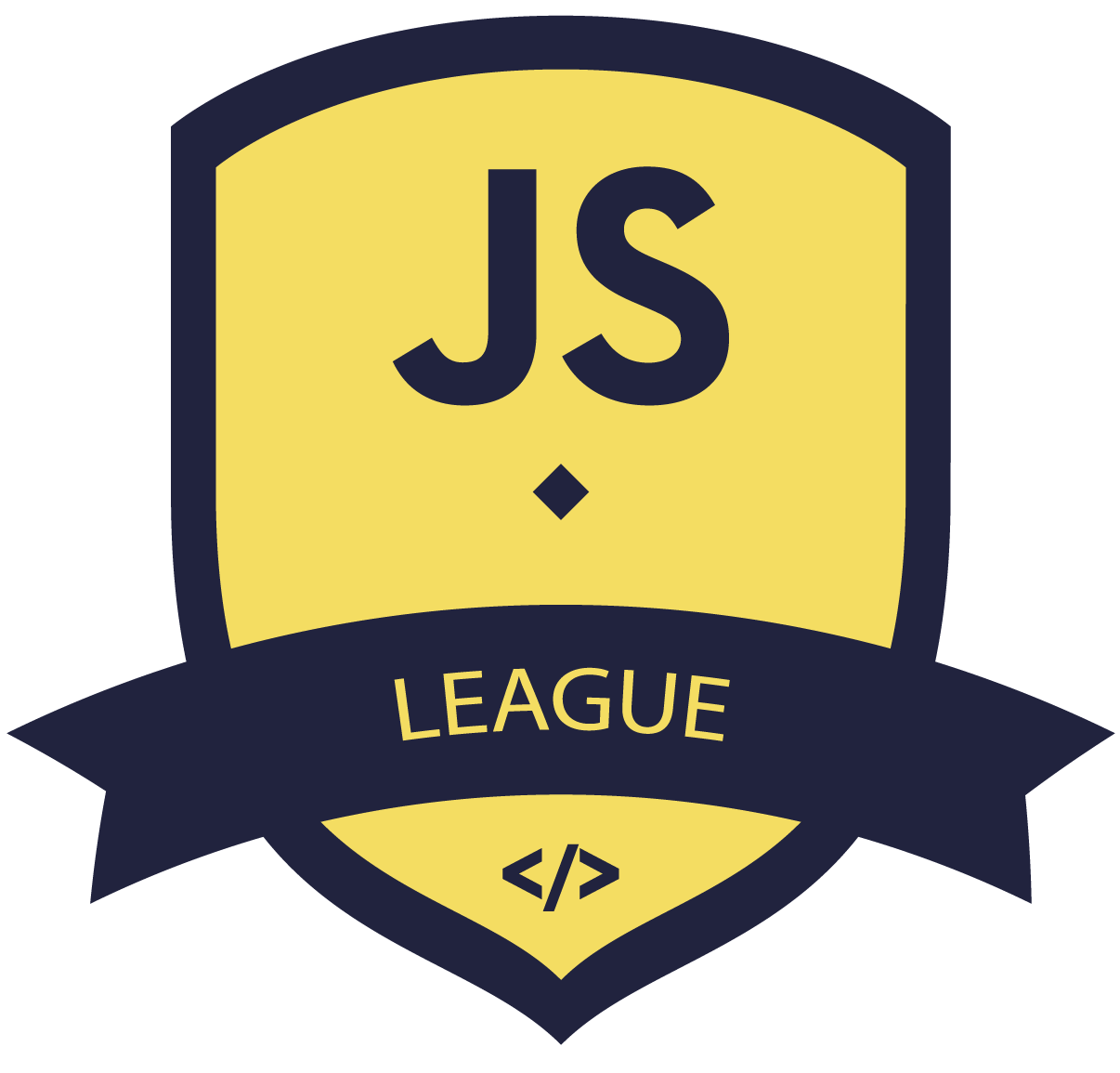
Classes
JavaScript Beyond the Basics
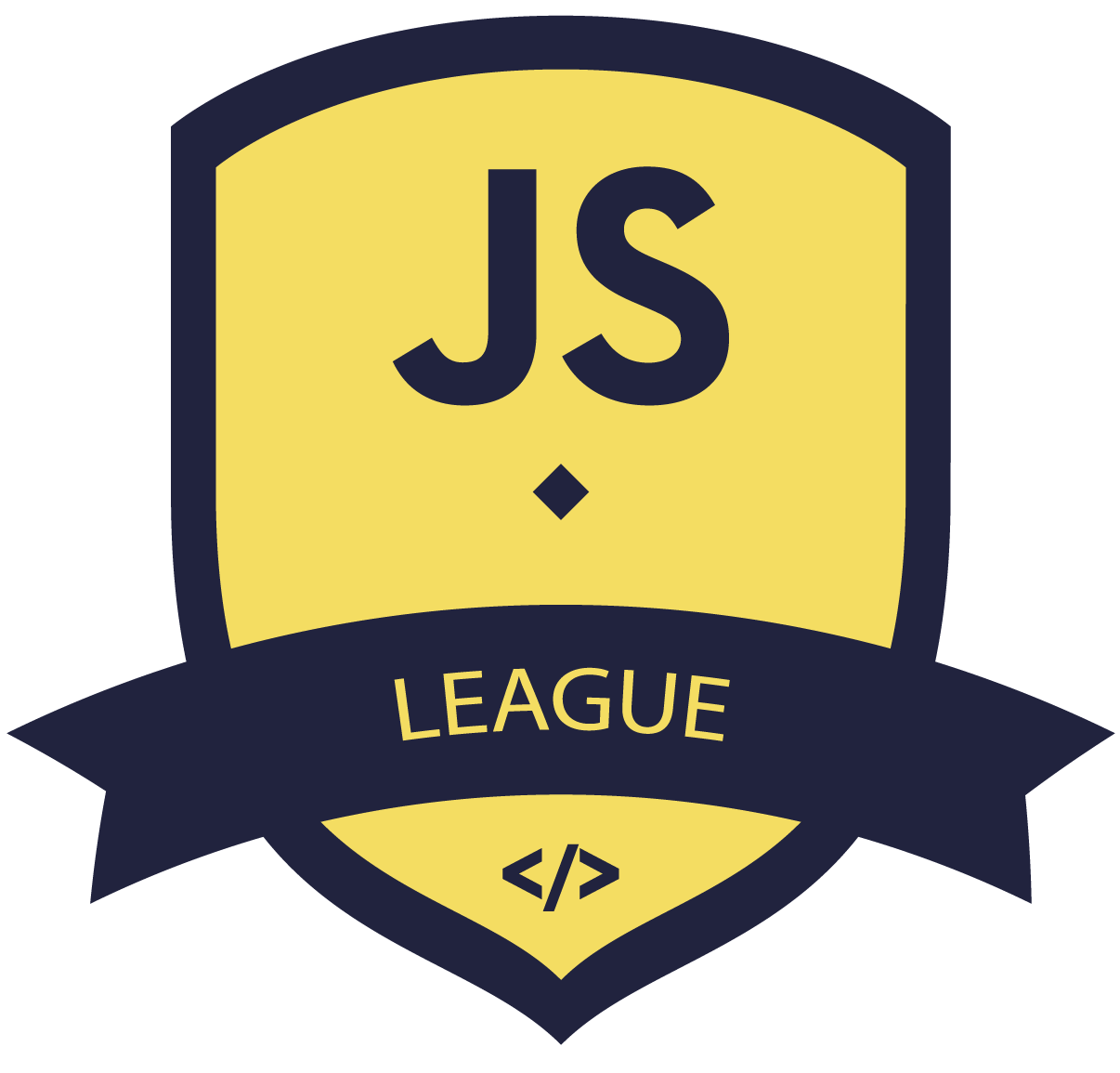
JavaScript Beyond the Basics
es5
var Person = function(fname, lname) {
this.fname = fname;
this.lname = lname;
}
Person.prototype.getFullName = function() {
return this.fname + " " + this.lname;
}
es6
class Person {
constructor(fname, lname) {
this.fname = fname;
this.lname = lname;
}
getFullName() {
return `${this.fname} ${this.lname}`
}
}
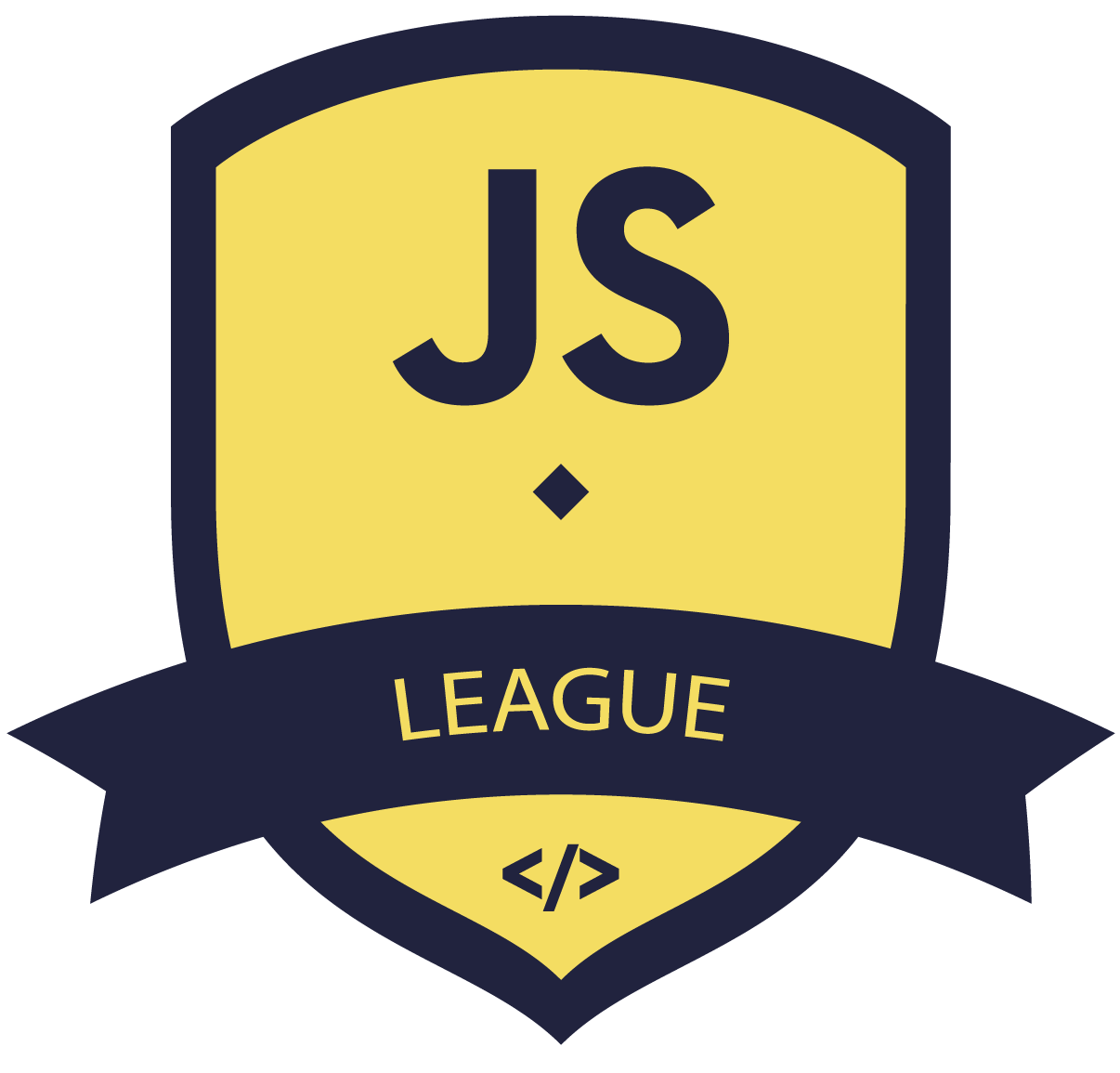
JavaScript Beyond the Basics
constructors and inheritance
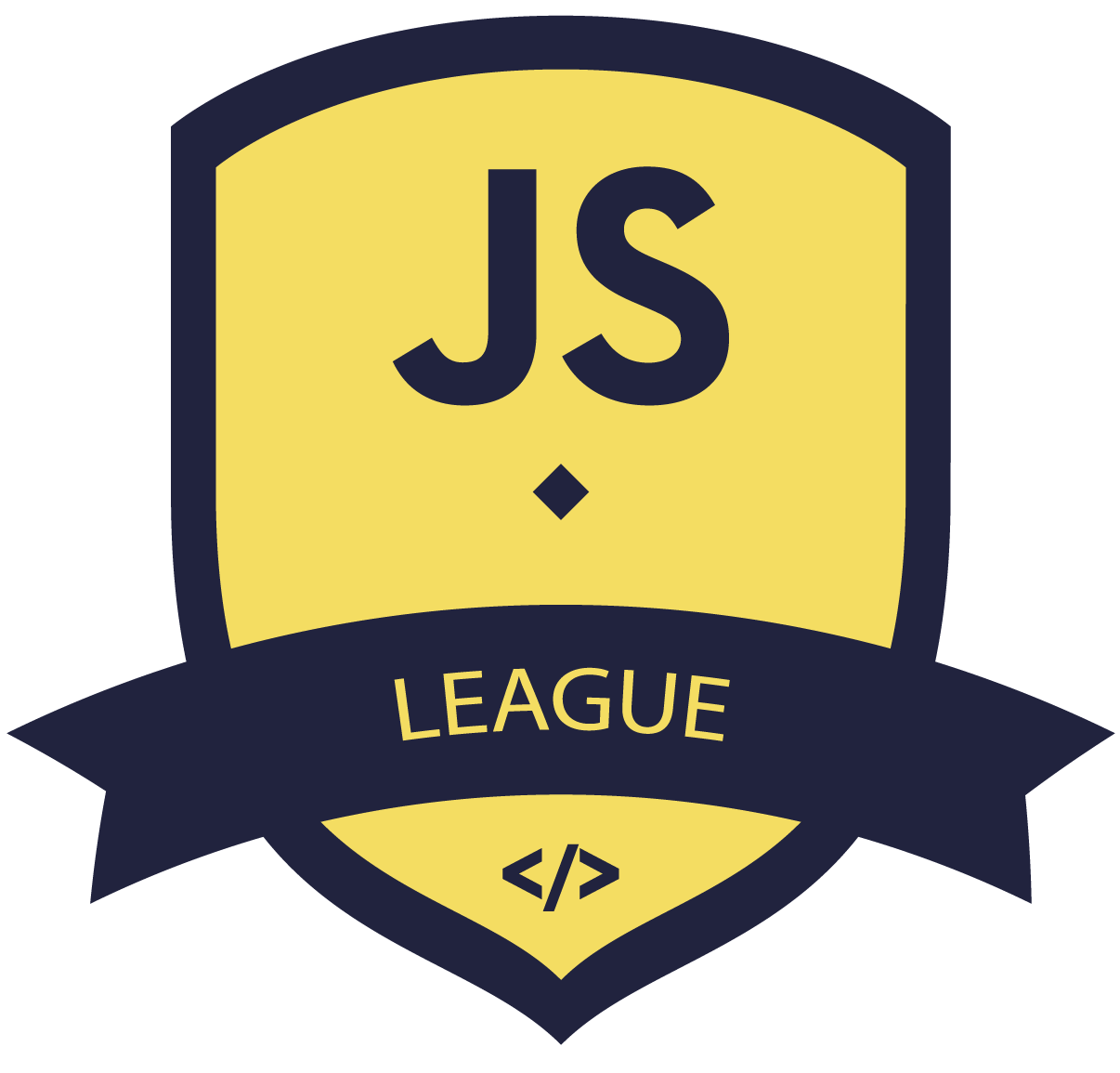
Functional programming
JavaScript Beyond the Basics
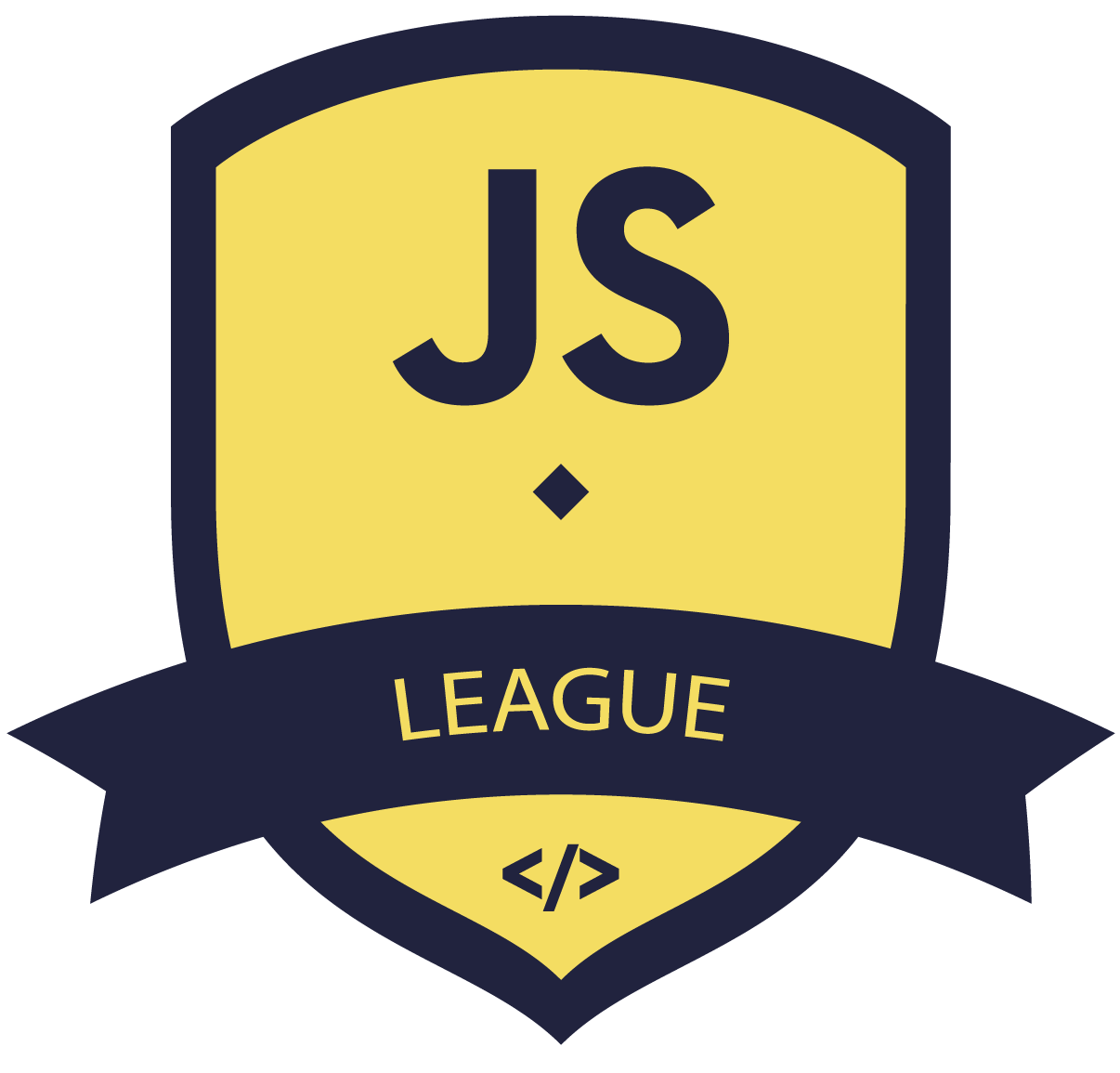
JavaScript Beyond the Basics
Two pillars of JavaScript:
Prototypal Inheritance
Functional Programming
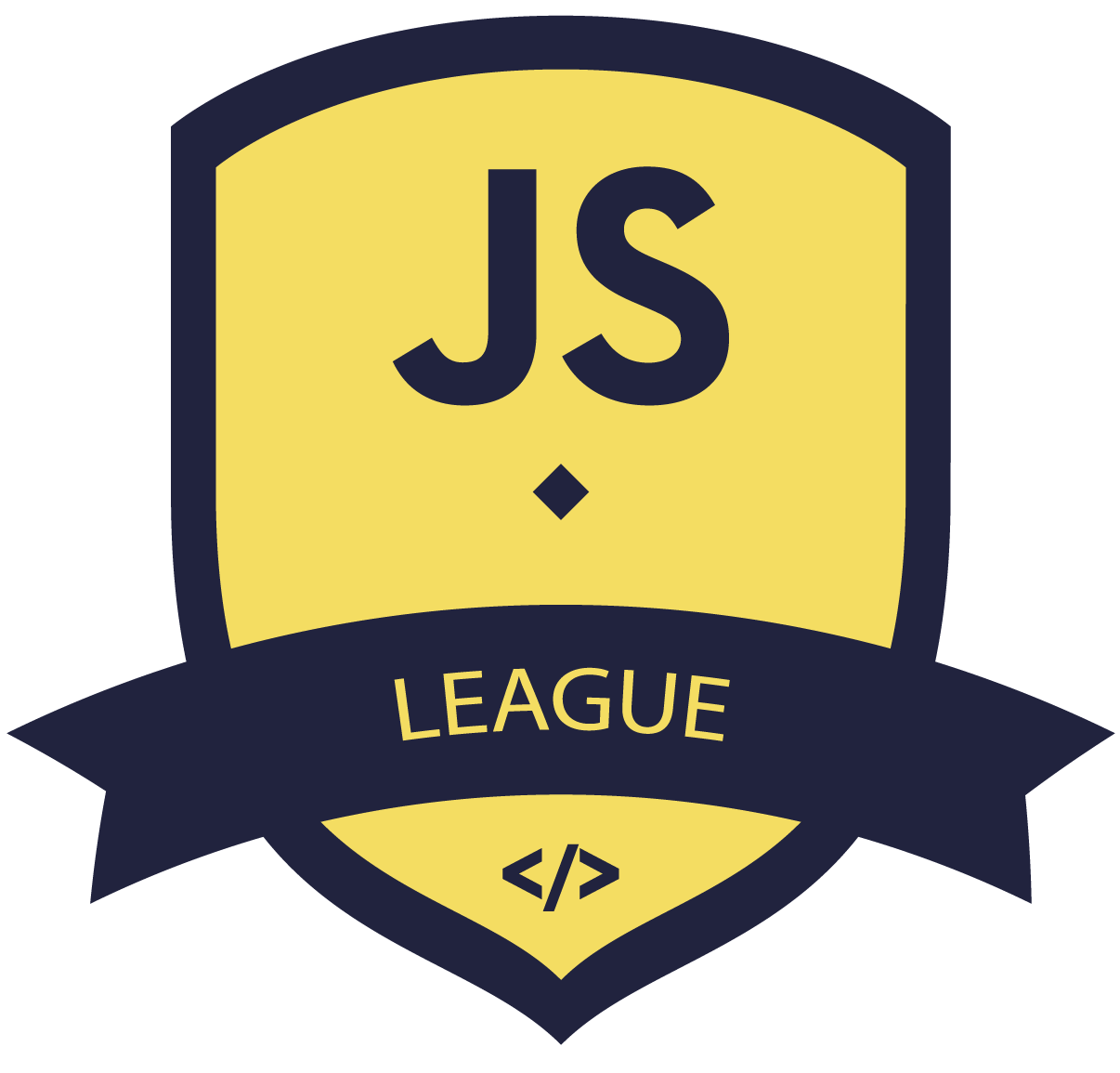
JavaScript Beyond the Basics
Functional Programming
Pure functions
Function composition
Avoid shared state
Avoid mutating state
Avoid side effects
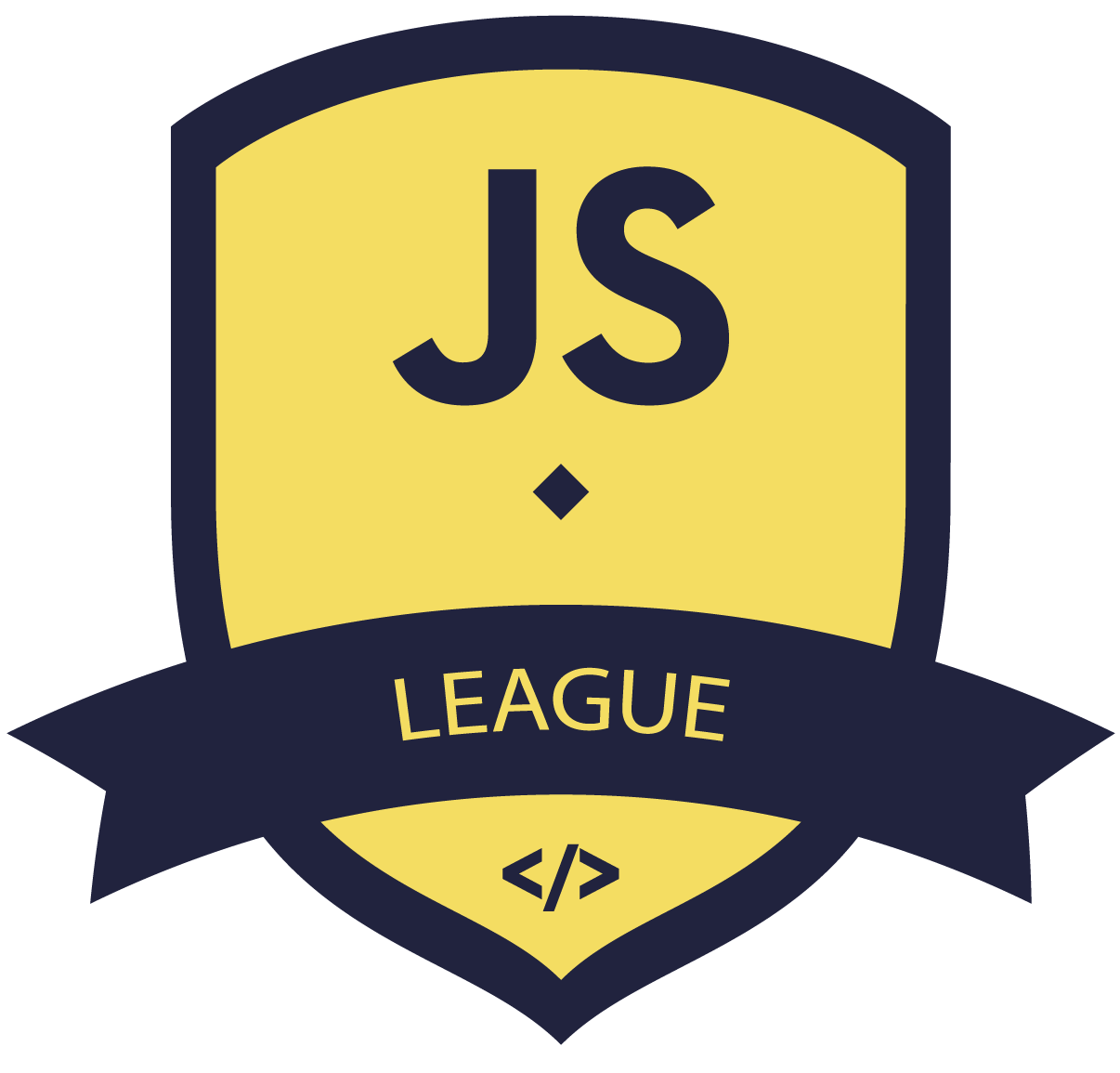
JavaScript Beyond the Basics
Pure functions
implements pattern input-output
given same input, has same output
no side-effects
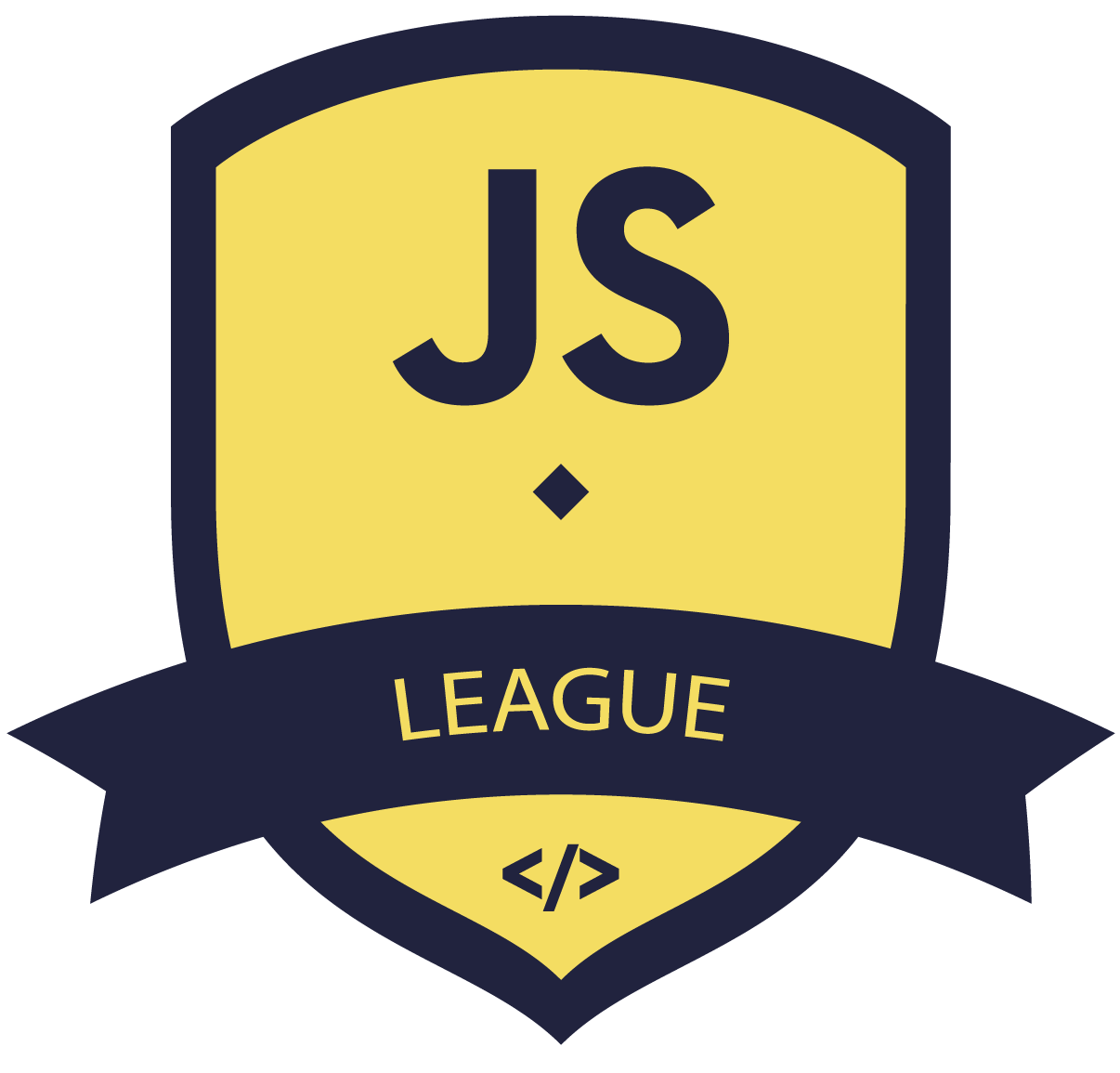
JavaScript Beyond the Basics
Function composition
combine multiple functions to result one
no shared state
high reusability
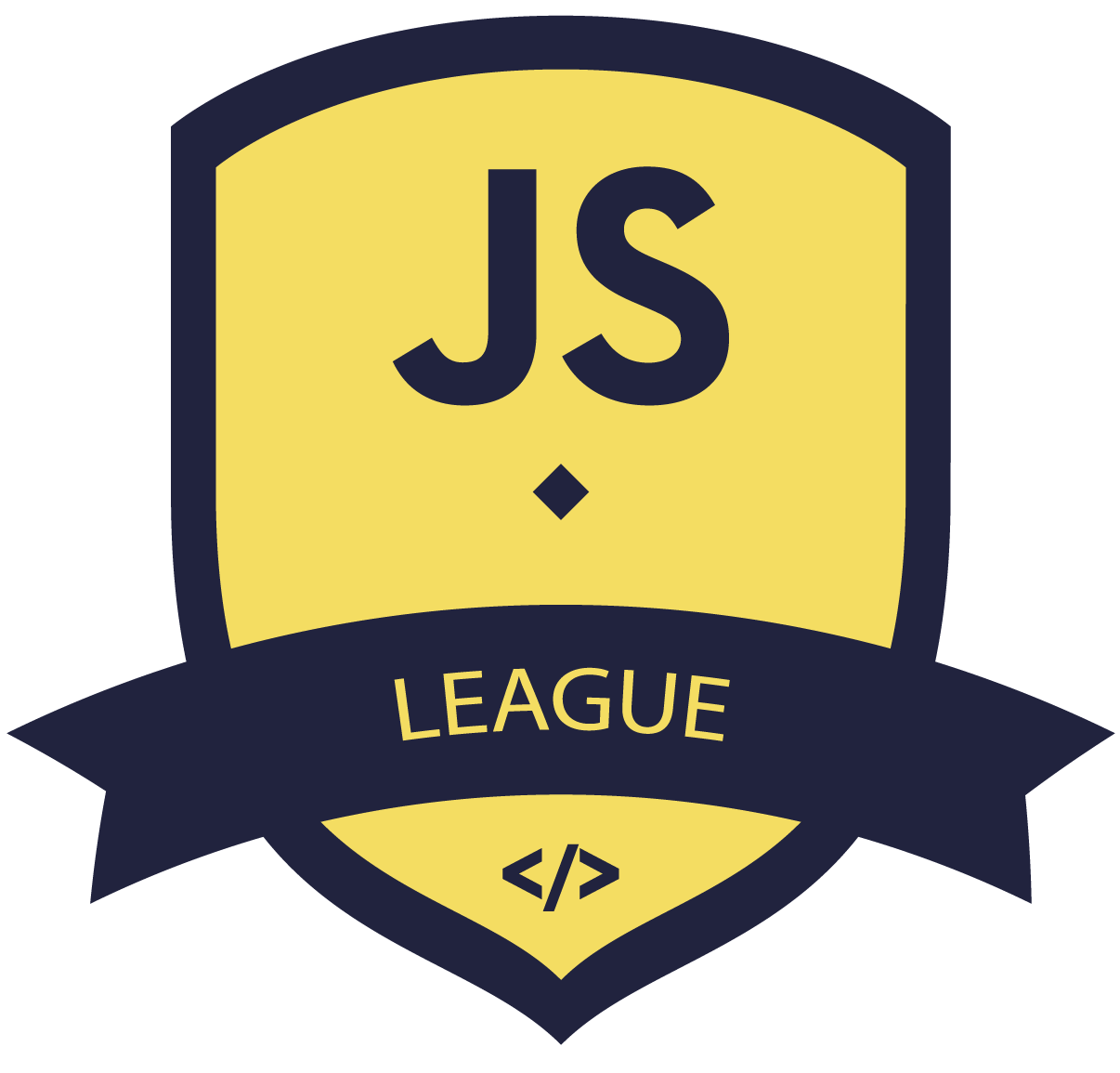
JavaScript Beyond the Basics
Avoid shared state
Avoid mutating state
Avoid side effects
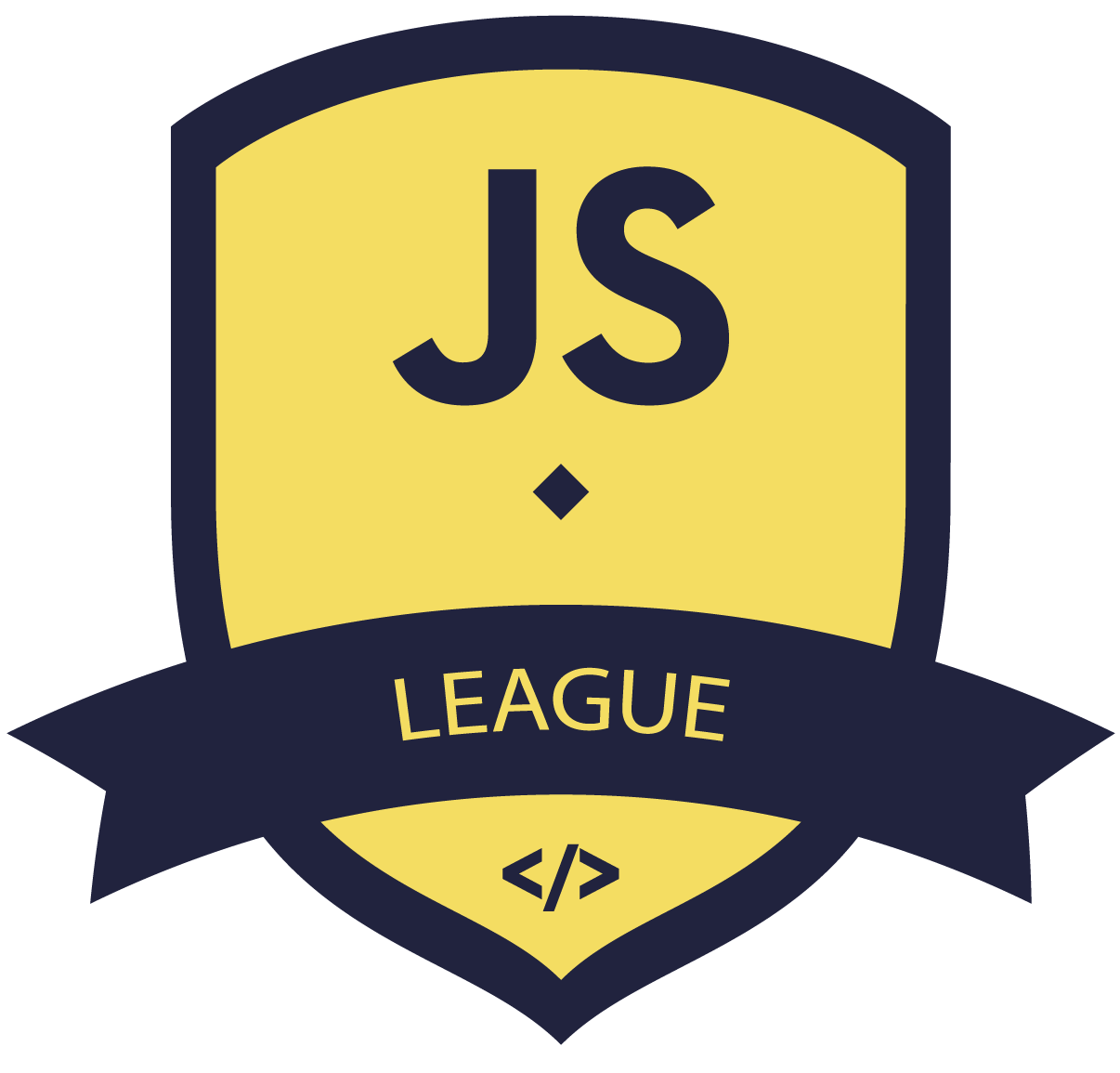
Set and Map
JavaScript Beyond the Basics
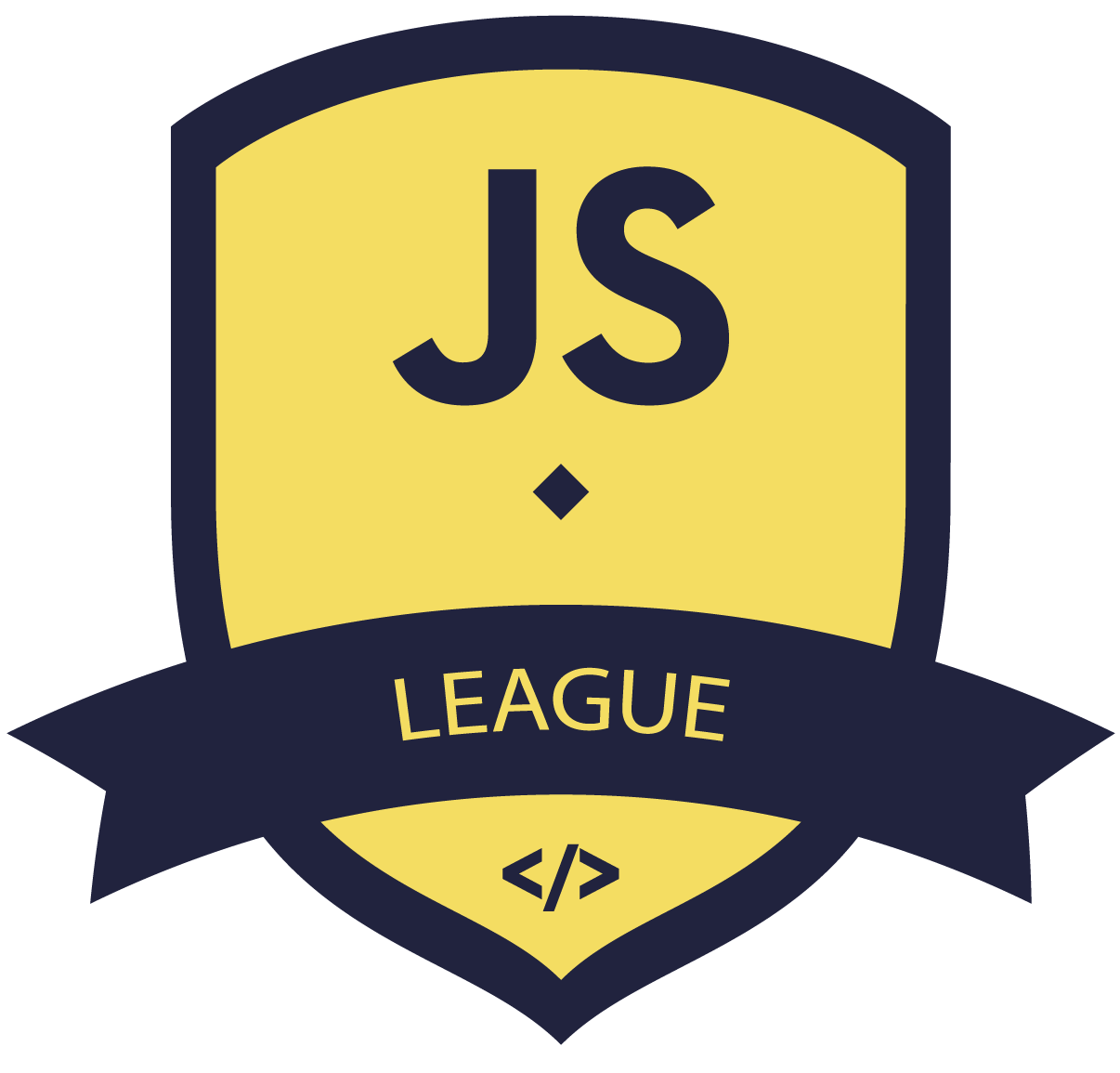
JavaScript Beyond the Basics
Map
Map is a collection of keyed data items, just like an Object. But the main difference is that Map allows keys of any type.
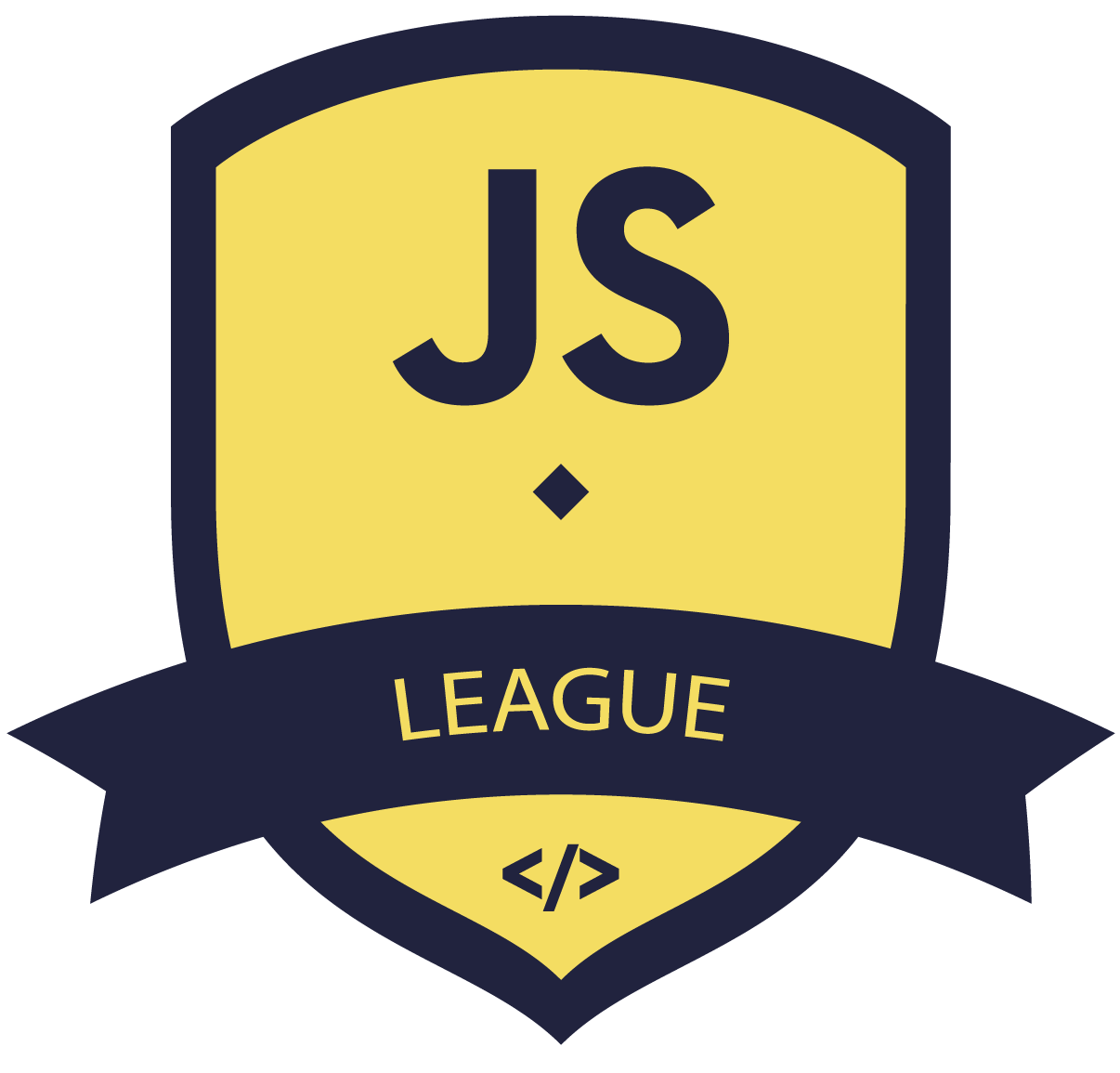
JavaScript Beyond the Basics
Set
A Set is a special type collection – “set of values” (without keys), where each value may occur only once.
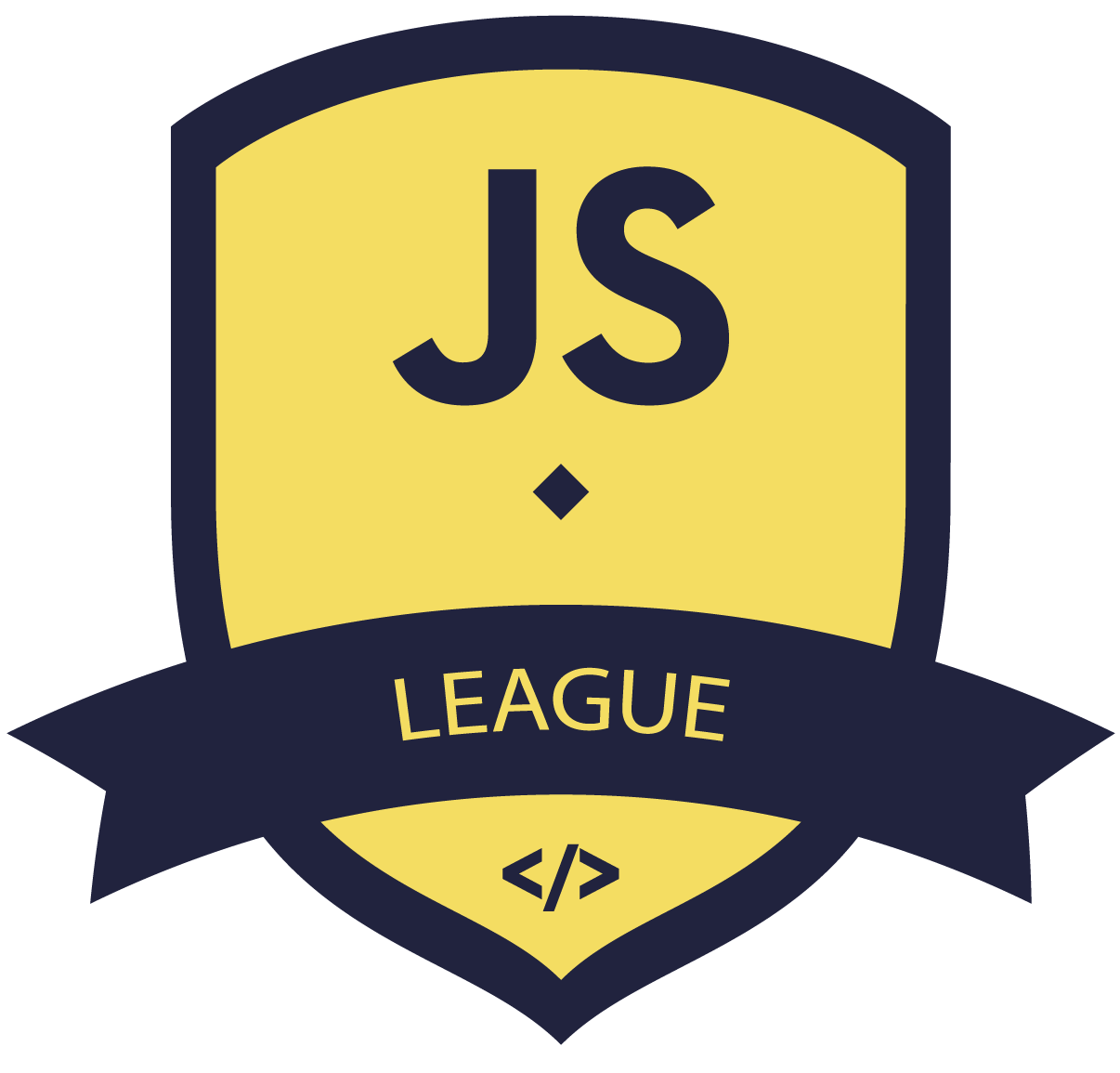
Iterators: generators, for...loops
JavaScript Beyond the Basics
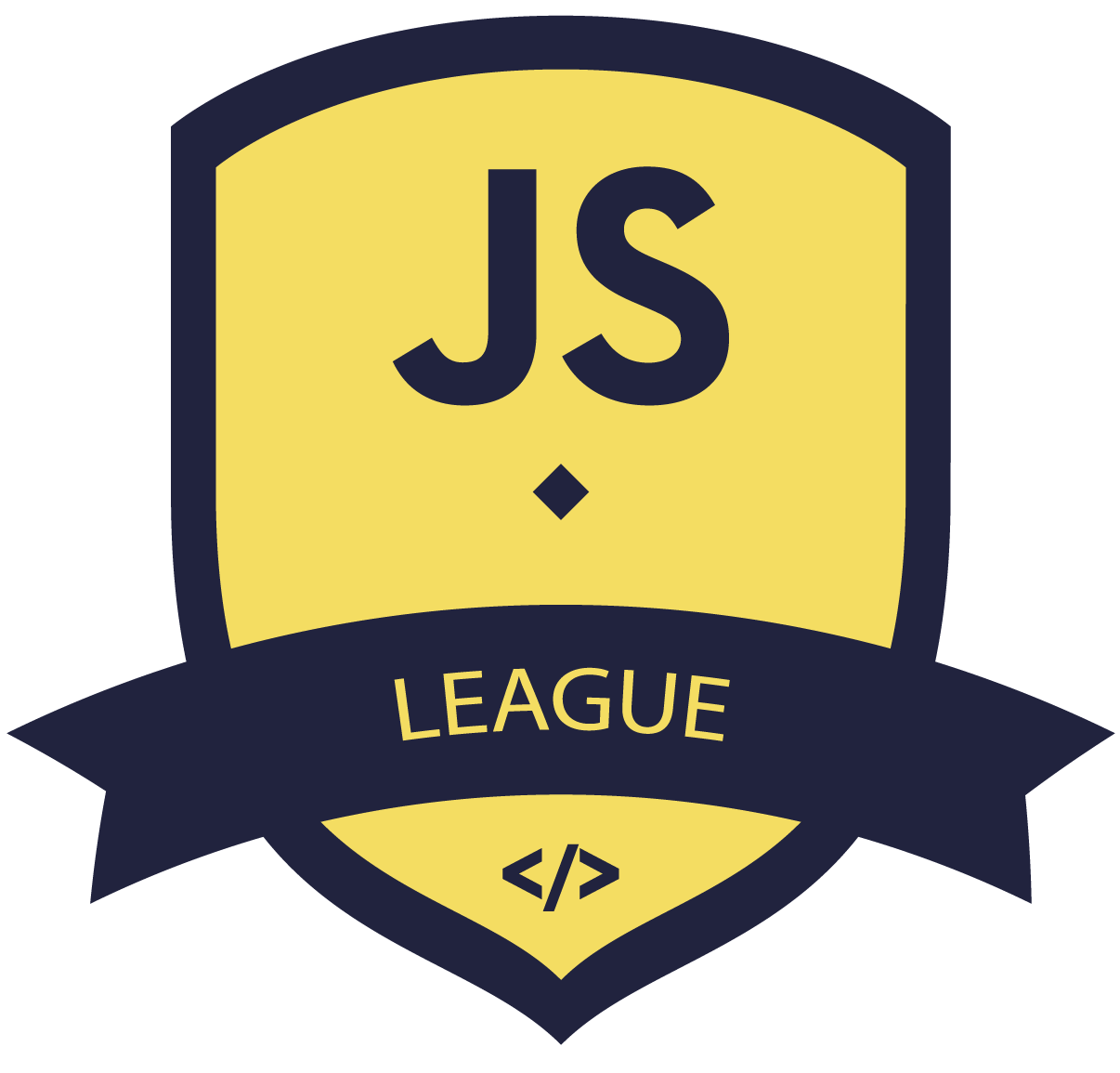
JavaScript Beyond the Basics
Generators
functions that behave as iterators
declared using function*
does not return values, yields them
can create iterators out of anything
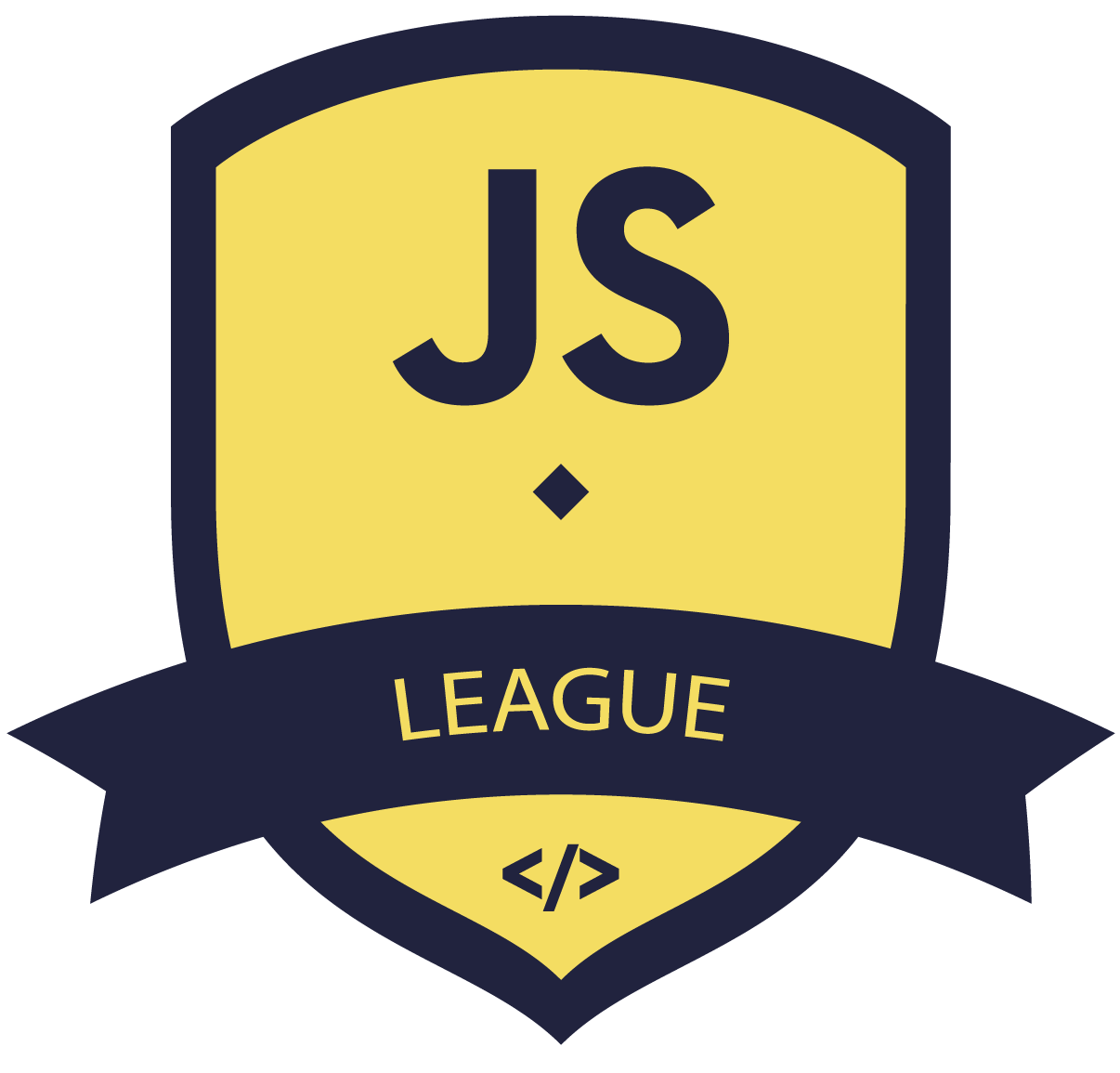
JavaScript Beyond the Basics

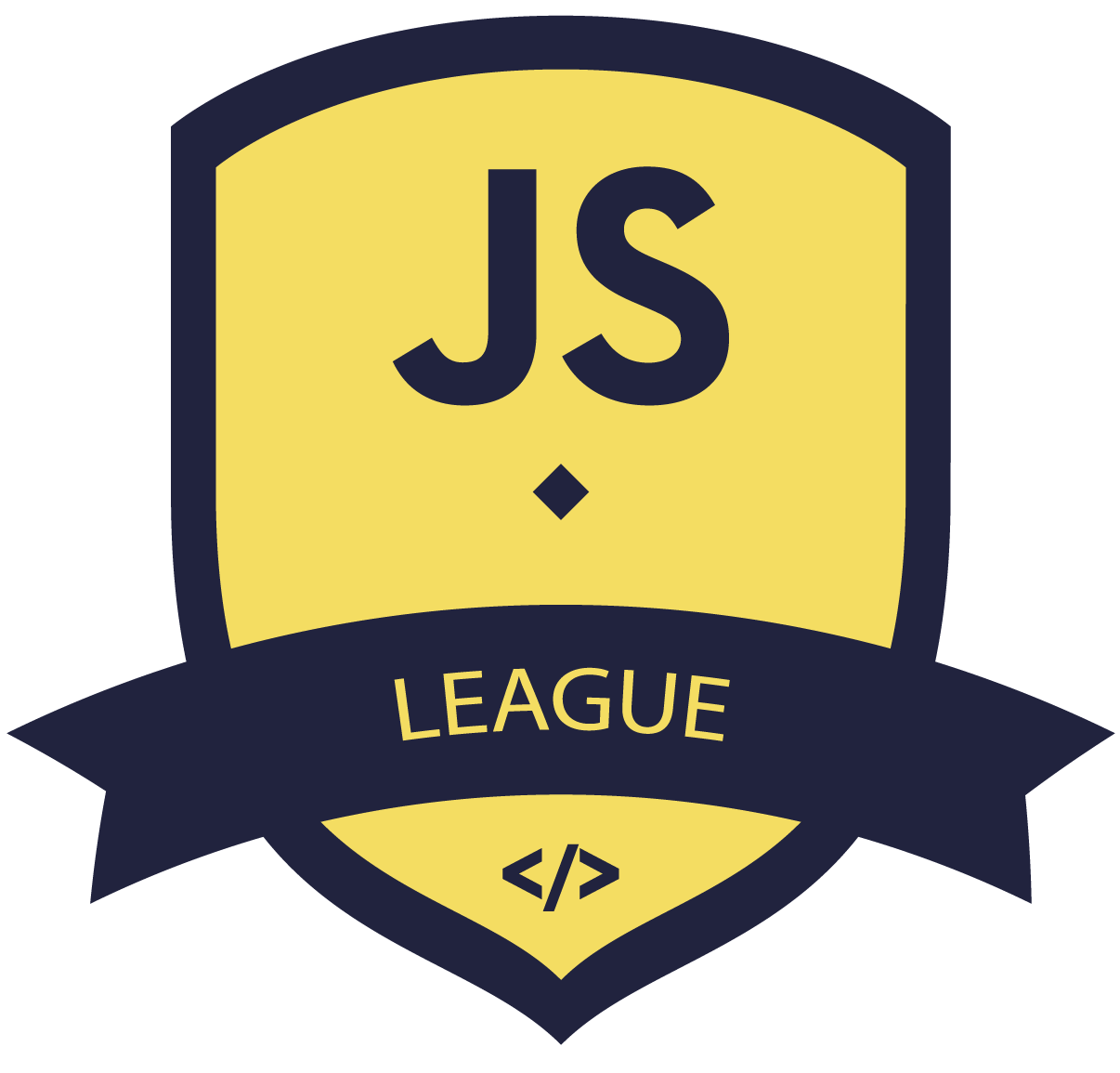
JavaScript Beyond the Basics
for...loop
iterate over values
iterate over keys
for...in
for...of
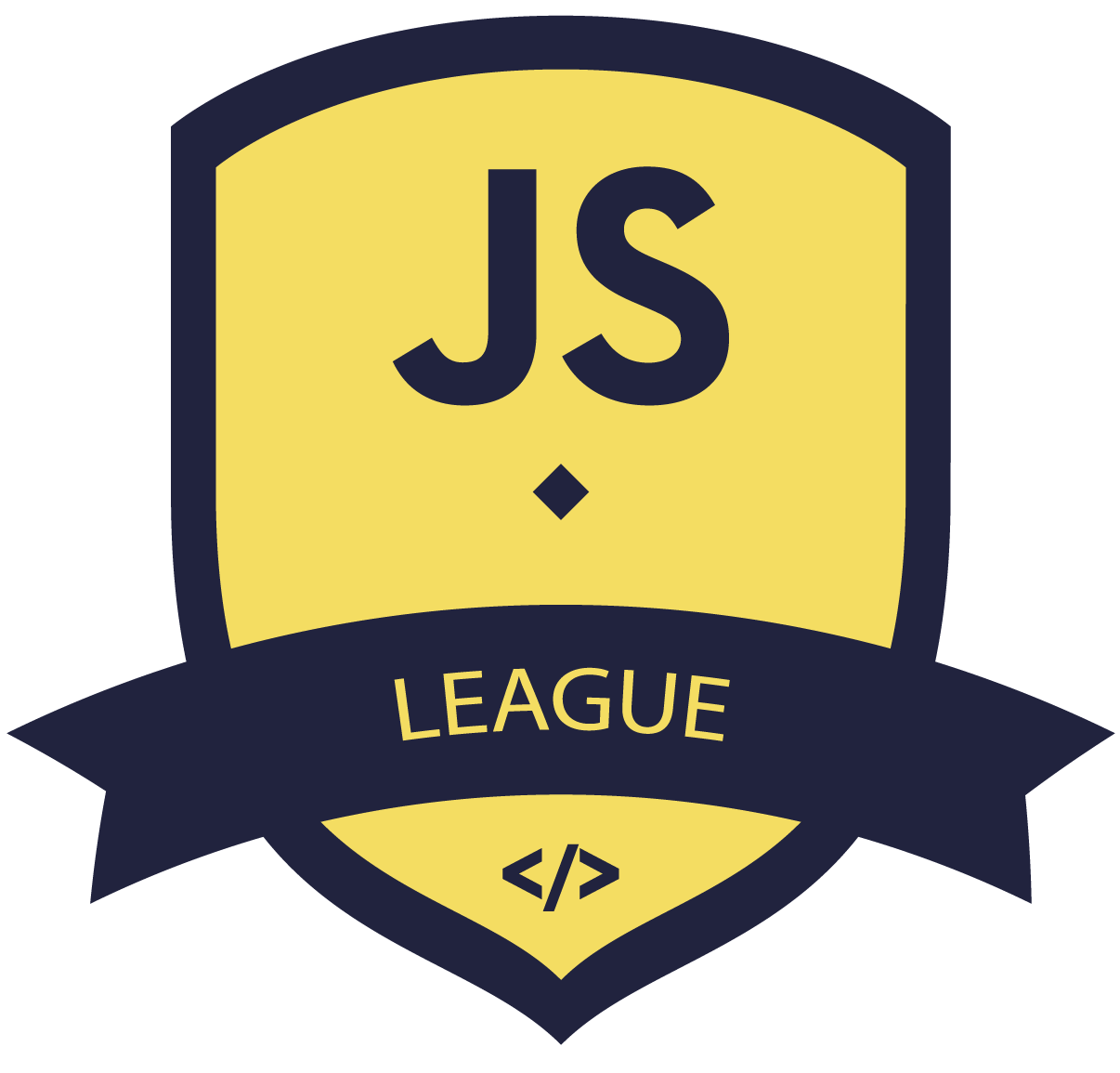
JavaScript Beyond the Basics
Hoisting
evaluating stuff that doesn't exist (yet)
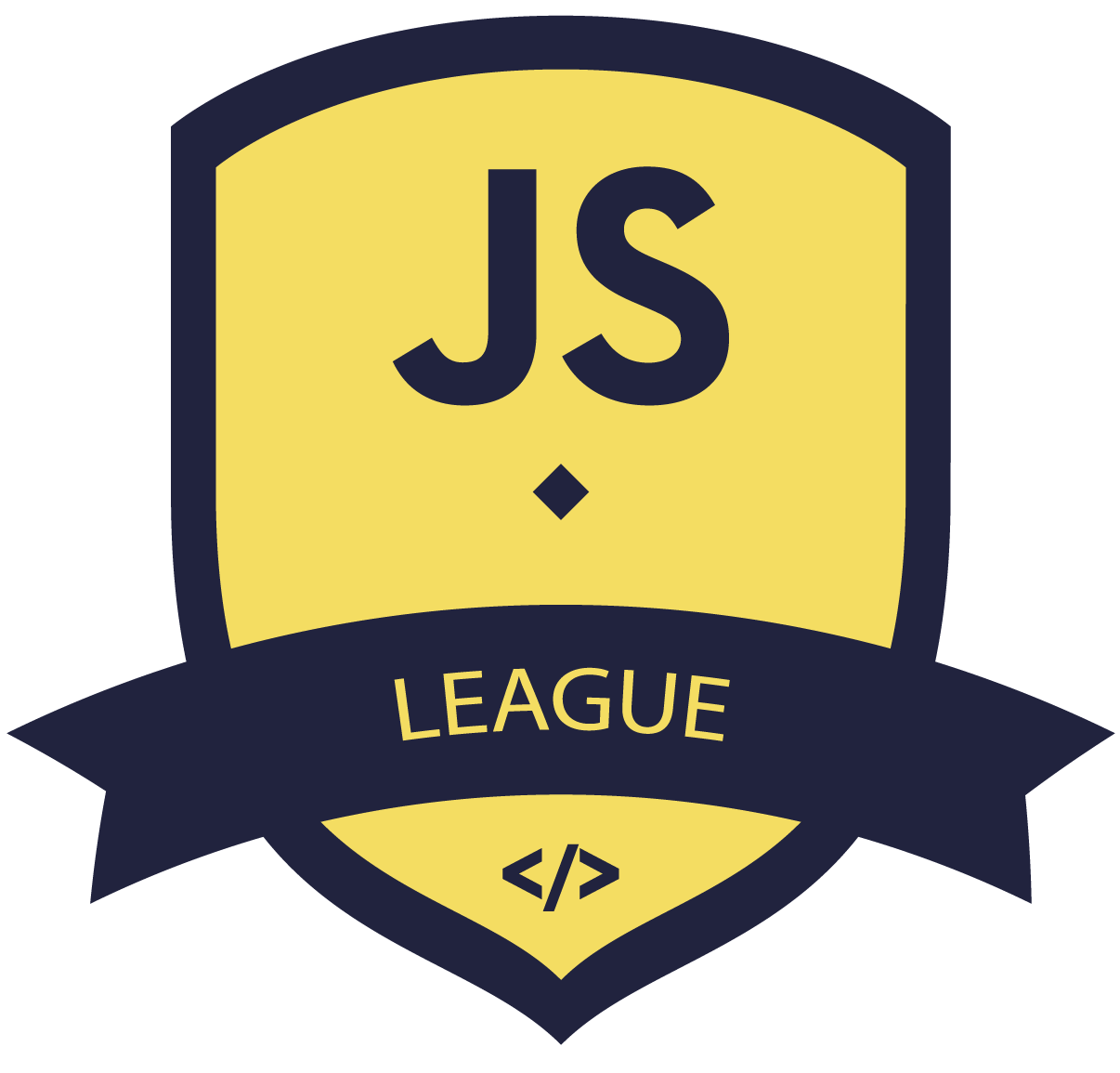
ES modules
JavaScript Beyond the Basics
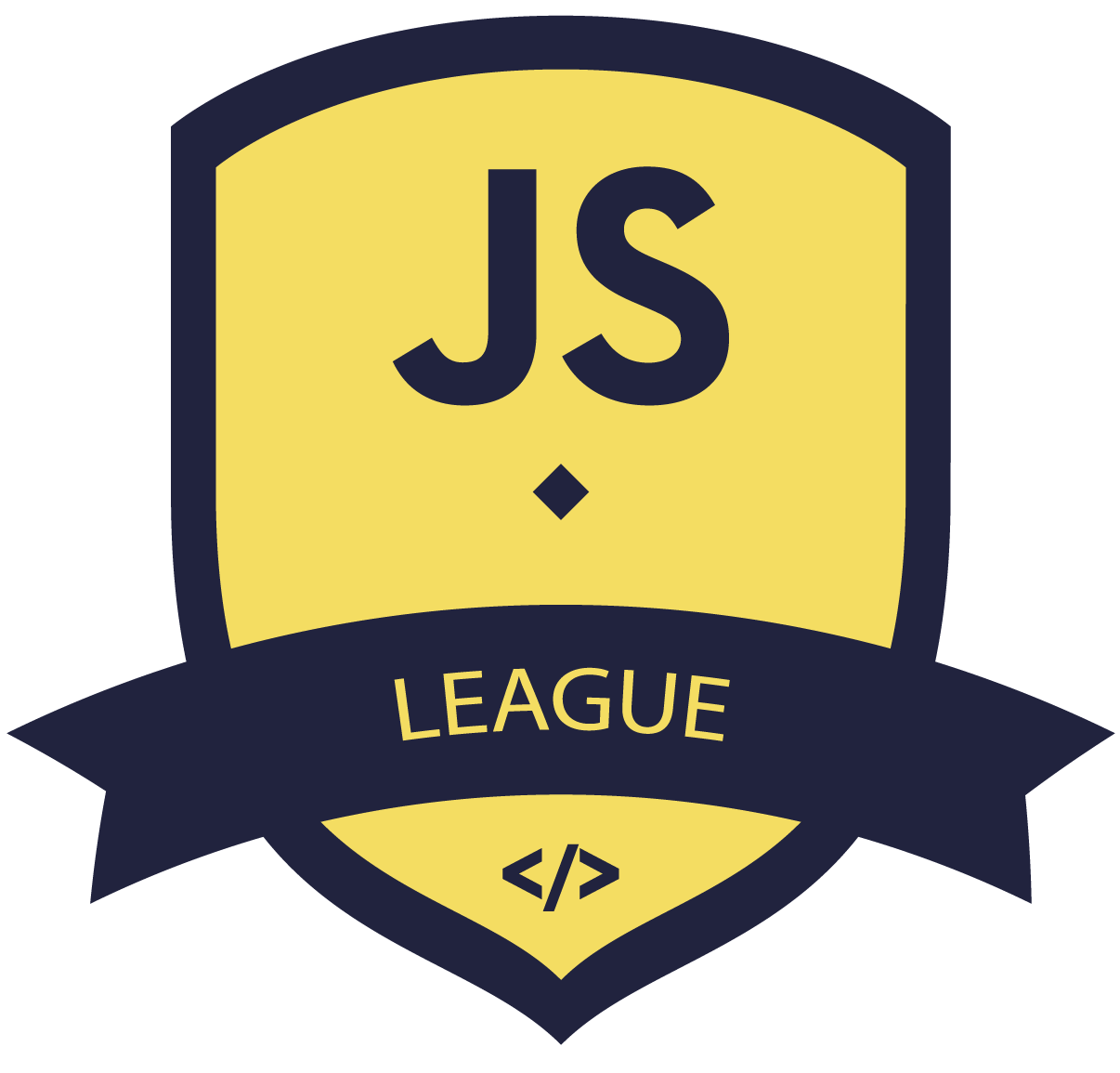
JavaScript Beyond the Basics
es5
// enums/numbers.js
var Numbers = {};
Numbers.ONE = 1;
Numbers.TWO = 2;
Numbers.THREE = 1;
Numbers.FOUR = 2;
// app.js
var EnumNumbers = {};
EnumNumbers.ONE = Numbers.ONE;
EnumNumbers.TWO = Numbers.TWO;
es6
// enums/numbers.js
export {
ONE: 1,
TWO: 2,
THREE: 3,
FOUR: 4
}
// app.js
import {
ONE,
TWO
} from "enums/numbers.js"
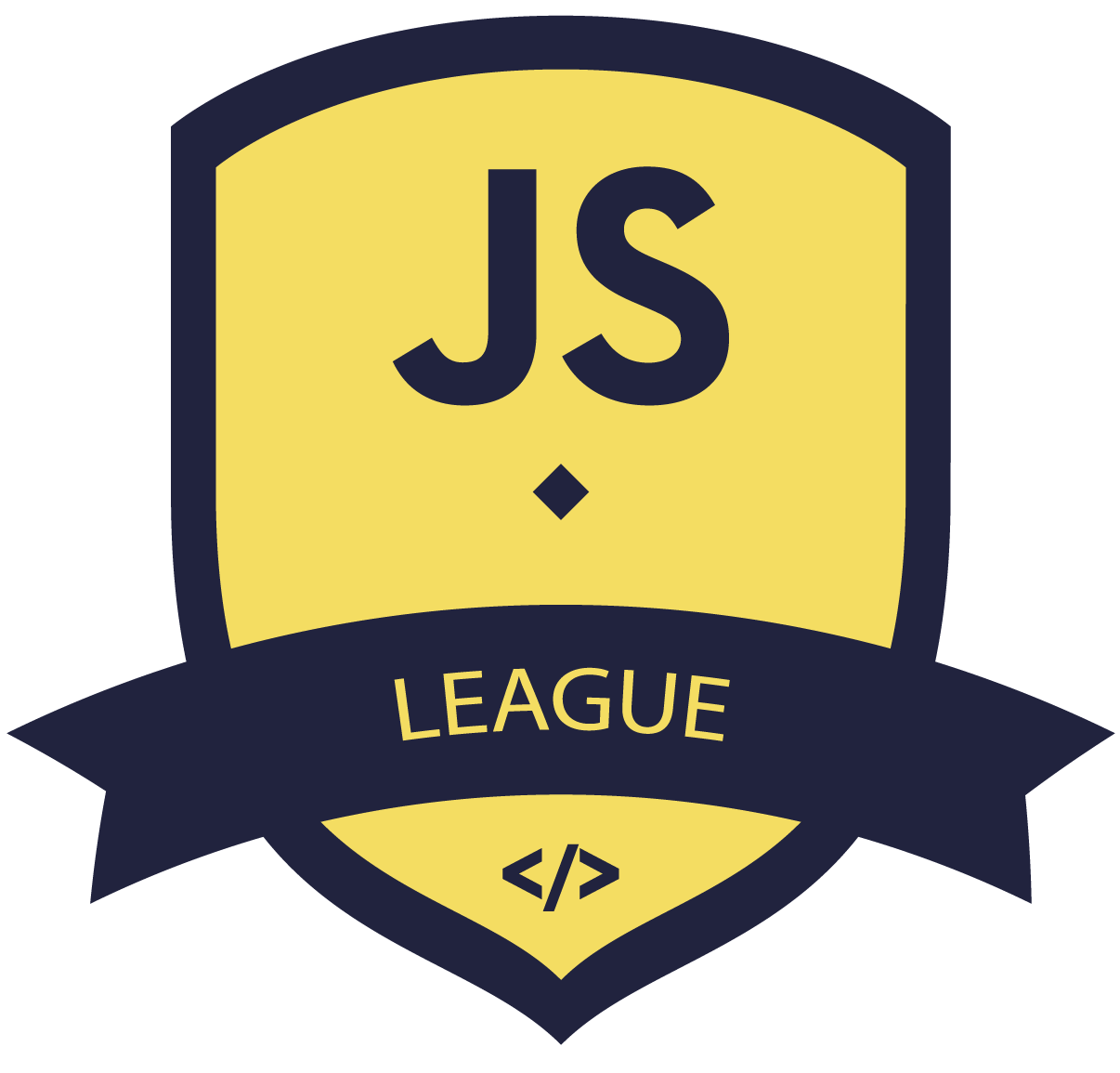
Observables
JavaScript Beyond the Basics
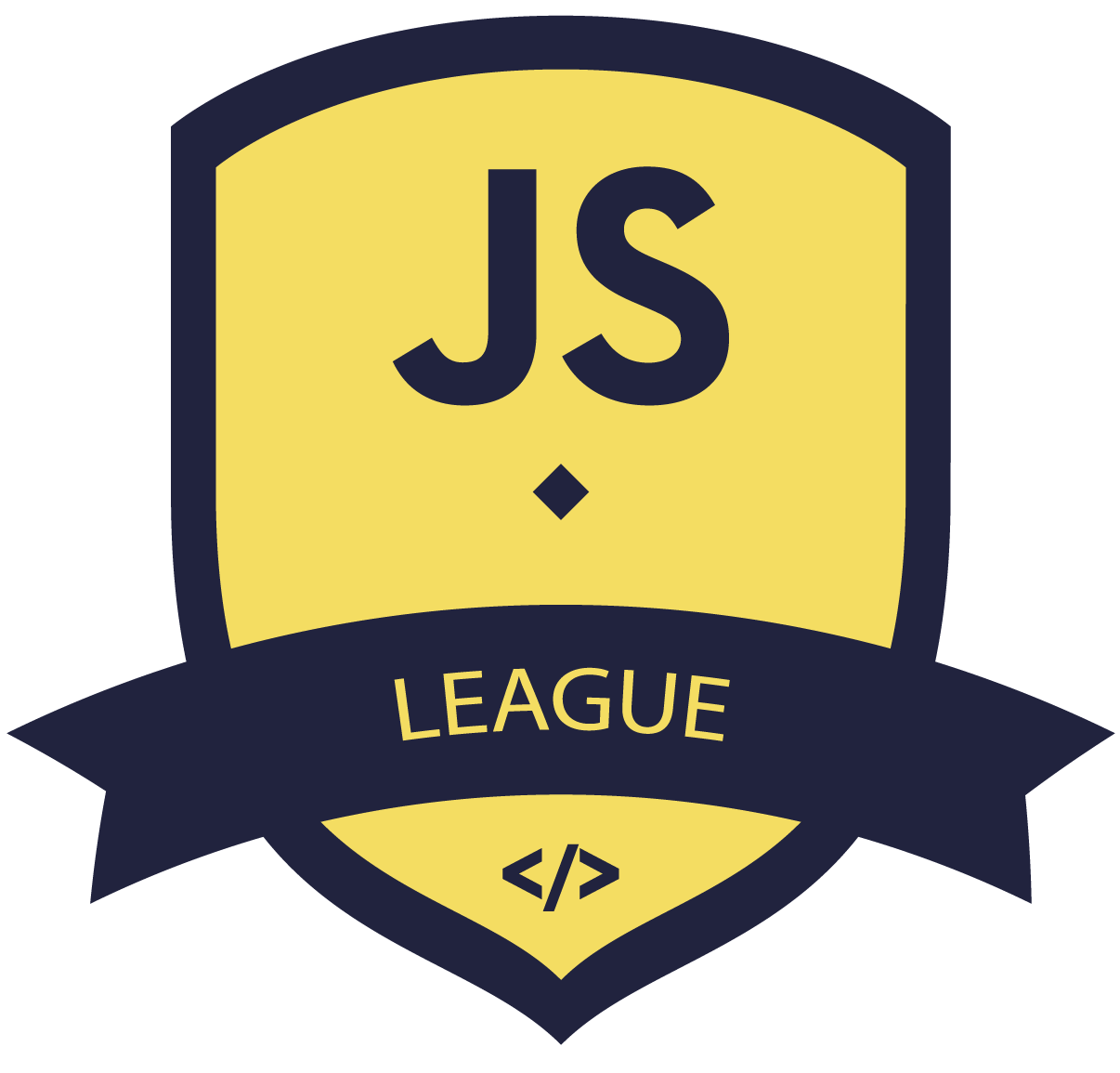
JavaScript Beyond the Basics
Observables
asynchronous turned into streams
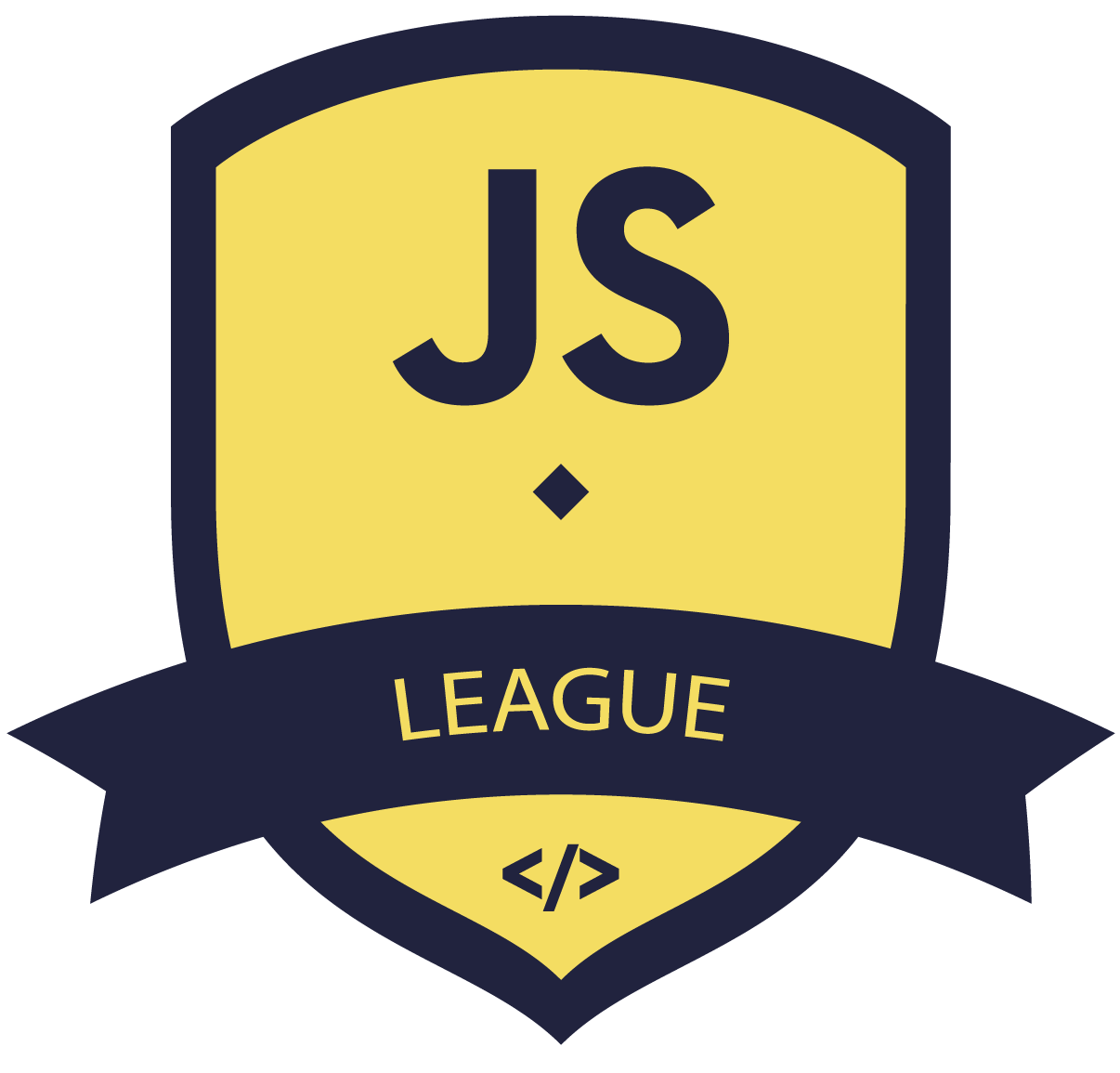
Error handling & debugging
JavaScript Beyond the Basics
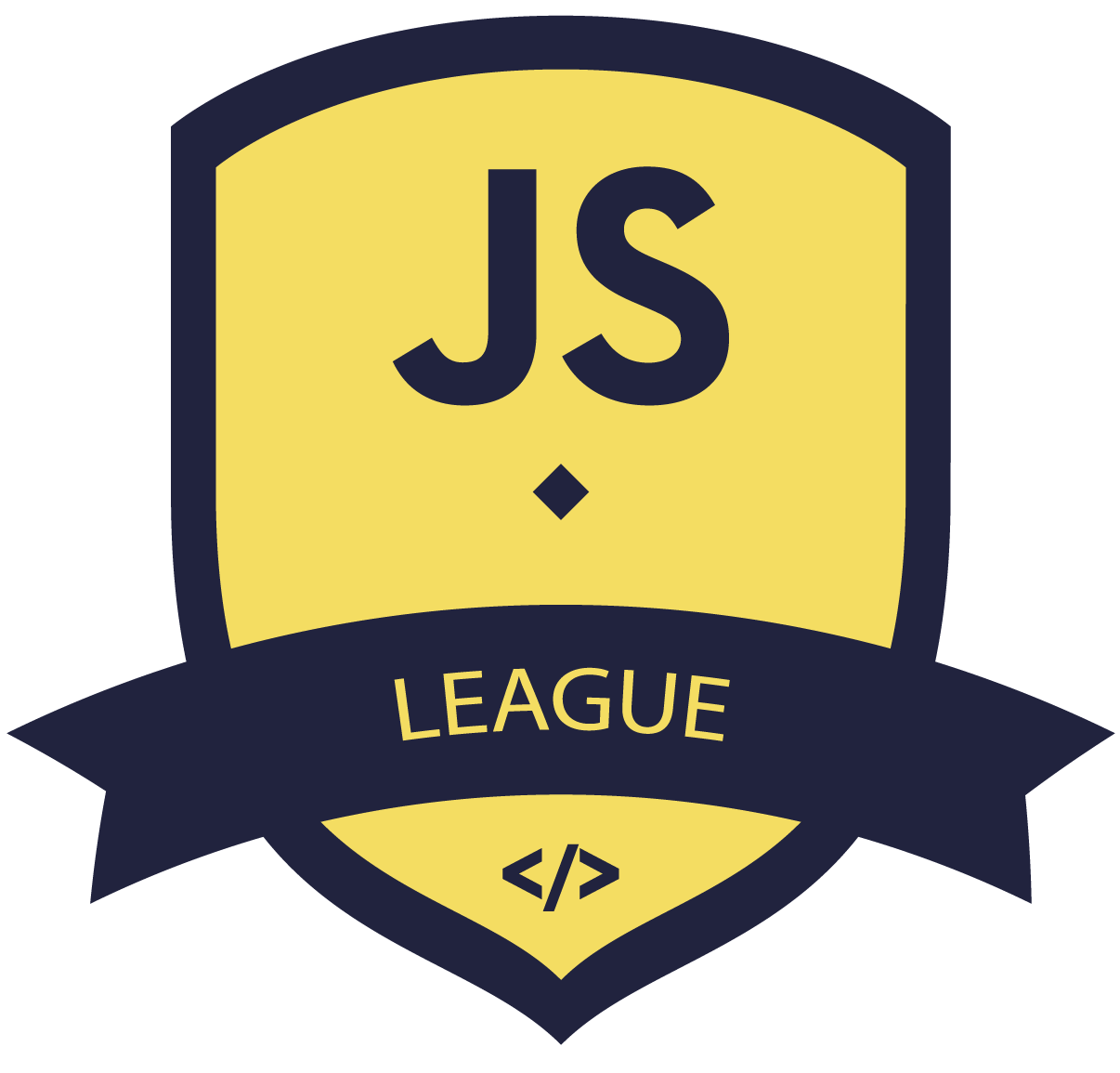
JavaScript Beyond the Basics
Errors
always use throw new Error as it provides stacktrace
throwing errors in async returns the actual error
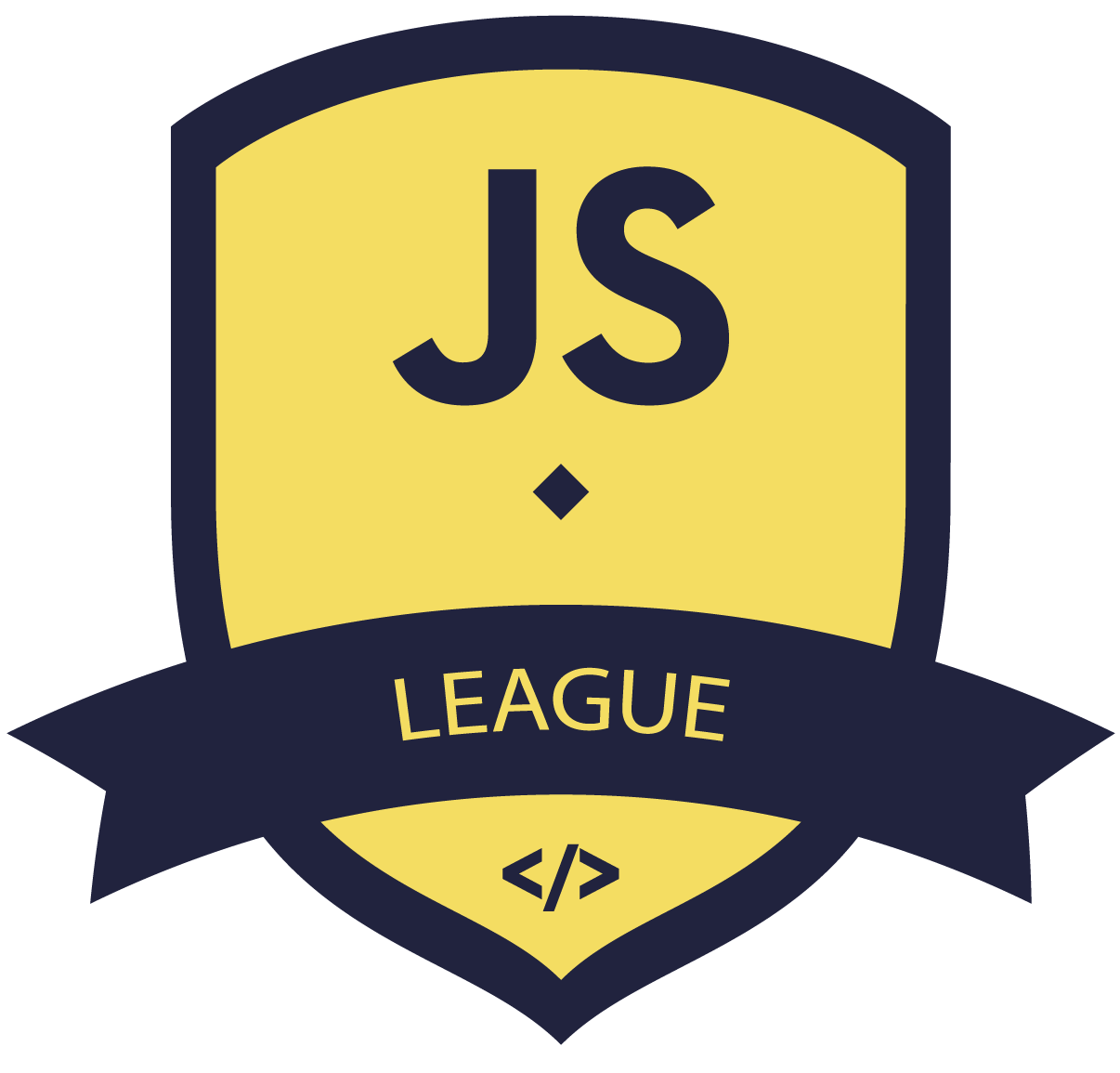
JavaScript Beyond the Basics
class ValidationError extends Error {
constructor(message) {
super(message)
this.name = 'ValidationError'
this.message = message
}
}
class PermissionError extends Error {
constructor(message) {
super(message)
this.name = 'PermissionError'
this.message = message
}
}
class DatabaseError extends Error {
constructor(message) {
super(message)
this.name = 'DatabaseError'
this.message = message
}
}
module.exports = {
ValidationError,
PermissionError,
DatabaseError
}
const {
ValidationError
} = require('./error')
function myFunction(input) {
if (!input)
throw new ValidationError(
'A validation error'
)
return input
}
new class based
error approach
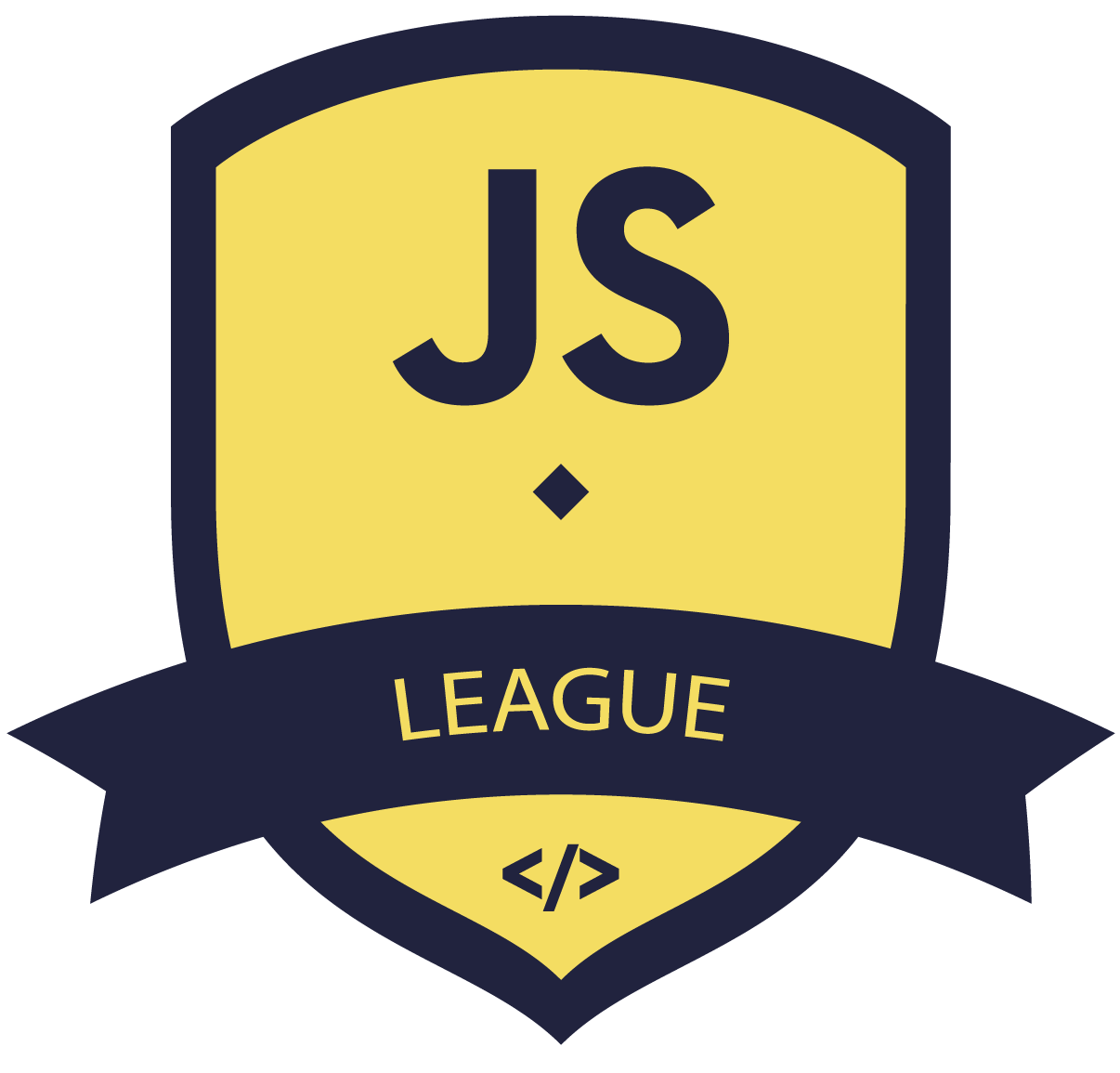
Q&A
JavaScript Beyond the Basics
Thank you!
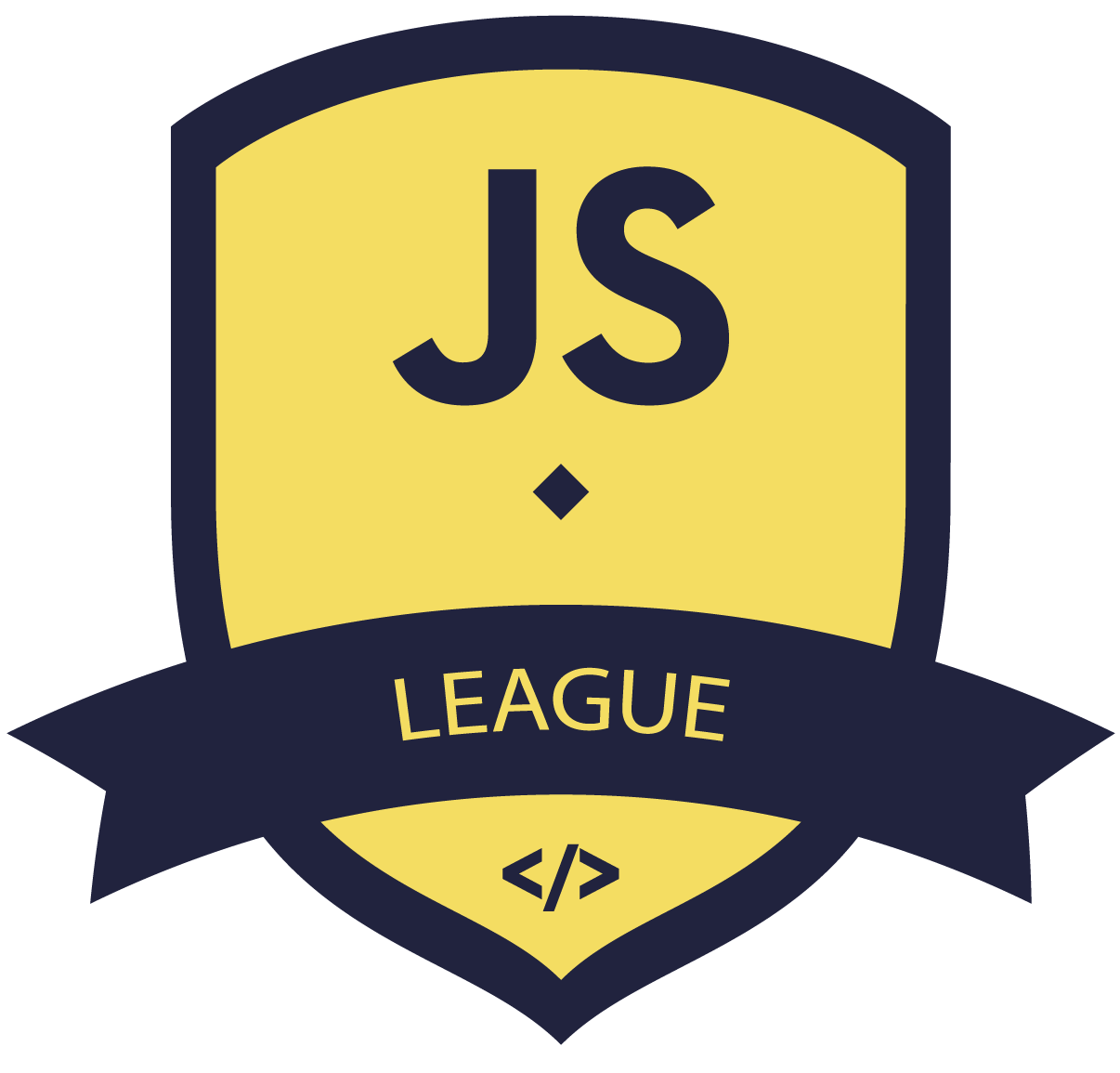
JavaScript Beyond the Basics
By Sabin Marcu
JavaScript Beyond the Basics
- 218