Intro to "modern" C++
A pretty old language
C++ standards
- C++98
- C++11
- C++14
- C++17
Tiobe Index (2017)
- Java 14.6%
- C 7%
- C++ 4.7%
- Python 3.5%
- C# 3.4%
- Visual Basic 3.39%
- JavaScript 3%
Vector iteration - C++ 98
#include <iostream>
#include <string>
#include <vector>
int main(int argc, char** argv)
{
std::vector<std::string> list;
list.push_back("hello");
list.push_back("world");
for(std::vector<std::string>::iterator it = list.begin(); it != list.end(); it ++)
{
std::string s = *it;
std::cout << s << std::endl;
}
return 0;
}
Vector iteration - Modern C++
#include <iostream>
#include <string>
#include <vector>
int main(int argc, char** argv)
{
std::vector<std::string> list = { "hello", "world" };
for(auto s : list)
std::cout << s << std::endl;
return 0;
}
Vector iteration - Modern C++
#include <iostream>
#include <string>
#include <vector>
int main(int argc, char** argv)
{
// Initializer lists
std::vector<std::string> list = { "hello", "world" };
// Auto types <3
// Range based for loops
for(auto s : list)
std::cout << s << std::endl;
return 0;
}
Lambda / async - C++ 98
#include <iostream>
#include <string>
#include <vector>
#include <pthread.h>
class Test
{
public:
Test(const std::string& name) : m_name(name) {}
std::string getName() { return m_name; }
private:
std::string m_name;
};
void* async_do(void* cls)
{
Test *t = static_cast<Test*>(cls);
std::cout << t->getName() << std::endl;
}
int main(int argc, char** argv)
{
Test t("Hello world");
pthread_t thread;
pthread_create(&thread, NULL, &async_do, &t);
pthread_join(thread, NULL);
return 0;
}
Lambda / async - Modern C++
#include <iostream>
#include <string>
#include <vector>
#include <thread>
class Test
{
public:
Test(const std::string& name) : m_name(name) {}
std::string getName() { return m_name; }
private:
std::string m_name;
};
int main(int argc, char** argv)
{
Test t("Hello world");
std::thread([=]()
{
std::cout << t.getName() << std::endl;
}).join();
return 0;
}
Grab bag - Modern C++
// auto init lists
auto intlist = { 1, 2, 3 };
// nested namespaces
namespace A::B::C { int i; }
// structured bindings
auto tuple = std::make_tuple(1, "foo", 3.1);
auto [ i, s, f ] = tuple;
std::cout << i << s << f << std::endl;
// UTF-8 Literals
char x = u8'x';
Future? C++20(???)
- Modules
- Concepts
- Co-routines
- Transaction memory
Where to get it?
- Clang
- C++11 since 3.3
- C++14 since 3.4
- C++17 since 4.0
- GCC
- C++11 since 4.8
- C++14 since 6.1
- C++17 since 7 (almost)
- MSVC
- C++11/14 since 2015
- C++17 2017 adds stuff
Good luck!
http://github.com/tru/modern-cxx
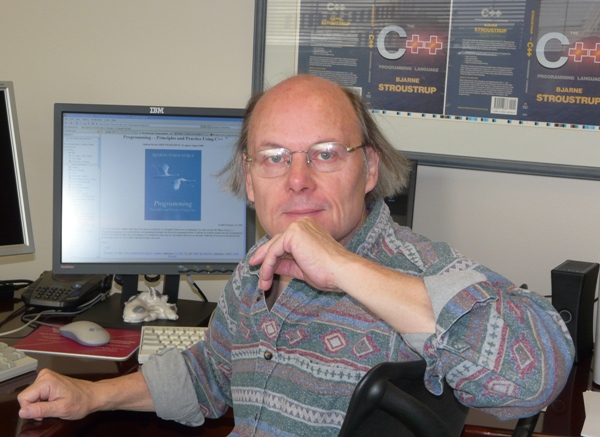
Intro to "modern" C++
By Tobias Hieta
Intro to "modern" C++
- 2,042