Intro to GraphQL
Jared Anderson
Stack Team @ LDS Church, ICS
October 10, 2017
What Isn't GraphQL?
GraphQL:
- not a graph database
- not even a database
- not a framework
- not framework specific
- not a programming language
- not programming language specific
- not a network protocol
- not a server
- not a library
- not just for web
So, What Is It?
GraphQL:
- an open source specification
- a query language for your API
- similar purpose of OData (but different)
- REST or RPC companion ➡ replacement
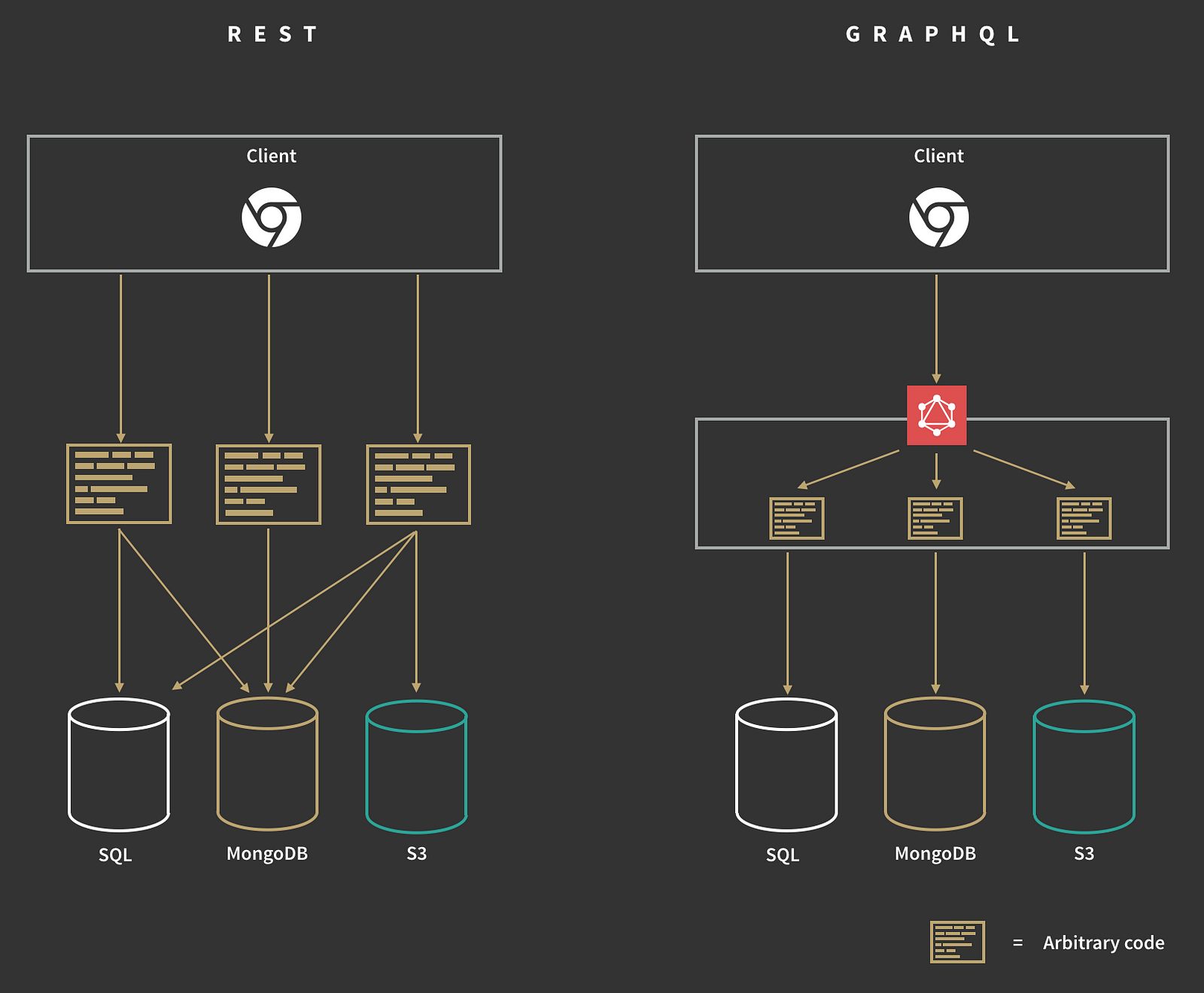
GraphQL, TMI:
- created by Facebook
- developed internally in 2012
- publicly release in 2015
- for web apps, mobile apps, desktop apps, all network connected apps.
- used by Facebook, Instagram, GitHub, Intuit, Twitter, Neo4J, New York Times, Yelp, and many more
What is a Graph?
A graph data structure consists of a finite (and possibly mutable) set of vertices or nodes or points, together with a set of unordered pairs of these vertices for an undirected graph or a set of ordered pairs for a directed graph.
😳
Uh, Let's Just Model Something
Standard Works?
🤔 how about
The Things
- Volumes
- Books
- Chapters
- Verses
The Things
A Volume
- has a title
- has books

The Things
A Book
- has a title
- belongs to a volume
- has chapters
- has verses

The Things
A Chapter
- has a title
- has a number
- belongs to a book
- has verses

The Things
A Verse
- has a title
- has a number
- has text
- has a book
- has a chapter

The Things
A Volume
- has a title
- has books
A Book
- has a title
- belongs to a volume
- has chapters
- has verses
A Chapter
- has a title
- has a number
- belongs to a book
- has verses
A Verse
- has a title
- has text
- has a number
- belongs to a book
- belongs to a chapter
Graph Diagram
simplification: graph diagram: 1 verse of 1 chapter of 1 book of 1 volume

Graphs are powerful tools for modeling many real-world phenomena because they resemble our natural mental models and verbal descriptions of the underlying process.
relationships can be... complex
IRL,
and graphs are better at NOT imposing restrictions that (should but) don't exist IRL
🙈

everything is connected
even our verse model is more complex than originally diagrammed
- verses have relationships with other verses
- next
- previous
- references
- etc

Anyway...
4 verse of 2 chapter of 2 book of 1 volume

🤕 Watch Your Head
humans can't think about (nor diagram) the whole graph at once; instead focus on one node at a time.
let computers manage big picture complexity
Extracting Trees from Graphs
💪
or why app developers love this thing
From This Graph....
Tell me:
- Which node is the top / root?
- Where's the start?
- How should I traverse?
- Where's the end?

well that depends...
In a view where we want to highlight a verse:

from this graph...
...to this tree
start

In a view where we want to list volumes and the title of their books:

from this graph...
...to this tree
start

In a view where we want the chapters in a book to be the focus:

from this graph...
...to this "tree"
start

there is no right way to traverse a graph
as it depends on the focus of the view
IOW
client-side, domain-model transforms

what we've been doing...
- under-fetching
- over-fetching

What if I told you
-
exactly the parts of the graph that you need...
-
at any depth...
-
in whatever shape you need...
-
in 1 request?
that you can grab
How?
Model the Business Domain as a Graph
1.

on the server
GraphQL Notation
Volume
type Volume {
id: ID!
title: String!
books: [Book]
}
GraphQL type notation for a

Book
GraphQL type notation for a
type Book {
id: ID!
slug: Slug!
title: String!
volume: Volume!
chapters: [Chapter]!
verses: [Verse]!
}

Chapter
type Chapter {
id: ID!
number: Int!
title: String!
verses: [Verse]!
book: Book!
}
GraphQL type notation for a

Verse
GraphQL type notation for a
type Verse {
id: ID!
title: String!
number: Int!
text: String!
chapter: Chapter!
book: Book!
}

Queries & Mutations
& Subscriptions
Queries
type Query {
getVolume(id: ID!): Volume!
getBook(id: ID!): Book!
getChapter(id: ID!): Chapter!
getVerse(id: ID!): Verse!
}
GraphQL notation
input CreateVolumeInput {
name: String!
}
type VolumeMutationResults {
volume: Volume!
}
type Mutation {
createVolume(input: CreateVolumeInput): VolumeMutationResults
}
Mutations
GraphQL notation
Subscriptions
GraphQL notation
🙅♂️
nothing to see here
(beyond the scope of this talk)
Create the Resolvers
2.

on the server
Resolving Queries
const Query = {
getVolume: (_, { id }) => Volumes.get(id),
getBook: (_, { id }) => Books.get(id),
getChapter: (_, { id }) => Chapters.get(id),
getVerse: (_, { id }) => Verses.get(id),
};
imagine `Volumes`, `Books`, `Chapters`, and `Verses` represent some data service (db, web service, etc) that only returns scalar values
Resolving Fields
// How to resolve fields on a Book
const Book = {
// this is the default behavior
// and here just to illustrate
id: ({id}) => id,
volume: ({volumeId}) => Volumes.get(volumeId),
chapters: ({id}) => Chapters.findByBookId(id)
};
every field on the object acts as a function
(defaults to identity function)
functions on fields are executed on demand only when client asks for those fields.
Resolve the Mutations
const Mutation = {
createVolume: async (_, { input }) => {
const volume = await Volumes.insert(input);
return { volume };
}
};
the volume and books field resolution function is executed on demand (only when client has asked for it).
Ask for Exactly What You Need
3.
on the clients


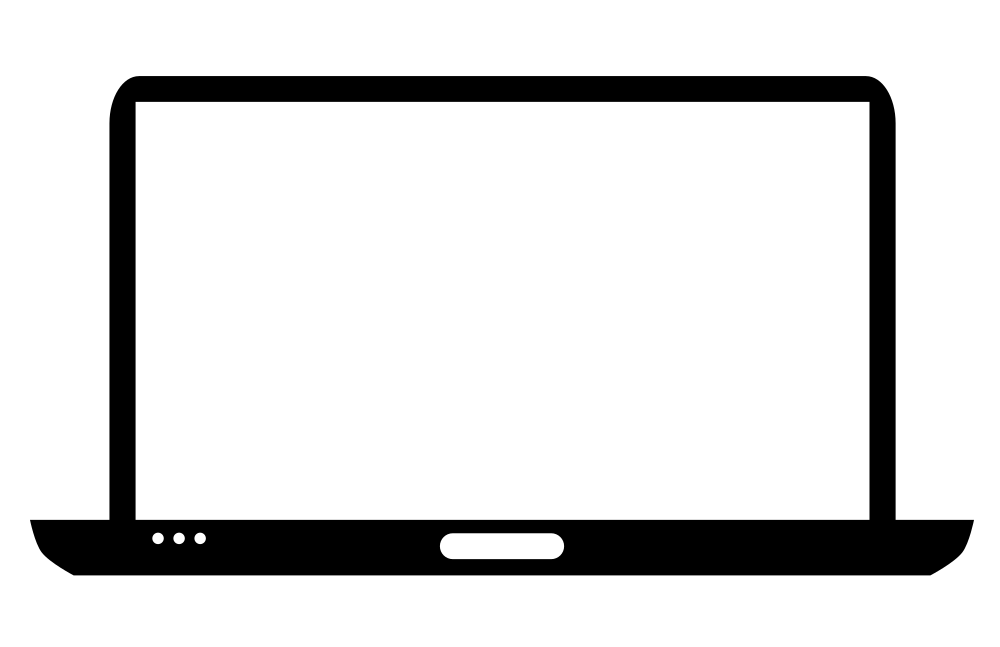
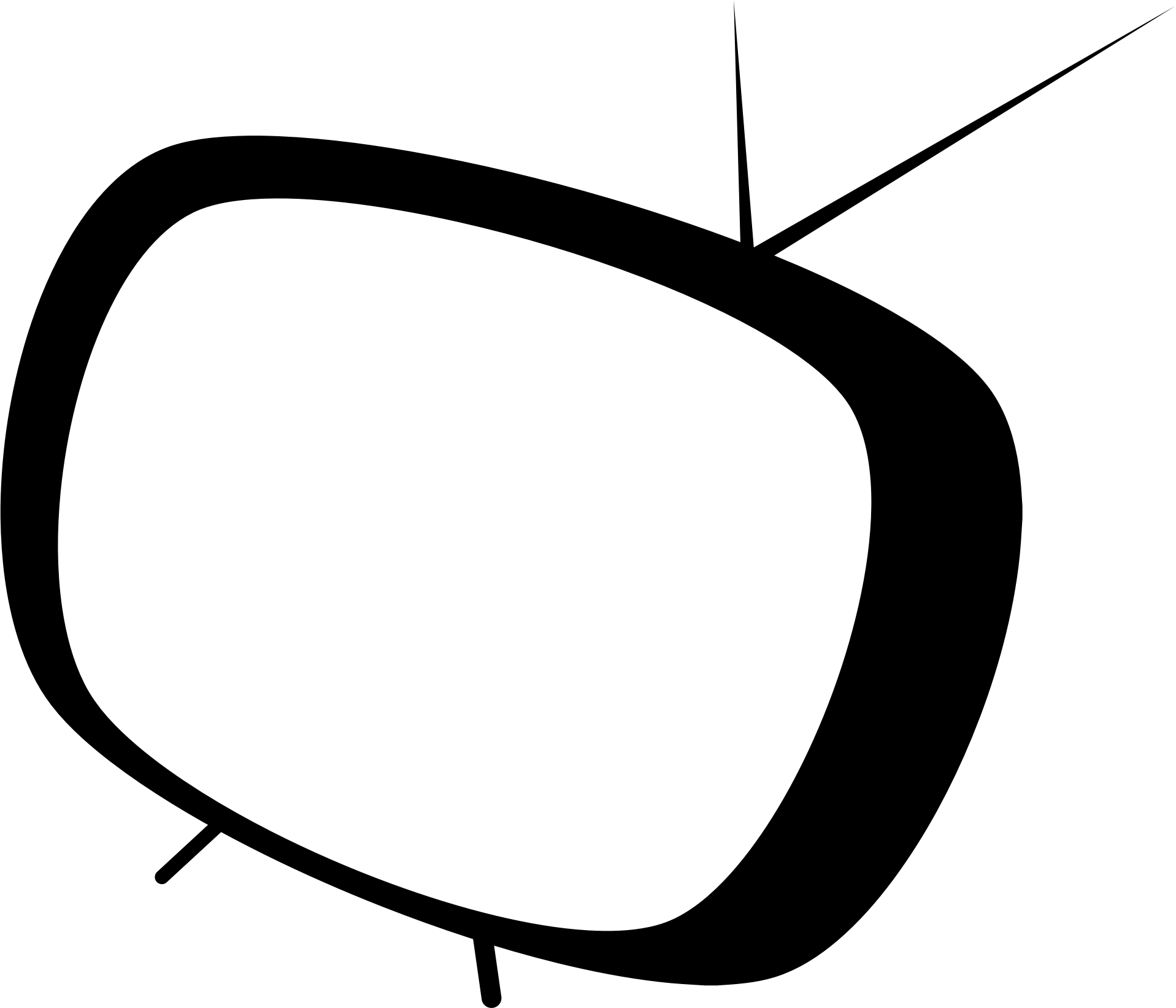
{
getVolume(id:"1") {
title
}
}
query
{
"data": {
"getVolume": {
"title": "Old Testament"
}
}
}
results
{
getVolume(id:"1") {
title
books {
title
}
}
}
query
{
"data": {
"getVolume": {
"title": "Old Testament",
"books": [
{
"title": "Genesis"
},
{
"title": "Exodus"
},
{
"title": "Leviticus"
},
{
"title": "Numbers"
},
{
"title": "Deuteronomy"
},
{
"title": "Joshua"
},
{
"title": "Judges"
},
{
"title": "Ruth"
},
{
"title": "1 Samuel"
},
{
"title": "2 Samuel"
},
{
"title": "1 Kings"
},
{
"title": "2 Kings"
},
{
"title": "1 Chronicles"
},
{
"title": "2 Chronicles"
},
{
"title": "Ezra"
},
{
"title": "Nehemiah"
},
{
"title": "Esther"
},
{
"title": "Job"
},
{
"title": "Psalms"
},
{
"title": "Proverbs"
},
{
"title": "Ecclesiastes"
},
{
"title": "Solomon's Song"
},
{
"title": "Isaiah"
},
{
"title": "Jeremiah"
},
{
"title": "Lamentations"
},
{
"title": "Ezekiel"
},
{
"title": "Daniel"
},
{
"title": "Hosea"
},
{
"title": "Joel"
},
{
"title": "Amos"
},
{
"title": "Obadiah"
},
{
"title": "Jonah"
},
{
"title": "Micah"
},
{
"title": "Nahum"
},
{
"title": "Habakkuk"
},
{
"title": "Zephaniah"
},
{
"title": "Haggai"
},
{
"title": "Zechariah"
},
{
"title": "Malachi"
}
]
}
}
}
results
{
getVolume(id:"1") {
title
books {
title
chapters {
title
}
}
}
}
query
{
"data": {
"getVolume": {
"title": "Old Testament",
"books": [
{
"title": "Genesis",
"chapters": [
{
"title": "Genesis 1"
},
{
"title": "Genesis 2"
},
{
"title": "Genesis 3"
},
{
"title": "Genesis 4"
},
{
"title": "Genesis 5"
},
{
"title": "Genesis 6"
},
{
"title": "Genesis 7"
},
{
"title": "Genesis 8"
},
{
"title": "Genesis 9"
},
{
"title": "Genesis 10"
},
{
"title": "Genesis 11"
},
{
"title": "Genesis 12"
},
{
"title": "Genesis 13"
},
{
"title": "Genesis 14"
},
{
"title": "Genesis 15"
},
{
"title": "Genesis 16"
},
{
"title": "Genesis 17"
},
{
"title": "Genesis 18"
},
{
"title": "Genesis 19"
},
{
"title": "Genesis 20"
},
{
"title": "Genesis 21"
},
{
"title": "Genesis 22"
},
{
"title": "Genesis 23"
},
{
"title": "Genesis 24"
},
{
"title": "Genesis 25"
},
{
"title": "Genesis 26"
},
{
"title": "Genesis 27"
},
{
"title": "Genesis 28"
},
{
"title": "Genesis 29"
},
{
"title": "Genesis 30"
},
{
"title": "Genesis 31"
},
{
"title": "Genesis 32"
},
{
"title": "Genesis 33"
},
{
"title": "Genesis 34"
},
{
"title": "Genesis 35"
},
{
"title": "Genesis 36"
},
{
"title": "Genesis 37"
},
{
"title": "Genesis 38"
},
{
"title": "Genesis 39"
},
{
"title": "Genesis 40"
},
{
"title": "Genesis 41"
},
{
"title": "Genesis 42"
},
{
"title": "Genesis 43"
},
{
"title": "Genesis 44"
},
{
"title": "Genesis 45"
},
{
"title": "Genesis 46"
},
{
"title": "Genesis 47"
},
{
"title": "Genesis 48"
},
{
"title": "Genesis 49"
},
{
"title": "Genesis 50"
}
]
},
{
"title": "Exodus",
"chapters": [
{
"title": "Exodus 1"
},
{
"title": "Exodus 2"
},
{
"title": "Exodus 3"
},
{
"title": "Exodus 4"
},
{
"title": "Exodus 5"
},
{
"title": "Exodus 6"
},
{
"title": "Exodus 7"
},
{
"title": "Exodus 8"
},
{
"title": "Exodus 9"
},
{
"title": "Exodus 10"
},
{
"title": "Exodus 11"
},
{
"title": "Exodus 12"
},
{
"title": "Exodus 13"
},
{
"title": "Exodus 14"
},
{
"title": "Exodus 15"
},
{
"title": "Exodus 16"
},
{
"title": "Exodus 17"
},
{
"title": "Exodus 18"
},
{
"title": "Exodus 19"
},
{
"title": "Exodus 20"
},
{
"title": "Exodus 21"
},
{
"title": "Exodus 22"
},
{
"title": "Exodus 23"
},
{
"title": "Exodus 24"
},
{
"title": "Exodus 25"
},
{
"title": "Exodus 26"
},
{
"title": "Exodus 27"
},
{
"title": "Exodus 28"
},
{
"title": "Exodus 29"
},
{
"title": "Exodus 30"
},
{
"title": "Exodus 31"
},
{
"title": "Exodus 32"
},
{
"title": "Exodus 33"
},
{
"title": "Exodus 34"
},
{
"title": "Exodus 35"
},
{
"title": "Exodus 36"
},
{
"title": "Exodus 37"
},
{
"title": "Exodus 38"
},
{
"title": "Exodus 39"
},
{
"title": "Exodus 40"
}
]
},
{
"title": "Leviticus",
"chapters": [
{
"title": "Leviticus 1"
},
{
"title": "Leviticus 2"
},
{
"title": "Leviticus 3"
},
{
"title": "Leviticus 4"
},
{
"title": "Leviticus 5"
},
{
"title": "Leviticus 6"
},
{
"title": "Leviticus 7"
},
{
"title": "Leviticus 8"
},
{
"title": "Leviticus 9"
},
{
"title": "Leviticus 10"
},
{
"title": "Leviticus 11"
},
{
"title": "Leviticus 12"
},
{
"title": "Leviticus 13"
},
{
"title": "Leviticus 14"
},
{
"title": "Leviticus 15"
},
{
"title": "Leviticus 16"
},
{
"title": "Leviticus 17"
},
{
"title": "Leviticus 18"
},
{
"title": "Leviticus 19"
},
{
"title": "Leviticus 20"
},
{
"title": "Leviticus 21"
},
{
"title": "Leviticus 22"
},
{
"title": "Leviticus 23"
},
{
"title": "Leviticus 24"
},
{
"title": "Leviticus 25"
},
{
"title": "Leviticus 26"
},
{
"title": "Leviticus 27"
}
]
},
{
"title": "Numbers",
"chapters": [
{
"title": "Numbers 1"
},
{
"title": "Numbers 2"
},
{
"title": "Numbers 3"
},
{
"title": "Numbers 4"
},
{
"title": "Numbers 5"
},
{
"title": "Numbers 6"
},
{
"title": "Numbers 7"
},
{
"title": "Numbers 8"
},
{
"title": "Numbers 9"
},
{
"title": "Numbers 10"
},
{
"title": "Numbers 11"
},
{
"title": "Numbers 12"
},
{
"title": "Numbers 13"
},
{
"title": "Numbers 14"
},
{
"title": "Numbers 15"
},
{
"title": "Numbers 16"
},
{
"title": "Numbers 17"
},
{
"title": "Numbers 18"
},
{
"title": "Numbers 19"
},
{
"title": "Numbers 20"
},
{
"title": "Numbers 21"
},
{
"title": "Numbers 22"
},
{
"title": "Numbers 23"
},
{
"title": "Numbers 24"
},
{
"title": "Numbers 25"
},
{
"title": "Numbers 26"
},
{
"title": "Numbers 27"
},
{
"title": "Numbers 28"
},
{
"title": "Numbers 29"
},
{
"title": "Numbers 30"
},
{
"title": "Numbers 31"
},
{
"title": "Numbers 32"
},
{
"title": "Numbers 33"
},
{
"title": "Numbers 34"
},
{
"title": "Numbers 35"
},
{
"title": "Numbers 36"
}
]
},
{
"title": "Deuteronomy",
"chapters": [
{
"title": "Deuteronomy 1"
},
{
"title": "Deuteronomy 2"
},
{
"title": "Deuteronomy 3"
},
{
"title": "Deuteronomy 4"
},
{
"title": "Deuteronomy 5"
},
{
"title": "Deuteronomy 6"
},
{
"title": "Deuteronomy 7"
},
{
"title": "Deuteronomy 8"
},
{
"title": "Deuteronomy 9"
},
{
"title": "Deuteronomy 10"
},
{
"title": "Deuteronomy 11"
},
{
"title": "Deuteronomy 12"
},
{
"title": "Deuteronomy 13"
},
{
"title": "Deuteronomy 14"
},
{
"title": "Deuteronomy 15"
},
{
"title": "Deuteronomy 16"
},
{
"title": "Deuteronomy 17"
},
{
"title": "Deuteronomy 18"
},
{
"title": "Deuteronomy 19"
},
{
"title": "Deuteronomy 20"
},
{
"title": "Deuteronomy 21"
},
{
"title": "Deuteronomy 22"
},
{
"title": "Deuteronomy 23"
},
{
"title": "Deuteronomy 24"
},
{
"title": "Deuteronomy 25"
},
{
"title": "Deuteronomy 26"
},
{
"title": "Deuteronomy 27"
},
{
"title": "Deuteronomy 28"
},
{
"title": "Deuteronomy 29"
},
{
"title": "Deuteronomy 30"
},
{
"title": "Deuteronomy 31"
},
{
"title": "Deuteronomy 32"
},
{
"title": "Deuteronomy 33"
},
{
"title": "Deuteronomy 34"
}
]
},
{
"title": "Joshua",
"chapters": [
{
"title": "Joshua 1"
},
{
"title": "Joshua 2"
},
{
"title": "Joshua 3"
},
{
"title": "Joshua 4"
},
{
"title": "Joshua 5"
},
{
"title": "Joshua 6"
},
{
"title": "Joshua 7"
},
{
"title": "Joshua 8"
},
{
"title": "Joshua 9"
},
{
"title": "Joshua 10"
},
{
"title": "Joshua 11"
},
{
"title": "Joshua 12"
},
{
"title": "Joshua 13"
},
{
"title": "Joshua 14"
},
{
"title": "Joshua 15"
},
{
"title": "Joshua 16"
},
{
"title": "Joshua 17"
},
{
"title": "Joshua 18"
},
{
"title": "Joshua 19"
},
{
"title": "Joshua 20"
},
{
"title": "Joshua 21"
},
{
"title": "Joshua 22"
},
{
"title": "Joshua 23"
},
{
"title": "Joshua 24"
}
]
},
{
"title": "Judges",
"chapters": [
{
"title": "Judges 1"
},
{
"title": "Judges 2"
},
{
"title": "Judges 3"
},
{
"title": "Judges 4"
},
{
"title": "Judges 5"
},
{
"title": "Judges 6"
},
{
"title": "Judges 7"
},
{
"title": "Judges 8"
},
{
"title": "Judges 9"
},
{
"title": "Judges 10"
},
{
"title": "Judges 11"
},
{
"title": "Judges 12"
},
{
"title": "Judges 13"
},
{
"title": "Judges 14"
},
{
"title": "Judges 15"
},
{
"title": "Judges 16"
},
{
"title": "Judges 17"
},
{
"title": "Judges 18"
},
{
"title": "Judges 19"
},
{
"title": "Judges 20"
},
{
"title": "Judges 21"
}
]
},
{
"title": "Ruth",
"chapters": [
{
"title": "Ruth 1"
},
{
"title": "Ruth 2"
},
{
"title": "Ruth 3"
},
{
"title": "Ruth 4"
}
]
},
{
"title": "1 Samuel",
"chapters": [
{
"title": "1 Samuel 1"
},
{
"title": "1 Samuel 2"
},
{
"title": "1 Samuel 3"
},
{
"title": "1 Samuel 4"
},
{
"title": "1 Samuel 5"
},
{
"title": "1 Samuel 6"
},
{
"title": "1 Samuel 7"
},
{
"title": "1 Samuel 8"
},
{
"title": "1 Samuel 9"
},
{
"title": "1 Samuel 10"
},
{
"title": "1 Samuel 11"
},
{
"title": "1 Samuel 12"
},
{
"title": "1 Samuel 13"
},
{
"title": "1 Samuel 14"
},
{
"title": "1 Samuel 15"
},
{
"title": "1 Samuel 16"
},
{
"title": "1 Samuel 17"
},
{
"title": "1 Samuel 18"
},
{
"title": "1 Samuel 19"
},
{
"title": "1 Samuel 20"
},
{
"title": "1 Samuel 21"
},
{
"title": "1 Samuel 22"
},
{
"title": "1 Samuel 23"
},
{
"title": "1 Samuel 24"
},
{
"title": "1 Samuel 25"
},
{
"title": "1 Samuel 26"
},
{
"title": "1 Samuel 27"
},
{
"title": "1 Samuel 28"
},
{
"title": "1 Samuel 29"
},
{
"title": "1 Samuel 30"
},
{
"title": "1 Samuel 31"
}
]
},
{
"title": "2 Samuel",
"chapters": [
{
"title": "2 Samuel 1"
},
{
"title": "2 Samuel 2"
},
{
"title": "2 Samuel 3"
},
{
"title": "2 Samuel 4"
},
{
"title": "2 Samuel 5"
},
{
"title": "2 Samuel 6"
},
{
"title": "2 Samuel 7"
},
{
"title": "2 Samuel 8"
},
{
"title": "2 Samuel 9"
},
{
"title": "2 Samuel 10"
},
{
"title": "2 Samuel 11"
},
{
"title": "2 Samuel 12"
},
{
"title": "2 Samuel 13"
},
{
"title": "2 Samuel 14"
},
{
"title": "2 Samuel 15"
},
{
"title": "2 Samuel 16"
},
{
"title": "2 Samuel 17"
},
{
"title": "2 Samuel 18"
},
{
"title": "2 Samuel 19"
},
{
"title": "2 Samuel 20"
},
{
"title": "2 Samuel 21"
},
{
"title": "2 Samuel 22"
},
{
"title": "2 Samuel 23"
},
{
"title": "2 Samuel 24"
}
]
},
{
"title": "1 Kings",
"chapters": [
{
"title": "1 Kings 1"
},
{
"title": "1 Kings 2"
},
{
"title": "1 Kings 3"
},
{
"title": "1 Kings 4"
},
{
"title": "1 Kings 5"
},
{
"title": "1 Kings 6"
},
{
"title": "1 Kings 7"
},
{
"title": "1 Kings 8"
},
{
"title": "1 Kings 9"
},
{
"title": "1 Kings 10"
},
{
"title": "1 Kings 11"
},
{
"title": "1 Kings 12"
},
{
"title": "1 Kings 13"
},
{
"title": "1 Kings 14"
},
{
"title": "1 Kings 15"
},
{
"title": "1 Kings 16"
},
{
"title": "1 Kings 17"
},
{
"title": "1 Kings 18"
},
{
"title": "1 Kings 19"
},
{
"title": "1 Kings 20"
},
{
"title": "1 Kings 21"
},
{
"title": "1 Kings 22"
}
]
},
{
"title": "2 Kings",
"chapters": [
{
"title": "2 Kings 1"
},
{
"title": "2 Kings 2"
},
{
"title": "2 Kings 3"
},
{
"title": "2 Kings 4"
},
{
"title": "2 Kings 5"
},
{
"title": "2 Kings 6"
},
{
"title": "2 Kings 7"
},
{
"title": "2 Kings 8"
},
{
"title": "2 Kings 9"
},
{
"title": "2 Kings 10"
},
{
"title": "2 Kings 11"
},
{
"title": "2 Kings 12"
},
{
"title": "2 Kings 13"
},
{
"title": "2 Kings 14"
},
{
"title": "2 Kings 15"
},
{
"title": "2 Kings 16"
},
{
"title": "2 Kings 17"
},
{
"title": "2 Kings 18"
},
{
"title": "2 Kings 19"
},
{
"title": "2 Kings 20"
},
{
"title": "2 Kings 21"
},
{
"title": "2 Kings 22"
},
{
"title": "2 Kings 23"
},
{
"title": "2 Kings 24"
},
{
"title": "2 Kings 25"
}
]
},
{
"title": "1 Chronicles",
"chapters": [
{
"title": "1 Chronicles 1"
},
{
"title": "1 Chronicles 2"
},
{
"title": "1 Chronicles 3"
},
{
"title": "1 Chronicles 4"
},
{
"title": "1 Chronicles 5"
},
{
"title": "1 Chronicles 6"
},
{
"title": "1 Chronicles 7"
},
{
"title": "1 Chronicles 8"
},
{
"title": "1 Chronicles 9"
},
{
"title": "1 Chronicles 10"
},
{
"title": "1 Chronicles 11"
},
{
"title": "1 Chronicles 12"
},
{
"title": "1 Chronicles 13"
},
{
"title": "1 Chronicles 14"
},
{
"title": "1 Chronicles 15"
},
{
"title": "1 Chronicles 16"
},
{
"title": "1 Chronicles 17"
},
{
"title": "1 Chronicles 18"
},
{
"title": "1 Chronicles 19"
},
{
"title": "1 Chronicles 20"
},
{
"title": "1 Chronicles 21"
},
{
"title": "1 Chronicles 22"
},
{
"title": "1 Chronicles 23"
},
{
"title": "1 Chronicles 24"
},
{
"title": "1 Chronicles 25"
},
{
"title": "1 Chronicles 26"
},
{
"title": "1 Chronicles 27"
},
{
"title": "1 Chronicles 28"
},
{
"title": "1 Chronicles 29"
}
]
},
{
"title": "2 Chronicles",
"chapters": [
{
"title": "2 Chronicles 1"
},
{
"title": "2 Chronicles 2"
},
{
"title": "2 Chronicles 3"
},
{
"title": "2 Chronicles 4"
},
{
"title": "2 Chronicles 5"
},
{
"title": "2 Chronicles 6"
},
{
"title": "2 Chronicles 7"
},
{
"title": "2 Chronicles 8"
},
{
"title": "2 Chronicles 9"
},
{
"title": "2 Chronicles 10"
},
{
"title": "2 Chronicles 11"
},
{
"title": "2 Chronicles 12"
},
{
"title": "2 Chronicles 13"
},
{
"title": "2 Chronicles 14"
},
{
"title": "2 Chronicles 15"
},
{
"title": "2 Chronicles 16"
},
{
"title": "2 Chronicles 17"
},
{
"title": "2 Chronicles 18"
},
{
"title": "2 Chronicles 19"
},
{
"title": "2 Chronicles 20"
},
{
"title": "2 Chronicles 21"
},
{
"title": "2 Chronicles 22"
},
{
"title": "2 Chronicles 23"
},
{
"title": "2 Chronicles 24"
},
{
"title": "2 Chronicles 25"
},
{
"title": "2 Chronicles 26"
},
{
"title": "2 Chronicles 27"
},
{
"title": "2 Chronicles 28"
},
{
"title": "2 Chronicles 29"
},
{
"title": "2 Chronicles 30"
},
{
"title": "2 Chronicles 31"
},
{
"title": "2 Chronicles 32"
},
{
"title": "2 Chronicles 33"
},
{
"title": "2 Chronicles 34"
},
{
"title": "2 Chronicles 35"
},
{
"title": "2 Chronicles 36"
}
]
},
{
"title": "Ezra",
"chapters": [
{
"title": "Ezra 1"
},
{
"title": "Ezra 2"
},
{
"title": "Ezra 3"
},
{
"title": "Ezra 4"
},
{
"title": "Ezra 5"
},
{
"title": "Ezra 6"
},
{
"title": "Ezra 7"
},
{
"title": "Ezra 8"
},
{
"title": "Ezra 9"
},
{
"title": "Ezra 10"
}
]
},
{
"title": "Nehemiah",
"chapters": [
{
"title": "Nehemiah 1"
},
{
"title": "Nehemiah 2"
},
{
"title": "Nehemiah 3"
},
{
"title": "Nehemiah 4"
},
{
"title": "Nehemiah 5"
},
{
"title": "Nehemiah 6"
},
{
"title": "Nehemiah 7"
},
{
"title": "Nehemiah 8"
},
{
"title": "Nehemiah 9"
},
{
"title": "Nehemiah 10"
},
{
"title": "Nehemiah 11"
},
{
"title": "Nehemiah 12"
},
{
"title": "Nehemiah 13"
}
]
},
{
"title": "Esther",
"chapters": [
{
"title": "Esther 1"
},
{
"title": "Esther 2"
},
{
"title": "Esther 3"
},
{
"title": "Esther 4"
},
{
"title": "Esther 5"
},
{
"title": "Esther 6"
},
{
"title": "Esther 7"
},
{
"title": "Esther 8"
},
{
"title": "Esther 9"
},
{
"title": "Esther 10"
}
]
},
{
"title": "Job",
"chapters": [
{
"title": "Job 1"
},
{
"title": "Job 2"
},
{
"title": "Job 3"
},
{
"title": "Job 4"
},
{
"title": "Job 5"
},
{
"title": "Job 6"
},
{
"title": "Job 7"
},
{
"title": "Job 8"
},
{
"title": "Job 9"
},
{
"title": "Job 10"
},
{
"title": "Job 11"
},
{
"title": "Job 12"
},
{
"title": "Job 13"
},
{
"title": "Job 14"
},
{
"title": "Job 15"
},
{
"title": "Job 16"
},
{
"title": "Job 17"
},
{
"title": "Job 18"
},
{
"title": "Job 19"
},
{
"title": "Job 20"
},
{
"title": "Job 21"
},
{
"title": "Job 22"
},
{
"title": "Job 23"
},
{
"title": "Job 24"
},
{
"title": "Job 25"
},
{
"title": "Job 26"
},
{
"title": "Job 27"
},
{
"title": "Job 28"
},
{
"title": "Job 29"
},
{
"title": "Job 30"
},
{
"title": "Job 31"
},
{
"title": "Job 32"
},
{
"title": "Job 33"
},
{
"title": "Job 34"
},
{
"title": "Job 35"
},
{
"title": "Job 36"
},
{
"title": "Job 37"
},
{
"title": "Job 38"
},
{
"title": "Job 39"
},
{
"title": "Job 40"
},
{
"title": "Job 41"
},
{
"title": "Job 42"
}
]
},
{
"title": "Psalms",
"chapters": [
{
"title": "Psalms 1"
},
{
"title": "Psalms 2"
},
{
"title": "Psalms 3"
},
{
"title": "Psalms 4"
},
{
"title": "Psalms 5"
},
{
"title": "Psalms 6"
},
{
"title": "Psalms 7"
},
{
"title": "Psalms 8"
},
{
"title": "Psalms 9"
},
{
"title": "Psalms 10"
},
{
"title": "Psalms 11"
},
{
"title": "Psalms 12"
},
{
"title": "Psalms 13"
},
{
"title": "Psalms 14"
},
{
"title": "Psalms 15"
},
{
"title": "Psalms 16"
},
{
"title": "Psalms 17"
},
{
"title": "Psalms 18"
},
{
"title": "Psalms 19"
},
{
"title": "Psalms 20"
},
{
"title": "Psalms 21"
},
{
"title": "Psalms 22"
},
{
"title": "Psalms 23"
},
{
"title": "Psalms 24"
},
{
"title": "Psalms 25"
},
{
"title": "Psalms 26"
},
{
"title": "Psalms 27"
},
{
"title": "Psalms 28"
},
{
"title": "Psalms 29"
},
{
"title": "Psalms 30"
},
{
"title": "Psalms 31"
},
{
"title": "Psalms 32"
},
{
"title": "Psalms 33"
},
{
"title": "Psalms 34"
},
{
"title": "Psalms 35"
},
{
"title": "Psalms 36"
},
{
"title": "Psalms 37"
},
{
"title": "Psalms 38"
},
{
"title": "Psalms 39"
},
{
"title": "Psalms 40"
},
{
"title": "Psalms 41"
},
{
"title": "Psalms 42"
},
{
"title": "Psalms 43"
},
{
"title": "Psalms 44"
},
{
"title": "Psalms 45"
},
{
"title": "Psalms 46"
},
{
"title": "Psalms 47"
},
{
"title": "Psalms 48"
},
{
"title": "Psalms 49"
},
{
"title": "Psalms 50"
},
{
"title": "Psalms 51"
},
{
"title": "Psalms 52"
},
{
"title": "Psalms 53"
},
{
"title": "Psalms 54"
},
{
"title": "Psalms 55"
},
{
"title": "Psalms 56"
},
{
"title": "Psalms 57"
},
{
"title": "Psalms 58"
},
{
"title": "Psalms 59"
},
{
"title": "Psalms 60"
},
{
"title": "Psalms 61"
},
{
"title": "Psalms 62"
},
{
"title": "Psalms 63"
},
{
"title": "Psalms 64"
},
{
"title": "Psalms 65"
},
{
"title": "Psalms 66"
},
{
"title": "Psalms 67"
},
{
"title": "Psalms 68"
},
{
"title": "Psalms 69"
},
{
"title": "Psalms 70"
},
{
"title": "Psalms 71"
},
{
"title": "Psalms 72"
},
{
"title": "Psalms 73"
},
{
"title": "Psalms 74"
},
{
"title": "Psalms 75"
},
{
"title": "Psalms 76"
},
{
"title": "Psalms 77"
},
{
"title": "Psalms 78"
},
{
"title": "Psalms 79"
},
{
"title": "Psalms 80"
},
{
"title": "Psalms 81"
},
{
"title": "Psalms 82"
},
{
"title": "Psalms 83"
},
{
"title": "Psalms 84"
},
{
"title": "Psalms 85"
},
{
"title": "Psalms 86"
},
{
"title": "Psalms 87"
},
{
"title": "Psalms 88"
},
{
"title": "Psalms 89"
},
{
"title": "Psalms 90"
},
{
"title": "Psalms 91"
},
{
"title": "Psalms 92"
},
{
"title": "Psalms 93"
},
{
"title": "Psalms 94"
},
{
"title": "Psalms 95"
},
{
"title": "Psalms 96"
},
{
"title": "Psalms 97"
},
{
"title": "Psalms 98"
},
{
"title": "Psalms 99"
},
{
"title": "Psalms 100"
},
{
"title": "Psalms 101"
},
{
"title": "Psalms 102"
},
{
"title": "Psalms 103"
},
{
"title": "Psalms 104"
},
{
"title": "Psalms 105"
},
{
"title": "Psalms 106"
},
{
"title": "Psalms 107"
},
{
"title": "Psalms 108"
},
{
"title": "Psalms 109"
},
{
"title": "Psalms 110"
},
{
"title": "Psalms 111"
},
{
"title": "Psalms 112"
},
{
"title": "Psalms 113"
},
{
"title": "Psalms 114"
},
{
"title": "Psalms 115"
},
{
"title": "Psalms 116"
},
{
"title": "Psalms 117"
},
{
"title": "Psalms 118"
},
{
"title": "Psalms 119"
},
{
"title": "Psalms 120"
},
{
"title": "Psalms 121"
},
{
"title": "Psalms 122"
},
{
"title": "Psalms 123"
},
{
"title": "Psalms 124"
},
{
"title": "Psalms 125"
},
{
"title": "Psalms 126"
},
{
"title": "Psalms 127"
},
{
"title": "Psalms 128"
},
{
"title": "Psalms 129"
},
{
"title": "Psalms 130"
},
{
"title": "Psalms 131"
},
{
"title": "Psalms 132"
},
{
"title": "Psalms 133"
},
{
"title": "Psalms 134"
},
{
"title": "Psalms 135"
},
{
"title": "Psalms 136"
},
{
"title": "Psalms 137"
},
{
"title": "Psalms 138"
},
{
"title": "Psalms 139"
},
{
"title": "Psalms 140"
},
{
"title": "Psalms 141"
},
{
"title": "Psalms 142"
},
{
"title": "Psalms 143"
},
{
"title": "Psalms 144"
},
{
"title": "Psalms 145"
},
{
"title": "Psalms 146"
},
{
"title": "Psalms 147"
},
{
"title": "Psalms 148"
},
{
"title": "Psalms 149"
},
{
"title": "Psalms 150"
}
]
},
{
"title": "Proverbs",
"chapters": [
{
"title": "Proverbs 1"
},
{
"title": "Proverbs 2"
},
{
"title": "Proverbs 3"
},
{
"title": "Proverbs 4"
},
{
"title": "Proverbs 5"
},
{
"title": "Proverbs 6"
},
{
"title": "Proverbs 7"
},
{
"title": "Proverbs 8"
},
{
"title": "Proverbs 9"
},
{
"title": "Proverbs 10"
},
{
"title": "Proverbs 11"
},
{
"title": "Proverbs 12"
},
{
"title": "Proverbs 13"
},
{
"title": "Proverbs 14"
},
{
"title": "Proverbs 15"
},
{
"title": "Proverbs 16"
},
{
"title": "Proverbs 17"
},
{
"title": "Proverbs 18"
},
{
"title": "Proverbs 19"
},
{
"title": "Proverbs 20"
},
{
"title": "Proverbs 21"
},
{
"title": "Proverbs 22"
},
{
"title": "Proverbs 23"
},
{
"title": "Proverbs 24"
},
{
"title": "Proverbs 25"
},
{
"title": "Proverbs 26"
},
{
"title": "Proverbs 27"
},
{
"title": "Proverbs 28"
},
{
"title": "Proverbs 29"
},
{
"title": "Proverbs 30"
},
{
"title": "Proverbs 31"
}
]
},
{
"title": "Ecclesiastes",
"chapters": [
{
"title": "Ecclesiastes 1"
},
{
"title": "Ecclesiastes 2"
},
{
"title": "Ecclesiastes 3"
},
{
"title": "Ecclesiastes 4"
},
{
"title": "Ecclesiastes 5"
},
{
"title": "Ecclesiastes 6"
},
{
"title": "Ecclesiastes 7"
},
{
"title": "Ecclesiastes 8"
},
{
"title": "Ecclesiastes 9"
},
{
"title": "Ecclesiastes 10"
},
{
"title": "Ecclesiastes 11"
},
{
"title": "Ecclesiastes 12"
}
]
},
{
"title": "Solomon's Song",
"chapters": [
{
"title": "Solomon's Song 1"
},
{
"title": "Solomon's Song 2"
},
{
"title": "Solomon's Song 3"
},
{
"title": "Solomon's Song 4"
},
{
"title": "Solomon's Song 5"
},
{
"title": "Solomon's Song 6"
},
{
"title": "Solomon's Song 7"
},
{
"title": "Solomon's Song 8"
}
]
},
{
"title": "Isaiah",
"chapters": [
{
"title": "Isaiah 1"
},
{
"title": "Isaiah 2"
},
{
"title": "Isaiah 3"
},
{
"title": "Isaiah 4"
},
{
"title": "Isaiah 5"
},
{
"title": "Isaiah 6"
},
{
"title": "Isaiah 7"
},
{
"title": "Isaiah 8"
},
{
"title": "Isaiah 9"
},
{
"title": "Isaiah 10"
},
{
"title": "Isaiah 11"
},
{
"title": "Isaiah 12"
},
{
"title": "Isaiah 13"
},
{
"title": "Isaiah 14"
},
{
"title": "Isaiah 15"
},
{
"title": "Isaiah 16"
},
{
"title": "Isaiah 17"
},
{
"title": "Isaiah 18"
},
{
"title": "Isaiah 19"
},
{
"title": "Isaiah 20"
},
{
"title": "Isaiah 21"
},
{
"title": "Isaiah 22"
},
{
"title": "Isaiah 23"
},
{
"title": "Isaiah 24"
},
{
"title": "Isaiah 25"
},
{
"title": "Isaiah 26"
},
{
"title": "Isaiah 27"
},
{
"title": "Isaiah 28"
},
{
"title": "Isaiah 29"
},
{
"title": "Isaiah 30"
},
{
"title": "Isaiah 31"
},
{
"title": "Isaiah 32"
},
{
"title": "Isaiah 33"
},
{
"title": "Isaiah 34"
},
{
"title": "Isaiah 35"
},
{
"title": "Isaiah 36"
},
{
"title": "Isaiah 37"
},
{
"title": "Isaiah 38"
},
{
"title": "Isaiah 39"
},
{
"title": "Isaiah 40"
},
{
"title": "Isaiah 41"
},
{
"title": "Isaiah 42"
},
{
"title": "Isaiah 43"
},
{
"title": "Isaiah 44"
},
{
"title": "Isaiah 45"
},
{
"title": "Isaiah 46"
},
{
"title": "Isaiah 47"
},
{
"title": "Isaiah 48"
},
{
"title": "Isaiah 49"
},
{
"title": "Isaiah 50"
},
{
"title": "Isaiah 51"
},
{
"title": "Isaiah 52"
},
{
"title": "Isaiah 53"
},
{
"title": "Isaiah 54"
},
{
"title": "Isaiah 55"
},
{
"title": "Isaiah 56"
},
{
"title": "Isaiah 57"
},
{
"title": "Isaiah 58"
},
{
"title": "Isaiah 59"
},
{
"title": "Isaiah 60"
},
{
"title": "Isaiah 61"
},
{
"title": "Isaiah 62"
},
{
"title": "Isaiah 63"
},
{
"title": "Isaiah 64"
},
{
"title": "Isaiah 65"
},
{
"title": "Isaiah 66"
}
]
},
{
"title": "Jeremiah",
"chapters": [
{
"title": "Jeremiah 1"
},
{
"title": "Jeremiah 2"
},
{
"title": "Jeremiah 3"
},
{
"title": "Jeremiah 4"
},
{
"title": "Jeremiah 5"
},
{
"title": "Jeremiah 6"
},
{
"title": "Jeremiah 7"
},
{
"title": "Jeremiah 8"
},
{
"title": "Jeremiah 9"
},
{
"title": "Jeremiah 10"
},
{
"title": "Jeremiah 11"
},
{
"title": "Jeremiah 12"
},
{
"title": "Jeremiah 13"
},
{
"title": "Jeremiah 14"
},
{
"title": "Jeremiah 15"
},
{
"title": "Jeremiah 16"
},
{
"title": "Jeremiah 17"
},
{
"title": "Jeremiah 18"
},
{
"title": "Jeremiah 19"
},
{
"title": "Jeremiah 20"
},
{
"title": "Jeremiah 21"
},
{
"title": "Jeremiah 22"
},
{
"title": "Jeremiah 23"
},
{
"title": "Jeremiah 24"
},
{
"title": "Jeremiah 25"
},
{
"title": "Jeremiah 26"
},
{
"title": "Jeremiah 27"
},
{
"title": "Jeremiah 28"
},
{
"title": "Jeremiah 29"
},
{
"title": "Jeremiah 30"
},
{
"title": "Jeremiah 31"
},
{
"title": "Jeremiah 32"
},
{
"title": "Jeremiah 33"
},
{
"title": "Jeremiah 34"
},
{
"title": "Jeremiah 35"
},
{
"title": "Jeremiah 36"
},
{
"title": "Jeremiah 37"
},
{
"title": "Jeremiah 38"
},
{
"title": "Jeremiah 39"
},
{
"title": "Jeremiah 40"
},
{
"title": "Jeremiah 41"
},
{
"title": "Jeremiah 42"
},
{
"title": "Jeremiah 43"
},
{
"title": "Jeremiah 44"
},
{
"title": "Jeremiah 45"
},
{
"title": "Jeremiah 46"
},
{
"title": "Jeremiah 47"
},
{
"title": "Jeremiah 48"
},
{
"title": "Jeremiah 49"
},
{
"title": "Jeremiah 50"
},
{
"title": "Jeremiah 51"
},
{
"title": "Jeremiah 52"
}
]
},
{
"title": "Lamentations",
"chapters": [
{
"title": "Lamentations 1"
},
{
"title": "Lamentations 2"
},
{
"title": "Lamentations 3"
},
{
"title": "Lamentations 4"
},
{
"title": "Lamentations 5"
}
]
},
{
"title": "Ezekiel",
"chapters": [
{
"title": "Ezekiel 1"
},
{
"title": "Ezekiel 2"
},
{
"title": "Ezekiel 3"
},
{
"title": "Ezekiel 4"
},
{
"title": "Ezekiel 5"
},
{
"title": "Ezekiel 6"
},
{
"title": "Ezekiel 7"
},
{
"title": "Ezekiel 8"
},
{
"title": "Ezekiel 9"
},
{
"title": "Ezekiel 10"
},
{
"title": "Ezekiel 11"
},
{
"title": "Ezekiel 12"
},
{
"title": "Ezekiel 13"
},
{
"title": "Ezekiel 14"
},
{
"title": "Ezekiel 15"
},
{
"title": "Ezekiel 16"
},
{
"title": "Ezekiel 17"
},
{
"title": "Ezekiel 18"
},
{
"title": "Ezekiel 19"
},
{
"title": "Ezekiel 20"
},
{
"title": "Ezekiel 21"
},
{
"title": "Ezekiel 22"
},
{
"title": "Ezekiel 23"
},
{
"title": "Ezekiel 24"
},
{
"title": "Ezekiel 25"
},
{
"title": "Ezekiel 26"
},
{
"title": "Ezekiel 27"
},
{
"title": "Ezekiel 28"
},
{
"title": "Ezekiel 29"
},
{
"title": "Ezekiel 30"
},
{
"title": "Ezekiel 31"
},
{
"title": "Ezekiel 32"
},
{
"title": "Ezekiel 33"
},
{
"title": "Ezekiel 34"
},
{
"title": "Ezekiel 35"
},
{
"title": "Ezekiel 36"
},
{
"title": "Ezekiel 37"
},
{
"title": "Ezekiel 38"
},
{
"title": "Ezekiel 39"
},
{
"title": "Ezekiel 40"
},
{
"title": "Ezekiel 41"
},
{
"title": "Ezekiel 42"
},
{
"title": "Ezekiel 43"
},
{
"title": "Ezekiel 44"
},
{
"title": "Ezekiel 45"
},
{
"title": "Ezekiel 46"
},
{
"title": "Ezekiel 47"
},
{
"title": "Ezekiel 48"
}
]
},
{
"title": "Daniel",
"chapters": [
{
"title": "Daniel 1"
},
{
"title": "Daniel 2"
},
{
"title": "Daniel 3"
},
{
"title": "Daniel 4"
},
{
"title": "Daniel 5"
},
{
"title": "Daniel 6"
},
{
"title": "Daniel 7"
},
{
"title": "Daniel 8"
},
{
"title": "Daniel 9"
},
{
"title": "Daniel 10"
},
{
"title": "Daniel 11"
},
{
"title": "Daniel 12"
}
]
},
{
"title": "Hosea",
"chapters": [
{
"title": "Hosea 1"
},
{
"title": "Hosea 2"
},
{
"title": "Hosea 3"
},
{
"title": "Hosea 4"
},
{
"title": "Hosea 5"
},
{
"title": "Hosea 6"
},
{
"title": "Hosea 7"
},
{
"title": "Hosea 8"
},
{
"title": "Hosea 9"
},
{
"title": "Hosea 10"
},
{
"title": "Hosea 11"
},
{
"title": "Hosea 12"
},
{
"title": "Hosea 13"
},
{
"title": "Hosea 14"
}
]
},
{
"title": "Joel",
"chapters": [
{
"title": "Joel 1"
},
{
"title": "Joel 2"
},
{
"title": "Joel 3"
}
]
},
{
"title": "Amos",
"chapters": [
{
"title": "Amos 1"
},
{
"title": "Amos 2"
},
{
"title": "Amos 3"
},
{
"title": "Amos 4"
},
{
"title": "Amos 5"
},
{
"title": "Amos 6"
},
{
"title": "Amos 7"
},
{
"title": "Amos 8"
},
{
"title": "Amos 9"
}
]
},
{
"title": "Obadiah",
"chapters": [
{
"title": "Obadiah 1"
}
]
},
{
"title": "Jonah",
"chapters": [
{
"title": "Jonah 1"
},
{
"title": "Jonah 2"
},
{
"title": "Jonah 3"
},
{
"title": "Jonah 4"
}
]
},
{
"title": "Micah",
"chapters": [
{
"title": "Micah 1"
},
{
"title": "Micah 2"
},
{
"title": "Micah 3"
},
{
"title": "Micah 4"
},
{
"title": "Micah 5"
},
{
"title": "Micah 6"
},
{
"title": "Micah 7"
}
]
},
{
"title": "Nahum",
"chapters": [
{
"title": "Nahum 1"
},
{
"title": "Nahum 2"
},
{
"title": "Nahum 3"
}
]
},
{
"title": "Habakkuk",
"chapters": [
{
"title": "Habakkuk 1"
},
{
"title": "Habakkuk 2"
},
{
"title": "Habakkuk 3"
}
]
},
{
"title": "Zephaniah",
"chapters": [
{
"title": "Zephaniah 1"
},
{
"title": "Zephaniah 2"
},
{
"title": "Zephaniah 3"
}
]
},
{
"title": "Haggai",
"chapters": [
{
"title": "Haggai 1"
},
{
"title": "Haggai 2"
}
]
},
{
"title": "Zechariah",
"chapters": [
{
"title": "Zechariah 1"
},
{
"title": "Zechariah 2"
},
{
"title": "Zechariah 3"
},
{
"title": "Zechariah 4"
},
{
"title": "Zechariah 5"
},
{
"title": "Zechariah 6"
},
{
"title": "Zechariah 7"
},
{
"title": "Zechariah 8"
},
{
"title": "Zechariah 9"
},
{
"title": "Zechariah 10"
},
{
"title": "Zechariah 11"
},
{
"title": "Zechariah 12"
},
{
"title": "Zechariah 13"
},
{
"title": "Zechariah 14"
}
]
},
{
"title": "Malachi",
"chapters": [
{
"title": "Malachi 1"
},
{
"title": "Malachi 2"
},
{
"title": "Malachi 3"
},
{
"title": "Malachi 4"
}
]
}
]
}
}
}
results
{
getVerse(id:"1") {
title
text
chapter {
book {
title
volume {
title
}
}
}
}
}
query
{
"data": {
"getVerse": {
"title": "Genesis 1:1",
"text": "IN the beginning God created the heaven and the earth.",
"chapter": {
"book": {
"title": "Genesis",
"volume": {
"title": "Old Testament"
}
}
}
}
}
}
results
{
q2: getVerse(id: "1") {
title
text
chapter {
book {
title
volume {
title
}
}
}
}
q1: getVerse(id: "41995") {
title
text
chapter {
book {
title
volume {
title
}
}
}
}
}
query
{
"data": {
"q2": {
"title": "Genesis 1:1",
"text": "IN the beginning God created the heaven and the earth.",
"chapter": {
"book": {
"title": "Genesis",
"volume": {
"title": "Old Testament"
}
}
}
},
"q1": {
"title": "Articles of Faith 1:13",
"text": "We believe in being honest, true, chaste, benevolent, virtuous, and in doing good to all men; indeed, we may say that we follow the admonition of Paul--We believe all things, we hope all things, we have endured many things, and hope to be able to endure all things. If there is anything virtuous, lovely, or of good report or praiseworthy, we seek after these things.",
"chapter": {
"book": {
"title": "Articles of Faith",
"volume": {
"title": "Pearl of Great Price"
}
}
}
}
}
}
results
dynamic and complex relationships?
query VerseSearch($input: VerseSearchInput) {
allVerses(input: $input, first:10) {
pageInfo {
total
hasNextPage
}
}
}
query
{
"data": {
"allVerses": {
"pageInfo": {
"total": 82,
"hasNextPage": true
}
}
}
}
results
{
"input": {
"keywords": "Jared"
}
}
input
query VerseSearch($input: VerseSearchInput) {
allVerses(input: $input, first:83) {
pageInfo {
total
hasNextPage
}
edges {
node {
title
text
}
}
}
}
query
{
"data": {
"allVerses": {
"pageInfo": {
"total": 82,
"hasNextPage": false
},
"edges": [
{
"node": {
"title": "Genesis 5:15",
"text": "And Mahalaleel lived sixty and five years, and begat Jared:"
}
},
{
"node": {
"title": "Genesis 5:16",
"text": "And Mahalaleel lived after he begat Jared eight hundred and thirty years, and begat sons and daughters:"
}
},
{
"node": {
"title": "Genesis 5:18",
"text": "And Jared lived an hundred sixty and two years, and he begat Enoch:"
}
},
{
"node": {
"title": "Genesis 5:19",
"text": "And Jared lived after he begat Enoch eight hundred years, and begat sons and daughters:"
}
},
{
"node": {
"title": "Genesis 5:20",
"text": "And all the days of Jared were nine hundred sixty and two years: and he died."
}
},
{
"node": {
"title": "Luke 3:37",
"text": "Which was the son of Mathusala, which was the son of Enoch, which was the son of Jared, which was the son of Maleleel, which was the son of Cainan,"
}
},
{
"node": {
"title": "Ether 1:32",
"text": "And Kib was the son of Orihah, who was the son of Jared;"
}
},
{
"node": {
"title": "Ether 1:33",
"text": "Which Jared came forth with his brother and their families, with some others and their families, from the great tower, at the time the Lord confounded the language of the people, and swore in his wrath that they should be scattered upon all the face of the earth; and according to the word of the Lord the people were scattered."
}
},
{
"node": {
"title": "Ether 1:34",
"text": "And the brother of Jared being a large and mighty man, and a man highly favored of the Lord, Jared, his brother, said unto him: Cry unto the Lord, that he will not confound us that we may not understand our words."
}
},
{
"node": {
"title": "Ether 1:35",
"text": "And it came to pass that the brother of Jared did cry unto the Lord, and the Lord had compassion upon Jared; therefore he did not confound the language of Jared; and Jared and his brother were not confounded."
}
},
{
"node": {
"title": "Ether 1:36",
"text": "Then Jared said unto his brother: Cry again unto the Lord, and it may be that he will turn away his anger from them who are our friends, that he confound not their language."
}
},
{
"node": {
"title": "Ether 1:37",
"text": "And it came to pass that the brother of Jared did cry unto the Lord, and the Lord had compassion upon their friends and their families also, that they were not confounded."
}
},
{
"node": {
"title": "Ether 1:38",
"text": "And it came to pass that Jared spake again unto his brother, saying: Go and inquire of the Lord whether he will drive us out of the land, and if he will drive us out of the land, cry unto him whither we shall go. And who knoweth but the Lord will carry us forth into a land which is choice above all the earth? And if it so be, let us be faithful unto the Lord, that we may receive it for our inheritance."
}
},
{
"node": {
"title": "Ether 1:39",
"text": "And it came to pass that the brother of Jared did cry unto the Lord according to that which had been spoken by the mouth of Jared."
}
},
{
"node": {
"title": "Ether 1:40",
"text": "And it came to pass that the Lord did hear the brother of Jared, and had compassion upon him, and said unto him:"
}
},
{
"node": {
"title": "Ether 1:41",
"text": "Go to and gather together thy flocks, both male and female, of every kind; and also of the seed of the earth of every kind; and thy families; and also Jared thy brother and his family; and also thy friends and their families, and the friends of Jared and their families."
}
},
{
"node": {
"title": "Ether 2:1",
"text": "And it came to pass that Jared and his brother, and their families, and also the friends of Jared and his brother and their families, went down into the valley which was northward, (and the name of the valley was Nimrod, being called after the mighty hunter) with their flocks which they had gathered together, male and female, of every kind."
}
},
{
"node": {
"title": "Ether 2:4",
"text": "And it came to pass that when they had come down into the valley of Nimrod the Lord came down and talked with the brother of Jared; and he was in a cloud, and the brother of Jared saw him not."
}
},
{
"node": {
"title": "Ether 2:8",
"text": "And he had sworn in his wrath unto the brother of Jared, that whoso should possess this land of promise, from that time henceforth and forever, should serve him, the true and only God, or they should be swept off when the fulness of his wrath should come upon them."
}
},
{
"node": {
"title": "Ether 2:13",
"text": "And now I proceed with my record; for behold, it came to pass that the Lord did bring Jared and his brethren forth even to that great sea which divideth the lands. And as they came to the sea they pitched their tents; and they called the name of the place Moriancumer; and they dwelt in tents, and dwelt in tents upon the seashore for the space of four years."
}
},
{
"node": {
"title": "Ether 2:14",
"text": "And it came to pass at the end of four years that the Lord came again unto the brother of Jared, and stood in a cloud and talked with him. And for the space of three hours did the Lord talk with the brother of Jared, and chastened him because he remembered not to call upon the name of the Lord."
}
},
{
"node": {
"title": "Ether 2:15",
"text": "And the brother of Jared repented of the evil which he had done, and did call upon the name of the Lord for his brethren who were with him. And the Lord said unto him: I will forgive thee and thy brethren of their sins; but thou shalt not sin any more, for ye shall remember that my Spirit will not always strive with man; wherefore, if ye will sin until ye are fully ripe ye shall be cut off from the presence of the Lord. And these are my thoughts upon the land which I shall give you for your inheritance; for it shall be a land choice above all other lands."
}
},
{
"node": {
"title": "Ether 2:16",
"text": "And the Lord said: Go to work and build, after the manner of barges which ye have hitherto built. And it came to pass that the brother of Jared did go to work, and also his brethren, and built barges after the manner which they had built, according to the instructions of the Lord. And they were small, and they were light upon the water, even like unto the lightness of a fowl upon the water."
}
},
{
"node": {
"title": "Ether 2:18",
"text": "And it came to pass that the brother of Jared cried unto the Lord, saying: O Lord, I have performed the work which thou hast commanded me, and I have made the barges according as thou hast directed me."
}
},
{
"node": {
"title": "Ether 2:20",
"text": "And the Lord said unto the brother of Jared: Behold, thou shalt make a hole in the top, and also in the bottom; and when thou shalt suffer for air thou shalt unstop the hole and receive air. And if it be so that the water come in upon thee, behold, ye shall stop the hole, that ye may not perish in the flood."
}
},
{
"node": {
"title": "Ether 2:21",
"text": "And it came to pass that the brother of Jared did so, according as the Lord had commanded."
}
},
{
"node": {
"title": "Ether 2:23",
"text": "And the Lord said unto the brother of Jared: What will ye that I should do that ye may have light in your vessels? For behold, ye cannot have windows, for they will be dashed in pieces; neither shall ye take fire with you, for ye shall not go by the light of fire."
}
},
{
"node": {
"title": "Ether 3:1",
"text": "And it came to pass that the brother of Jared, (now the number of the vessels which had been prepared was eight) went forth unto the mount, which they called the mount Shelem, because of its exceeding height, and did molten out of a rock sixteen small stones; and they were white and clear, even as transparent glass; and he did carry them in his hands upon the top of the mount, and cried again unto the Lord, saying:"
}
},
{
"node": {
"title": "Ether 3:6",
"text": "And it came to pass that when the brother of Jared had said these words, behold, the Lord stretched forth his hand and touched the stones one by one with his finger. And the veil was taken from off the eyes of the brother of Jared, and he saw the finger of the Lord; and it was as the finger of a man, like unto flesh and blood; and the brother of Jared fell down before the Lord, for he was struck with fear."
}
},
{
"node": {
"title": "Ether 3:7",
"text": "And the Lord saw that the brother of Jared had fallen to the earth; and the Lord said unto him: Arise, why hast thou fallen?"
}
},
{
"node": {
"title": "Ether 3:21",
"text": "And it came to pass that the Lord said unto the brother of Jared: Behold, thou shalt not suffer these things which ye have seen and heard to go forth unto the world, until the time cometh that I shall glorify my name in the flesh; wherefore, ye shall treasure up the things which ye have seen and heard, and show it to no man."
}
},
{
"node": {
"title": "Ether 3:25",
"text": "And when the Lord had said these words, he showed unto the brother of Jared all the inhabitants of the earth which had been, and also all that would be; and he withheld them not from his sight, even unto the ends of the earth."
}
},
{
"node": {
"title": "Ether 4:1",
"text": "And the Lord commanded the brother of Jared to go down out of the mount from the presence of the Lord, and write the things which he had seen; and they were forbidden to come unto the children of men until after that he should be lifted up upon the cross; and for this cause did king Mosiah keep them, that they should not come unto the world until after Christ should show himself unto his people."
}
},
{
"node": {
"title": "Ether 4:4",
"text": "Behold, I have written upon these plates the very things which the brother of Jared saw; and there never were greater things made manifest than those which were made manifest unto the brother of Jared."
}
},
{
"node": {
"title": "Ether 4:7",
"text": "And in that day that they shall exercise faith in me, saith the Lord, even as the brother of Jared did, that they may become sanctified in me, then will I manifest unto them the things which the brother of Jared saw, even to the unfolding unto them all my revelations, saith Jesus Christ, the Son of God, the Father of the heavens and of the earth, and all things that in them are."
}
},
{
"node": {
"title": "Ether 6:1",
"text": "And now I, Moroni, proceed to give the record of Jared and his brother."
}
},
{
"node": {
"title": "Ether 6:2",
"text": "For it came to pass after the Lord had prepared the stones which the brother of Jared had carried up into the mount, the brother of Jared came down out of the mount, and he did put forth the stones into the vessels which were prepared, one in each end thereof; and behold, they did give light unto the vessels."
}
},
{
"node": {
"title": "Ether 6:9",
"text": "And they did sing praises unto the Lord; yea, the brother of Jared did sing praises unto the Lord, and he did thank and praise the Lord all the day long; and when the night came, they did not cease to praise the Lord."
}
},
{
"node": {
"title": "Ether 6:14",
"text": "And Jared had four sons; and they were called Jacom, and Gilgah, and Mahah, and Orihah."
}
},
{
"node": {
"title": "Ether 6:15",
"text": "And the brother of Jared also begat sons and daughters."
}
},
{
"node": {
"title": "Ether 6:16",
"text": "And the friends of Jared and his brother were in number about twenty and two souls; and they also begat sons and daughters before they came to the promised land; and therefore they began to be many."
}
},
{
"node": {
"title": "Ether 6:19",
"text": "And the brother of Jared began to be old, and saw that he must soon go down to the grave; wherefore he said unto Jared: Let us gather together our people that we may number them, that we may know of them what they will desire of us before we go down to our graves."
}
},
{
"node": {
"title": "Ether 6:20",
"text": "And accordingly the people were gathered together. Now the number of the sons and the daughters of the brother of Jared were twenty and two souls; and the number of sons and daughters of Jared were twelve, he having four sons."
}
},
{
"node": {
"title": "Ether 6:23",
"text": "And now behold, this was grievous unto them. And the brother of Jared said unto them: Surely this thing leadeth into captivity."
}
},
{
"node": {
"title": "Ether 6:24",
"text": "But Jared said unto his brother: Suffer them that they may have a king. And therefore he said unto them: Choose ye out from among our sons a king, even whom ye will."
}
},
{
"node": {
"title": "Ether 6:25",
"text": "And it came to pass that they chose even the firstborn of the brother of Jared; and his name was Pagag. And it came to pass that he refused and would not be their king. And the people would that his father should constrain him, but his father would not; and he commanded them that they should constrain no man to be their king."
}
},
{
"node": {
"title": "Ether 6:27",
"text": "And it came to pass that neither would the sons of Jared, even all save it were one; and Orihah was anointed to be king over the people."
}
},
{
"node": {
"title": "Ether 6:29",
"text": "And it came to pass that Jared died, and his brother also."
}
},
{
"node": {
"title": "Ether 7:5",
"text": "And when he had gathered together an army he came up unto the land of Moron where the king dwelt, and took him captive, which brought to pass the saying of the brother of Jared that they would be brought into captivity."
}
},
{
"node": {
"title": "Ether 8:1",
"text": "And it came to pass that he begat Omer, and Omer reigned in his stead. And Omer begat Jared; and Jared begat sons and daughters."
}
},
{
"node": {
"title": "Ether 8:2",
"text": "And Jared rebelled against his father, and came and dwelt in the land of Heth. And it came to pass that he did flatter many people, because of his cunning words, until he had gained the half of the kingdom."
}
},
{
"node": {
"title": "Ether 8:5",
"text": "And they were exceedingly angry because of the doings of Jared their brother, insomuch that they did raise an army and gave battle unto Jared. And it came to pass that they did give battle unto him by night."
}
},
{
"node": {
"title": "Ether 8:6",
"text": "And it came to pass that when they had slain the army of Jared they were about to slay him also; and he plead with them that they would not slay him, and he would give up the kingdom unto his father. And it came to pass that they did grant unto him his life."
}
},
{
"node": {
"title": "Ether 8:7",
"text": "And now Jared became exceedingly sorrowful because of the loss of the kingdom, for he had set his heart upon the kingdom and upon the glory of the world."
}
},
{
"node": {
"title": "Ether 8:8",
"text": "Now the daughter of Jared being exceedingly expert, and seeing the sorrows of her father, thought to devise a plan whereby she could redeem the kingdom unto her father."
}
},
{
"node": {
"title": "Ether 8:9",
"text": "Now the daughter of Jared was exceedingly fair. And it came to pass that she did talk with her father, and said unto him: Whereby hath my father so much sorrow? Hath he not read the record which our fathers brought across the great deep? Behold, is there not an account concerning them of old, that they by their secret plans did obtain kingdoms and great glory?"
}
},
{
"node": {
"title": "Ether 8:11",
"text": "And now Omer was a friend to Akish; wherefore, when Jared had sent for Akish, the daughter of Jared danced before him that she pleased him, insomuch that he desired her to wife. And it came to pass that he said unto Jared: Give her unto me to wife."
}
},
{
"node": {
"title": "Ether 8:12",
"text": "And Jared said unto him: I will give her unto you, if ye will bring unto me the head of my father, the king."
}
},
{
"node": {
"title": "Ether 8:13",
"text": "And it came to pass that Akish gathered in unto the house of Jared all his kinsfolk, and said unto them: Will ye swear unto me that ye will be faithful unto me in the thing which I shall desire of you?"
}
},
{
"node": {
"title": "Ether 8:17",
"text": "And it was the daughter of Jared who put it into his heart to search up these things of old; and Jared put it into the heart of Akish; wherefore, Akish administered it unto his kindred and friends, leading them away by fair promises to do whatsoever thing he desired."
}
},
{
"node": {
"title": "Ether 9:3",
"text": "And the Lord warned Omer in a dream that he should depart out of the land; wherefore Omer departed out of the land with his family, and traveled many days, and came over and passed by the hill of Shim, and came over by the place where the Nephites were destroyed, and from thence eastward, and came to a place which was called Ablom, by the seashore, and there he pitched his tent, and also his sons and his daughters, and all his household, save it were Jared and his family."
}
},
{
"node": {
"title": "Ether 9:4",
"text": "And it came to pass that Jared was anointed king over the people, by the hand of wickedness; and he gave unto Akish his daughter to wife."
}
},
{
"node": {
"title": "Ether 9:6",
"text": "For so great had been the spreading of this wicked and secret society that it had corrupted the hearts of all the people; therefore Jared was murdered upon his throne, and Akish reigned in his stead."
}
},
{
"node": {
"title": "Ether 10:2",
"text": "And it came to pass that Shez did remember the destruction of his fathers, and he did build up a righteous kingdom; for he remembered what the Lord had done in bringing Jared and his brother across the deep; and he did walk in the ways of the Lord; and he begat sons and daughters."
}
},
{
"node": {
"title": "Ether 11:17",
"text": "And it came to pass that there arose another mighty man; and he was a descendant of the brother of Jared."
}
},
{
"node": {
"title": "Ether 12:20",
"text": "And behold, we have seen in this record that one of these was the brother of Jared; for so great was his faith in God, that when God put forth his finger he could not hide it from the sight of the brother of Jared, because of his word which he had spoken unto him, which word he had obtained by faith."
}
},
{
"node": {
"title": "Ether 12:21",
"text": "And after the brother of Jared had beheld the finger of the Lord, because of the promise which the brother of Jared had obtained by faith, the Lord could not withhold anything from his sight; wherefore he showed him all things, for he could no longer be kept without the veil."
}
},
{
"node": {
"title": "Ether 12:24",
"text": "And thou hast made us that we could write but little, because of the awkwardness of our hands. Behold, thou hast not made us mighty in writing like unto the brother of Jared, for thou madest him that the things which he wrote were mighty even as thou art, unto the overpowering of man to read them."
}
},
{
"node": {
"title": "Ether 12:30",
"text": "For the brother of Jared said unto the mountain Zerin, Remove--and it was removed. And if he had not had faith it would not have moved; wherefore thou workest after men have faith."
}
},
{
"node": {
"title": "Moroni 1:1",
"text": "Now I, Moroni, after having made an end of abridging the account of the people of Jared, I had supposed not to have written more, but I have not as yet perished; and I make not myself known to the Lamanites lest they should destroy me."
}
},
{
"node": {
"title": "Doctrine and Covenants 17:1",
"text": "BEHOLD, I say unto you, that you must rely upon my word, which if you do with full purpose of heart, you shall have a view of the plates, and also of the breastplate, the sword of Laban, the Urim and Thummim, which were given to the brother of Jared upon the mount, when he talked with the Lord face to face, and the miraculous directors which were given to Lehi while in the wilderness, on the borders of the Red Sea."
}
},
{
"node": {
"title": "Doctrine and Covenants 52:38",
"text": "And again, verily I say unto you, let Jared Carter be ordained a priest, and also George James be ordained a priest."
}
},
{
"node": {
"title": "Doctrine and Covenants 79:1",
"text": "VERILY I say unto you, that it is my will that my servant Jared Carter should go again into the eastern countries, from place to place, and from city to city, in the power of the ordination wherewith he has been ordained, proclaiming glad tidings of great joy, even the everlasting gospel."
}
},
{
"node": {
"title": "Doctrine and Covenants 79:4",
"text": "Wherefore, let your heart be glad, my servant Jared Carter, and fear not, saith your Lord, even Jesus Christ. Amen."
}
},
{
"node": {
"title": "Doctrine and Covenants 94:14",
"text": "And on the first and second lots on the north shall my servants Reynolds Cahoon and Jared Carter receive their inheritances--"
}
},
{
"node": {
"title": "Doctrine and Covenants 102:3",
"text": "Joseph Smith, Jun., Sidney Rigdon and Frederick G. Williams were acknowledged presidents by the voice of the council; and Joseph Smith, Sen., John Smith, Joseph Coe, John Johnson, Martin Harris, John S. Carter, Jared Carter, Oliver Cowdery, Samuel H. Smith, Orson Hyde, Sylvester Smith, and Luke Johnson, high priests, were chosen to be a standing council for the church, by the unanimous voice of the council."
}
},
{
"node": {
"title": "Doctrine and Covenants 102:34",
"text": "The twelve councilors then proceeded to cast lots or ballot, to ascertain who should speak first, and the following was the result, namely: 1, Oliver Cowdery; 2, Joseph Coe; 3, Samuel H. Smith; 4, Luke Johnson; 5, John S. Carter; 6, Sylvester Smith; 7, John Johnson; 8, Orson Hyde; 9, Jared Carter; 10, Joseph Smith, Sen.; 11, John Smith; 12, Martin Harris."
}
},
{
"node": {
"title": "Doctrine and Covenants 107:47",
"text": "Jared was two hundred years old when he was ordained under the hand of Adam, who also blessed him."
}
},
{
"node": {
"title": "Doctrine and Covenants 107:53",
"text": "Three years previous to the death of Adam, he called Seth, Enos, Cainan, Mahalaleel, Jared, Enoch, and Methuselah, who were all high priests, with the residue of his posterity who were righteous, into the valley of Adam-ondi-Ahman, and there bestowed upon them his last blessing."
}
},
{
"node": {
"title": "Moses 6:20",
"text": "And Mahalaleel lived sixty-five years, and begat Jared; and Mahalaleel lived, after he begat Jared, eight hundred and thirty years, and begat sons and daughters. And all the days of Mahalaleel were eight hundred and ninety-five years, and he died."
}
},
{
"node": {
"title": "Moses 6:21",
"text": "And Jared lived one hundred and sixty-two years, and begat Enoch; and Jared lived, after he begat Enoch, eight hundred years, and begat sons and daughters. And Jared taught Enoch in all the ways of God."
}
},
{
"node": {
"title": "Moses 6:24",
"text": "And it came to pass that all the days of Jared were nine hundred and sixty-two years, and he died."
}
}
]
}
}
}
results
{
"input": {
"keywords": "Jared"
}
}
input
query VerseSearch($input:VerseSearchInput) {
getBook(id:"80") {
title
versesConnection(input:$input, first:2) {
pageInfo {
total
}
edges {
node {
title
text
}
}
}
}
}
query
{
"data": {
"getBook": {
"title": "Ether",
"versesConnection": {
"pageInfo": {
"total": 63
},
"edges": [
{
"node": {
"title": "Ether 1:32",
"text": "And Kib was the son of Orihah, who was the son of Jared;"
}
},
{
"node": {
"title": "Ether 1:33",
"text": "Which Jared came forth with his brother and their families, with some others and their families, from the great tower, at the time the Lord confounded the language of the people, and swore in his wrath that they should be scattered upon all the face of the earth; and according to the word of the Lord the people were scattered."
}
}
]
}
}
}
}
results
{
"input": {
"keywords": "Jared"
}
}
input
Features & Benefits
more
1 endpoint
inversion of control
clients know best what they need and when
get exactly what you need
- no under-fetching
- no over-fetching
including multiple resources on a single request
via type system
- no ambiguity on API capability
- types
- fields
- queries
- mutations
- strong, predictable contract
- app and api devs can work in parallel
Server Describes Capability
flexibility for client to change without disrupting server
Powerful Tooling
- know exactly what data you can request from your API without leaving your editor
- highlight potential issues before sending a query
- take advantage of improved code intelligence
- explore via GraphiQL
Evolve without Versions
because clients gets only what they ask for, new fields can be added without bloating or breaking current clients
Language & Database Agnostic
C# / .NET
Clojure
Elixir
Erlang
Go
Groovy
Java
JavaScript
PHP
Python
Scala
Ruby
Server Libs
Client Libs
C# / .NET
Go
Java / Android
JavaScript
Swift / Objective-C iOS
Databases
SQL
NOSQL
Graph
or no database
Challenges & Awkwardness
"Up-and-Coming"
Batching & Caching
Recursive Types
GraphiQL Demo
Shoutout:
- https://dev-blog.apollodata.com/how-do-i-graphql-2fcabfc94a01
- https://dev-blog.apollodata.com/the-concepts-of-graphql-bc68bd819be3
- http://graphql.org/learn/thinking-in-graphs/
- http://graphql.org/
Reference
Intro to GraphQL
By Jared Anderson
Intro to GraphQL
- 1,300