
The Founder

- Previously worked as a Creative Technologist at Google
- Core Dev at Meteor
- From 2016 working full-time on the Vue.JS framework
Apr. 2015: this happened
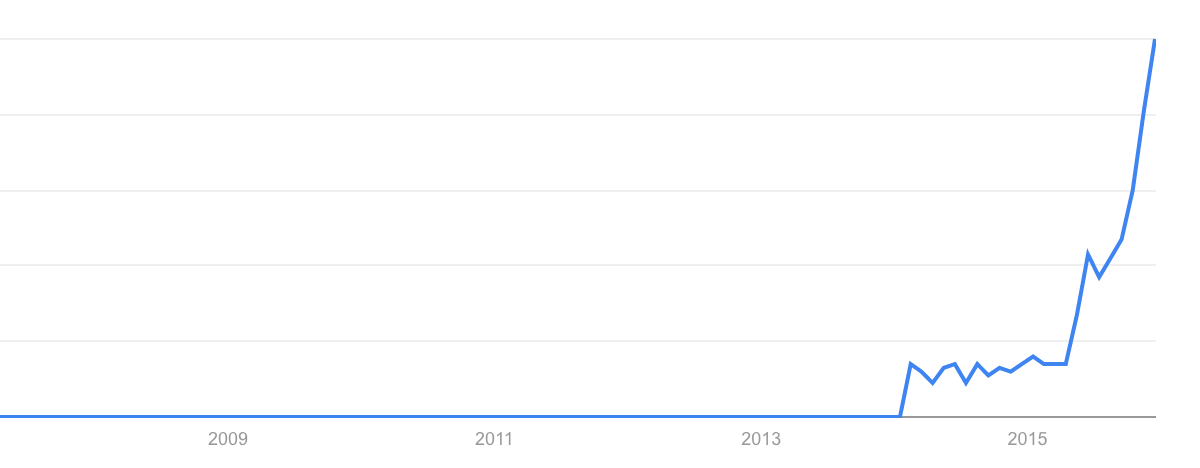

Is the holy place between Angular and React
Why so much hype?
- Simple API
- Easy to get started with
- Combined with
vue -router can create nice SPA's
Plus the Laravel Community
Today (on Mar 7 2018)


vs. React
vs. Angular

What does the syntax looks like?
<div id="app">
<p>{{ message }}</p>
</div>
<script src="https://unpkg.com/vue"></script>
var app = new Vue({
el: '#app',
data: {
message: 'Hello Vue!'
}
})
Declarative Rendering
Declarative Rendering
if( user.likes() ) {
if( hasBlue() ) {
removeBlue();
addGrey();
} else {
removeGrey();
addBlue();
}
}
Button
"LIKE"
<div v-if="user.liked">
<blue-like></blue-like>
</div>
<div v-else>
<grey-like></grey-like>
</div>
Imperative
Declarative
Conditionals
<div id="app">
<span v-if="seen">Now you see me</span>
</div>
<script type="text/javascript">
var app = new Vue({
el: '#app',
data: {
seen: true
}
})
</script>
Loops
<div id="app">
<ol>
<li v-for="todo in todos">
{{ todo.text }}
</li>
</ol>
</div>
<script type="text/javascript">
var app = new Vue({
el: '#app',
data: {
todos: [
{ text: 'Learn JavaScript' },
{ text: 'Learn Vue' },
{ text: 'Build something awesome' }
]
}
})
</script>
Handling User Input
<div id="app">
<p>{{ message }}</p>
<button v-on:click="reverseMessage">Reverse Message</button>
</div>
<script type="text/javascript">
var app = new Vue({
el: '#app',
data: {
message: 'Hello Vue.js!'
},
methods: {
reverseMessage: function () {
this.message = this.message.split('').reverse().join('')
}
}
})
</script>
Handling User Input
<div id="app">
<p>{{ message }}</p>
<input v-model="message">
</div>
<script type="text/javascript">
var app = new Vue({
el: '#app',
data: {
message: 'Hello Vue!'
}
})
</script>
Composing with Components

Each component is a little Vue instance
Vue.component('todo-item', {
props: ['todo'],
template: '<li>{{ todo.text }}</li>'
})
Composing with Components
Vue.component('todo-item', {
props: ['todo'],
template: '<li>{{ todo.text }}</li>'
})
var app = new Vue({
el: '#app',
data: {
groceryList: [
{ id: 0, text: 'Vegetables' },
{ id: 1, text: 'Cheese' },
{ id: 2, text: 'Whatever else humans are supposed to eat' }
]
}
})
<ol>
<todo-item v-for="item in groceryList" v-bind:todo="item">
</todo-item>
</ol>
Build in directives
-
v-text (Updates the element’s textContent)
-
v-html (Updates the element’s innerHTML
-
v-if (Conditionally render the element)
-
v-show (Toggle’s the element’s display CSS property)
-
v-else (Denote the “else block” for v-if and v-show
-
v-for (Loop throuth an array of object)
Build in directives
-
v-on (Used for events DOM or custom)
-
v-bind (Bind html attribute)
-
v-model (Create a two-way binding on a form input element)
-
v-ref (Create ref to template element)
-
v-el (Create reference to DOM element)
-
v-pre (Dont evaluate contents)
-
v-cloak (hide element until fully parsed)
Instance Lifecycle Hooks

new Vue({
data: {
a: 1
},
created: function () {
console.log('a is: ' + this.a)
}
})
Computed Properties
<div id="example">
{{ message.split('').reverse().join('') }}
</div>
div id="example">
<p>Original message: "{{ message }}"</p>
<p>Computed reversed message: "{{ reversedMessage }}"</p>
</div>
var vm = new Vue({
el: '#example',
data: {
message: 'Hello'
},
computed: {
// a computed getter
reversedMessage: function () {
// `this` points to the vm instance
return this.message.split('').reverse().join('')
}
}
})
How is it different from...
jQuery? 🤔
Angular? (simpler, faster, less opinionated, more flexible)
React? (faster, simpler tooling and prefers HTML/CSS over Javascript for templates)
What should I choose?
-
If you work at Google: Angular
-
If you love TypeScript: Angular (or React)
-
If you love object-orientated-programming (OOP): Angular
-
If you need guidance, structure and a helping hand: Angular
-
If you work at Facebook: React
-
If you like flexibility: React
-
If you love big ecosystems: React
-
If you like choosing among dozens of packages: React
What should I choose?
-
If you love JS & the “everything-is-Javascript-approach”: React
-
If you like really clean code: Vue
-
If you want the easiest learning curve: Vue
-
If you want the most lightweight framework: Vue
-
If you want separation of concerns in one file: Vue
-
If you are working alone or have a small team: Vue (or React)
What should I choose?
-
If your app tends to get really large: Angular (or React)
-
If you want to build an app with react-native: React
-
If you want to have a lot of developers in the pool: Angular or React
THANKS
Vue Basics
By Amit Gupta
Vue Basics
- 624