Introduction to ECMAScript 6

Amit Gupta
Software Engineer, SquareBoat
SquareBoat builds awesome mobile and web apps for startups

P.S. We're hiring!
squareboat.com
Agenda
-
Status
-
let Variables and Constants
-
Destructuring
-
Arrow Functions
-
Template literals
-
Modules
-
Classes
-
Generators
-
Promises
-
Live Coding Session

Before we start..
a small quiz...


Terminology
-
ECMAScript: a language standardized by ECMA International
-
JavaScript: the commonly used name for implementations of the ECMAScript standard
-
TC 39: the group of people who develop the ECMA-262 standard
-
ECMAScript 6: the 6th edition of ECMA-262, the current edition of this standard

What is ECMAScript?
Let's go back in time..


History
- ECMAScript 1: 1997
- ECMAScript 2: 1998
- ECMAScript 3: 1999
- ECMAScript 4: Abandoned
- ECMAScript 5: 2009
- ECMAScript 6: 2015

Goals for ES6
- Complex Apps
- Libraries
- Code Generators
Better support for:

Constants and Variables


Function-Scoped
var links = [];
for(var i=0; i<5; i++) {
links.push({
onclick: function() {
console.log('link: ', i);
}
});
}
links[0].onclick();
links[1].onclick();
Block-Scoped
var links = [];
for(let i=0; i<5; i++) {
links.push({
onclick: function() {
console.log('link: ', i);
}
});
}
links[0].onclick(); // link : 0
links[1].onclick(); // link : 1
var
let
// link : 5
// link : 5
ECMAScript 5
ECMAScript 6
Variables

Constants and Variables
let
function test() {
let foo = 1;
if (foo === 1) {
let foo = 22;
console.log(foo); // 22
}
if (foo === 22) { // will not execute
let foo = 33;
console.log(foo);
}
console.log(foo); // 1
}
test();

Constants and Variables
const
Block-Scoped
-
only be defined once (within their scope)
-
cannot change the value once set
const msg = 'hello world';
var msg = 123; // Syntax error: msg already defined.
msg = 123; // will silently fail, msg not changed

Constants and Variables
Destructuring

Ability to extract values from objects or arrays into variables

Creating Variables from Object Properties
ECMAScript 5
var meetup = {
group: 'jslovers',
title: 'ECMA6',
venue: 'Cool Space'
};
var group = meetup.group;
var title = meetup.title;
var venue = meetup.venue;
console.log(group); // jslovers
console.log(title); // ECMA6
console.log(venue); // Cool Space
var meetup = {
group: 'jslovers',
title: 'ECMA6',
venue: 'Cool Space'
};
let {group,title,venue} = meetup;
console.log(group); // jslovers
console.log(title); // ECMA6
console.log(venue); // Cool Space
ECMAScript 6

Destructuring
var meetup = {
group: 'jslovers',
title: 'ECMA6',
venue: 'Cool Space'
};
let {group: _group, title: _title, venue: _venue} = meetup;
console.log(_group); // jslovers
console.log(_title); // ECMA6
console.log(_venue); // Cool Space
Can also change the name of the local variable

Destructuring
Creating Variables from Arrays Elements
ECMAScript 5
let brews = [
"Corona",
"Foster’s",
"Budweiser"
];
let x = brews[0];
let y = brews[1];
let z = brews[2];
console.log(x); // Corona
console.log(y); // Foster’s
console.log(z); // Budweiser
let brews = [
"Corona",
"Foster’s",
"Budweiser"
];
let [x, y, z] = brews;
console.log(x); // Corona
console.log(y); // Foster’s
console.log(z); // Budweiser
ECMAScript 6

Destructuring
Functions with Multiple Return Values
function getDate() {
let date = new Date();
return [date.getDate(), date.getMonth() + 1, date.getFullYear()];
}
let [day, month, year] = getDate();
console.log(day); // 5
console.log(month); // 2
console.log(year); // 2017

Destructuring
Swapping values
ECMAScript 5
let a = 1;
let b = 2;
let temp;
temp = a;
a = b;
b = temp;
console.log(a); // 2
console.log(b); // 1
let a = 1;
let b = 2;
[b, a] = [a, b]
console.log(a); // 2
console.log(b); // 1
ECMAScript 6

Destructuring
Spread operator
function addStrings(a, b) {
return a + " " + b;
}
let vals = ['Go', 'Vegan'];
addStrings(vals[0], vals[1])
// returns "Go Vegan"
function addStrings(a, b) {
return a + " " + b;
}
let vals = ['Go', 'Vegan'];
addStrings(...vals)
// returns "Go Vegan"

ECMAScript 5
ECMAScript 6
Destructuring
Arrow Functions

-
a shorthand way of declaring functions
-
shares the scope with the parent

Functions
ECMAScript 5
var addAndLog = function(a, b) {
let c = a + b;
console.log(a, '+' ,b ,'=' ,c);
return c;
};
addAndLog(1, 2);
// 1 + 2 = 3 (returns 3)
let addAndLog = (a, b) => {
let c = a + b;
console.log(a,'+',b,'=',c);
return c;
};
addAndLog(1, 2);
// 1 + 2 = 3 (returns 3)
ECMAScript 6

Arrow Functions
Fat Arrow Functions in ECMAScript 6
let add = (a, b) => a + b;
add(1,2); // 3
let addOne = a => a + 1;
addOne(1); // 2
let pi = () => 22/7;
pi(); // 3.142857142857143

Arrow Functions
Fat Arrow Functions Shares the Scope of Parent
ECMAScript 5
function Person() {
this.age = 23;
setTimeout(function() {
console.log(this.age);
}, 1000);
}
var p = new Person();
function Person() {
this.age = 23;
setTimeout(() => {
console.log(this.age); // 23
}, 1000);
}
var p = new Person();
ECMAScript 6
// undefined

var that = this;
setTimeout(() => {
console.log(that.age); // 23
}, 1000);
Arrow Functions
Default Parameters
ECMAScript 5
function add(a, b) {
b = typeof b !== 'undefined' ? b : 1;
return a + b;
}
add(1); // returns 2
function add(a, b = 1) {
return a + b;
}
add(1); // returns 2
ECMAScript 6
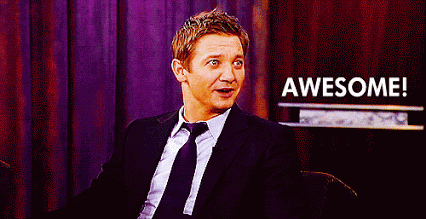

Arrow Functions
Template String Literals

-
back tick character (`) is used to build multi-line strings
-
${} syntax to reference variables and expressions

String Literals
let phrase = `If nothing goes right, go to sleep.`;
console.log(phrase);
let multiLine = `I can't hear you,
so I'll just laugh and hope it wasn't a question.`;
console.log(multiLine);
let back = `Who the hell uses \` in a sentence?`;
console.log(back);

Template String Literals
String Substitution
let name = 'jslovers';
console.log(`Hello ${name}`); // Hello jslovers
var meetup = {
group: 'jslovers',
title: 'ECMA6',
venue: 'Cool Space'
};
console.log(`We are ${meetup.group},
learning ${meetup.title} at a ${meetup.venue}`);
// We are jslovers,
// learning ECMA6 at a Cool Space

Template String Literals
Expressions
console.log(`Today is ${new Date()}`);
// Today is Sun Feb 05 2017 10:10:10 GMT+0530 (IST)
console.log(`Value of pi is ${22/7}`);
// Value of pi is 3.142857142857143

Template String Literals
Generators


-
Functions that can be paused and resumed
-
Declared using the * next to the normal method
-
Special keyword yield is used to pause the execution
-
Follows the iterable pattern
What is a Generator?

Generators
What is a Generator?
function* generator() {
console.log('First');
yield 'Second';
console.log('Third');
yield 'Fourth';
}
let gen = generator();
console.log(gen.next().value);
// First
// Second
console.log(gen.next().value);
// Third
// Fourth
console.log(gen.next().value);
// undefined

Generators
Use Case
function* objectEntries(obj) {
const propKeys = Reflect.ownKeys(obj);
// returns an array of the object's own property keys
for (const propKey of propKeys) {
yield [propKey, obj[propKey]];
}
}

const batman = { first: 'Bruce', last: 'Wayne' };
for (const [key, value] of objectEntries(batman)) {
console.log(`My ${key} name is ${value}`);
}
// My first name is Bruce
// My last name is Wayne
Generators
Classes

-
Syntactic sugar on top of concepts that already exists
-
Defined using the new class keyword

Classes

ECMAScript 5
function Point(x, y) {
this.x = x;
this.y = y;
this.toString = function() {
return '('+this.x+','+this.y+')';
};
}
var point = new Point(1, 3);
console.log(point.toString());
// (1, 3)
class Point {
constructor(x, y) {
this.x = x;
this.y = y;
}
toString() {
return `(${this.x}, ${this.y})`;
}
}
let point = new Point(1, 3);
console.log(point.toString());
// (1, 3)
ECMAScript 6
Classes
Inheritance
class ColorPoint extends Point {
constructor(x, y, color) {
super(x, y);
this.color = color;
}
toString() {
return super.toString() + ' in ' + this.color;
}
}
let colorPoint = new ColorPoint(10, 15, 'green');
console.log(colorPoint.toString()); // (10, 15) in green

Classes
Promises


Proxy for value that's not yet available
-
Represents the result of an asynchronous operation
-
Caller attaches event handler to returned promise
-
Executes a method and wait for the result to be processed without locking up the rest of the application

Promises
var promise = new Promise( function (resolve, reject) {
// some code (synchronous or asynchronous)
// ...
if (/* the expected result/behavior is obtained */) {
return resolve( params);
} else {
return reject( err);
}
});
think of then(handler) as addEventListener("done", handler)

promise
.then( function (params) {
//the promise was fulfilled
console.log(params);
})
.catch( function (err) {
// the promise was rejected
console.log( err);
});
Promises
let wait = function(delay = 0) {
return new Promise((resolve, reject) => {
setTimeout(() => {
console.log('Waiting is done');
resolve();
}, delay);
});
}
ECMAScript 6 Promises

wait(3000)
.then(() => {
console.log('3 seconds have passed!');
});
console.log('I am executed');
// I am executed
// Waiting is done
// 3 seconds have passed!
Promises
Modules


-
Modules are just a way of packaging related JavaScript code in its own scope
-
It is consumed by other JavaScript programs.
-
It is represented by a file
Modules

Modules
// utility.js
export function generateRandom() {
return Math.random();
}
export function sum(a, b) {
return a + b;
}
// app.js
import { generateRandom, sum } from 'utility';
// or
import * from 'utility';
console.log(generateRandom()); // logs a random number
console.log(sum(1, 2)); // 3

Modules
Backward Compatibility
- Compatibility tables: https://kangax.github.io/compat-table/es6
- Follow @esdiscuss
- caniuse.com
When can I use what?

How to Use ES6 Today?
- Babel http://babeljs.io
- Traceur https://github.com/google/traceur-compiler
- TypeScript (Superset of JavaScript that aims to align with ECMAScript) http://www.typescriptlang.org
Transpilers

How to Use ES6 Today?
- Webpack http://webpack.github.io
- Browserify http://browserify.org
- Rollup http://rollupjs.org
Module Bundlers

Live Coding Session



Now go and write some next-gen JavaScript!
Thanks
Any queries? Open to Q&A

@akaamitgupta
akaamitgupta@gmail.com
Introduction to ECMAScript 6
By Amit Gupta
Introduction to ECMAScript 6
- 1,795