Introduction to JavaScript
Text
What is JavaScript?
"JavaScript is a high level, dynamic, untyped, and interpreted programming language."
Wikipedia -
What is JavaScript?
- Developed by Brendan Eich.
- A programming language used by most, if not all, organizations when it comes to web development.
- Runs on all browsers.
- JS is the de-facto lingua-franca for front end web.
Things you can do.
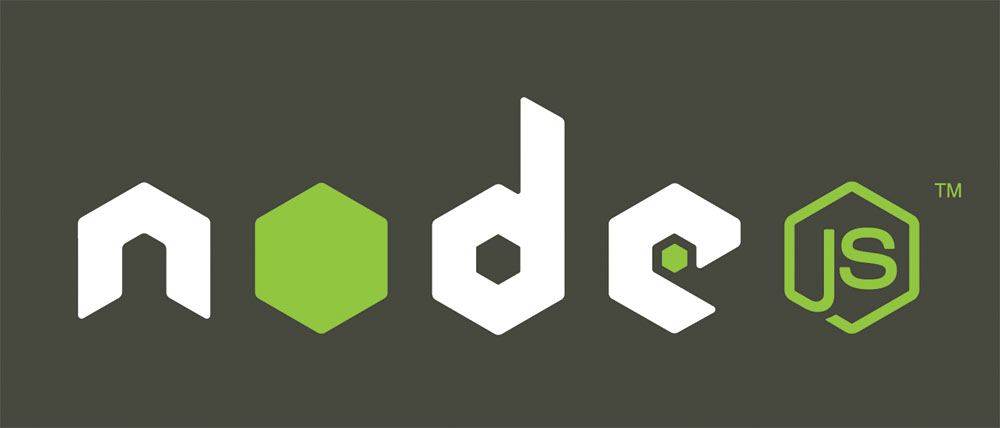
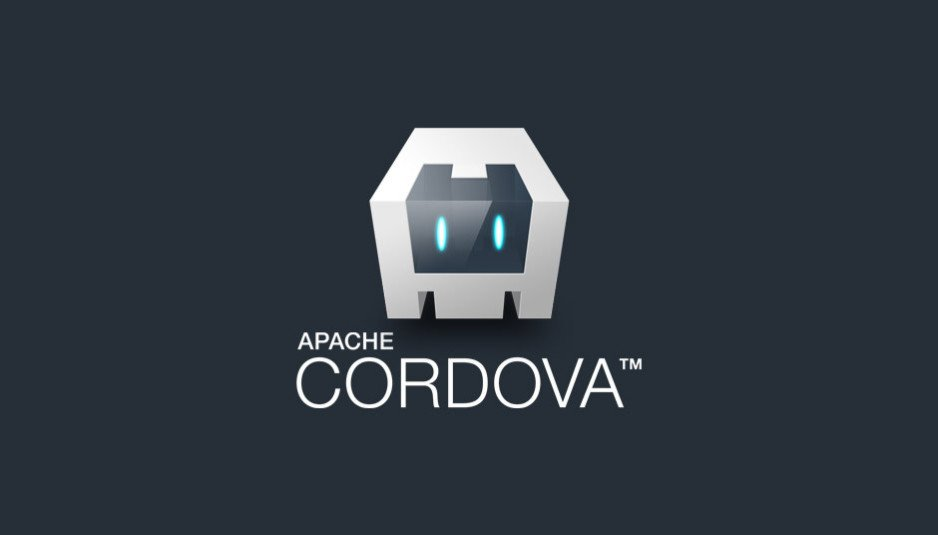
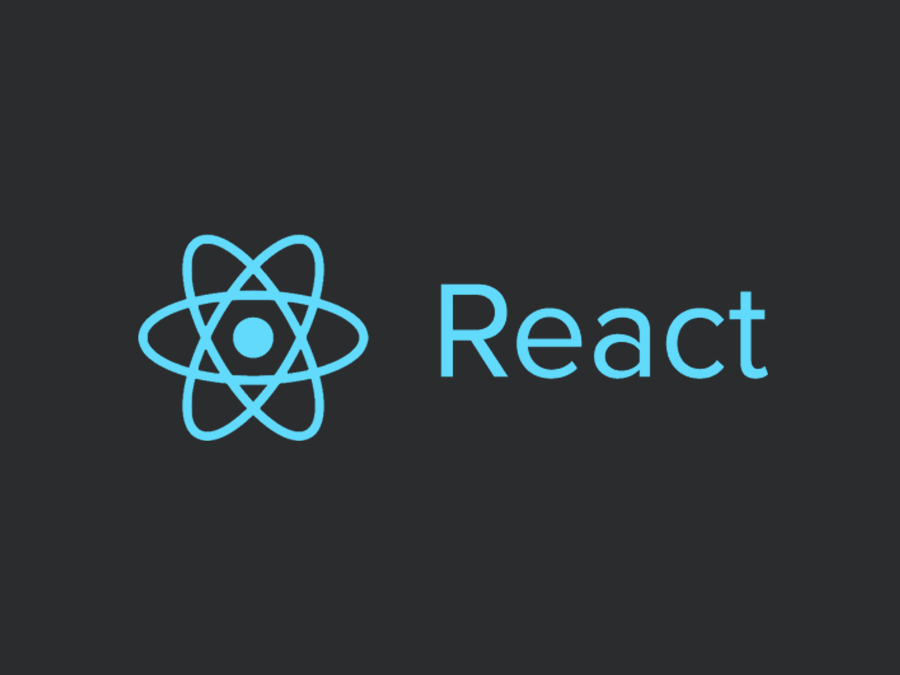
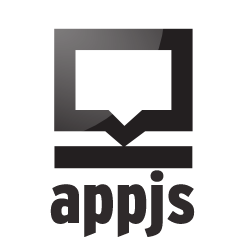
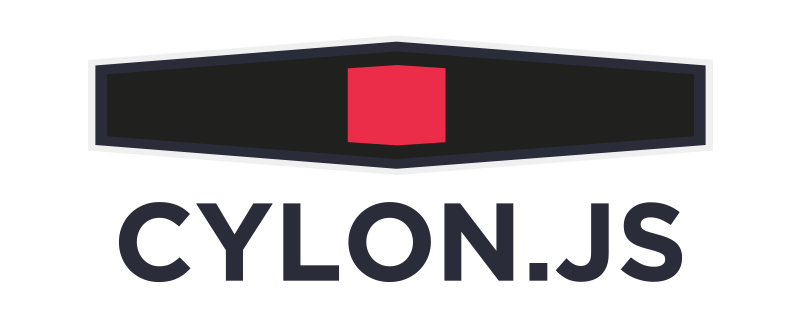
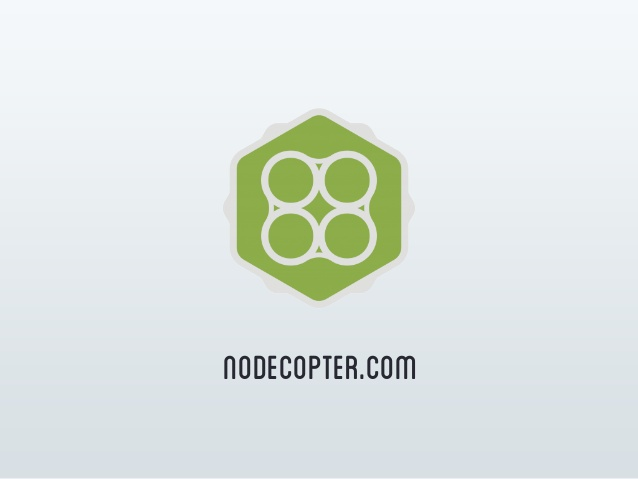
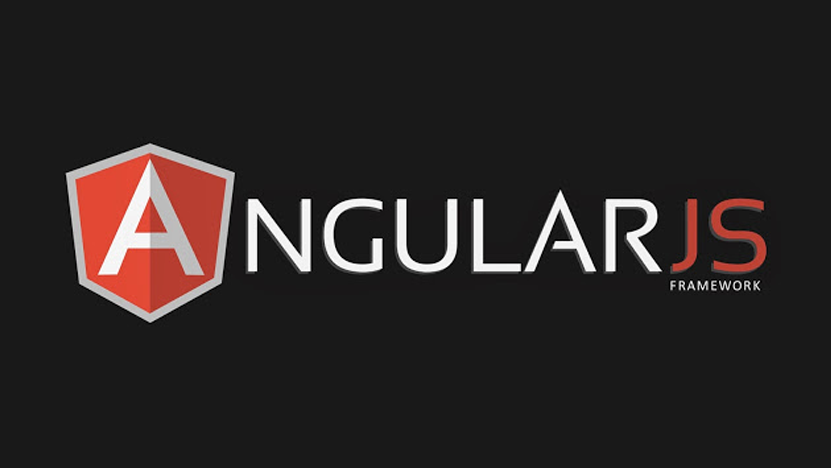
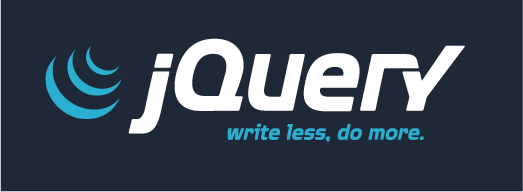
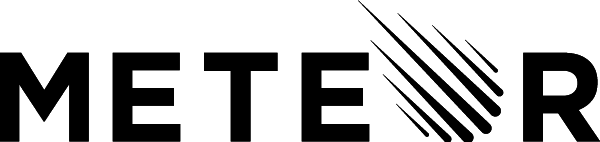
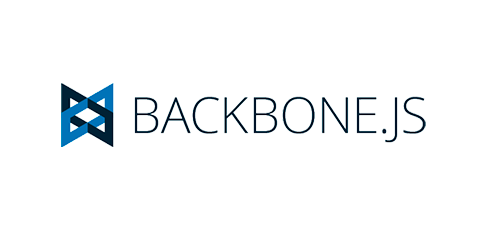
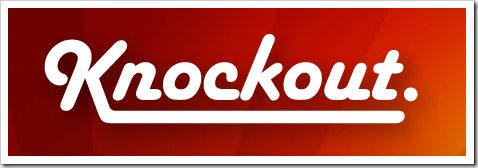
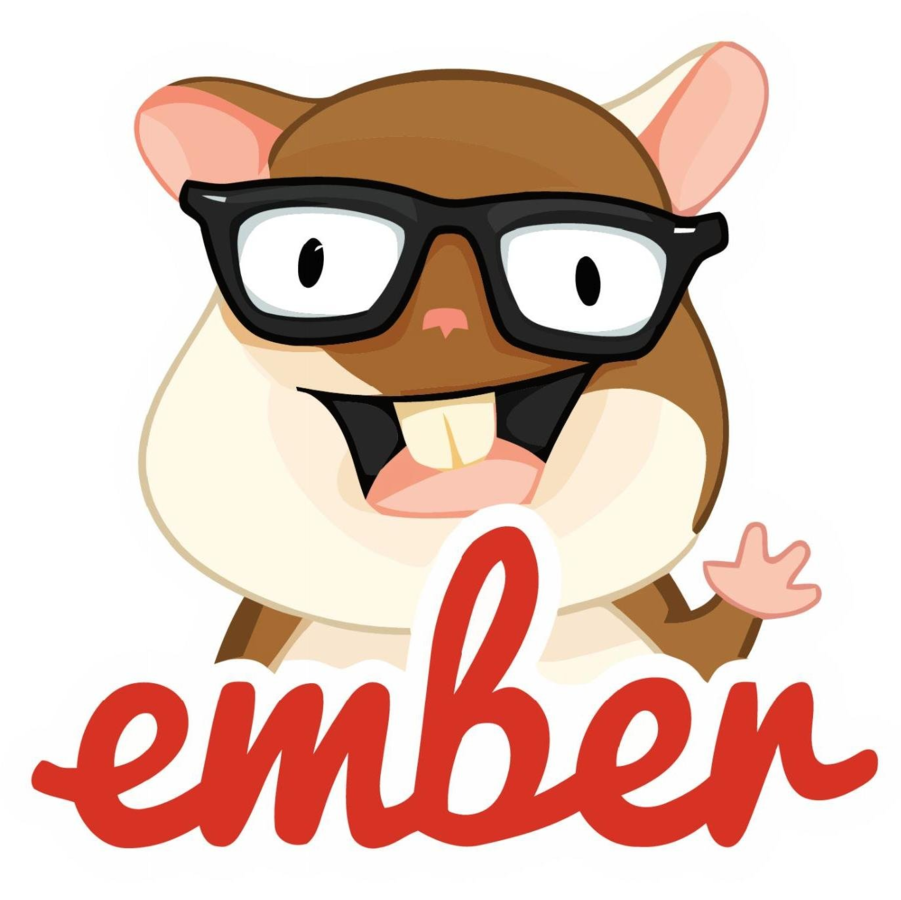
Learning Objectives
- Arithmetic
- Variables
- Methods
- Strings
- Functions
- **Arrays**
- **Operators**
What to Expect
- You will have rudimentary knowledge of JavaScript basics.
- This will arm you with enough knowledge to take our "intermediate" level JavaScript course.
- You will NOT have enough knowledge to learn a web framework.
- You will NOT have enough knowledge to make a dynamic web page.
Arithmetic
> 1 + 2;
3
//Try the following:
> 4 - 3;
> 5 * 6;
> 10 / 2;
> 9 / 2;
> 9 % 2;
> 7 + 8 * 9;
> (7 + 8) * 9;
> 0/0;
> 0/4;
Variables
> var someNumber = 42;
- someNumber is a variable we set to 42.
- Use var keyword whenever you want to create a new variable.
- In JavaScript, it's conventional to give variable names that start with lowercase letter, followed by capitalizing the first letter of subsequent words. This is called camelcase.
Variables Practice
//For reference
> var num1 = 42; //assigns 42 to num1
> var num2 = 12; //assigns 12 to num2
> var num3 = num1 + num2; //adds num1 and num2 and assigns it to num3.
> var num4 = num3; //assigns num4 the value of num3.
> num1 = 21; //num1's new value is 21.
> num1; //This will display the value of num1 (21) to the console.
- Create a variable called someNumber and set it equal to 100.
- Create a variable called favoriteNumber and set it equal to your favorite number.
- Create another variable called avisFavorite and set it to 21.
- Subtract your favorite number from mine and assign it to someNumber.
- Create a variable called finalNumber and set it equal to 25 times someNumber.
- Display someNumber to the console.
- Display finalNumber to the console.
Methods
> 2345.67.toExponential();
"2.34567e+3"
- toExponential() is called a method.
- Think of it as a message you send to the number, and the result that JavaScript gives you as the number's response to that message.
- There are other methods (toFixed, valueOf, toPrecision, etc..) built into JavaScript.
- More here: http://goo.gl/elelI9
Methods Continued
> 1.3368e3.toFixed();
"1337"
toFixed() will round to the nearest whole number
> 1234.5.toFixed(2);
"1234.50"
- The 2 in the parentheses is an argument to the toFixed() method.
- Arguements provide a bit more information to methods to help them know what they're supposed to do.
- In this case, the argument was optional.
Here's how we tell how many decimal places to use:
Methods Continued
> 4.12345.toPrecision(4);
"4.123"
toPrecision() defines how many total digits to display of the number.
> var someNumber = 1.337134
> someNumber.toPrecision(4);
"1.337"
You can also call a method on a variable, since the variable is a placeholder for the number assigned to it.
Method Practice
//For reference
> 456.2356.toFixed(2); //Will display "456.24"
> 123.123.toPrecision(5); // Will display "123.12"
> 135.6236.toExponential(); //Will display "1.356236e+2"
/*The values returned by the methods are strings. I will explain strings (characters inside quotes)
in the next slide. For now, if you stored the string value returned by the methods above into
a variable, you will need to convert that value into a number.
e.g. a = "123"; //a is being assigned a string, not a number.
a = parseFloat(a); //this will convert the string to a number. Now you can call number methods on this.
*/
- Convert the following to exponential notation:
- 4569.234
- Convert it back.
- Have the number set to one decimal place.
- Repeat 1-3 for a variable set equal to any number you choose.
Strings
> "Hello world!";
- The stuff inside the quotes is called a string.
- Strings can include letters, punctuation, and even numbers.
> var myString = "Strings can contain characters like @, $, and %.";
- We can set variables equal to strings.
- We can call methods on strings.
> "supercalifragilisticexpialidocious".toUpperCase();
Strings Continued
> var word = "Biggie";
> word.concat("Smalls");
We can call a method on a variable assigned to a string:
- The concat() method (which concatenates, or combines, two strings) returns a string, which then has toUpperCase() called on it.
- toUpperCase() returns the final result.
> "Tupac".concat("Shakur").toUpperCase();
We can even chain methods:
Strings Continued
> "coding temple".charAt(5);
"g"
String methods can also take numbers as arguments for certain methods:
Computers start counting at zero, hence the reason why "g" was displayed instead of "n".
More about string methods here:
https://goo.gl/UZBEqn
String Practice
- Set a variable equal to a string that is a greeting to your best friend.
- Call a method on that variable.
- Set another variable with a string in all uppercase.
- Call down toLowerCase() on the previous variable.
- Use Concat() to concat the two previous variables.
- Now concatenate them using the shortcut.
- Find the character at the third position in either of the variables. (Hint: counting is off by one)
- Try out a few other methods from: https://goo.gl/UZBEqn
//For Reference
> var ct = "coding temple"; //this will assign "coding temple" to variable ct.
> var hw = "hello world"; //this will assign "hello world" to variable hw.
> ct.toUpperCase(); //this will return "CODING TEMPLE". It will NOT change the value of ct.
> hw.concat(ct); //this will return "coding templehello world". It combines two strings together.
> hw + " " + ct; //this will return "coding temple hello world". It does the same as above, but adds a space between.
Functions
> alert("THE DOW DROPPED MORE THAN 1000 POINTS!");
- alert() is a function.
- A function is something that performs an action.
- Just like a method, a function can take arguments.
> prompt("What is love?");
Try this:
Functions Continued
> var favoriteSong = prompt("What is your favorite song?"); //I'll type "Barbie Girl"
> favoriteSong;
"Barbie Girl"
- The prompt function asks the user for an input.
- The response from the user is returned as a string from the function.
- That response is then assigned to a variable.
Try this:
> confirm("Annie, are you ok? Are you ok, Annie?");
//+1 for those who know the artist.
Functions Practice
- Use alert() to pop up a dialog box with a warning for the user. The warning message can be anything.
- Use confirm() to ask a yes or no question.
- Use prompt() to ask a question.
- save the response to a variable and then run any string method of your choice on it (e.g. toUpperCase())
Writing Functions
> var greeting = function() { alert('Greetings from Coding Temple!'); };
> greeting();
- greeting() pops up a dialog box with text in it.
- Just like how we assigned numbers and strings to variables, we can also assign functions to variables.
- Whenever you call the function. The code within the enclosing brackets gets executed.
Writing Functions Continued
> var giveAlert = function(theMessage) { alert(theMessage); };
> giveAlert("YOUR COMPUTER HAS BEEN INFECTED WITH A VIRUS. DOWNLOAD SECURITY SUI...lol jk.");
- The argument to the function is the long string.
- The argument is assigned to a variable named theMessage - we call that variable a parameter.
- The parameter can then be used just like any other variable.
Writing Functions Continued
> var add = function(num1, num2){ return num1 + num2; };
> add(2, 3);
- The return keyword returns the result from the function. Without it, JavaScript will return undefined.
- We call the add function with arguments 2 and 3.
- The parameters num1 and num2 are set to the values passed in. num1 = 2 and num2 = 3.
- The code within the function is run. In this case, 2 + 3 is run.
- The result is then returned.
Writing Functions Continued
> var add = function(num1, num2){ return num1 + num2; };
> add(2, 3);
- The return keyword returns the result from the function. Without it, JavaScript will return undefined.
- We call the add function with arguments 2 and 3.
- The parameters num1 and num2 are set to the values passed in. num1 = 2 and num2 = 3.
- The code within the function is run. In this case, 2 + 3 is run.
- The result is then returned.
Writing Functions Practice
//For reference
> var add = function(num1, num2){ return num1 + num2; };
> add(2, 3);
- Prompt the user for a name and return a greeting to them. e.g. "Hi Legolas!"
- Return the difference between two numbers
- Return the product of two numbers.
- Return the square of number.
- Return the cube of a number.
Where to go from here?
Codecademy
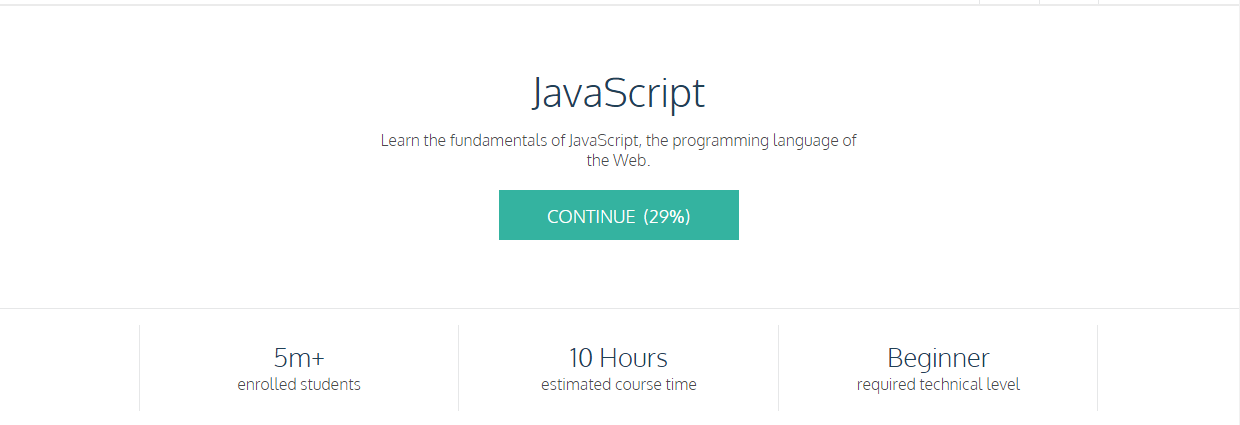
Where to go from here?
Coursera
(starts November 2015)
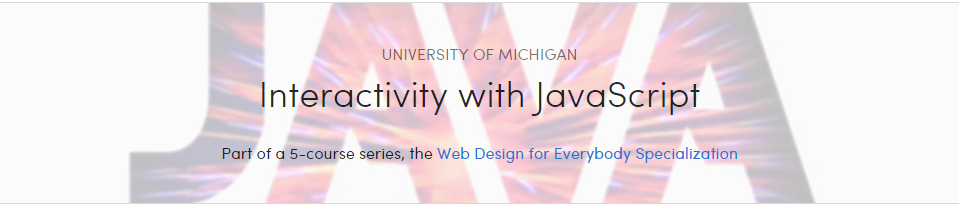
Where to go from here?
Khan Academy
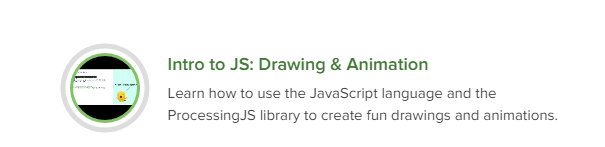
Where to go from here?
JavaScript and JQuery: Interactive Front-End Web Development
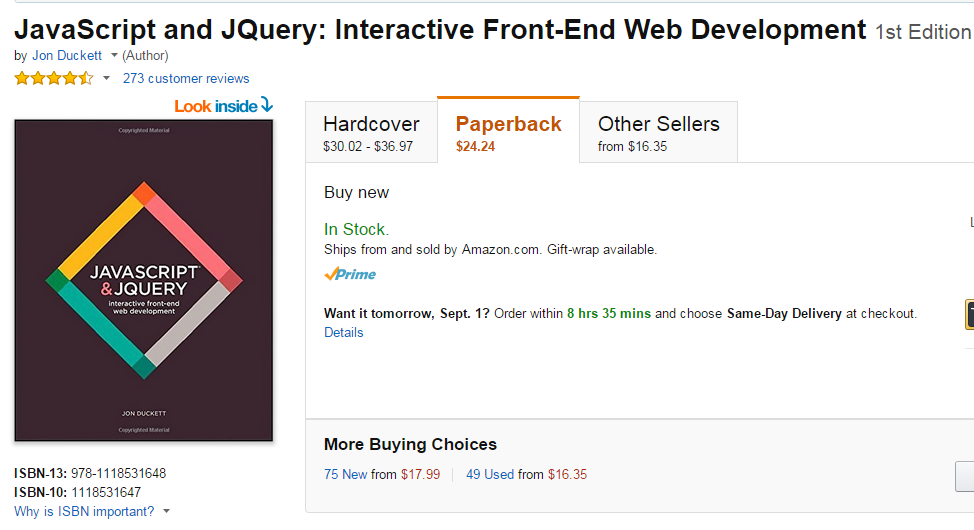
Where to go from here?
Coding Temple
Intermediate JS Workshop

Thanks for attending!
Arrays
- An array stores a list of values.
- You do not need to specify how many items you need in order to create it.
- You can create an array and give it a name just like you would with any other variable.
- Values are inside a pair of square brackets, separated by commas.
- The values do not need to be the same type.
- Indexing is off by one.
> var colors;
> colors = ['white', 'black', 'custom'];
> colors[0]; //this will return "white". Indexing is off by one.
Arrays Continued
> var numbers = [1,2,3,4];
> numbers.forEach(function(element){ console.log(element * element);})
//^The above will print out the square of each element.
forEach() executes a provided function once per array element.
var numbers = [1,2,3,4];
for (var i = 0; i < numbers.length; i++){ console.log(numbers[i] * numbers[i]);}
^The above accomplishes the same functionality as forEach.
for() loop executes code within square brackets until a condition is reached.
JS Workshop
By avipatel91
JS Workshop
- 897