PSR
PHP Standards Recommendations
FIG
Framework Interoperability Group
Process
- Pre-Draft
- Draft
- Review
- Accepted
- Deprecated
- Abandoned
PSR 0 (deprecated)
Remplacé par le PSR-4
PSR 1 & PSR 2
Pour un code tout propre
<?php
namespace Vendor\Package;
use FooInterface;
use BarClass as Bar;
use OtherVendor\OtherPackage\BazClass;
class Foo extends Bar implements FooInterface
{
public function sampleMethod($a, $b = null)
{
if ($a === $b) {
bar();
} elseif ($a > $b) {
$foo->bar($arg1);
} else {
BazClass::bar($arg2, $arg3);
}
}
final public static function bar()
{
// method body
}
}
PSR 3
LoggerInterface
<?php
namespace Psr\Log;
interface LoggerInterface
{
public function emergency(string $message, array $context = array()): void;
public function alert(string $message, array $context = array()): void;
public function critical(string $message, array $context = array()): void;
public function error(string $message, array $context = array()): void;
public function warning(string $message, array $context = array()): void;
public function notice(string $message, array $context = array()): void;
public function info(string $message, array $context = array()): void;
public function debug(string $message, array $context = array()): void;
public function log(string $level, $message, array $context = array()): void;
}
PSR 4
Autoloader
\Graf\Framework | ./lib |
---|---|
\Graf\Framework\Demo\Server | ./lib/Demo/Server.php |
PSR 6
Caching Interface
<?php
namespace Psr\Cache;
interface CacheItemInterface
{
public function getKey(): string;
public function get();
public function isHit(): bool;
public function set($value);
public function expiresAt($expiration);
public function expiresAfter($time);
}
<?php
namespace Psr\Cache;
interface CacheItemPoolInterface
{
public function getItem(string $key): CacheItemInterface;
public function getItems(array $keys = array()): iterable;
public function hasItem(string $key): bool;
public function clear(): bool;
public function deleteItem(string $key): bool;
public function deleteItems(array $keys): bool;
public function save(CacheItemInterface $item): bool;
public function saveDeferred(CacheItemInterface $item): bool;
public function commit(): bool;
}
PSR 16
"Simple" Caching Interface
<?php
namespace Psr\SimpleCache;
interface CacheInterface
{
public function get(string $key, $default = null);
public function set(string $key, $value, $ttl = null): bool;
public function delete(string $key): bool;
public function clear(): bool;
public function getMultiple(iterable $keys, $default = null);
public function setMultiple(iterable $values, $ttl = null): bool;
public function deleteMultiple(iterable $keys): bool;
public function has(string $key): bool;
}
PSR 7
HTTP message interfaces
<?php
namespace Psr\Http\Message;
interface MessageInterface
{
public function getProtocolVersion(): string;
public function withProtocolVersion(string $version): self;
public function getHeaders(): array;
public function hasHeader(string $name): bool;
public function getHeader(string $name): array;
public function getHeaderLine(string $name): string;
public function withHeader(string $name, $value): self;
public function withAddedHeader(string $name, $value): self;
public function withoutHeader(string $name): self;
public function getBody(): StreamInterface;
public function withBody(StreamInterface $body): self;
}
<?php
namespace Psr\Http\Message;
interface ServerRequestInterface extends RequestInterface
{
public function getServerParams(): array;
public function getCookieParams(): array;
public function withCookieParams(array $cookies): self;
public function getQueryParams(): array;
public function withQueryParams(array $query): self;
public function getUploadedFiles(): array;
public function withUploadedFiles(array $uploadedFiles): self;
public function getParsedBody();
public function withParsedBody($data): self;
public function getAttributes(): array;
public function getAttribute(string $name, $default = null);
public function withAttribute(string $name, $value): self;
public function withoutAttribute(string $name): self;
}
<?php
namespace Psr\Http\Message;
interface RequestInterface extends MessageInterface
{
public function getRequestTarget(): string;
public function withRequestTarget($requestTarget): self;
public function getMethod(): string;
public function withMethod(string $method): self;
public function getUri(): UriInterface;
public function withUri(UriInterface $uri, bool $preserveHost = false): self;
}
PSR 11
ContainerInterface
<?php
namespace Psr\Container;
interface ContainerInterface
{
public function get(string $id);
public function has(string $id): bool;
}
PSR 13
Link Interfaces
<?php
namespace Psr\Link;
interface LinkInterface
{
public function getHref(): string;
public function isTemplated(): bool;
public function getRels(): array;
public function getAttributes(): array ;
}
Draft
PSR 5 (draft)
PHPDoc
PSR 8 (draft)
Huggable Interface
PSR 9 & 10 (draft)
Security Reporting
PSR 12 (draft)
Encore plus de règles de formatage
<?php
namespace Vendor\Package;
use FooClass;
use BarClass as Bar;
use Vendor\Package\Namespace\{
SubnamespaceOne\ClassA,
SubnamespaceOne\ClassB,
SubnamespaceTwo\ClassY,
ClassZ,
};
class ClassName extends ParentClass implements \ArrayAccess, \Countable
{
// constants, properties, methods
}
PSR 14 (draft)
Event Manager
<?php
namespace Psr\EventManager;
interface EventManagerInterface
{
public function attach(string $event, callable $callback, int $priority = 0): bool;
public function detach(string $event, callable $callback): bool;
public function clearListeners(string $event): void;
public function trigger($event, $target = null, $argv = array());
}
PSR 15 (draft)
HTTP Middlewares
<?php
namespace Psr\Http\ServerMiddleware;
use Psr\Http\Message\ResponseInterface;
use Psr\Http\Message\ServerRequestInterface;
interface MiddlewareInterface
{
public function process(
ServerRequestInterface $request,
DelegateInterface $delegate
): ResponseInterface;
}
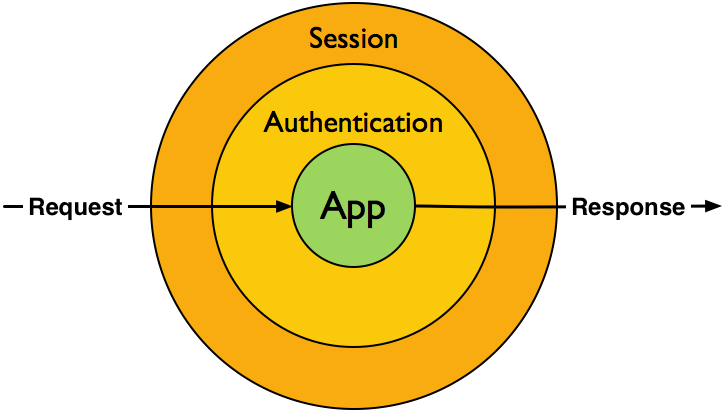
PSR 17 (draft)
HTTP Factories
<?php
namespace Psr\Http\Message;
use Psr\Http\Message\RequestInterface;
use Psr\Http\Message\UriInterface;
interface RequestFactoryInterface
{
public function createRequest($method, $uri): RequestInterface;
}
interface ResponseFactoryInterface
{
public function createResponse($code = 200): ResponseInterface;
}
Qu'est ce que le PSR ?
By Jonathan Boyer
Qu'est ce que le PSR ?
Petite présentation sur l'utilité du PSR et de ses standards
- 19,216