living in Harmony:
exploring ES6
ES6 aka ECMAScript 6 aka Harmony
'Harmony' is the next-generation spec for the ECMAScript language.
Currently in draft status, expected to be finalised in December 2014.
Excellent semantic and syntactic improvements.
WHATScript?
1994 - Netscape Navigator 2.0 introduces Mocha scripting language
Later Renamed to LiveScript, then renamed to JavaScript
1996 - Microsoft introduces JavaScript support to Internet Explorer 3.0
Their implementation is later renamed to JScript to avoid copyright issues
1997 - Industry standard, EMCAScript, is born
(ECMA === European Computer Manufacturers Association)
1998 - ES2
Editorial changes to meet ISO requirements
1999 - ES3
Regular expressions, try/catch + more
2009 - ES5
Strict mode, Array.map, reduce, etc. Object.keys, create, etc + more
living in Harmony
Syntactical Improvements
Destructuring
Block Scope
Default Arguments
Arrow Functions
Rest/Spread Operators
Semantic Improvements
Classes
Modules
Tooling
node --harmony
traceur
destructuring arrays
var a = 10,
b = 20;
var [a, b] = [10, 20];
var today = function() {
return [17, 7, 2014];
};
var [day, month, year] = today();
var [, , year] = today();
destructuring objects
var {foo: a, bar: b} = {foo: 10, bar: 20};
var today = function() {
return {
d: 17,
m: 7,
y: 2014
};
};
var {d: day, m: month, y: year} = today();
var {y: year} = today();
destructuring arguments
var fn = function({title: title, author: author}) {
console.log(title, author);
};
var book = {
title: "Atlas Shrugged",
author: "Ayn Rand"
};
fn(book);
function/block scope
var fn = function() {
var foo = 'bar';
console.log(foo); // 'bar'
};
console.log(foo); // Throws
var fn = function() {
for (var i = 0; i < 10; i++) {
var foo = i;
}
console.log(foo); // ?
}
fn();
block scope - 'let' keyword
var fn = function() {
for (var i = 0; i < 10; i++) {
let foo = i;
}
console.log(foo); // Throws
}
fn();
default arguments
var fn = function(name) {
name = name || 'default';
console.log(name);
}
fn('foo'); // 'foo'
fn(); // 'default'
var fn = function(name = 'default') {
console.log(name);
}
fn('foo'); // 'foo'
fn(); // 'default'
arrow functions
var square = function(n) {
return n * n;
};
console.log(square(2)) // 4
var square = n => n * n;
console.log(square(2)); // 4
var ages = people.map(function(person) {
return person.age;
});
var ages = people.map(person => person.age);
rest operator
var sum = function() {
var numbers = Array.prototype.slice.call(arguments);
return numbers.reduce(function(total, number) {
return total + number;
});
};
console.log(sum(1,2,3)) // 6
var sum = function(...numbers) {
return numbers.reduce(function(total, number) {
return total + number;
});
};
console.log(sum(1,2,3)) // 6
spread operator
var numbers = [4,8,15,16,23,42];
var result = sum.apply(null, numbers);
console.log(sum(numbers)) // 108
var numbers = [4,8,15,16,23,42];
console.log(sum(...numbers)) // 108
class syntax
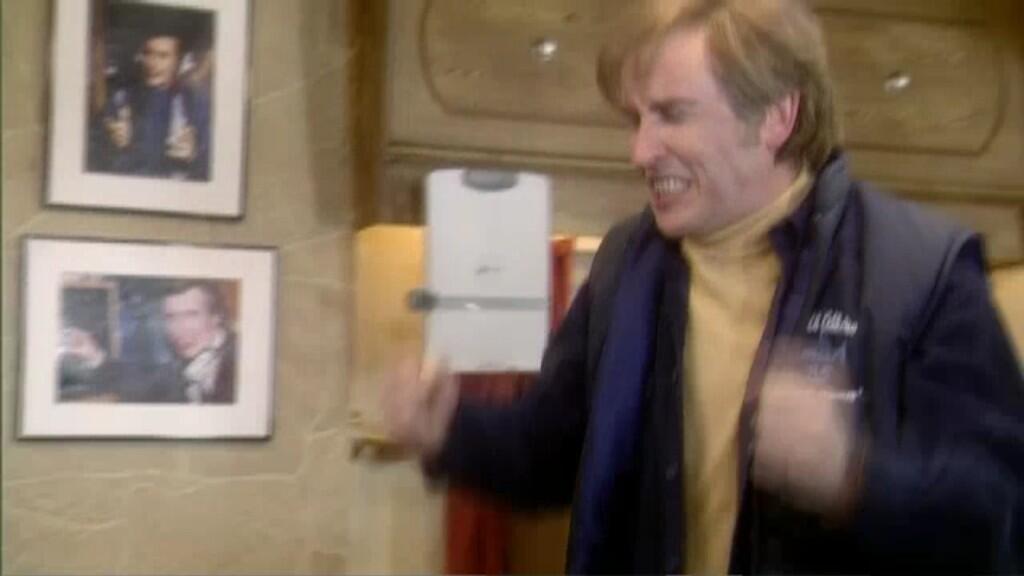
Stop Getting Javascript Wrong!
- This isn't Java
- Embrace Prototypal Inheritance
- ES5 makes this easy
- Just because you can...
- Doesn't mean you should.
- Just because others do it...
- Doesn't mean you need to.
- ES5 makes this easy
- Doesn't mean you should.
- Doesn't mean you need to.

y u do dis?
// Mootools
var Monkey = new Class({
initialize: function(name){
this.name = name;
}
});
// Prototype
var Monkey = Class.create({
initialize: function(name) {
this.name = name;
}
});
// YUI
var Monkey = Y.Base.create('monkey', null, [], {
initializer: function(name) {
this.name = name;
}
});
// dojo
var Monkey = declare(null, {
constructor: function(name){
this.name = name;
}
});
object.create()
// Base
var monkey = {
food: "Bananas",
diet: function() {
console.log("I eat " + this.food);
}
};
monkey.diet(); // "I eat Bananas"
// Inherit
var charles = Object.create(monkey, {
food: { value: "Biscuits" }
});
charles.diet(); // "I eat Biscuits"
object.create()
var human = Object.create(monkey, {
food: { value: "Complex Carbohydrates" },
diet: {
value: function() {
// Call super
monkey.diet.call(this);
console.log('... only on Fridays');
}
}
});
human.diet();
class Monkey {
constructor(food) {
this.food = food;
}
diet() {
console.log("I eat " + this.food);
}
}
var charles = new Monkey('Bananas');
charles.diet();
class Human extends Monkey {
constructor(food) {
super(food);
}
diet() {
console.log("I eat moderate amounts of " + this.food);
}
}
var warren = new Human('Complex Carbohyrdrate');
warren.diet();
exploring ES6
By Warren Seymour
exploring ES6
- 1,276