The Promised Land
Handling asynchronous behaviour in PHP
Warren Seymour
warren@radify.io
@woogoose
Asynchronous PHP
- HHVM/Hack - async/await
- Guzzle - Promises
- React - Callbacks + Promises
- PHP 7+ (maybe?)
Callback Hell - Serial
step1('foo', function($result1) {
// step2 depends on result of step1
step2($resultOne, function($result2) {
// Do something with result of step2
});
});
Callback Hell - Parallel
$counter = 0;
$handler = function($result) use (&$counter) {
$counter += 1;
if ($counter === 2) {
// Do something...
}
};
doSomething('foo', $handler);
doSomethingElse('bar', $handler);
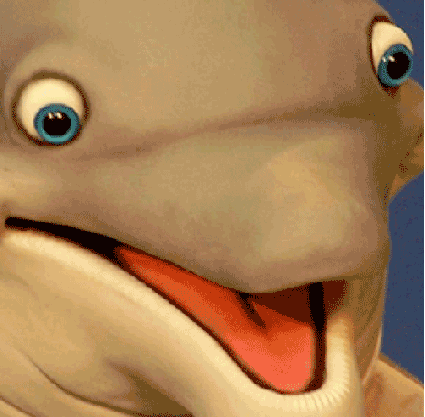
[spaghetti intensifies]
All hail the Promise
promiseMe('foo')
->then(function($result) {
// do something with result
})
->otherwise(function($error) {
// handle error
})
->always(function() {
// clean up
});
Serial Calls
promiseMe('foo')
->then(function($result) {
return promiseMe('bar');
})
->then(function($result2) {
// do something with second result
});
Parallel Calls
use React\Promise;
Promise\all([
promiseMe('foo'),
promiseMe('bar')
])
->then(function($results) {
// Do something with array of results
})->catch(function() {
// Handle error immediately
});
Isn't this where we came in?
use React\Promise\Deferred;
function promiseMe($params) {
$deferred = new Deferred();
doSomething($params, function($result) use ($deferred) {
$deferred->resolve($result);
});
return $deferred->promise();
}
What now?
- Async
ismay be coming to PHP - Promises > Callbacks
- A powerful pattern to be aware of
The Promised Land
By Warren Seymour
The Promised Land
Handling asynchronous behaviour in PHP
- 2,502