JavaScript 101
World of ECMAScript
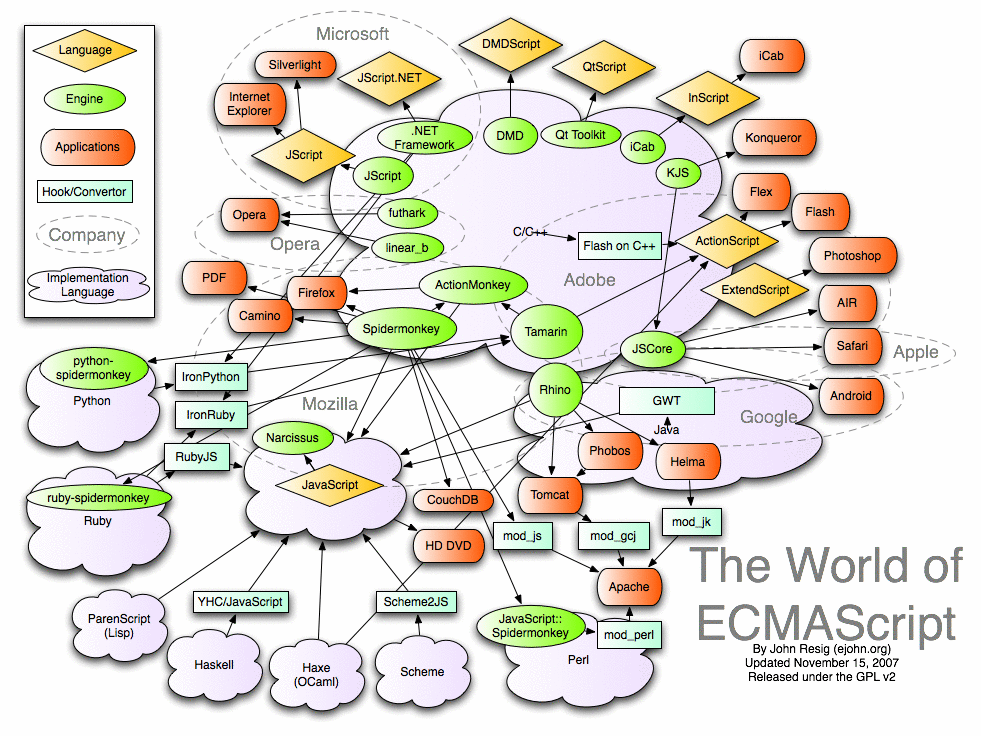
Primitive Types
/** A few primitives **/
// booleans
true, false
// strings
"hello", 'world', "hello \nworld"
// numbers
10, 0x10, 010, 10.0, 10.
/** And two trivial **/
// no assigned value or property not exists
undefined
// a special value indicating no value
null
JavaScript is a completely dynamic language - no "types"
Implicit Type Conversion
String | Number | Boolean | Object | |
---|---|---|---|---|
undefined | "undefined" | NaN | false | Error |
null | "null" | 0 | false | Error |
Nonempty string | Numeric value or Nan | true | String | |
Empty string | 0 | false | String | |
0 | "0" | false | Number | |
NaN | "NaN" | false | Number | |
Infinity | "Infinity" | true | Number | |
Negative infinity | "-Infinity | true | Number | |
number | string value | true | Number | |
true | "true" | 1 | Boolean | |
false | "false" | 0 | Boolean |
Variable Declaration
- Variable is declared using
var keyword - Variables are scoped to the function, not to the block
var a = 0; // "a" is a "global" variable here
function test() {
b = 10; // QUESTION: Is "b" a variable?
for (var i = 0; i < 10; i++) {
a++; // global variable is visible in inner scopes
}
return i; // == 10 because "i" is scoped under "test()", not "for"
}
Operators
Most akin to most other major languages, the tricky part is logical operators:
// If the first operand can be converted to true,
// returns its unconverted value
var foo = "test" || 200; // "test"
// If the first operand cannot be converted to true,
// returns its unconverted value
var foo = '' && 200; // ''
// Otherwise, its second operand is evaluated,
// and returns the value of expresssion
var bar = '' || 0 || 200; // 200
var bar = "test" && 200; // 200
|| is widely used to make a default value chain
&& could be considered as a short for if () expr;
Built-in Objects
The built-in objects are a subtype of native objects which are built into the ECMAScript prior to the beginning of a program.
-
Primitive Wrapper Objects: Boolean, String, Number
-
Core Objects: Object, Array, Function
-
Util Objects: Math, Date, RegExp, Error
-
Literal & JSON
Question: Is "document" a built-in object?
Primitive Wrappers
- Boolean, String, Number are built-in wrappers for boolean, string, number primitives
- Wrapper objects have useful properties and methods, e.g. substring, toFixed, etc.
- While these methods actually work on primitives, too, via the boxing mechanism
var s = new String("string"); // typeof s === 'object'
var n = new Number(10.0); // typeof n === 'object'
var b = new Boolean(true); // typeof b === 'object'
s.substr(1) === "string".substr(1) === 'tring'
n.toFixed() === 10.0.toFixed() === 10..toFixed() === 10
Tips and Tricks: Primitive's Property
The Object Object
- Object is the "root" of all built-in objects, which can be considered as hash table of key-value pairs
- While just avoid to use object constructor with primitive value, it may cause mess
var o = new Object(); // {}
o.foo = "bar"; // { foo: 'bar' }
delete o.foo; // {}
var s = new Object("string"); // = new String("string")
var n = new Object(10.0); // = new Number(10.0)
var b = new Object(true); // = new Boolean(true)
The Array Object
- Akin to most other major languages
- Array constructor curiousness:
- The length property retrieves the index of the next available (unfilled) position at the end of the array, and is auto updated as new elements are added.
var a1 = new Array(); // [] - empty array
var a2 = new Array('a', 'b'); // ['a','b'] - array with 2 items
var a3 = new Array(2); // [empty x 2] - empty with length of 2
a1.length === 0
a2.length === a3.length === 2
// What happens if length is set to 0
a3.length = 0;
JavaScript Object Literals
var o = new Object();
o.prop1 = val1;
o.prop2 = val2;
var a = new Array(
element1,
element2,
...,
elementN
);
var f = new Function(
"arg1",
"arg2",
...,
"argN",
"statement"
);
var r = new RegExp("exp", "flags");
var o = {
prop1: val1,
prop2: val2
};
var a = [
element1,
element2,
...,
elementN
];
var f = function(arg1, ..., argN) {
statements
};
var r = /exp/flags;
JavaScript Object Notation
- JSON is a lightweight data-interchange format, which derived from JavaScript programming language.
- Parse JSON text into JavaScript object via eval function
JavaScript 101
By Haili Zhang
JavaScript 101
- 828