INTRO O
PROBLEM SOLVING AND
PROGRAMMING IN PYTHON
(use the Space key to navigate through all slides)

Prof. Andrea Gallegati |
Prof. Dario Abbondanza |

CIS 1051 - WHILE LOOP

Understanding the
statement
REPEATING Actions in Code
while
Repeating Until a Condition is Met
- Keep asking for a password until the correct one.
- Keep rolling a dice until you get a 6.
- Count down from 10 to 1 before a rocket launch.

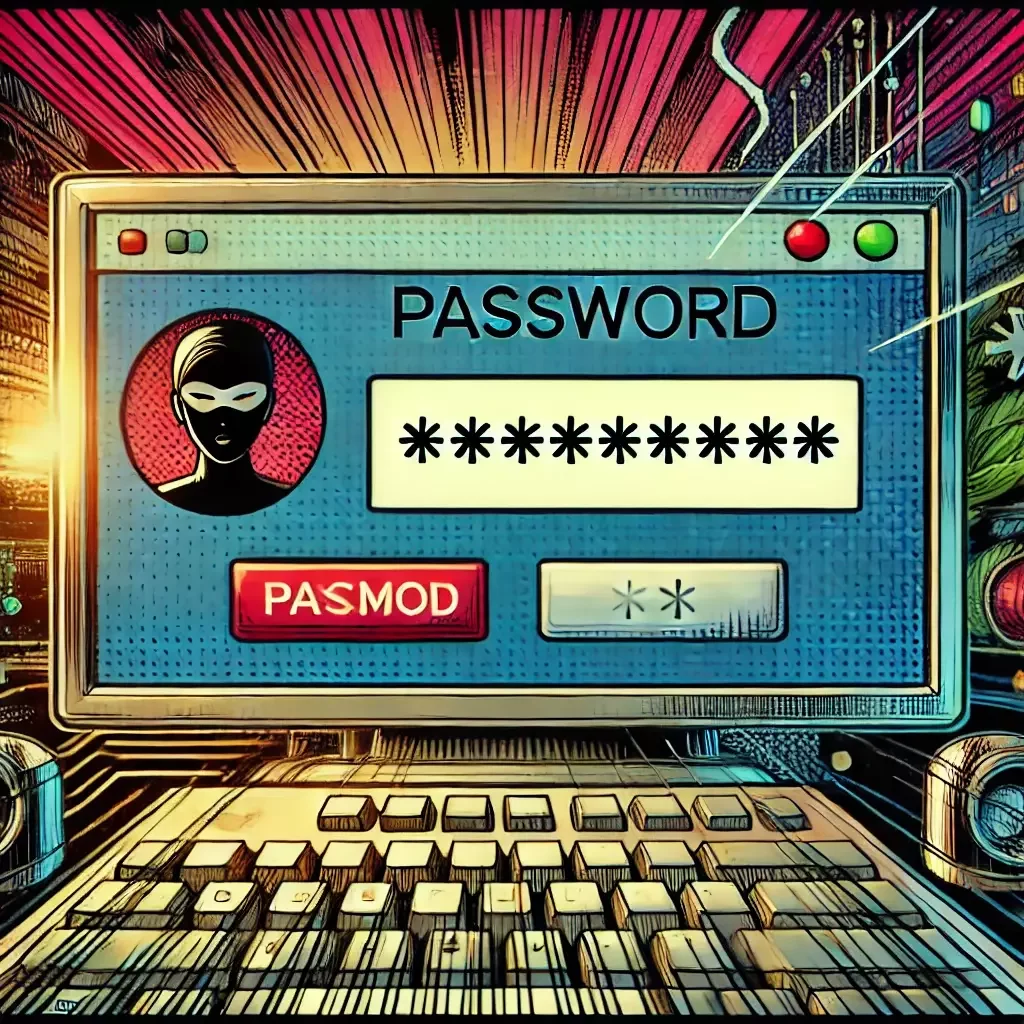

while
loops
repeat actions
as long as a condition remains true.
Syntax:
write Python programs that
repeat the same code execution
while condition:
# Code to repeat (indented block)
pass
- The condition is checked before each iteration.
- If it's
True
, the loop executes. - If it's
False
, the loop stops.
Example: Counting Up
count = 1 # Start at 1
while count <= 5: # Keep going while count is ≤ 5
print("Count:", count)
count += 1 # Increase count by 1
-
count
starts at 1. - Each time,
count
increases. - The loop stops at 6.
Example: Launch Countdown!
countdown = 5
while countdown > 0:
print("T-minus", countdown)
countdown -= 1 # Reduce countdown
print("🚀 Liftoff!")
- Runs while
countdown > 0
. -
Decreasing
countdown
. -
Stops,
"Liftoff!"
prints.
Example: Using it for User Input
password = ""
while password != "python123": # Keep asking
password = input("Enter the password: ")
print("Access granted! ✅")
Useful when we don't know how many times we'll repeat an action.
- entering
"python123"
, the loop stops. - for anything else, the loop keeps asksing.
Using break
to Exit a Loop Early
number = 1
while number <= 10:
print("Checking number:", number)
if number == 7: # Stop when number is 7
print("Number 7 found! Stopping loop.")
break
number += 1
Sometimes, we need to exit a loop immediately.
break
exits the loop even if the condition is stillTrue
.
Using continue
to Skip an Iteration
number = 0
while number < 10:
number += 1
if number % 2 == 0:
continue # Skip even numbers
print(number)
It skips the rest of the loop's code for the current iteration.
- if even,
continue
skips
theprint()
statement.
Using else
within a while
Loop
number = 1
while number <= 5:
print(number)
number += 1
else:
print("Loop finished successfully!")
else
block runs if the loop
completes normally (without break
).
- no
break
, thenelse
block runs.
⚠️ Danger ZONE
If the loop condition never becomes False
, it runs forever.
✅ To avoid infinite loops, make sure:
- condition eventually becomes
False
. - modify variables inside the loop (like increasing
count
).
Example: Infinite Loop
while True:
print("This loop runs forever!")
If the loop condition never becomes False
, it runs forever.
- If always
True
, it never stops. -
Ctrl+C
to STOP the program.
Example: Simulating a Dice Roll 🎲
import random
roll = 0
while roll != 6:
roll = random.randint(1, 6) # Roll a die (1 to 6)
print("Rolled:", roll)
print("🎲 Got a 6! Loop stopped.")
we don’t know how many times
it will run!
- it keeps rolling until we get
6
- the number is random, thus we don’t know how many rolls it takes
Recap
-
while
loop repeats actions as long as condition isTrue
.
- Use
break
to exit a loop immediately. - Use
continue
to skip an iteration.
-
while
loop can have anelse
block
(runs if there’s nobreak
).
Be careful of infinite loops—always make sure the condition eventually becomes False
.
Try It Yourself!
write a program that keeps asking the user for a number (greater than 10) until they enter it!
number = 0 # Start with a number less than 10
while number <= 10:
number = int(input("Enter a number greater than 10: "))
print("Thanks! You entered", number)
Great Job! 🎉
Now you understand while loops and how to repeat actions until a condition is met!
Try experimenting with your
own conditions! 🚀
This was crafted with
A Framework created by Hakim El Hattab and contributors
to make stunning HTML presentations
while-loop
By Andrea Gallegati
while-loop
- 29