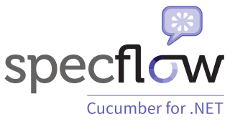
an introduction
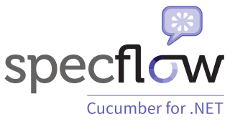
an introduction
what is BDD?
what is specflow & gherkin?
writing tests in gherkin
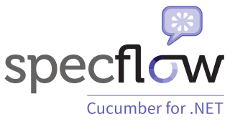
an introduction
what is BDD?
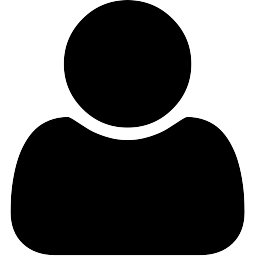
developer
write code
write test
tests fail
tests pass
TDD
// arrange
var x = 10;
var y = 10;
var expected = 20;
// act
var actual = Math.Add(x, y);
// assert
Assert.AreEqual(expected, actual);
AreEqual failed, expected <10> got <0>
class Math {
function Add(number x, number y) {
// TODO: add numbers
return 0;
}
}
class Math {
function Add(number x, number y) {
return x + y;
}
}
Test: add numbers
Result: pass
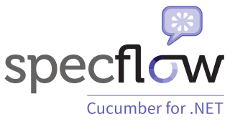
an introduction
what is BDD?
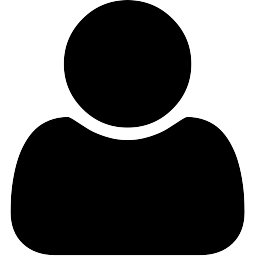
tester
report results
write test
run tests
TDD, BDD
Given a product costs 100
And VAT is 20%
When a VAT receipt is produced
Then the subtotal is 100
And the total is 120
Feature: Receipts
Scenario: An order with VAT
Running...
Result: failed
> Then the subtotal is 100
Expected 100 got 120
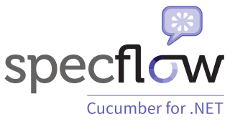
an introduction
what is BDD?
business
approve changes
define rules
produce acceptance criteria
TDD, BDD, ATDD
As a business customer
I want VAT receipts
So that I can do my accounts
Feature: Tax Receipts
Scenario: Order as a UK business
Given a UK business makes an order
When the payment is processed
Then the receipt includes tax information
Scenario: Order as a Lilliput business
Given a Lilliput business makes an order
When the payment is processed
Then the receipt has no tax information
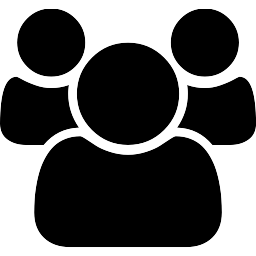
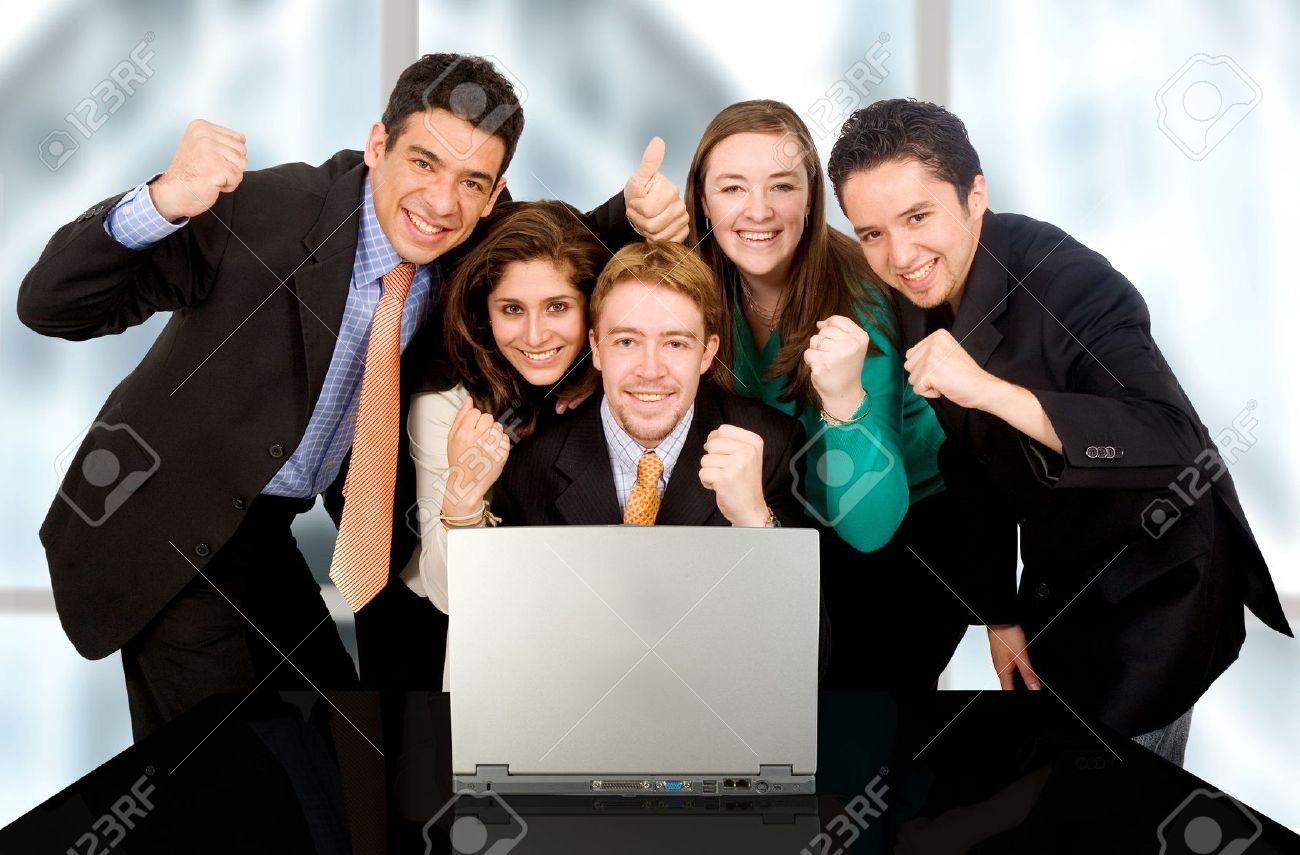
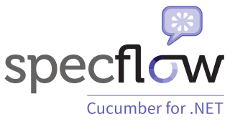
an introduction
what is specflow and gherkin?
what is specflow:
specflow is a tool kit that brings gherkin testing to .net and Visual Studio
1. tools to help testers write gherkin in VS
it includes:
2. libraries to run gherkin tests
what is gherkin:
gherkin is a business friendly language for tests
gherkin tests live in feature files, e.g. VatReceipt.feature
why:
we get an executable and business friendly language
we can write tests before development is ready
when tests fail the errors are easier to identify
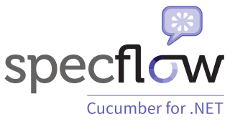
an introduction
writing tests in gherkin
the feature file
Feature:
Feature: ReceiptToClient
In order to post a receipt from the client into the client account
I want to be able to post a RTC journal
Scenario:
Scenario: I edit and post a previously saved RTC journal
Given I have opened a previously saved RTC journal
When I make changes to the journal and save
Then I see a message indicating the journal posted
And the journal reflects the new changes
Feature describes the feature under test. The code here is not executed.
Scenario defines a test. Each scenario is executed once in a test run.
Given, When and Then must be used to start each test step. You can also use And and But if you are writing more complex tests.
specflow in Visual Studio will generate C# code behind the feature files testers create.
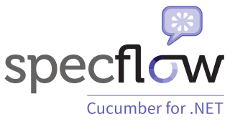
an introduction
writing tests in gherkin
the feature file
Background:
Background:
Given I have logged into LexisOne as Eric
Using parameters:
Scenario: I can create a client with the minimum information
Given I am creating a client with name "Fred"
And customer group "UK-CL-UK"
When I save a new client
Then the client is saved with no errors
Background is executed before a scenario runs. Use it if you are writing the same Given steps over and over!
specflow will detect "quoted" strings and numbers and pass them as parameters to the code it generates:
specflow in Visual Studio will generate C# code behind the feature files testers create.
[Given(@"I am creating a client with name ""(.*)""")]
public void GivenIAmCreatingAClientWithName(string p0)
{
// do something with the name "Fred"
}
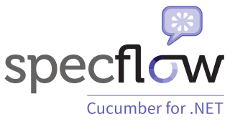
an introduction
writing tests in gherkin
the feature file
Table parameters:
Scenario: I can log in as different users
Given the following users exist
| Username | Password |
| Bob | bob123 |
| Alice | ilovebob |
When I am logged in as each user
Then I can see my profile
The Given step in this scenario receives the data as a Table:
specflow in Visual Studio will generate C# code behind the feature files testers create.
[Given(@"the following users exist")]
public void GivenTheFollowingUsersExist(Table table)
{
// capture the users so other steps see them
ScenarioContext.Current.Add("users", table);
}
[Given(@"I can see my profile")]
public void ThenICanSeeMyProfile()
{
// capture the users so other steps see them
var table = (Table)ScenarioContext.Current["users"];
}
Further steps load the data from ScenarioContext:
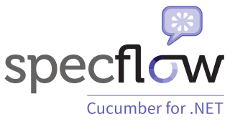
an introduction
writing tests in gherkin
the feature file
Scenario Outline:
Scenario Outline: I can log in as a user
Given I login with <username> and <password>
When I view my profile
Then I can see my avatar
Examples:
| Username | Password |
| Bob | bob123 |
| Alice | ilovebob |
specflow in Visual Studio will generate C# code behind the feature files testers create.
A Scenario Outline consists of Scenario Outline and the Example section.
Unlike the Table parameter, this data is available on a row by row basis. The scenario here generates multiple tests.
[When(@"I log in with ""(.*)"" and ""(.*)""")]
public void ILogInWithUsernameAndPassword(string p0, string p1)
{
// this runs once per row instead
// p0 is username, p1 is password
}
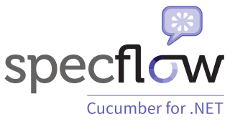
an introduction
questions
specflow introduction
By Benjamin Babik
specflow introduction
- 188