React
Components & Props
Hvordan får du barna dine til å pusse tennene sine?
Agenda
- Class components vs Functional components
- Components
- Filstruktur
- Props
- Events and handling
- Callback/function param
- Innebygd event props
- (Hvis tid...)
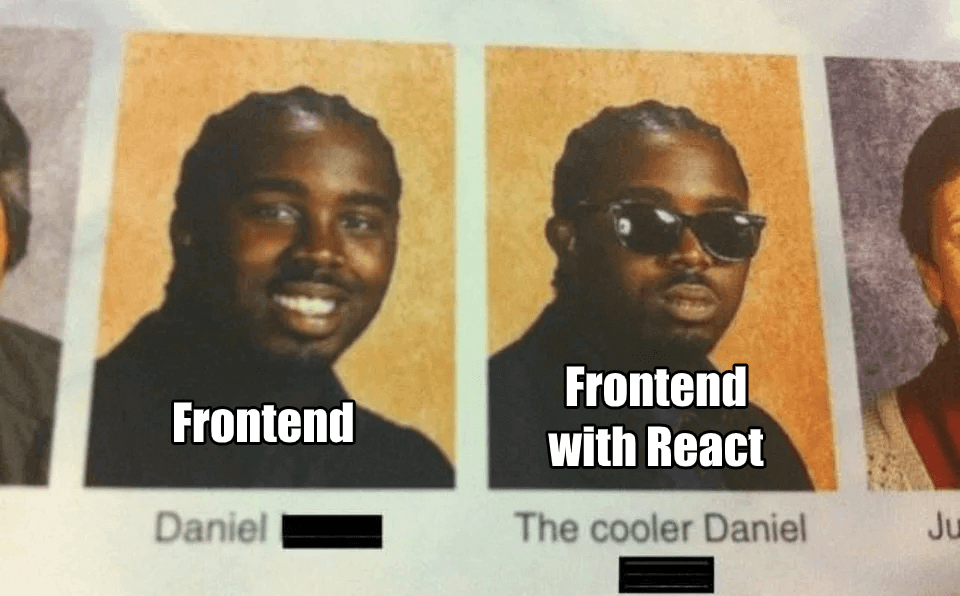
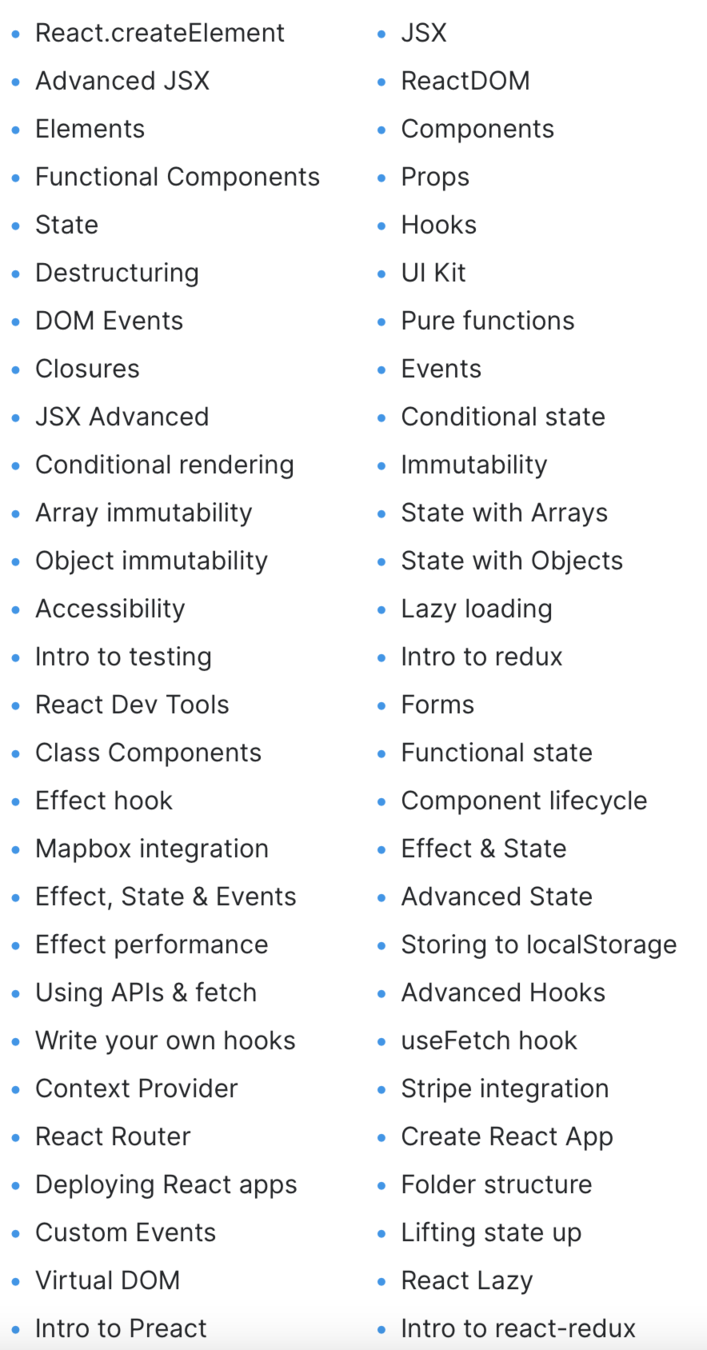
Class components
vs
Functional components
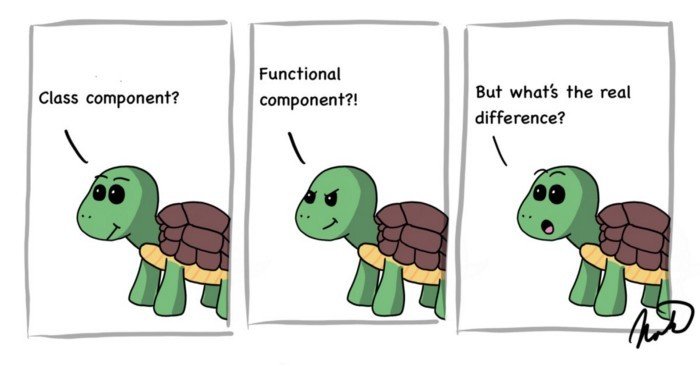
Class
Functional
import React, { Component } from "react";
class HelloWorld extends Component {
render() {
return <h1>Hello, World</h1>;
}
}
export default HelloWorld
import React from "react";
const HelloWorld = () => {
return (
<h1>Hello World</h1>
);
};
/*
const HelloWorld = function () {
return <h1>Hello World</h1>;
};
*/
// const HelloWorld = () => <h1>Hello World</h1>;
export default HelloWorld;
Hvordan får du barna dine til å pusse tennene sine?
Component
Props
Component
Component
- Gjenbrukbarhet
- Tommelfinger regel
- Samme kode mer enn 2 ganger
- Lag en komponent!
- Hold komponentene små
- KISS (Keep It Simple Stupid)
- Samme kode mer enn 2 ganger
- Kontakt skjema :D
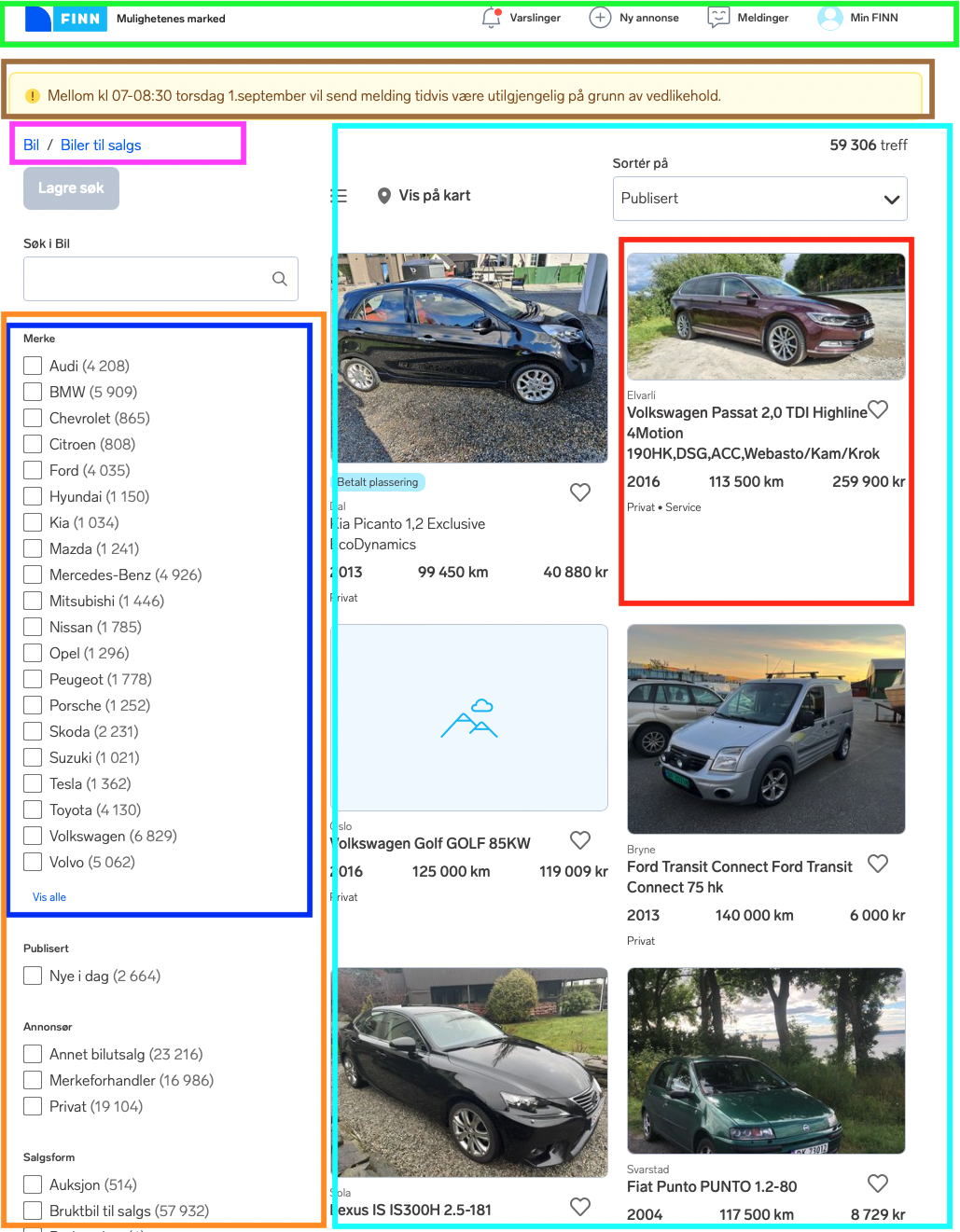
Component
- Gjenbrukbarhet
- Tommelfinger regel
- Samme kode mer enn 2 ganger
- Lag en komponent!
- Hold komponentene små
- KISS (Keep It Simple Stupid)
- Samme kode mer enn 2 ganger
- Kontakt skjema :D
// App.js
import React from 'react';
import Welcome from './Welcome'
const App = () => {
return (
<Welcome />
<Welcome />
<Welcome />
)
}
export default App;
// Welcome.js
import React from 'react';
const Welcome = () => {
return <h1>Hello World</h1>;
}
export default Welcome;
Component
- Navn konvensjon - Stor bokstav
- Bruk logiske og beskrivende navn
- Fil og mappe struktur
- .js eller .jsx
- Varierer
-
Is there a recommended way to structure React projects?
React doesn’t have opinions on how you put files into folders. That said there are a few common approaches popular in the ecosystem you may want to consider.-
https://reactjs.org/docs/faq-structure.html
-
#common/
Avatar.js
Avatar.css
APIUtils.js
APIUtils.test.js
#feed/
index.js
Feed.js
Feed.css
FeedStory.js
FeedStory.test.js
FeedAPI.js
#profile/
index.js
Profile.js
ProfileHeader.js
ProfileHeader.css
ProfileAPI.js
#api/
APIUtils.js
APIUtils.test.js
ProfileAPI.js
UserAPI.js
#components/
Avatar.js
Avatar.css
Feed.js
Feed.css
FeedStory.js
FeedStory.test.js
Profile.js
ProfileHeader.js
ProfileHeader.css
Grouping by Feature or routes
Grouping by type or “role”
Component - Struktur
- Min preferanse
#components/
#ComponentNameFolder/
ComponentName.jsx
ComponentName.css
ParentComponentName.jsx
ParentComponentName.css
ChildComponentName.jsx
ChildComponentName.css
#common/
#Buttons/
PrimaryButton.jsx
PrimaryButton.css
SecondaryButton.jsx
SecondaryButton.css
#AdList/
AdList.jsx
AdList.css
#pages/
#Home/
Home.jsx
Home.css
#AboutUs/
AboutUs.jsx
AboutUs.css
#common/
mvaCalculations.js
dateFormatter.js
App.jsx
Demo
Props
Props
- Properties
- Parameter (?)
- Optional
- Sende ("pass") data til en annen komponent
const Welcome = props => {
const { name } = props;
return <h1>Hello, {name}</h1>;
// return <h1>Hello, {props.name}</h1>;
}
// ------------------------------------------
class Welcome extends React.Component {
render() {
return <h1>Hello, {this.props.name}</h1>;
}
}
Props
- Properties
- Parameter (?)
- Optional
- Sende ("pass") data til en annen komponent
- props vs attribute
- props navn === attribute navn
- Verdien kan være "hva som helst"
- Verdien kan ikke endres
- Read only!
// Welcome.js
const Welcome = props => {
const { name } = props;
return <h1>Hello {name}</h1>;
}
export default Welcome;
// ------------------------------------------
// App.js
import Welcome from './Welcome'
const App = () => {
return (
<Welcome name="Kari" />
);
}
export default App;
Attribute
Props
Props
- Default props verdier
- Bl.a.: children
- Legg merke til < >
- Bl.a.: children
// Welcome.js
const Welcome = props => {
return <div>{props.children}</div>;
}
export default Welcome;
// ------------------------------------------
// App.js
import Welcome from './Welcome'
const App = () => {
return (
<Welcome>
<p>Hello Kari</p>
</Welcome>
);
}
export default App;
Demo
- button
- Cusom
- children
Events and handling
Button event props - Demo
Spørsmål?
Hooks: useState()
- Generelt hooks
- Hooks regler
- useState vs setState
- useState( )
- useState with Array and Objects (Hvis tid)
- Custom hooks (???)
Neste gang
2. Components & Props
By dawood11
2. Components & Props
2. Components & Props
- 65