It's not me, it's VUE

APPMAN - András Takács (att@pulilab.com)
DON NICO - Nicolò Maria Mezzopera (nico@pulilab.com)
MR F - Fekete György (f@pulilab.com)
2017-10-19 VUEJS Budapest meetup @ LogMeInHQ
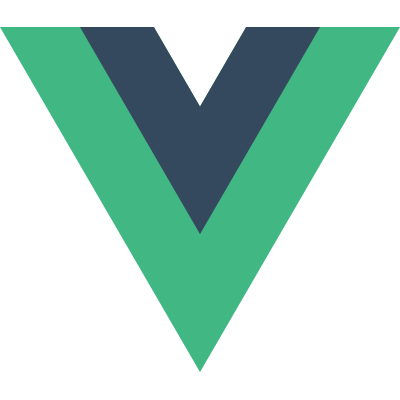
VUE.JS Introduction
The Progressive
JavaScript Framework
- Approachable
- Versatile
- Performant
- Incrementally adoptable
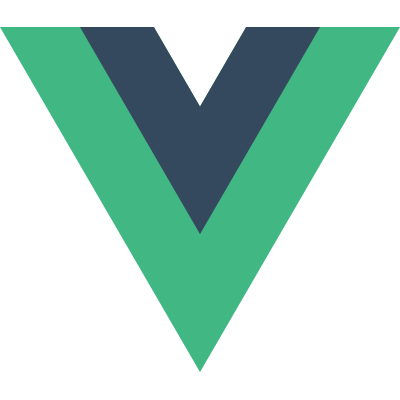
VUE.JS Introduction

<script src="https://unpkg.com/vue"></script>
var app = new Vue({
el: '#app',
data: {
message: 'Hello Vue!'
}
})
<div id="app">
{{ message }}
</div>
VUE.JS Features

- Declarative rendering
- Virtual DOM
- Component system
Vue.component('todo-item', {
template: '<li>This is a todo</li>'
})
<ol>
<todo-item></todo-item>
</ol>

VUE.JS Features

- MVVM pattern - Reactivity

var vm = new Vue({
data: data
)}
VUE.JS instance

const vm = new Vue({
props: ['default'],
data () {
return {
a: 1,
b: 2
}
},
computed: {
c () {
return this.a + this.b;
}
},
methods: {
divide (value) {
const final = value ? value : this.default
return this.c / final;
}
},
components: {}
})
VUE.JS Caveats

- Vue cannot detect the following changes to an array with:
- vm.items[indexOfItem] = newValue
- vm.items.length = newLength
- Instead:
- Vue.set(vm.items, indexOfItem, newValue)
- vm.items.splice(newLength)
VUE.JS Caveats

- Vue cannot detect property addition or deletion:
var vm = new Vue({
data: {
a: 1
}
})
// `vm.a` is now reactive
vm.b = 2
// `vm.b` is NOT reactive
- Instead:
- Vue.set(vm, 'b', 2)
- this.b = Object.assign({}, this.b, {
lol: 1,
omg: 2
})
VUEX State Management

- The state, which is single source of truth that drives our app
- The view, which is just a declarative mapping of the state
- The actions, which are the possible ways the state could change in reaction to user inputs from the view.
VUEX State Management


VUEX State Management

- Multiple views may depend on the same piece of state.
- Actions from different views may need to mutate the same piece of state.
Why We Chose It

the problem we wanted to solve:
- Build an amazing UI
- 100% test coverage
- flexible business logic
- great flexibility for the designer
Considering all of the above VUEJS was our solution
Summary

-
vue-cli
-
Hello Word
-
Our Strategy
-
Stack
vue-cli & Hello Word

(sudo) npm install -g vue-cli
vue init webpack vuejs-is-awesome
cd vuejs-is-awesome
npm install
npm run dev
Our Strategy

- Put all the Business Logic in the state manager
- Keep components atomic and simple
- Ajax layer handled by the state manager
- Fully unit test all the 'js' files
- Fully e2e test all '.vue or view' components
- Plugins system to 'drop-in' new functionalities
- Aggressive linting and code uniformity
Stack I

- Backend / API served trough Django
- Axios to handle AJAX calls
- Server Side Rendering and Routing with Nuxt
- Vuex as a state manager, divided in meaningful namespaced modules
- AvaJS for Asynchronous unit test
- CypressJS for integration / e2e tests
Stack II

Nuxt.js: a framework for creating Universal Vue.js Applications
We used it for
- Routing
- Server Side Rendering and SEO
- Plugin System
- CSS Minification / Optimisation
- Code Structure
- Integrated fully with Vuex
- Middleware logic
- Layouts
Stack III

Vuex: a state management pattern + library for Vue.js applications
Enabled us to:
- Have one way data binding in our components
- Time Traveling debugging
- Debug logs contain the data structure snapshotted when the bug occurs
- It's the core of the application and serves all the view component reliably
Stack IV

A pinch of probability is worth a pound of perhaps.”— James Thurber
We LOVE Testing...
...but unit test are not enough ...
... and component unit testing are to prone to break:
- We moved all the logic to the store, being able to write 'stable' and reliable tests
- E2E test for the main 'flows' of the app and for every fixed bug (Cypres)
Battle of Frameworks


Battle of Frameworks

- Solve the same problem by different framework: Angular 2, React, Polymer, Vue
- should have:
- sitebuild friendly
- speedy
- good docs
- easy to learn
- support
- community
- hybrid app development
THE WINNER IS

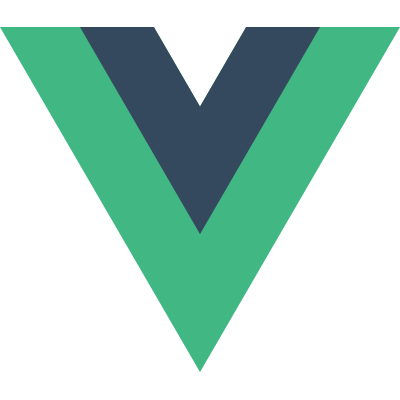

Our concerns were

- One man show?
- Immature?
- Docs?
- Hybrid app?
- Native app?
- Community?
- Speed?
- How to convince mgmt?
The Javascript Family

Family and relatives:
Dad Angular JS
Son React JS
Son Vue JS
Fat Uncle Ember Js
Unpopular Uncle PolymerJS
Grandpa jQuery
PERSONAS

Angular JS
70 y.o. overweight dad
Has his hands in a lot of projects
A bit slow, fixated on his own traditions
Likes stability, dislikes other families approaches
Popular
Can not stop thinking that Grandpa jQuery was right

PERSONAS

React
30 y.o. first rebel son
Hipster attitude but with a lot of friends
Drinks a (gluten free) pumpkin flavoured mocaccino
Refuses all the teaching coming from his Dad but in his rebellion discovered a lot of cool new approaches

PERSONAS

Vue Js
18 y.o. .late son
Learned from dad's mistakes
Ready to embrace his older brothers cool ideas
Too busy studying to make friends during childhood but of increasing popularity
Has a hard time stepping out of his popular brother's shadow

PERSONAS

The Question:
Now that grandpa jQuery is ready to retire, what will be the name written on his will ? Who will inerith his legacy?

SPEED

Comparisons

Angular | React | VueJs | |
---|---|---|---|
Docs | 2 | 3 | 5 |
Size | 143 kb | 43kb | 23kb |
Speed | 3 | 3 | 4 |
Memory | 3 | 3 | 4 |
Learning Curve | 2 | 2 | 5 |
3rd party libs | 5 | 4 | 3 |
Stability / support | LTS | Frozen API | Semver |
Upgrade | 1 | 5 | 3 |
Community | 5 | 5 | 3 |
Jobs | 4 | 5 | 2 |
Flexibility | 2 | 3 | 5 |
Native | 4 (NativeScript) | 5 (ReactNative) | 2 (Weex) |
Hybrid | 5 ( Ionic) | 5 (ReactNative) | 5 (Quasar) |
Project comparisons

D.H.A (A) | Malibu (R) | IMP (V) | |
---|---|---|---|
Size | Big | Small | Big |
Speed | 3 | 2 | 5 |
# Refactor | 1 | 5 | 5 |
# Bugs | 2 | 3 | 4 |
State Mgmt. | N/Y (Redux) | N | Y (Vuex) |
UI Speed | 3 | 5 | 5 |
Js Version | ES6 | ES6 | ES6 |
SSR / SEO | N | Y | Y |
WHEN, WHO ?

- ❤ Typescript: A
- OOP: A
- Big Projects: R || V
- Small Projects: V
- Rely on 3rd party: A || R
- Native: R
- Sitebuild: V
- Performance: V
- All-in-one: A
- Learning Curve: V
- TDD: A || R || V
- Clean Code: V

"NaNNaNNaNNaNNaNNaNNaN"



About Quasar framework
A framework for building web apps,
PWAs, hybrid mobile apps (Cordova) or Electron apps
with the same codebase
based on VUE 2.0
The Ionic of VUE

PWA - Progressive Web Applications
Google's 2 cents on
how to make web apps from now on
- Works offline ( service workers )
- Installable to home screen ( manifest.json, icons )
- Push notifications ( 3rd party )
- under 3s load time, animations ...
- Google Lighthouse

Cordova
Open source tool to build iOS & Android apps
- that use Html & Js & Css inside (via webView)
- has connection to native features via plugins
- core plugins have good documentations, but...
- still need xCode, java & Android SDK
- platform version changing can affect plugins
2 platforms, one codebase,
maybe some plugins & functionality "if"-ed

How does it fit into the ecosystem?


How does it fit into the ecosystem?

Hopefully not gonna see:
// 要做:
vuejs.org team section

quasar-cli
- global npm package
- based on Webpack
- all loaders configured, base ones inside package.json
- comes with BABEL
- sets up the project for you
folder structure & build tools - quite aggressive ESLint setup
- well done, start taking a look, and customize it

UI & Styling in Quasar
- baked in Stylus as CSS preprocessor
global variables used within Quasar components - material design UI component library
inputs, buttons, layouts, sidebars, tabs...
under the hood: .vue components - helper classes (flex, platform-specific & syntactic sugar)
- two default themes: ios & mat

Quasar Ionic
31,781 ⭐️ vs 3.796 ⭐️
- Ionic focuses solely on hybrid apps
- Ionic tries keeping up with Angular versioning
1, 2... 4 - Ionic View VS Quasar Play
- Ionic ecosystem
- Ionic 4 is gonna ship with standard web components
we'll able to use VUE inside

Latest improvements in Quasar
- v0.13 => v0.14
- tree-shaking, like _
- improved components
- PWA capabilities
- improved documentation

Why did we go with it?
worked with Ionic & Cordova before
Angular fatigue
VUE inside
needed a motivation pill after Polymer
something new & familiar at once
Well around framework, not only for web apps

Tricks & tips & best practices I: VUE
- API? Axios, vue-axios
- state? vuex
- getters > props
- local data? vuex-persistedstate
auth, multilang, offline - layer out libraries
axios, moment
network error handling, common function lib - scope Css in templates
- know your lifecycles!

data magic
DOM magic

Tricks & tips & best practices II: Quasar
- separate platform-dependent code
- q-pull-to-refresh
- q-ajax-bar
- q-inline-datetime
- always look out for frameworks own solutions
.styl variables, classes, layout

Tricks & tips & best practices III: Cordova
- Separate cordova plugin code
- researching the right one
- prepare for tedious testing of plugins
- cordova-plugin-camera
- cordova-plugin-file-opener2
- cordova-plugin-file-transfer
- phonegap-plugin-barcodescanner
-
cordova-plugin-downloadmanager
-
com.jcesarmobile.filepicker (iOS)
& com.cesidiodibenedetto.filechooser (Android)

Questions?

Thank you!
What's next
http://bit.ly/2x7Fpiw

Thank you!

https://slides.com/gyorgyfekete/its-not-me-its-vue
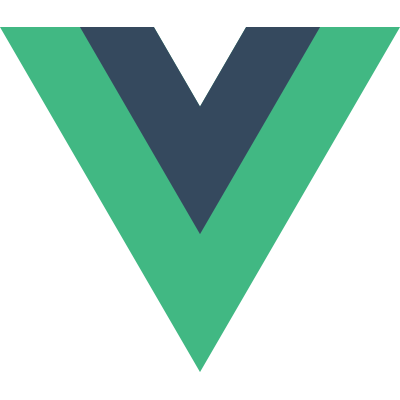
It's not me it's VUE
By don_nico
It's not me it's VUE
VUE.JS MEETUP BUDAPEST
- 645