Introduction to Node.js
JS u browser-u
JS u browser-u

JS izvan browsera

JS izvan browser-a
libuv
Generating a basic web server using Node.js and npm
Web Server
A web server is software designed to respond to requests over the internet by loading or processing data

HTTP - Hypertext transfer protocol
- Web servers follow Hypertext Transfer Protocol (HTTP)
- HTTP is a protocol which allows the fetching of resources
- such as HTML documents
- foundation of any data exchange on the Web
- client-server protocol
- requests are initiated by the recipient, usually the Web browser
GET and POST
- One way that a client and server communicate is through HTTP verbs
- Verbs indicate what type of request is being made
- the user is trying to load a new web page
- the user is updating information in their profile page
- Two most widely used HTTP methods are GET and POST

Request-response cycle

Creating a Node.js module
Node.js modul
- A Node.js application is made up of many JavaScript files
- Each JavaScript file or folder containing a code library is called a module
JavaScript objects and adding properties
export object and require function
Constructing a Node.js application with npm
npm
- npm - a package manager for Node.js
- npm is responsible for managing the external packages
- install
- remove
- modify
npm

npm
-
npm install <package>
- --save
- --global
-
npm install <package>
- -S
- -g
-
npm install <package>
- --save-dev
- --save-prod

Installing a Node.js package with npm
package.json
- Every Node.js application or module contains a package.json file
- defines the properties of the project
- positioned at the root level of the project
- specifies:
- the version of current release
- the name of application
- the main application file
package.json
{
"name": "recipe_connection",
"version": "1.0.0",
"description": "An app to share cooking recipes",
"main": "main.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "Jon Wexler",
"license": "ISC",
}
Instalacija paketa
npm install cities --save
{
"name": "recipe_connection",
"version": "1.0.0",
"description": "An app to share cooking recipes",
"main": "main.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "Jon Wexler",
"license": "ISC",
"dependencies": {
"cities": "^2.0.0"
}
}
Instalacija paketa

Processing request and generating response
JavaScript functions
- Function is a "subprogram" that can be called by code external (or internal in the case of recursion) to the function
- In JavaScript, functions are first-class objects
- they can have properties and methods like any object
- what distinguishes them from other objects is that functions can be called
- they are Function objects.
JavaScript arrow functions
- Arrow functions are anonymous functions with their own special syntax that accept a fixed number of arguments, and operate in the context of their enclosing scope - i.e. the function or other code where they are defined.
=>
HTTP Status Codes
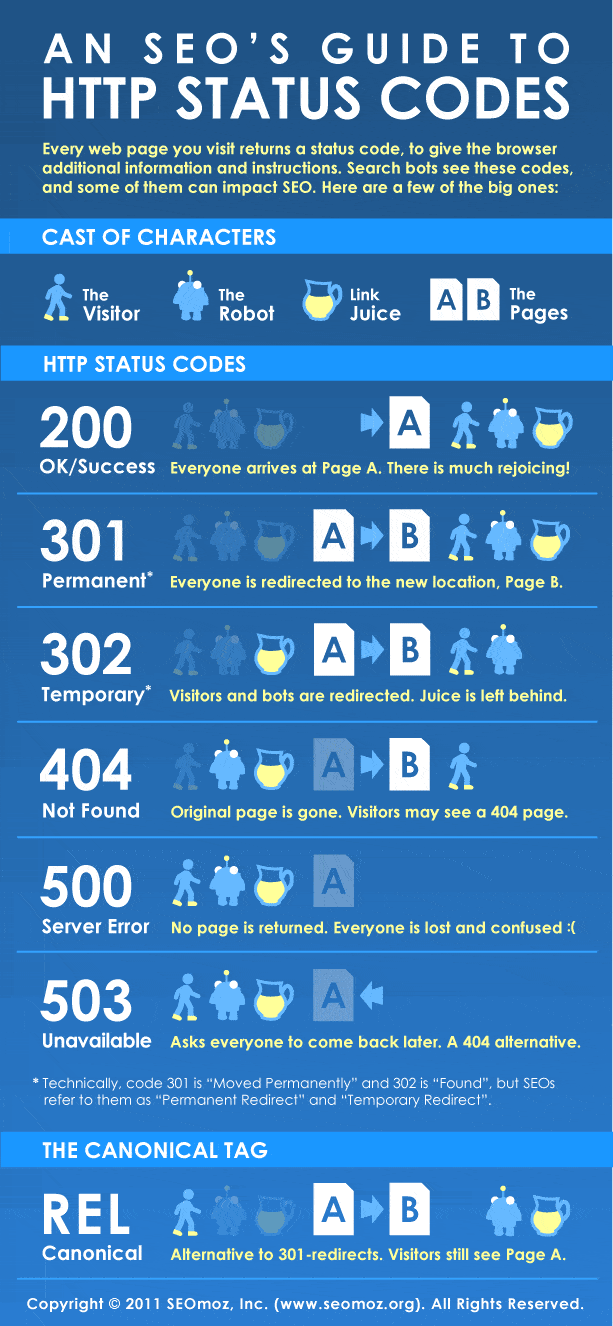

Callback function
- A callback is an anonymous function that’s set up to be invoked as soon as another function completes
- benefit of using callbacks is that server
doesn’t have to wait for the original function to complete processing before running other
code
- benefit of using callbacks is that server
Callback function

Running a web server in your browser
node main.js
Odabrana poglavlja - P02
By Elmedin Selmanovic
Odabrana poglavlja - P02
- 344