JavaScript Crash Course
Цикли
5
Зміст:
- Що таке цикл?
- Цикл for
- Цикл while
- Цикл do...while
- Використання циклів
Що таке цикл?
Коротко про цикли
Цикл — різновид керівної конструкції у високорівневих мовах програмування, призначена для організації багаторазового виконання набору інструкцій (команд).
Також циклом може називатися будь-яка багатократно виконувана послідовність команд, організована будь-яким чином (наприклад, із допомогою умовного переходу).
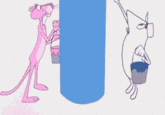
Коротко про цикли
Блок-схема цикла
Серія команд
true
false
Умова
Створення лічильника
Зміна лічильника
Приклад :)
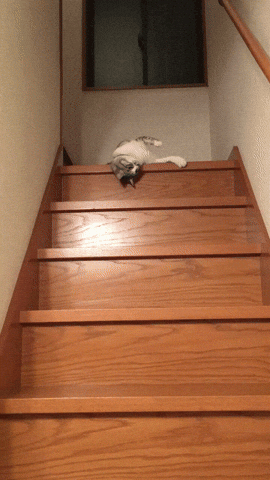
Для проходження сходинок, потрібно:
- визначити кількість повторень (ітерацій)
- описати інструкцію (спукс на сходинку нижче)
- додати умову, щоб вийти із цикла при досягненні фінальної точки
Цикл for
Цикл for
Серія команд
true
false
Умова
Створення лічильника
Зміна лічильника
Складові цикла for
for (let i = 0; i < 10; i++) {
// do something per itteration
}
декларація ітератора
умова
зміна ітератора (збільшення/зменшення
інструкції
ключове слово
Як створити цикл for
let sum = 0;
// in the loop we will find a sum of nums from 0 to 10
for (let i = 0; i <= 10; i++) {
sum = sum + i;
}
console.log('sum of nums from 0 to 10 is ', sum);
Знаходження суми
Цикл for для роботи з масивами
const nums = [1, 2, 3, 4, 5, 6];
let sum = 0;
// in the loop we will find a sum of nums array items
for (let i = 0; i < nums.length; i++) {
sum = sum + nums[i];
}
console.log('sum of nums items is ', sum);
Знаходження суми чисел масиву
Цикл for для роботи з масивами
const words = ['May', 'the', 'Force', 'be', 'with', 'you'];
let sentence = '';
// in the loop we will find a sum of nums array items
for (let i = 0; i < words.length; i++) {
sentence += words[i] + ' ';
}
console.log(sentence);
Знаходження рядка із слів в масиві
Цикл for для роботи з масивами
const words = ['you', 'with', 'be', 'Force', 'the', 'May'];
let sentence = '';
// in the loop we will find a sum of nums array items
for (let i = words.length - 1; i >= 0; i--) {
sentence += words[i] + ' ';
}
console.log(sentence);
Знаходження рядка із слів в масиві в зворотньому порядку
Цикл for...in для роботи з об'єктами
const obj = {
one: '1',
two: '2',
three: '3',
};
let sentence = '';
// in the loop we will find a sum of nums array items
for (let key in obj) {
sentence += key + '; ';
}
console.log(sentence);
Створення рядка із ключів об'єкта
Цикл while
Цикл while
Серія команд
Умова
true
false
Складові цикла while
while (n < 3) {
// instructions
}
умова
інструкції
ключове слово
Як створити цикл while
let n = 0;
while (n < 3) {
n++;
}
console.log(n);
Знаходження суми
Цикл while для роботи з масивами
const nums = [1, 2, 3, 4, 5, 6];
let n = 0;
let sum = 0;
// with the loop we will find a sum of numbers in the array
while (n < nums.length) {
sum = sum + nums[n];
n++;
}
console.log('sum of nums items is ', sum);
Знаходження суми чисел масиву
Цикл while для роботи з масивами
const words = ['May', 'the', 'Force', 'be', 'with', 'you'];
let sentence = '';
let n = 0;
// with the loop we will find a sum of numbers in the array
while (n < words.length) {
sentence += words[n] + ' ';
n++;
}
console.log(sentence);
Знаходження рядка із слів в масиві
Цикл do...while
Цикл do...while
Серія команд
true
false
Умова
Складові цикла do...while
do {
// instructions
} while (n < 3)
умова
інструкції
ключове слово
Цикл do...while для математичних підрахунків
let n = 5;
let result = 1;
// with the loop we will find n!
do {
result *= n;
n--;
} while (n >= 1)
console.log(result);
Знаходження значення факторіал числа n
Використання циклів
Отримання даних і їх використання
const data = [
{ user: 'John' },
{ user: 'Tom' },
{ user: 'Petr' },
];
// use data
function greeting(userName) {
return "Hello, " + userName + "!";
}
greeting(data[0].user);
greeting(data[1].user);
greeting(data[2].user);
// later we will use it together with loops :)
Рішення без цикла
Час покращити рішення :)
Отримання даних і їх використання
const data = [
{ user: 'John' },
{ user: 'Tom' },
{ user: 'Petr' },
];
// use data
function greeting(userName) {
return "Hello, " + userName + "!";
}
for (let i = 0; i < data.length; i++) {
console.log(greeting(data[i].user));
}
Рішення з циклом
Поєднання із об'єктами
const song = {
1: "We don't need no education",
2: "We don't need no thought control",
3: "No dark sarcasm in the classroom",
4: "Teacher, leave them kids alone",
5: "Hey, teacher, leave them kids alone",
6: "All in all, it's just another brick in the wall",
7: "All in all, you're just another brick in the wall",
8: "We don't need no education",
9: "We don't need no thought control",
10: "No dark sarcasm in the classroom",
11: "Teachers, leave them kids alone",
12: "Hey, teacher, leave them kids alone",
13: "All in all, it's just another brick in the wall",
};
const songText = song[1] + song[2] + song[3] +
song[4] + song[5] + song[6] +
song[7] + song[8] + song[9] +
song[10] + song[11] + song[12] + song[13];
console.log(songText);
Рішення без цикла
Час покращити рішення :)
Поєднання із об'єктами
const song = {
1: "We don't need no education",
2: "We don't need no thought control",
3: "No dark sarcasm in the classroom",
4: "Teacher, leave them kids alone",
5: "Hey, teacher, leave them kids alone",
6: "All in all, it's just another brick in the wall",
7: "All in all, you're just another brick in the wall",
8: "We don't need no education",
9: "We don't need no thought control",
10: "No dark sarcasm in the classroom",
11: "Teachers, leave them kids alone",
12: "Hey, teacher, leave them kids alone",
13: "All in all, it's just another brick in the wall",
};
let songText = '';
for (let key in song) {
songText += song[key];
console.log(song[key]);
}
Рішення з циклом
Q & A
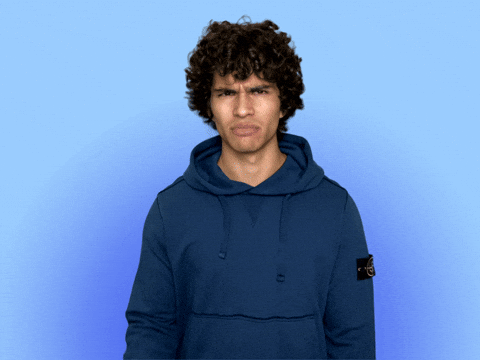
7 JavaScript Crash Course
By Inna Ivashchuk
7 JavaScript Crash Course
- 474