
WPGraphQL
Interacting with WordPress
data in a new way
WordCamp Phoenix 2018
Who Am I?
- Senior WordPress Engineer at Digital First Media
- Denver Native
- WordPress Developer for 9+ years
- Creator / Maintainer of WPGraphQL
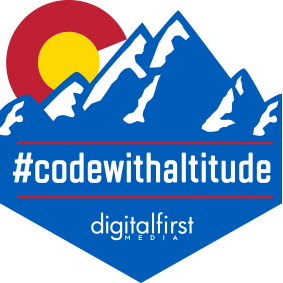

What this talk will cover
Intro to GraphQL
Using WPGraphQL
GraphQL
A Query Language for your API
GraphQL is a query language for APIs and a runtime for fulfilling those
queries with your existing data. GraphQL provides a complete
and understandable description of the data in your API, gives
clients the power to ask for exactly what they need
and nothing more, makes it easier to evolve APIs over time,
and enables powerful developer tools
GraphQL
Graph != Graph Databases
Graph != Graph Search
Graph = Application Data Graph
QL = Query Language
GraphQL: A Query Language for Your Application Data Graph
Post
Category
Category
Category
Post
title
"Hello World"
title
"GoodBye Mars"
Image
Image
Image
name
"news"
name
"crime"
name
"sports"
Image
GraphQL
GraphQL lets us pick trees out of the Application Data Graph
GraphQL
query {
post(id: "...") {
title
link
coauthors {
name
}
}
}
{
data: {
post: {
title: "Hello World!"
link: "http://site.com/hello-world"
coauthors: [
{
name: "John Doe",
},
{
name: "Jane Smith",
}
]
}
}
}
GraphQL
Post
CoAuthor
CoAuthor
CoAuthor
Post
Featured
Image
title
"Hello World"
title
"GoodBye Mars"
Avatar
Avatar
Avatar
name
"jane doe"
name
"sue smith"
name
"john doe"
query {
posts {
edges {
node {
}
}
}
}
author {
name
avatar(size: 50) {
url
}
}
followLink
title
featuredImage(width: 300) {
url
}
excerpt
site {
name
link
}







GraphQL

WPGraphQL
A Query Language for your WordPress API
GraphQL
A Query Language for your API
GraphQL is a query language for APIs and a runtime for fulfilling those
queries with your existing data. GraphQL provides a complete
and understandable description of the data in your API, gives
clients the power to ask for exactly what they need
and nothing more, makes it easier to evolve APIs over time,
and enables powerful developer tools
WPGraphQL is an extendable open-source WordPress plugin that brings the power of GraphQL to your WordPress install. WPGraphQL defines GraphQL Types for core WordPress entities, such as posts, terms and users, and defines core entry points into the WordPress Application Data Graph via GraphQL Queries and Mutations. WPGraphQL gives clients the power to ask for exactly what they need from WordPress and enables powerful developer tools.


- Download the plugin from Github
- Add to your WordPress install
- /wp-content/plugins
- Activate the plugin
- Flush the permalinks
Using the Plugin

Tools to Run Queries
WPGraphiQL
https://github.com/wp-graphql/wp-graphiql
Download, install & activate plugin, navigate to GraphiQL in the WordPress Dashboard

-
explore GraphQL Documentation
-
query a list of posts
-
query nested resources
-
query multiple root resources
-
using the node query
-
using query fragments with GraphQL
-
using aliases with GraphQL
-
use variables with GraphQL
-
queries with variables and pagination
-
mutation - createPost
Exploring WPGraphQL

GraphQL Docs
IDE Support
GraphQL Tooling

Let's build a plugin!
Extending WPGraphQL
<?php
/**
* Plugin Name: WPGraphQL WordCamp Publishers Extension
* Description: Plugin that extends WPGraphQL
*/
- Create a new directory
- /plugins/wpgraphql-wordcamp-publishers
- Create a new plugin file
- wpgraphql-wordcamp-publishers.php

Add a Root Entry Point
Extending WPGraphQL


Add a Root Entry Point
Extending WPGraphQL
add_action( 'graphql_root_queries', function( $fields ) {
$fields['wordCampRocks'] = [
'type' => \WPGraphQL\Types::string(),
'description' => __( 'An example field showing how to add
to the root schema', 'wp-graphql-publishers' ),
'resolve' => function() {
return 'Yes, it does';
},
];
return $fields;
} );

Add a Field to the Post Schema
Extending WPGraphQL


Add a Field to the Post Schema
Extending WPGraphQL
add_action( 'graphql_post_fields', function( $fields ) {
$fields['color'] = [
'type' => \WPGraphQL\Types::string(),
'description' => __( 'An example showing how to add a
field to the "post" schema', 'wp-graphql-publishers' ),
'resolve' => function( \WP_Post $post ) {
return get_post_meta( $post->ID, 'color', true );
},
];
return $fields;
}, 10, 1);

Add Custom Post Type to the Schema
Extending WPGraphQL


Add Custom Post Type to the Schema
Extending WPGraphQL
add_action( 'init', function() {
register_post_type( 'book', [
'label' => __( 'Books', 'wp-graphql-publishers' ),
'supports' => [ 'title', 'editor', 'custom-fields' ],
'public' => true,
'show_in_graphql' => true,
'graphql_single_name' => 'book',
'graphql_plural_name' => 'books',
] );
} );

Add Custom Taxonomy to the Schema
Extending WPGraphQL


Add Custom Taxonomy to the Schema
Extending WPGraphQL
add_action( 'init', function() {
register_taxonomy( 'genre', 'book', [
'label' => __( 'Genre' ),
'public' => true,
'show_in_graphql' => true,
'graphql_single_name' => 'genre',
'graphql_plural_name' => 'genres',
'hierarchical' => true,
]);
} );

Add a Custom Field with Mutation Support
Extending WPGraphQL


Add a Custom Field with Mutation Support
Extending WPGraphQL
add_action( 'graphql_book_fields', function( $fields ) {
$fields['price'] = [
'type' => \WPGraphQL\Types::string(),
'description' => __( 'The price of the book', 'wp-graphql-publishers' ),
'resolve' => function( \WP_Post $book ) {
$price = get_post_meta( $book->ID, 'price', true );
return ! empty( $price ) ? $price : null;
},
];
return $fields;
}, 10, 1);
1. Add the field to the type

Add a Custom Field with Mutation Support
Extending WPGraphQL
add_action( 'graphql_post_object_mutation_input_fields', function( $fields, \WP_Post_Type $post_type_object ) {
if ( 'book' === $post_type_object->name ) {
$fields['price'] = [
'type' => \WPGraphQL\Types::string(),
'description' => __( 'The price of the book', 'wp-graphql-publishers' ),
];
}
return $fields;
}, 10, 2 );
2. Add the input field to the mutation input

Add a Custom Field with Mutation Support
Extending WPGraphQL
add_action( 'graphql_post_object_mutation_update_additional_data', 'prefix_update_price', 10, 3 );
function prefix_update_price( $post_id, $input, \WP_Post_Type $post_type_object ) {
if ( 'book' === $post_type_object->name && ! empty( $input['price'] ) ) {
update_post_meta( $post_id, 'price', $input['price'] );
}
}
3. Add the input field to the mutation input

Add a Custom Field with Mutation Support
Extending WPGraphQL
add_action( 'graphql_post_object_mutation_input_fields', function( $fields, \WP_Post_Type $post_type_object ) {
if ( 'book' === $post_type_object->name ) {
$fields['price'] = [
'type' => \WPGraphQL\Types::string(),
'description' => __( 'The price of the book', 'wp-graphql-publishers' ),
];
}
return $fields;
}, 10, 2 );
2. Add the input type
WCPHX: WPGraphQL - Interacting with WordPress Data in a new Way
By Jason Bahl
WCPHX: WPGraphQL - Interacting with WordPress Data in a new Way
- 1,892