Intro to Programming
for Information and Data Science
Joel Ross
Spring 2025
LIS 511
Script for the Day
-
Introductions
-
Syllabus and course structure
-
What is Programming?
-
Hello Python!
-
Variables
-
First Exercise
Hi, I'm Joel Ross!
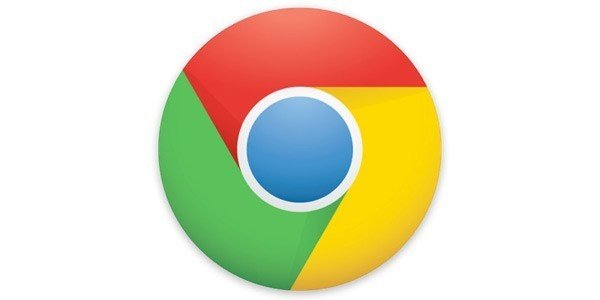
Associate Teaching Professor
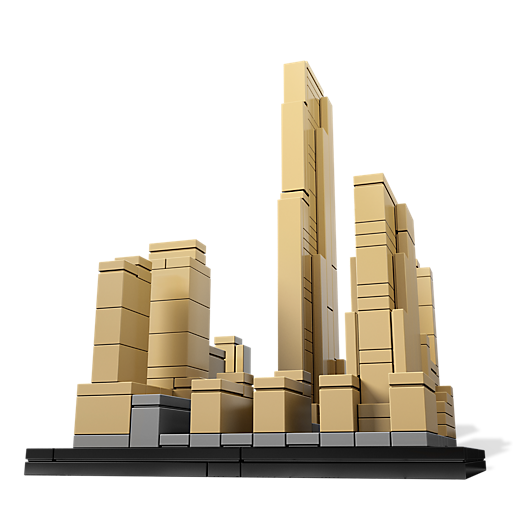
Email joelross@uw.edu
Zoom https://washington.zoom.us/my/joelross
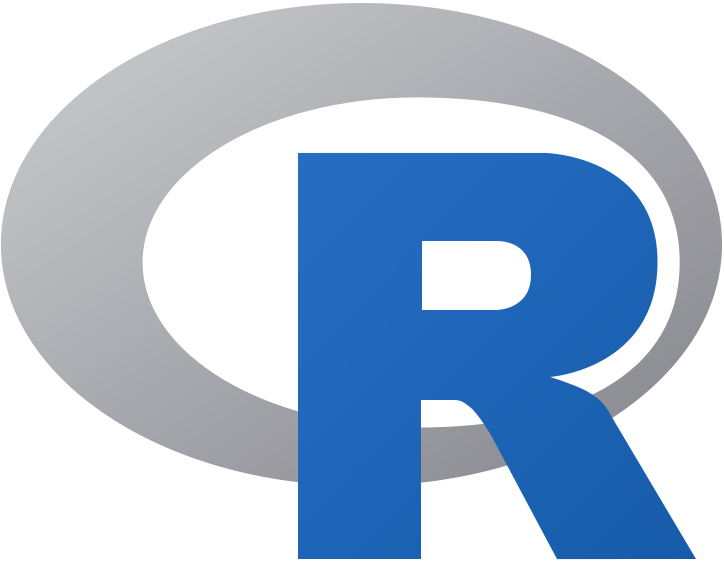
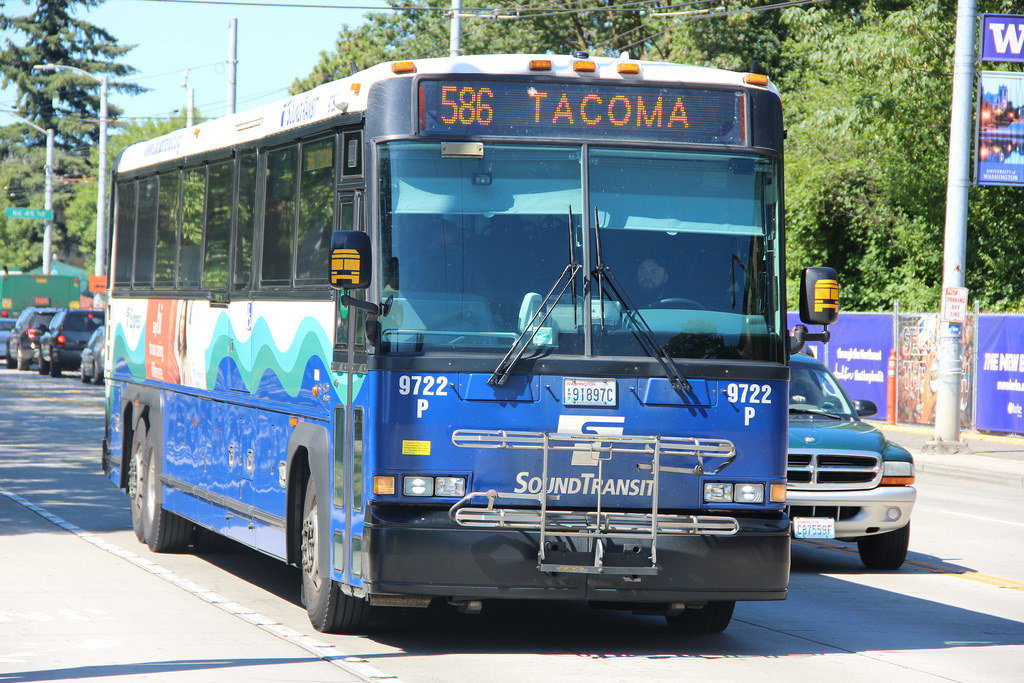
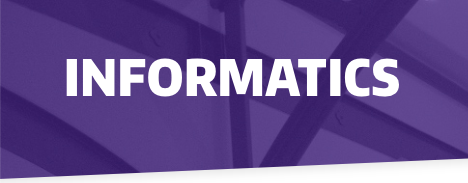
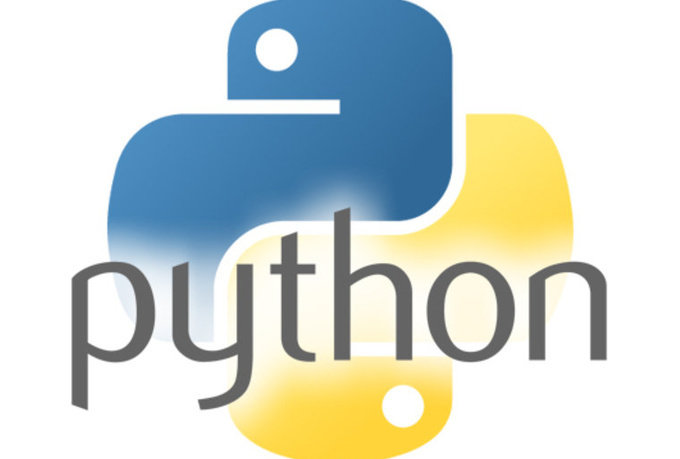
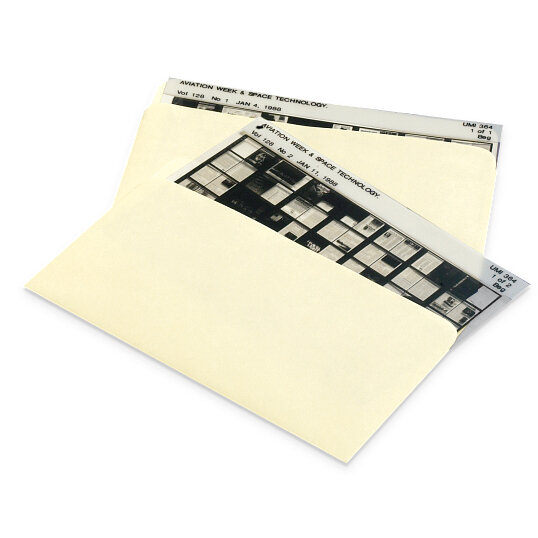
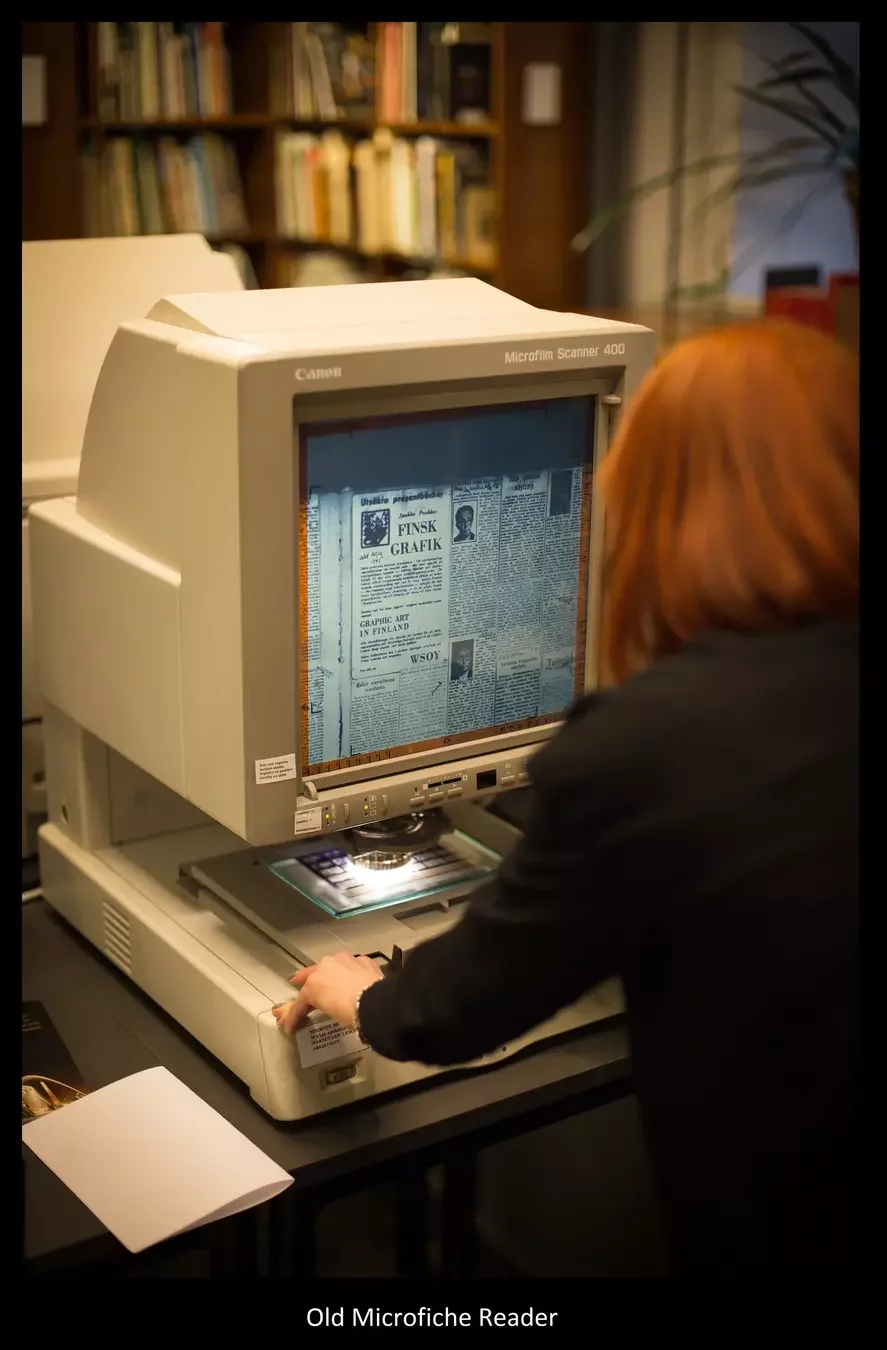
Introduce to yourself to your neighbor!
Meet someone new!
Meet and Greet!
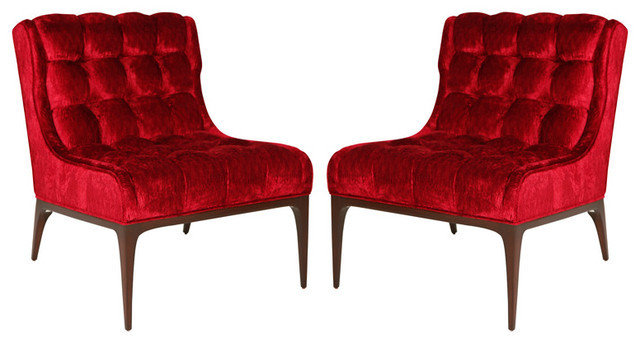
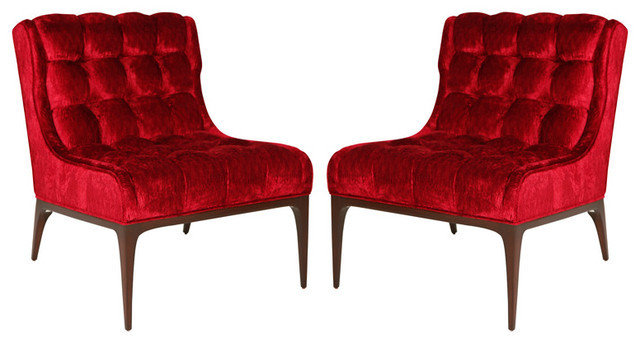
Everyone raise a hand
(Put it down again)
You are all capable of both speaking and raising your hand.
ASK LOTS OF QUESTIONS!
Canvas & Syllabus
This course is both
in-person and online!
In-Person & Online
In-person (section A):
- A somewhat "flipped" class: read text, watch videos at home; come to class to do work and activities
- Class meetings for review, questions, and working!
- Class sessions will be streamed and recorded
Online (section B):
- Online asynchronous course, move at your own pace!
- Welcome to attend the in-person sessions (remote in)
Flipped Class
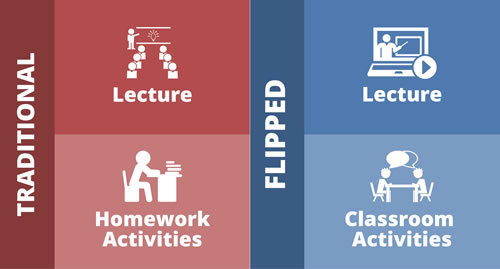
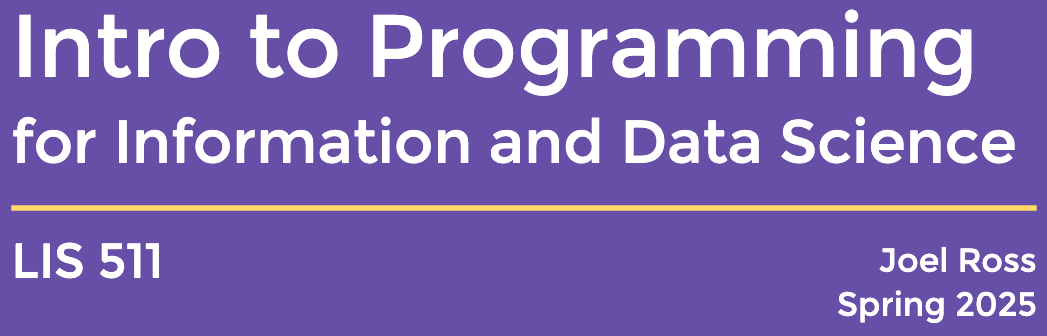
Course Modules
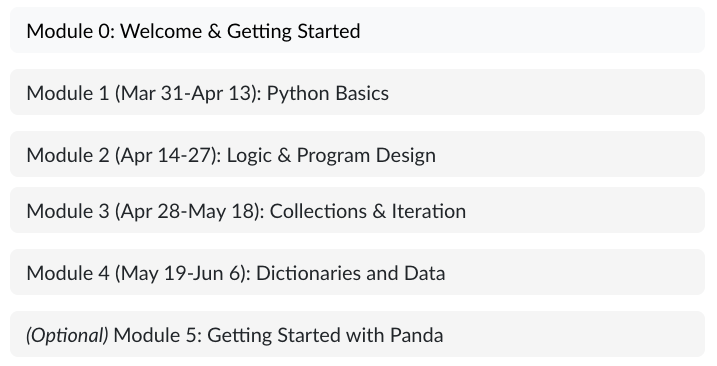
What we'll be learning
Programming
Writing instructions for a computer in a language it understands
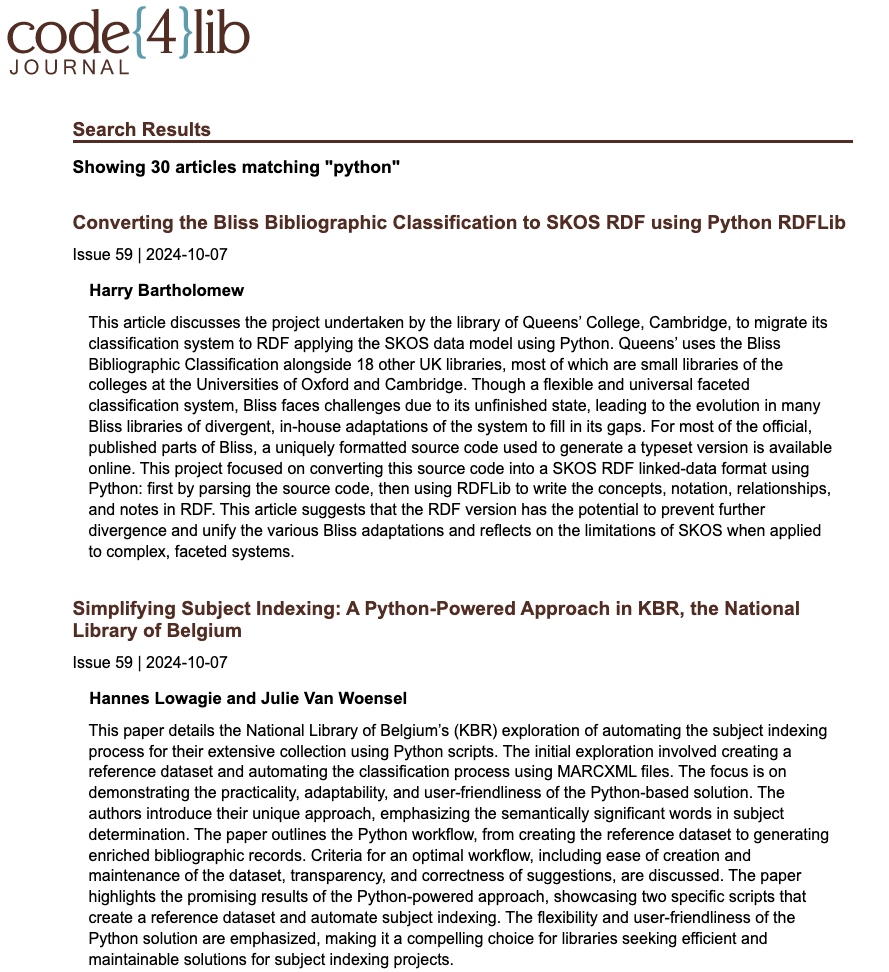
We need to convert
data
into
information
raw numbers
interpreted data
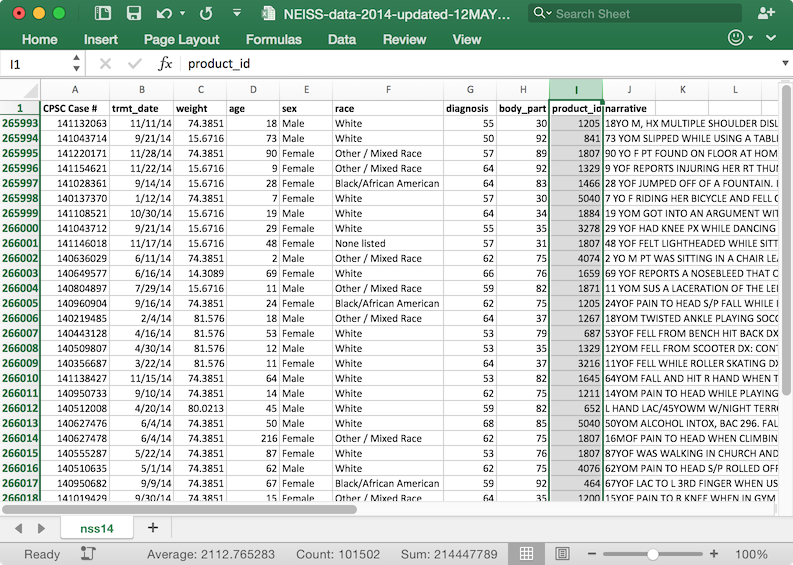
Which product is the most dangerous?
Algorithm
A set of step-by-step instructions for solving a problem.
Needs to be unambiguous, executable, and terminating
Should we do this by hand?
Slow
Tedious
Error-prone
How to scale?
How to reproduce?
Solution: get someone else to do the boring work for us!
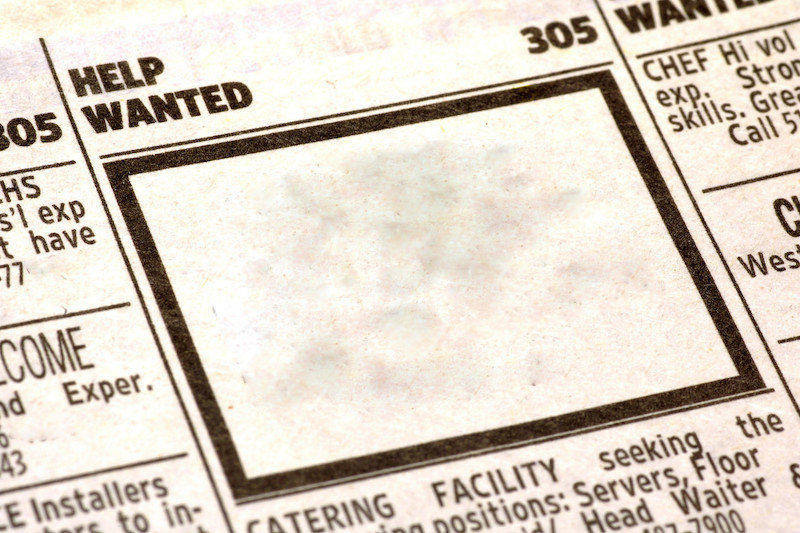
World's greatest assistant!
Our new assistant
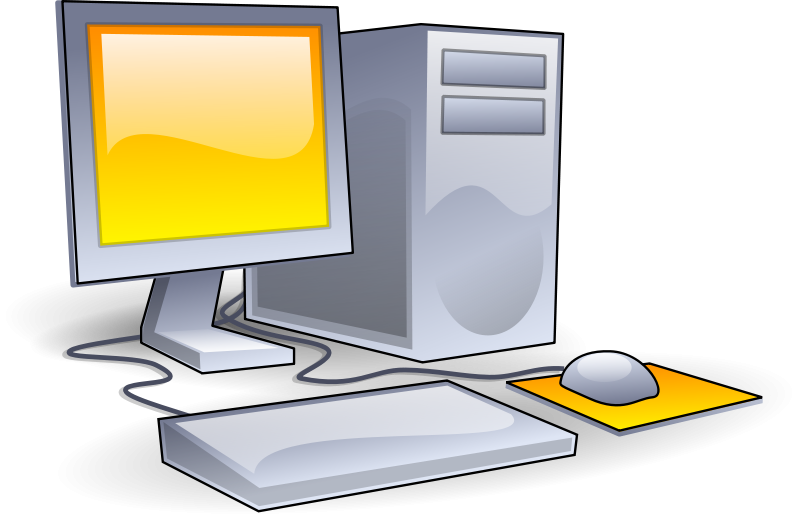
First Programmer:
Ada Lovelace (1815-1852)
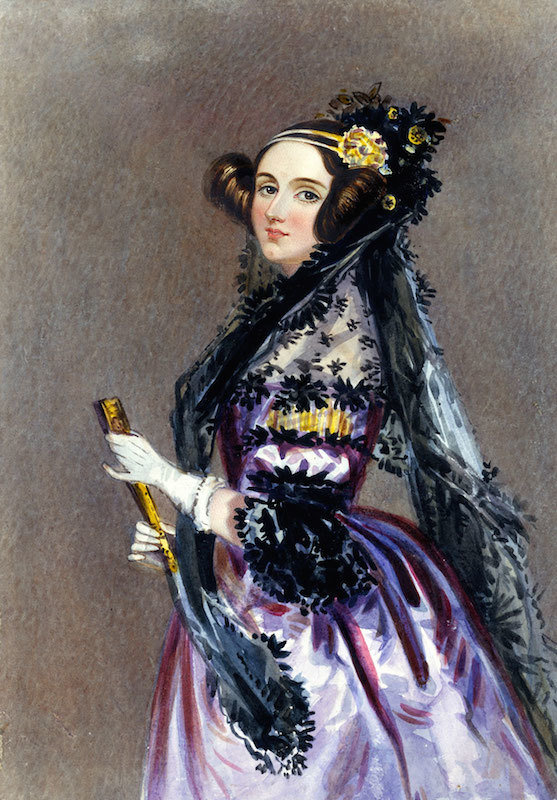
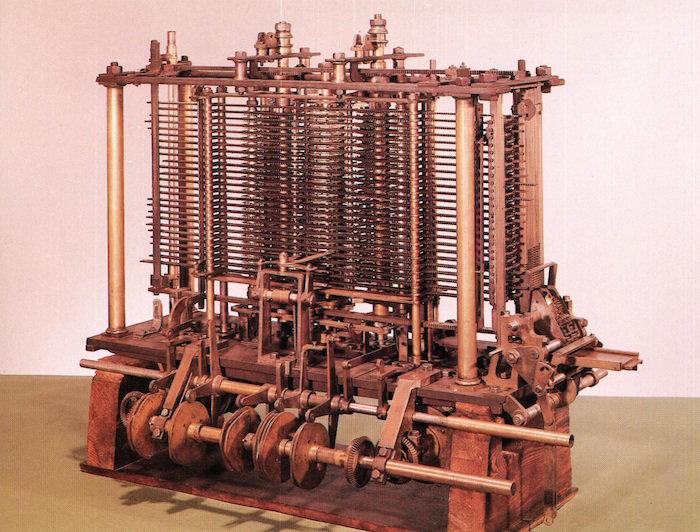
Charles Babbage's Analytical Engine (designed 1837; never built)
We need to tell the computer what to do!
Problem
Computers don't
speak English!
Programming
Writing instructions for a computer in a language it understands
Computers Speak Binary
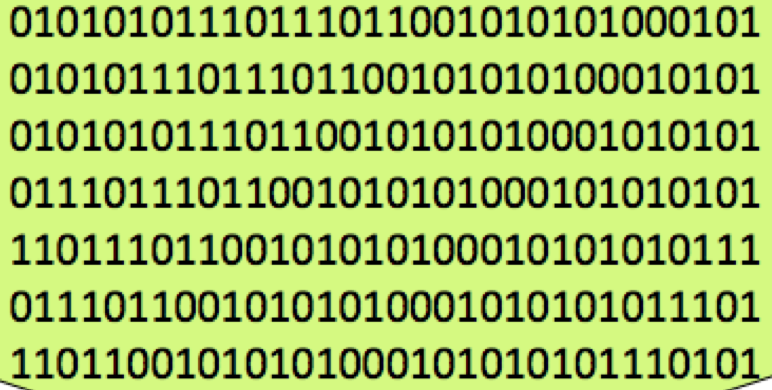
Computers just do math. That's it.
Programming Language
A language that a human can write, and which can be interpreted by a computer
(A formal language, not a natural one)
Example Code: Python!
# This is the programming language Python
# It does math! (Applies the quadratic formula)
import math
def roots(a, b, c):
det = math.sqrt(b * b - 4 * a * c)
x1 = (-b + det)/(2 * a)
x2 = (-b - det)/(2 * a)
return (x1, x2)
x = roots(1, 5, -14)
print(x)
Abstraction
The process of generalization; of working with higher-level representations rather than specific details
A necessary skill when programming
Course Objective
Learn to give instructions to computers so they do the boring stuff!
Programming
Syntax
"How do I tell the computer what to do?"
Algorithm
"What do I tell the computer to do?"
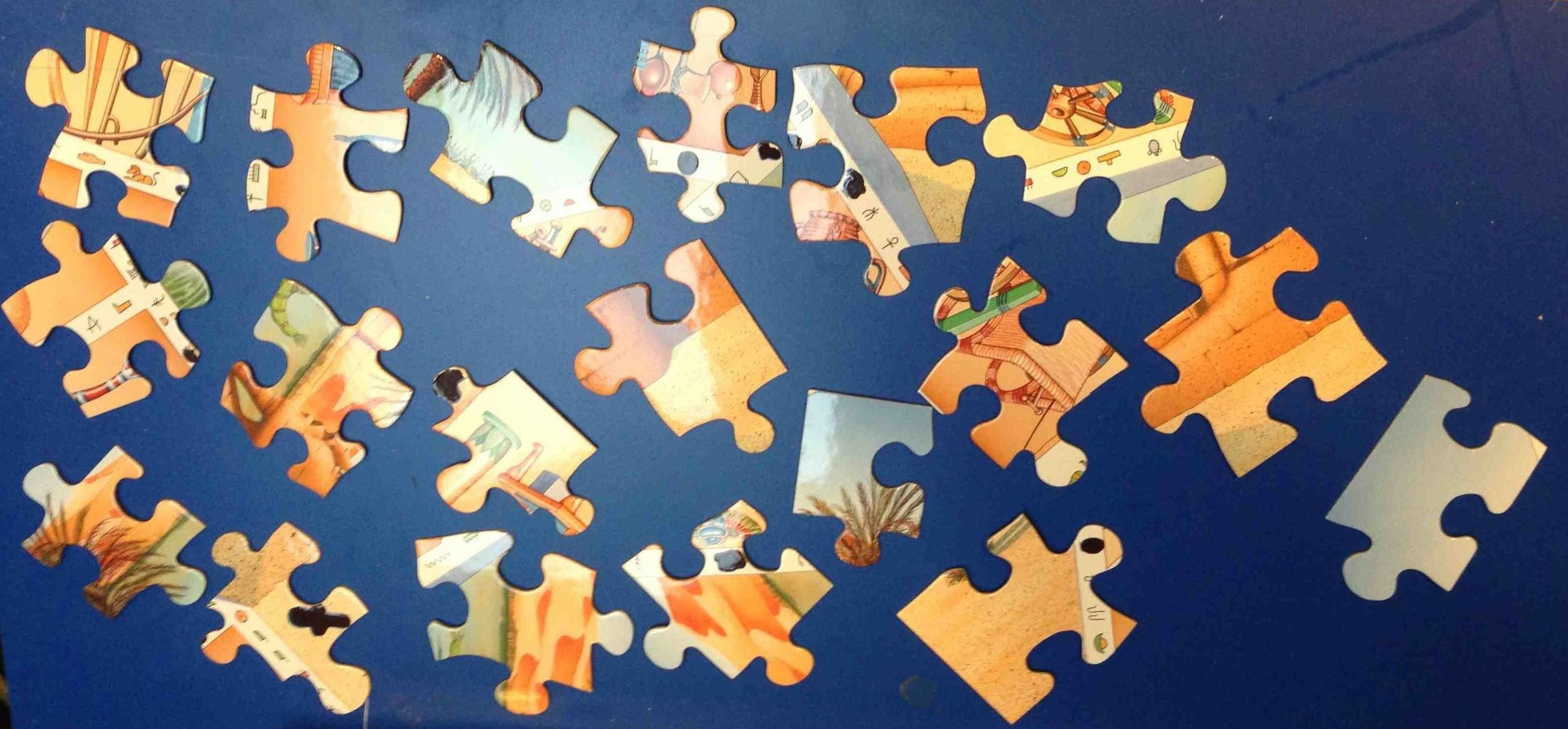
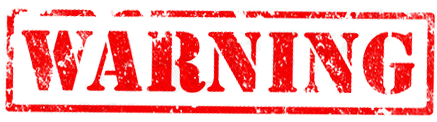
Programming involves a lot of failure and frustration
It's not your fault, and we are here to support you!
Any questions so far?
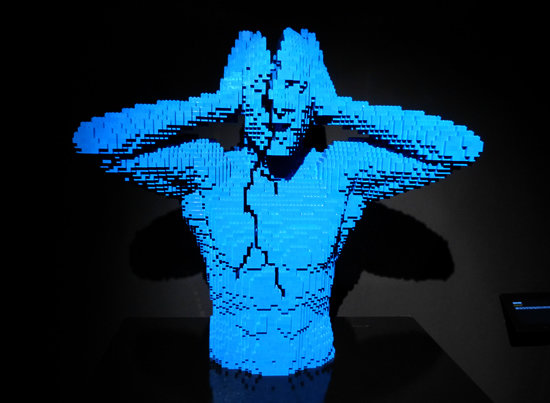
BREAK
Nathan Sawaya
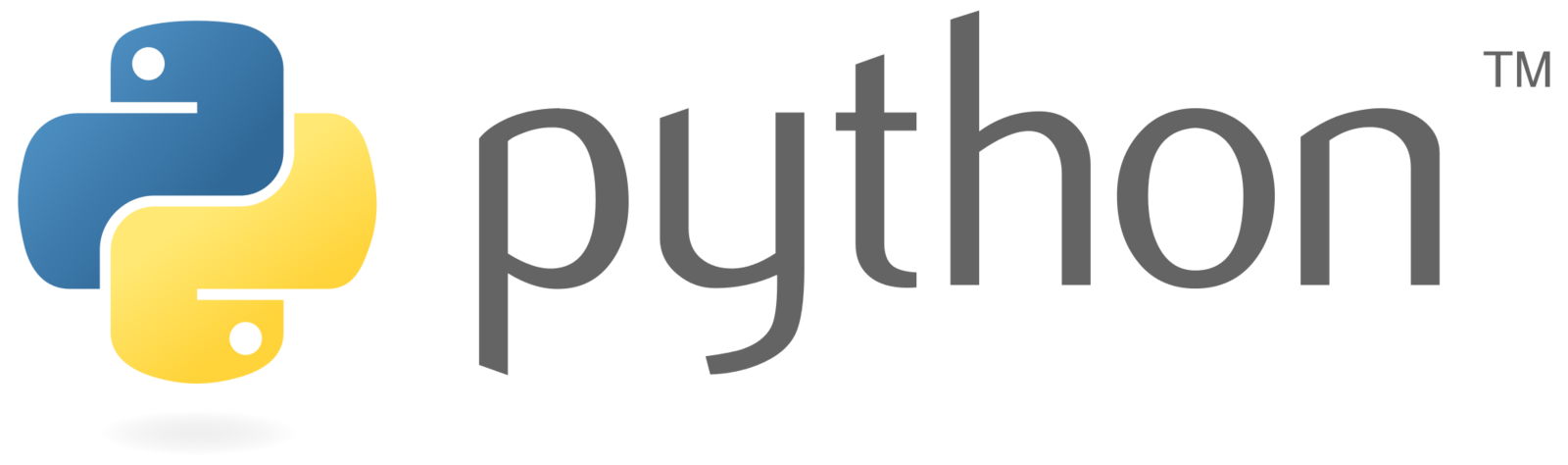
A high-level, general purpose, interpreted programming language
Jupyter Notebooks
Jupyter Notebooks are interactive web applications that can be used to write and execute Python programs (as well as textual content). Notebooks can also be shared with others!
We will do all our programming in Jupyter notebooks hosted on the Ed platform online.
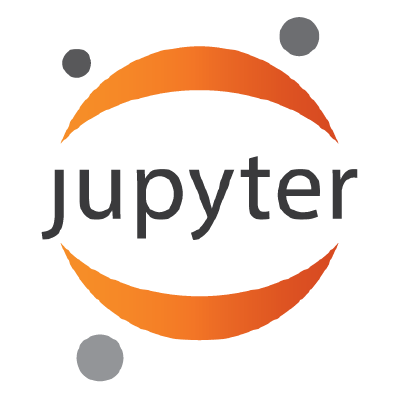
Using Jupyter
Access Jupyter notebooks on Ed
Type your code into a code cell.
-
shift + enter
to execute a cell
You can add additional cells, including text-only cells
- Do not add any cells in assignment notebooks! It will cause things to break
You can "restart" the interpreter if needed

# My first program
print("Hello world!")
Printing
Use
print()
to print whatever is in the parentheses to the console. This is an example of a
function.
What could go wrong?
# My first program
print "Hello world!"
# My first program
print("Hello world!)
# My first program
print("Hello wold!")
Python 2 syntax!
Computer Bugs
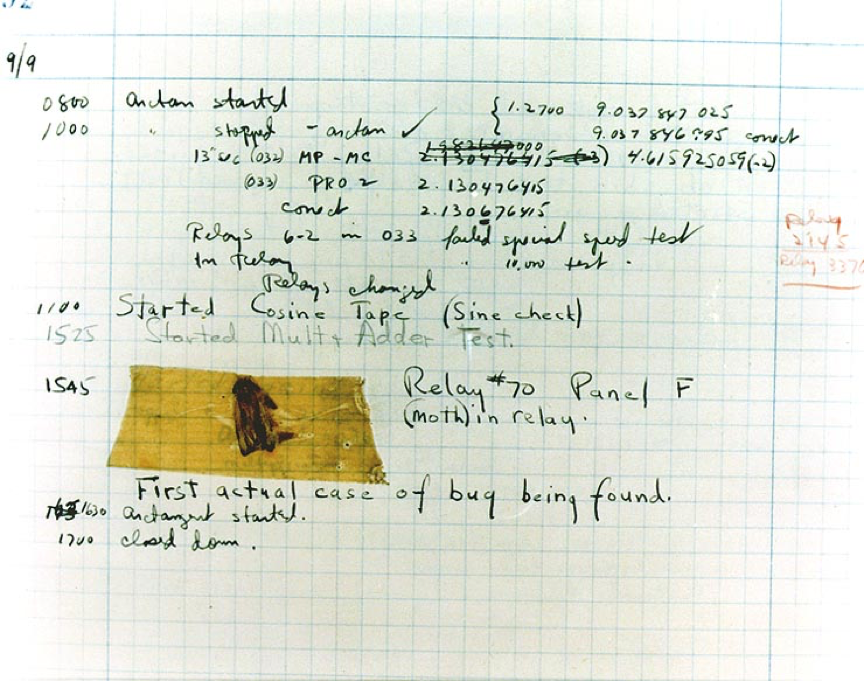
First computer bug (1946)
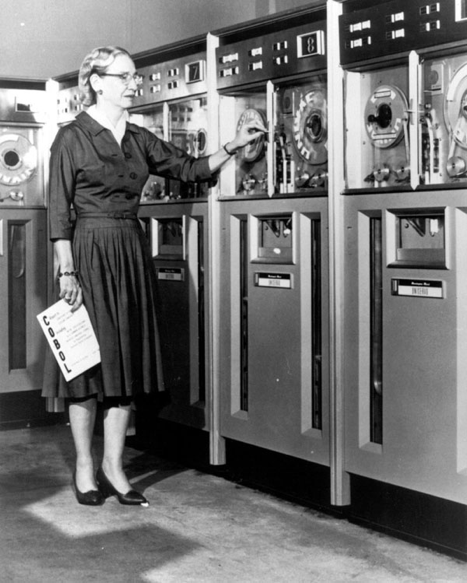
Admiral Grace Hopper
Types of Bugs
Syntax Error
Logical Error
Semantic Error
- An error in the use of the Python language. A problem with how you said something.
- Interpreter will error at the site of the problem.
- An error in your approach to solve a problem.
- Interpreter will not error, but will not do what you want.
- An error in the algorithm you used. A problem with what you said to do.
- Interpreter will error, but possibly after the problem.
-
Read the error message
-
"Play Computer"
-
Inspect intermediate values
-
Rubber duck debugging
-
Ask for help!
Some Debugging Tips
Variables
A label that refers to a value (data)
variable
(label)
value
(data)
assignment
num_cups_coffee = 3
When a label is on the left,
it means the variable (the nametag)
When a label is on the right,
it means the value (the data)
Assignment is
not Equality!
Assignment gives a label to a value. Changing which value a variable refers to doesn't change other variables.
x = 3
y = 4
x = y # assign the value of y (4) to x
print(x) # 4
y = 5
print(x) # 4
Naming Variables
# valid program, but what does it mean?
a = 35.0
b = 12.50
c = a * b
# much better!
hours = 35.0
pay_rate = 12.50 # needs a raise!
earnings = hours * pay_rate
# Get out.
x1q3z9ahd = 35.0
x1q3z9afd = 12.50
x1q3p9afd = x1q3z9ahd * x1q3z9afd
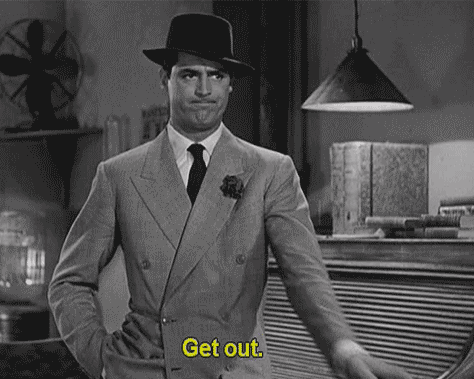
Good variable names describe what data they are labeling. Naming things is one of the hardest parts of computer programming.
Data Types
Numbers
Integers (whole numbers) and floats (decimals) are used to store numbers.
x = 2 # whole number
y = 3.5 # decimal (floating point) number
# can perform mathematical operations
z = x + y
# Use parentheses to enforce order of operations
z = 3*(x-y)**2
operator
operands
If an operand is a
float
or you use the division (/
) operator, the result will be a float.
Strings
Strings of characters (letters, punctuation, symbols, etc). Written in single or double quotes.
my_name = "Joel" # 'Joel' would be equivalent
# Can include any keyboard symbol (and more!)
course = "LIS 511: Intro to Programming!"
# Can concatenate strings together
greeting = "Hello" + " " + "World"
# Convert a number to a string to concatenate it
course_code = "LIS " + str(511)
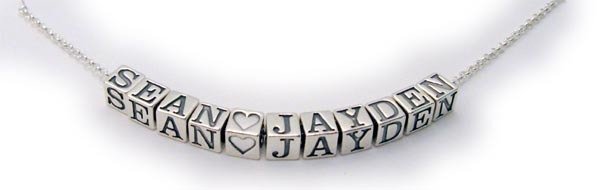
Exercise 1.1: Variables
Your Turn!
Go to Ed and open up Exercise 1.1: Variables. Give it a try, and if you have questions let me know!
Action Items!
- Complete Module 0
- Keep working on Module 1
- Complete Section 1.1
- Start Section 1.2. Do steps 1-2 (through "calling function")
- Bring questions/etc to next meeting!
Next time: functions!
lis511sp25-introduction
By Joel Ross
lis511sp25-introduction
- 65