Webserver Programming I
Info 253a: Web Architecture
Kay Ashaolu
What is a server?
- A computer program or a device that provides functionality for other programs or devices, called "clients"
- Servers can provide various functionalities, often called "services", such as sharing data or resources among multiple clients, or performing computation for a client
- A single server can serve multiple clients, and a single client can use multiple servers
Server = "computer program"
- A server is technically not the computer that runs this computer program.
- A server is a program that is hosted by a computer (called a host)
- We will learn how to write and run these programs to service "clients"
- Example of clients include desktop browsers, mobile browsers, curl (via the terminal)
The Request-Response model
- Client sends a request to the Server, Server responds back with data and typcially an acknolwedgement of receipt
- The Server has declared specific paths (called routes) that when accessed would execute specific functions
- Functions would return a response, which is sent to the Client
Review
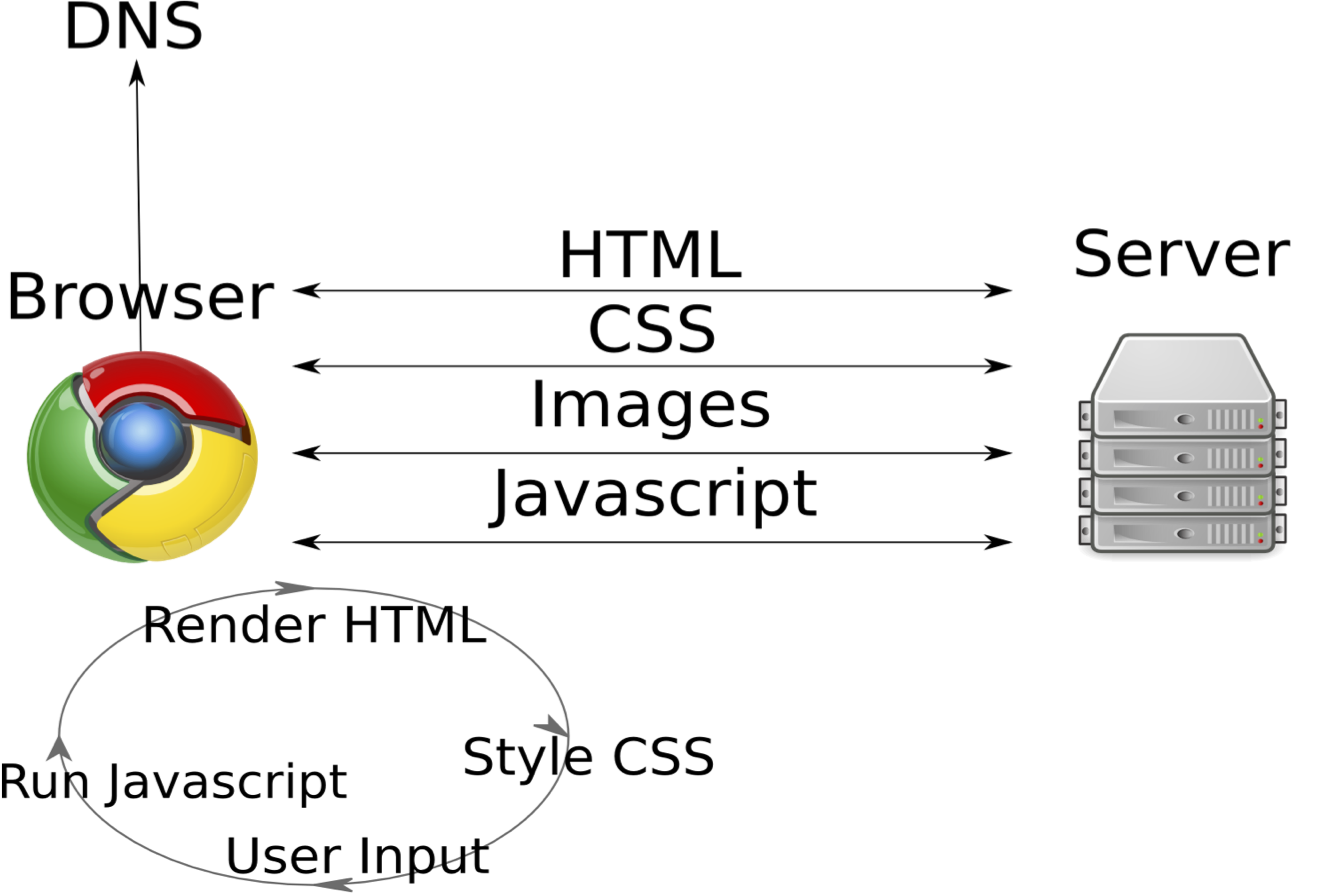
Let's build our own server
- We will be using NodeJS, a runtime environment for building server-side applications
- NodeJS uses JavaScript as its programming language
NodeJS is an application like everything else
- Need to install it on your host (e.g. your computer)
- Once installed, you can pass it a JavaScript file with the instructions on how to run your server
Let's get started
And that's it for installation!
sudo apt-get install nodejs
Well not quite
- One of the strengths of NodeJS is the sheer number of open source libraries that exist
- These libraries add functionality to the core NodeJS modules
- For this week we will be using the library express to demonstrate server programming
- Afterwards we will be introducing another framework NextJS that is built around React
NPM
- Central repository for external NodeJS Libraries
- Need to install it on your host
sudo apt-get install npm
Let's create our first server
- Open a temrinal and navigate to a blank folder
npm init -y
- Now you have a NodeJS project
What did that do?
- Created a single file named package.json
- Think of this file as the manifest for your server application
- It indicates the name of your application and where to find it
- It also contains all of the information to install and run your application
Package.json
{
"name": "test",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC"
}
Let's install external libraries
- Here we are going to install express, a NodeJS library for writing web servers
- Do it by typing the following:
npm install express --save
- The "npm install express" part downloads the express module to a folder called "node_modules"
- The "--save" part saves a reference to the dependency (library) in that "package.json" file
Updated package.json
{
"name": "test",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC",
"dependencies": {
"express": "^4.14.0"
}
}
Now let's run our own webserver
app.js
var express = require('express'); // Adding the express library
var app = express(); // initializing applicaiton
// Define your routes here
app.get('/', function (req, res) {
res.send("<h1>Hello World</h1>");
});
// Start up server on port 3000 on host localhost
var server = app.listen(3000, function () {
console.log('Server on localhost listening on port 3000');
});
Run your webserver
-
nodejs app.js
- And then go to "http://localhost:3000/" on a browser on your computer
What's going on?
- We first created a variable that points to a function that contains the express module
- Then we ran the express function and it returned a variable that we assigned to "app"
- We then set up some routes
- And at the end we started our server by telling our app to listen to port 3000
- Note the console.log statement: in the server runtime environment console.log logs to the terminal
Note about routes
- Routes associate a path to a function that sends a response back to the client
- So if a client asks for the root path: http://localhost:3000/, it will run the above function
- The response body will be "<h1>Hello World</h1>"
app.get('/', function (req, res) {
res.send("<h1>Hello World</h1>");
});
Questions?
Webserver Programming I - Frontend Webarch
By kayashaolu
Webserver Programming I - Frontend Webarch
Course Website: https://www.ischool.berkeley.edu/courses/info/253a
- 712