Object Oriented Programming

Let's review some core concepts about OOP
@kenji_lozano
Kenji Lozano
Senior Software Developer, Truextend
Angular, React and Java
Javascript Lover

Sucre, Bolivia
Cochabamba






Spring, .NET
WHY OOP?!
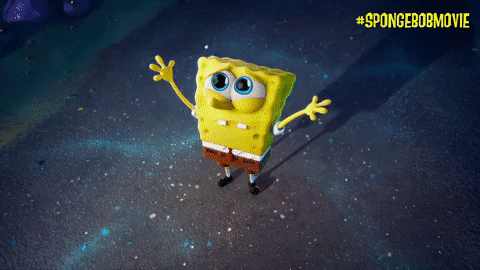
Keep in mind... ;)
Based on your interviews we got the following:
- Most of you have a "REGULAR" OOP Knowledge
- We expect a "GOOD" to "EXCELLENT" knowledge
So...
What is OOP?
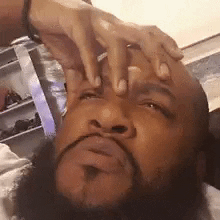
Paradigm
Four Pillars of Object Oriented Programming
Encapsulation
Encapsulation
... is the mechanism of hiding of data implementation by restricting access to public methods through Access Modifiers
class ExampleClass {
private int integerValue;
private String stringValue;
public int getIntegerValue(){
return integerValue;
}
public String getStringValue(){
return stringValue;
}
public void setIntegerValue(int newValue){
integerValue = newValue;
}
public void setStringValue(String newValue){
stringValue = newValue;
}
}
Encapsulation
Abstraction
Abstraction
... is the act of removing characteristics from something in order to reduce it to a set of essential functions or characteristics
Abstraction
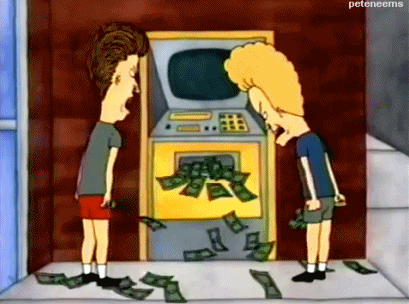
Security
Servers
Transactions
Nobody cares about it!
Abstraction
What to have an abstraction to draw and get area of a Shape
Abstraction
public interface Shape{
public void draw();
public double getArea();
}
Abstraction
public class Circle implements Shape{
private double radius;
public Circle(double r){
this.radius = r;
}
void draw(){
System.out.println("Drawing Circle");
}
public double getArea(){
return Math.PI*this.radius*this.radius;
}
public double getRadius(){
return this.radius;
}
}
Abstraction
public class Test{
public static void main (String args[]){
Shape c = new Circle(8);
c.draw();
System.out.println("Area=" + c.getArea());
}
}
Pro-Tip
Encapsulation vs Abstraction
Encapsulation is realization of your desired abstraction.
Abstraction is more about hiding the implementation details. Encapsulation is about wrapping the implementation (code) and the data it manipulates (variables) within the same class
Inheritance
Inheritance
... expresses “is-a” and/or “has-a” relationship between two objects
Inheritance
class Teacher {
String designation = "Teacher";
String collegeName = "Beginnersbook";
void does(){
System.out.println("Teaching");
}
}
public class PhysicsTeacher extends Teacher{
String mainSubject = "Physics";
}
public static void main(String args[]){
PhysicsTeacher obj = new PhysicsTeacher();
System.out.println(obj.collegeName);
System.out.println(obj.designation);
System.out.println(obj.mainSubject);
obj.does();
}
Beginnersbook
Teacher
Physics
Teaching
Polymorphism
Polymorphism
... is the ability of an object to take on many forms.
... is one of the OOPs feature that allows us to perform a single action in different ways
Polymorphism
public class Animal{
...
public void sound(){
System.out.println("Animal is making a sound");
}
}
public class Horse extends Animal{
...
@Override
public void sound(){
System.out.println("Neigh");
}
}
public class Cat extends Animal{
...
@Override
public void sound(){
System.out.println("Meow");
}
}
public static void main(String args[]){
Animal obj = new Horse();
obj.sound();
}
Neight
Don't Forget The Four OOP Pillars
Encapsulation
Abstraction
Inheritance
Polymorphism
So, this was just a quick talk about the most important OOP concepts
Challenge Time!

Event Ticketing App
Want to have you modeling an app that let us buy tickets for any event.
You could have the use case of Stadium "Felix Capriles"
Could be just a Class Diagram or whatever you want to show us your modeling skills
Consider that you could have more than 1 types of tickets
You MUST use the Four Pillars of Object Oriented Programming
You MUST consider the Scalability, Adaptability and Flexibility
Event Ticketing App
We expect something like this:
https://stackify.com/oop-concept-abstraction/
Event Ticketing App
If we (Kenji) like your solution, will send you a gift (usually food)
The organizer (Kenji) reserves the rights to have a winning solution
Thank You!
Hope you enjoyed this talk!
Resources
https://www.edureka.co/blog/java-abstraction/
https://stackify.com/oop-concept-abstraction/
https://richardeng.medium.com/a-simple-explanation-of-oop-46a156581214
https://medium.com/learn-how-to-program/chapter-3-what-is-object-oriented-programming-d0a6ec0a7615
https://medium.com/from-the-scratch/oop-everything-you-need-to-know-about-object-oriented-programming-aee3c18e281b
https://medium.com/swlh/oop-concept-in-javascript-119f03b0a134
https://www.javacodemonk.com/what-are-four-principles-of-oop-how-aggregation-is-different-than-composition-5b534baf
OOP
By Kenji Lozano
OOP
- 78