Javascript - Another API Review
Leon Noel

#100Devs
And that's why you remind me
That I can never be lost in the sauce, I'm a boss
And everything was a lesson, from that loss, I gained it all
Agenda
-
Questions?
-
Let's Talk - #100Devs
-
Review - Functions && Loops
-
Review - Arrays
-
Review - Objects
-
Review - APIs
-
Review - Local Storage
-
Homework - JS Review / Push
Questions
About last class or life

Checking In
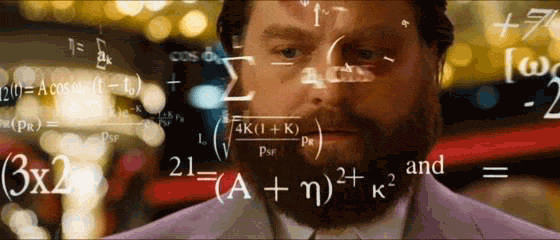
Like and Retweet the Tweet
!checkin
No Networking Until May
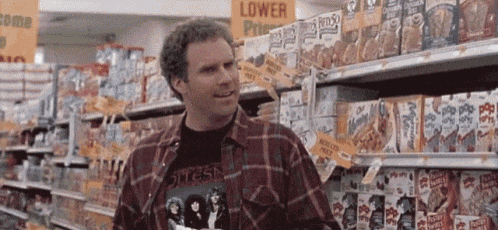
Client Deadline: May 3rd
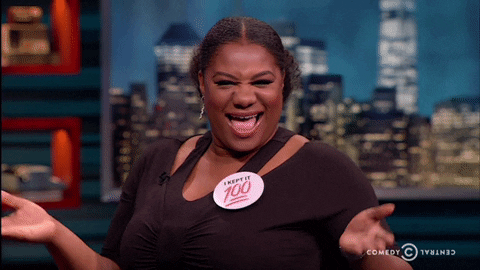
Client Alternatives
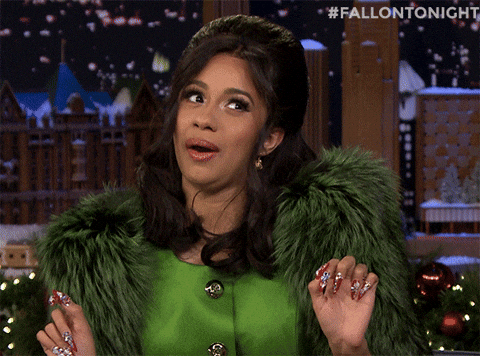
Grassroots Volunteer*
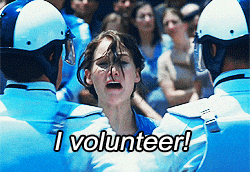
Free Software / Open Source
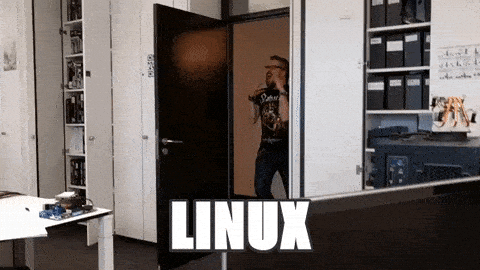
Codewars !Clan
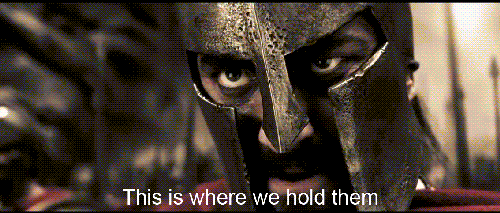
Clan: #100Devs - leonnoel.com/twitch
Submitting Work
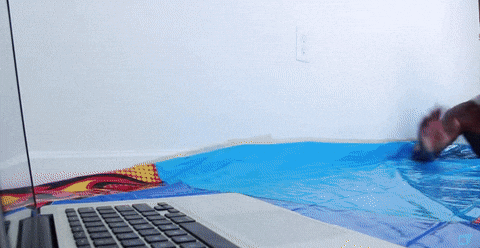
"Break"
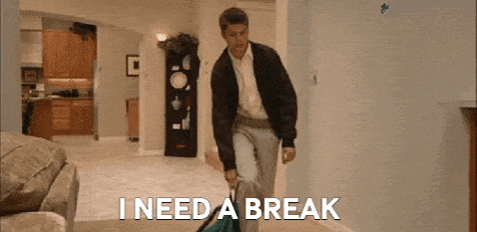
No "Class" Next Week
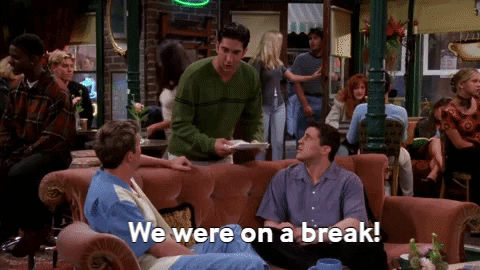
Work Together Nights
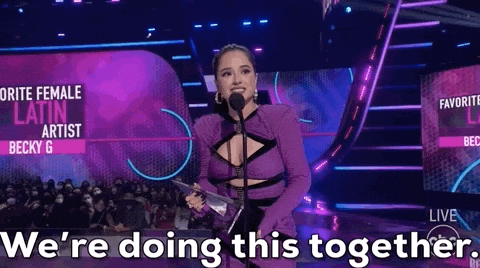
Tuesday / Thursday
Homework
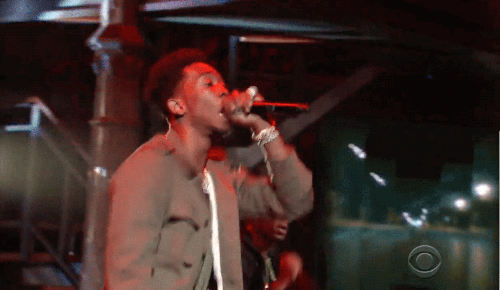
GIT BOWL
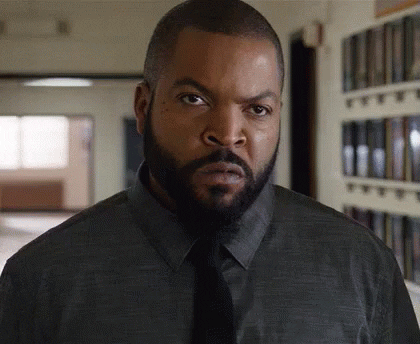
Sunday April 24th
Programming
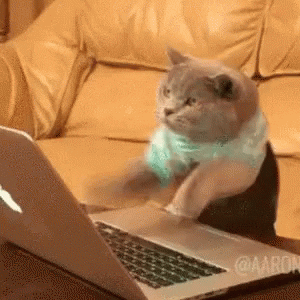
What is a program?
A program is a set of instructions that you write to tell a computer what to do
What is a programming?
Programming is the task of writing those instructions in a language that the computer can understand.
JAVASCRIPT

Loops
What are loops?
Loops
-
Repeat an action some number of times!
-
Three main types of loops in Javascript
-
For, while, and do while loops
-
Each type offers a different way to determine the start and end points of a loop
Loops - For
for (let i = 1; i < 5; i++) {
console.log(i)
}
Arrays
What are arrays?
Toasters
Arrays
-
A data structure to store ordered collections!
-
Array elements are numbered starting with zero
-
Arrays have many methods to manage the order of elements
-
Can be created by a constructor or literal notation
Declaring Arrays
let newArr = []
Literal Notation
Declaring Arrays
newArr = ['Zebra',true,21]
Arrays are populated with elements
Elements can be of any type
Array Indexing

Array Indexing
newArr = ['Zebra',,true,21]
console.log( newArr[0] ) //Zebra
console.log( newArr[1] ) //undefined
console.log( newArr[2] ) //true
console.log( newArr[3] ) //21
Elements can be accessed by their index numbers
Array Indexing
newArr = ['Zebra',,true,21]
newArr[1] = 'Bob'
console.log( newArr )
// ['Zebra','Bob',true,21]
Elements can be updated by using the index at that position
Array Length
console.log( newArr.length ) //4
How do you determine how many elements are in your array?
Array Iteration
let bestColors = ['green','blue','yellow','black']
bestColors.forEach((x,i)=> console.log(x))
Iterates through an array passing in the value and index of each element
Objects
What are objects?
Objects
-
Objects are a collection of variables and functions!
-
Objects represent the attributes and behavior of something used in a program
-
Object variables are called properties and object functions are called methods
-
Objects store "keyed" collections
Think of a physical object

What are it's attributes and behaviors?
Stopwatch Object
let stopwatch = {}
stopwatch.currentTime = 12
stopwatch.tellTime = function(time){
console.log(`The current time is ${time}.`)
}
stopwatch.tellTime(stopwatch.currentTime)
APIs
What are APIs?
APIs
-
A simple interface for some complex action!
-
Think of a restaurant menu! Remember those...
-
Lets one thing communicate with another thing without having to know how things are implemented.
APIs

APIs
Fetch Fido, Fetch!
fetch("https://dog.ceo/api/breeds/image/random")
.then(res => res.json()) // parse response as JSON
.then(data => {
console.log(data)
})
.catch(err => {
console.log(`error ${err}`)
});
API returns a JSON object that we can use within our apps
APIs
Stop trying to make Fetch happen!
fetch(url)
.then(res => res.json()) // parse response as JSON
.then(data => {
console.log(data)
})
.catch(err => {
console.log(`error ${err}`)
});
Some APIs need Query Parameters to return the correct data
const url = 'https://www.thecocktaildb.com/api/json/v1/1/search.php?s=margarita'
Let's Code

Local Storage
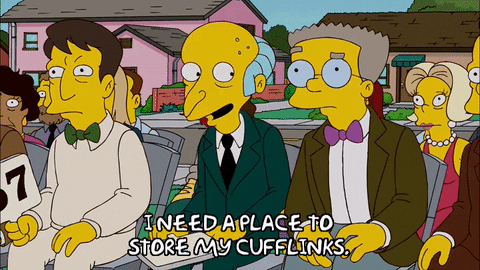
Local Storage
Allows you to store data across browser sessions
Put Item Into Local Storage
localStorage.setItem('bestFriend', 'Bob')
Get Item Out Of Local Storage
localStorage.getItem('bestFriend', 'Bob')
Remove Item In Local Storage
localStorage.removeItem('bestFriend', 'Bob')
Remove All In Local Storage
localStorage.clear()
Let's Code

Homework
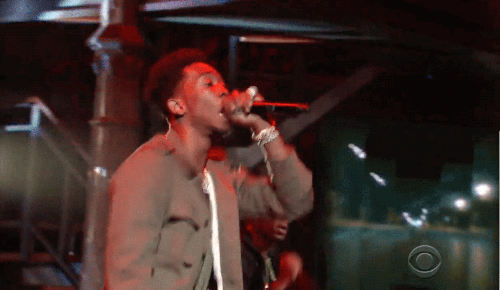
#100Devs - Another API Review
By Leon Noel
#100Devs - Another API Review
Class 28 of our Free Web Dev Bootcamp for folx affected by the pandemic. Join live T/Th 6:30pm ET leonnoel.com/twitch and ask questions here: leonnoel.com/discord
- 4,808