Leon Noel

Big O
#100Devs
"But that was the shit that I prayed about I thank God I made it out
Damn it feels good to go pick up my momma and take her out
Lil Tony got paper now all in my bank account, and it ain't shit to debate about"
Agenda
-
Questions?
-
Discuss - #HUNTOBER2022
-
Discuss - You ARE ready!
-
Learn - Big O
-
Homework
Questions
About class or life

Checking In
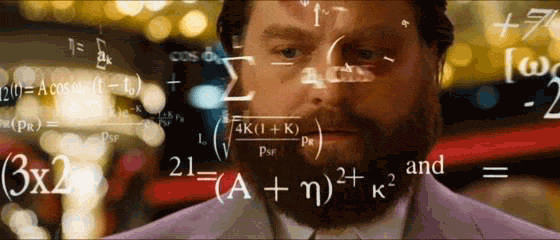
Like and Retweet the Tweet
!checkin
!newsletter
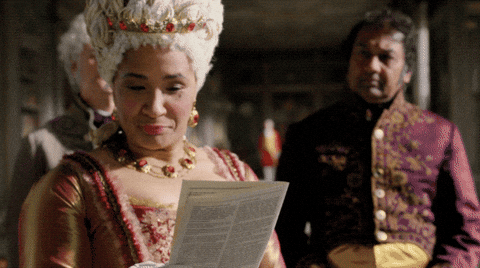
#HUNTOBER2022
!templates
4 More Classes
Building Hitlists & How To Get A Job
FRIDAY TEA
6:00pm ET in Classroom
SUNDAY OFFICE HOURS
1pm ET
Daily Question
Daily Standup
(Mon-Fri 6pm EST)
WE NEED TO TALK
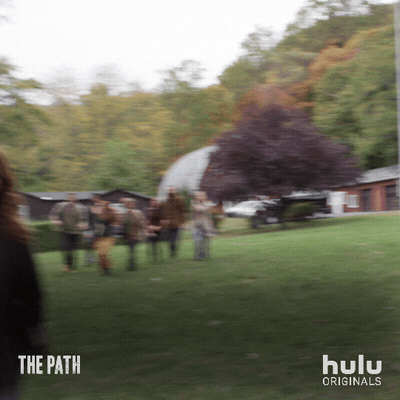
Ready? Others?
P.R.E.P
Parameters, Returns, Examples, Pseudo Code
Methods Questions
Brute Force
ITS ALL YOU NEED
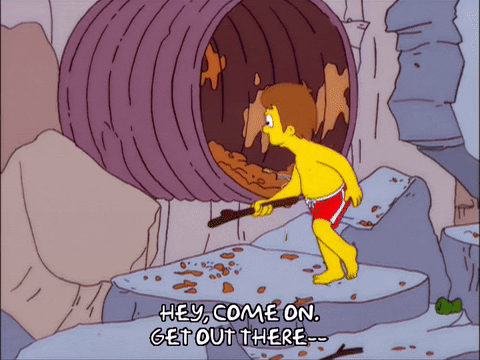
But... Let's Open More Doors
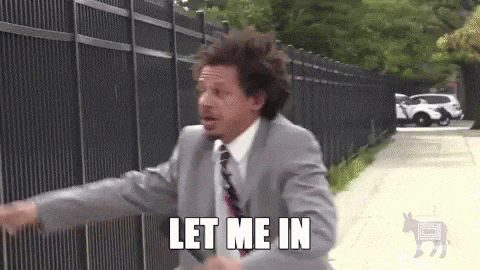
A data structure is simply an organized way of storing data
It is a pattern of collecting and organizing data for performing various operations correctly and efficiently.
BUT WHY?
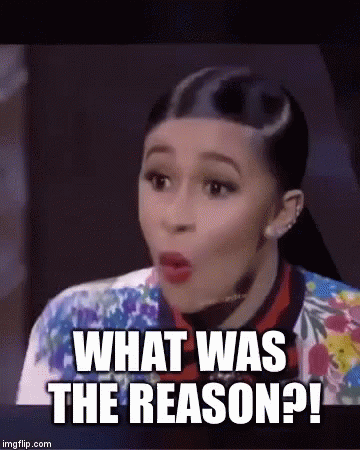
What if I wanted to store information about a stock trader?
Object or Array?
Object Makes The Most Sense
let stockTrader = {
firstName: 'Bob',
lastName: 'Wehadababyitsaboy',
location: 'New York, New York, USA',
jobTitle: 'Trading Associate',
yoe: 1,
recentTrades: ['tsla','gme','amc']
}
What if I wanted to store recent tsla purchase prices?
Object or Array?
Array Makes The Most Sense
let tslaPurchasePrices =
[208.17, 205.05, 217.57, 220.48]
An algorithm is just the steps you take to solve a problem
Thinking through appropriate
algorithms is how we can efficiently solve problems
We care about
Space
&
Time
Space
How much memory is being used
Time
How many operations are executed per input
When taking about the efficiency of a solution we can use
Big-O Notation
BUT WHY?
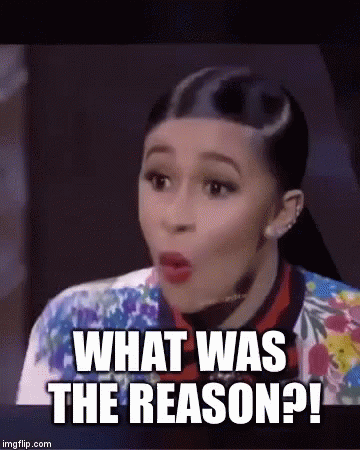
Big-O notation mathematically describes the complexity of an algorithm in terms of time and space
BUT WHY?
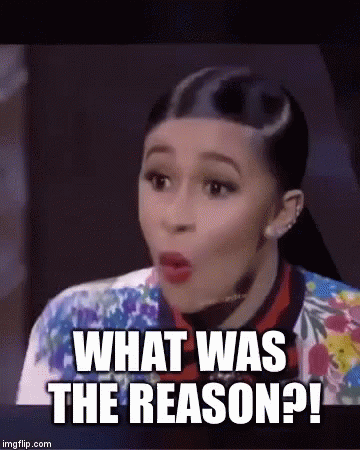
All computers, environments, ect... are different
We care about
rough estimates
Common Complexities

O(1)
For all inputs to our algorithm there is and will always be only one operation required
- Order 1
- Constant Time
O(1) Example
No matter how many inputs are located in num there will only ever be one operation needed!
const nums = [1,2,3,4,5]
const firstNumber = nums[0]
O(N)
For all inputs to our algorithm there will be one
operation per input
- Order n
- Linear
- Linear Scailing
O(N) Example
Here we sum the numbers in the array. We have to add each number to a running sum, so we have to operate on each one. This means one operation per input.
const nums = [1,2,3,4,5]
let sum = 0
for(let num of nums){
sum += num
}
O(1) VS O(N)
Summing function for a sorted, contiguous array of integers that starts with the number 1? Could easily be O(n) but...
const sumContiguousArray = function(ary){
//get the last item
const lastItem = ary[ary.length - 1]
//Gauss's trick
return lastItem * (lastItem + 1) / 2
}
const nums = [1,2,3,4,5]
const sumOfArray = sumContiguousArray(nums)
O(N^2)
Quadratic
Text
const hasDuplicates = function(num){
for(let i = 0; i < nums.length; i++){
const thisNum = nums[i]
for(let j = 0; j < nums.length; j++){
if(j !== i){
const otherNum = nums[j]
}
}
if(otherNum === thisNum) return true
}
return false
}
const nums = [1,2,3,4,5,5]
hasDuplicates(nums) //true
O(N^2)
Here we’re iterating over our array, which we already know is O(n), and another iteration inside it, which is another O(n). For every item in our list, we have to loop our list again to calculate what we need.
O(LOG N)
Logarithmic Time
As you loop your operations are halved
Divide and Conquer?

Committing these to memory is important
I have a problem
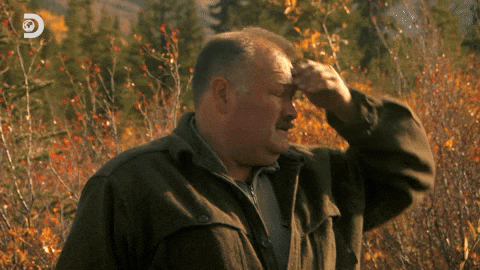
The price of boxes is
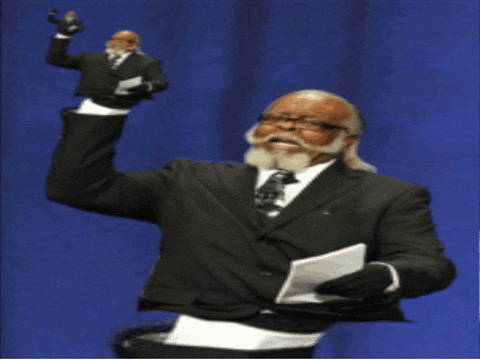
TOO DAMN HIGH


Let's take a look
I want to create a bidding platform
How can we find the min and max bid?
Let's loop through
all the bids
Text
const findMaxBid = function(bids){
let maxBid = bids[0],
minBid = bids[0]
for(let i = 0; i < bids.length; i++){
for(let j = 0; j < bids.length; j++){
if(bids[i] > bids[j] && bids[i] > maxBid ){
maxBid = bids[i]
}else if(bids[i] < bids[j] && bids[i] < minBid){
minBid = bids[i]
}
}
}
return [minBid, maxBid]
}
const allBids = [2,7,3,1,4,5,5]
console.log(findMaxBid(allBids))
Really bad code?,
but what is the complexity?

What if one loop found the min and the other found the max
Finding the max bid
Text
const findMaxBid = function(bids){
let maxBid = bids[0]
for(let i = 0; i < bids.length; i++){
if(bids[i] > maxBid){
maxBid = bids[i]
}
}
return maxBid
}
const allBids = [1,2,7,3,4,5,5]
console.log(findMaxBid(allBids))
2n?
What if our data was already sorted?
2?
What is an example of constant time?
What is an example of linear time?
What is an example of quadratic time?
What is an example of logarithmic time?
Let's explore some JS complexities
.pop() ?
.shift() ?
.length ?
forEach, map, reduce ?
Homework
Do: Take your first application step!
Do: https://frontendmasters.com/courses/practical-algorithms
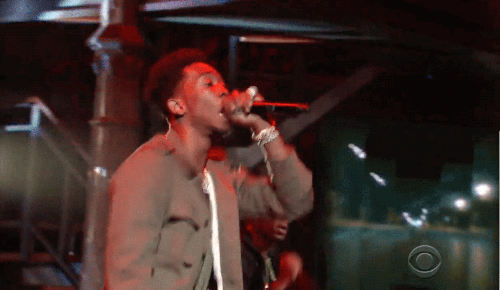
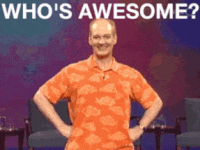
#100devs - Big O (cohort 02)
By Leon Noel
#100devs - Big O (cohort 02)
Class 63 of our Free Web Dev Bootcamp for folx affected by the pandemic. Join live T/Th 6:30pm ET leonnoel.com/twitch and ask questions here: leonnoel.com/discord
- 1,354