Javascript - Arrays
#100Devs
Leon Noel

Metal Fist terrorists claim responsibility
Broken household name usually said in hostility
Um... what is MF? You silly
Agenda
-
Questions?
-
Let's Talk - #100Devs
-
Review - Functions && Loops
-
Learn - Arrays
-
Learn - Array Iteration
-
Learn - Array Methods
-
Homework - Objects
Questions
About last class or life

Checking In
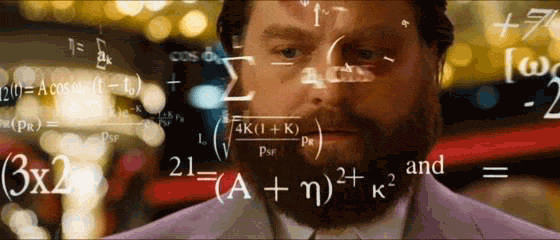
Like and Retweet the Tweet
!checkin
Want to be fancy?
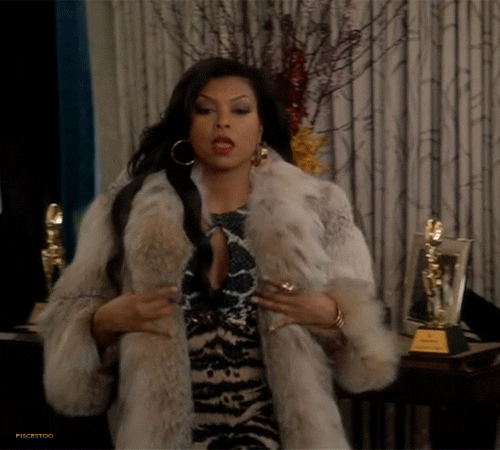
Best Clip Get's Special Color On Discord!
Submitting Work
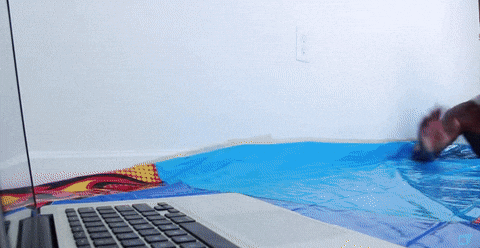
Networking
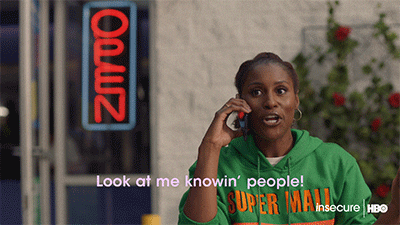
3 Individuals Already In Tech
2 Coffee Chats
USE THE SHEET!
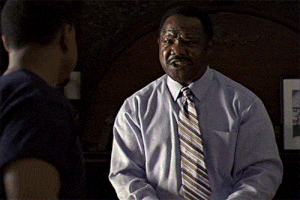
Coding Challenges
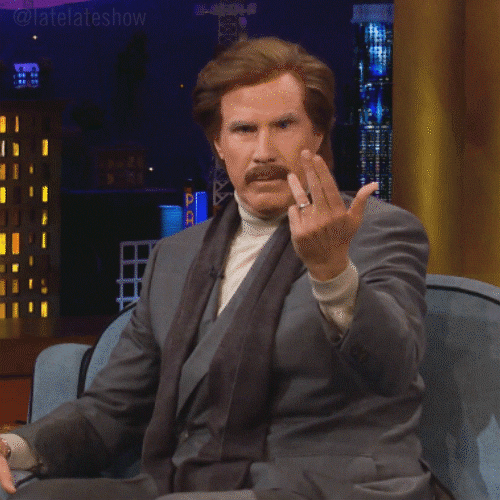
Daily
Codewars 8kyu Fundamentals
Paid Client
Due by Mar. 31st
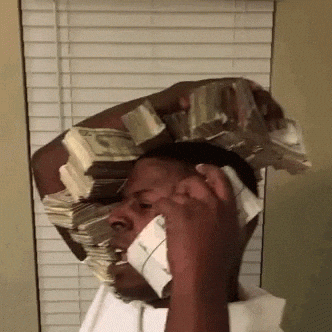
TURN IT UP!
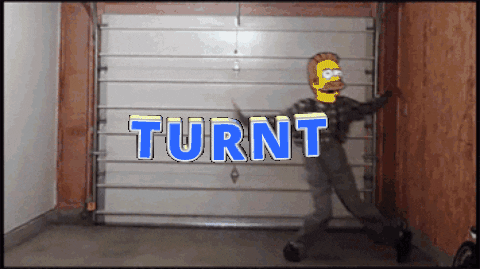
Programming
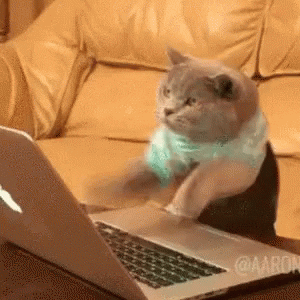
A computer will do what you tell it to do.
What is a program?
A program is a set of instructions that you write to tell a computer what to do
What is a programming?
Programming is the task of writing those instructions in a language that the computer can understand.
JAVASCRIPT

Has a specific 'Syntax'
Syntax: "Spelling and grammar" rules of a programming language.
Variables
Declaration: let age
Assignment: age = 25
Both at the same time:
let age = 25
Conditional Syntax
if(condition is true) {
//Do this cool stuff
}else if(condition is true){
//Do this other cool stuff
}else{
//Default cool stuff
}
🚨 DANGER 🚨
Assignment vs. Comparison
Multiple Conditions
if (name === "Leon" && status === "Ballin"){
//Wink at camera
}
Multiple Conditions
if (day === "Saturday" || day === "Sunday"){
//It is the weekend
}
Functions
What are functions?
Functions
-
Functions are simple sets of instructions!
-
Functions are reusable
-
Functions perform one action as a best practice
-
They form the basic "building blocks" of a program
Functions
function name(parameters){
//body
}
//call
name(arguments)
Functions
function yell(word){
alert(word)
}
yell("HELLO")
Loops
What are loops?
Loops
-
Repeat an action some number of times!
-
Three main types of loops in Javascript
-
For, while, and do while loops
-
Each type offers a different way to determine the start and end points of a loop
Loops - For
for (let i = 1; i < 5; i++) {
console.log(i)
}
Loops - While
let count = 0
while(count < 5){
console.log(count)
count++
}
Let's Code
Bring It On - All Or Nothing!

Arrays
What are arrays?
Toasters
Arrays
-
A data structure to store ordered collections!
-
Array elements are numbered starting with zero
-
Arrays have many methods to manage the order of elements
-
Can be created by a constructor or literal notation
Declaring Arrays
let newArr = new Array()
Array Constructor
Declaring Arrays
let newArr = []
Literal Notation
Declaring Arrays
newArr = ['Zebra',true,21]
Arrays are populated with elements
Elements can be of any type
Declaring Arrays
🚨Empty spaces leave an empty element 🚨
Array Indexing

Array Indexing
newArr = ['Zebra',,true,21]
console.log( newArr[0] ) //Zebra
console.log( newArr[1] ) //undefined
console.log( newArr[2] ) //true
console.log( newArr[3] ) //21
Elements can be accessed by their index numbers
Array Indexing
newArr = ['Zebra',,true,21]
newArr[1] = 'Bob'
console.log( newArr )
// ['Zebra','Bob',true,21]
Elements can be updated by using the index at that position
Array Indexing
let cars = ['Honda', 'Toyota', 'Ford', 'Tesla']
let nums = [1,2,3]
cars = nums
console.log( cars ) //[1,2,3]
You can overwrite whole arrays by assigning an array to a different array

Array Length
console.log( newArr.length ) //4
How do you determine how many elements are in your array?
Let's Code
Array Dat - In It To Win It

Array Iteration
let bestColors = ['green','blue','yellow','black']
for(let i = 0; i < bestColors.length;i++){
console.log( bestColors[i] )
}
Iterates through an array passing in the value and index of each element
Array Iteration
let bestColors = ['green','blue','yellow','black']
bestColors.forEach((x,i)=> console.log(x))
Iterates through an array passing in the value and index of each element
Let's Code
Array Dat - Fight To The Finish

Other Arrays Methods
let bestRappers2020 = ['6ix9ine','Polo G','6ix9ine']
let removed = bestRappers2020.shift()
console.log( bestRappers2020 ) // ['Polo G', '6ix9ine']
Remove item from the beginning of an Array
Other Arrays Methods
let bestRappers2020 = ['Polo G','6ix9ine']
let removedAgain = bestRappers2020.pop()
console.log( bestRappers2020 ) // ['Polo G']
Remove item from the end of an Array
Other Arrays Methods
let bestRappers2020 = ['Polo G']
let removed = bestRappers2020.unshift('Dylan')
console.log( bestRappers2020 ) // ['Dylan','Polo G']
Add item to the beginning of an Array
Other Arrays Methods
let bestRappers2020 = ['Dylan','Polo G']
let removed = bestRappers2020.push('Dylan')
console.log( bestRappers2020 ) // ['Dylan','Polo G','Dylan']
Add item to the end of an Array
Other Arrays Methods
let bestRappers2020 = ['Dylan','Polo G','Dylan']
let bestRappersAllTime = bestRappers2020.map(x => 'Dylan')
bestRappersAllTime.unshift('Dylan')
bestRappersAllTime.push('Dylan')
console.log( bestRappersAllTime )
// ['Dylan','Dylan','Dylan', 'Dylan', 'Dylan']

Let's Code
Array Dat - Worldwide Cheersmack

Homework
Read: JSWay Arrays
Read: https://javascript.info/array-methods
Read: JSWay Objects
Read: https://eloquentjavascript.net/04_data.html
Do: Minimum of 1 https://codewars.com 8 Kyu Fundamentals Track EVERY DAY - 20 mins then look at solution!
Do: https://javascript30.com Day 04 Array Cardio (super hard, do it on Discord together)
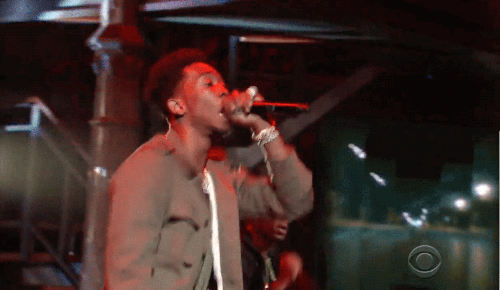
#100Devs - Javascript Arrays (cohort 2)
By Leon Noel
#100Devs - Javascript Arrays (cohort 2)
This is class 19 for our Free Web Dev Bootcamp for folx affected by the pandemic. Join live T/Th 6:30pm ET leonnoel.com/twitch and ask questions here: leonnoel.com/discord
- 3,617