Javascript - More Basics
Leon Noel
"If I get caught up in the storm, my brain gon storm the most"

#100Devs
Agenda
-
Questions?
-
Let's Talk - Imposter Syndrome
-
Learn - What is programming?
-
Learn - JS Basics
-
Review - Previous Class Work
-
Learn - Pseudo Code
-
Build - Temperature Converter
-
Learn - Functions
-
Build - Functions
-
Homework - Read Please
Questions
About last class or life

Checking In
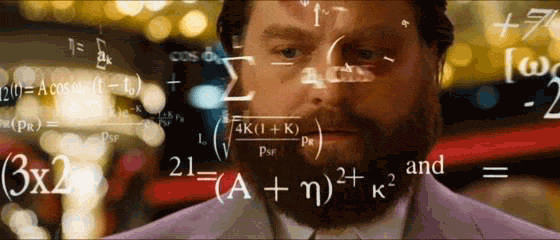
Like and Retweet the Tweet
!checkin

Submitting Work
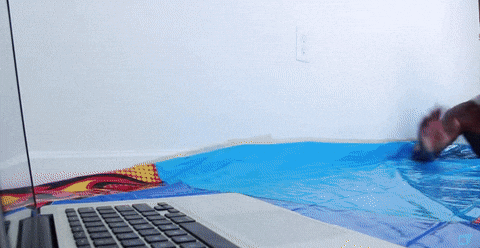
Share What JS You Added in codepen.io
Submit URLs here: https://forms.gle/G7LhHnyTA7zYq7UV6
Networking
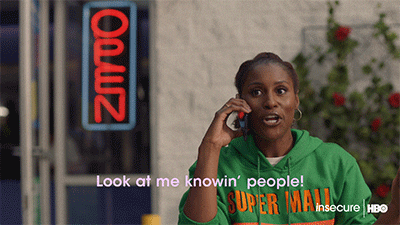
2 Individuals Already In Tech
Push? 1 Coffee Chat
Imposter Syndrome
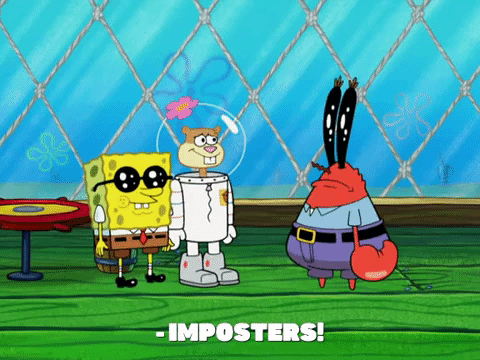
The Golden Rule
SEPERATION OF CONCERNS
-
HTML = Content / Structure
-
CSS = Style
-
JS = Behavior / Interaction
IDs & Classes

IDs
#zebra {
color: red;
}
IDs are used for selecting distinct elements
Only one id with the same value per document
#idName
<section>
<p>Hello, Twitch!</p>
<p id="zebra">Hello, Youtube!</p>
</section>
Classes
.bob {
color: red;
}
Classes are for selecting multiple elements
Multiple with same value allowed per document
.className
<section>
<p class="robot">Hello, Twitch!</p>
<p id="zebra" class="bob">Hello, Youtube!</p>
<p class="bob">Goodbye, Mixer!</p>
</section>
Programming
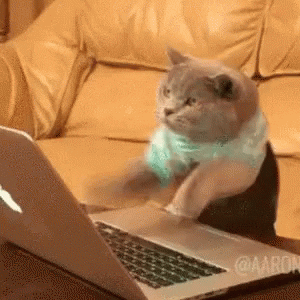
A computer will do what you tell it to do.
What is a program?
A program is a set of instructions that you write to tell a computer what to do
What is a programming?
Programming is the task of writing those instructions in a language that the computer can understand.
True Story
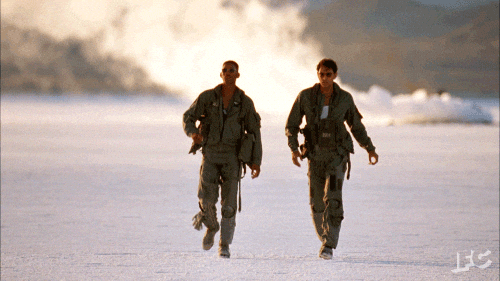
JAVASCRIPT

Has a specific 'Syntax'
Syntax: "Spelling and grammar" rules of a programming language.
Variables
What are variables?
Variables
- We can tell our program to remember values for us to use later on
- The entity we use to store the value is called a variable
Variables
Declaration: let age
Assignment: age = 25
Both at the same time:
let age = 25
Variable Conventions
camelCase:
let numberOfApples = 9
Variables
& Data Types
What can you store in variables?
Strings
-
Stores text
-
String is surrounded by quotes
"How is the weather today?"
'Warm'
Strings
Double vs Single Quoted Strings:
'They "purchased" it'
"It's a beautiful day"
Numbers
Represent Numerical Data
int: 29
float: 5.14876
Numbers
Signed
int: +4
float: -10.375
Arithmetic In Javascript

Making Decisions
It's either TRUE or FALSE
If you are greater than 18 you are an adult
if (age > 18){
console.log("You are an adult")
}
Comparisons:
Equality
Are two things equal?
9 === 9 //true
7 === 3 //false
"Hello" === "Hello" //true
Logical Operators

Conditional Syntax
if(condition is true) {
//Do cool stuff
}
Conditional Syntax
if(condition is true) {
//Do this cool stuff
}else if(condition is true){
//Do this other cool stuff
}else{
//Default cool stuff
}
Conditional Syntax
const pizza = "Dominos"
if (pizza === "Papa Johns") {
console.log("Scram!")
} else if(pizza === "Dominos") {
console.log("All aboard the train to flavor town")
} else {
console.log("What are you even doing with your life?")
}
🚨 DANGER 🚨
Assignment vs. Comparison
Multiple Conditions
if (name === "Leon" && status === "Ballin"){
//Wink at camera
}
Multiple Conditions
if (day === "Saturday" || day === "Sunday"){
//It is the weekend
}
Let's Code
Class, Weekend, or Boring Day?

Let's Code
Angry Parent Simulator

Pseudo Code
Let's Code
A Temperature Converter

In Chat:
What is the best TV show of all time?
Answer:
The Bachelor
Let's Code
Bachelor Code

Functions
What are functions?
Functions
-
Functions are simple sets of instructions!
-
They form the basic "building blocks" of a program
Functions
function name(parameters){
//body
}
//call
name(arguments)
Functions
function yell(word){
alert(word)
}
yell("HELLO")
Let's Code
Simple Functions

Let's Code
Bring It Home!

Homework
Read: https://javascript.info/function-expressions + Tasks
Read: https://javascript.info/arrow-functions-basics + Tasks
Read:https://github.com/thejsway/thejsway/blob/master/manuscript/chapter04.md
Do: Delete the JS and do it again for all assignments
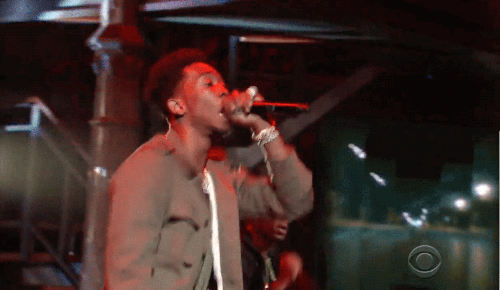
#100Devs Javascript More Basics (cohort 2)
By Leon Noel
#100Devs Javascript More Basics (cohort 2)
Class 13 of our Free Web Dev Bootcamp for folx affected by the pandemic. Join live T/Th 6:30pm ET leonnoel.com/twitch and ask questions here: leonnoel.com/discord
- 3,076