Javascript - Objects Fun
Leon Noel
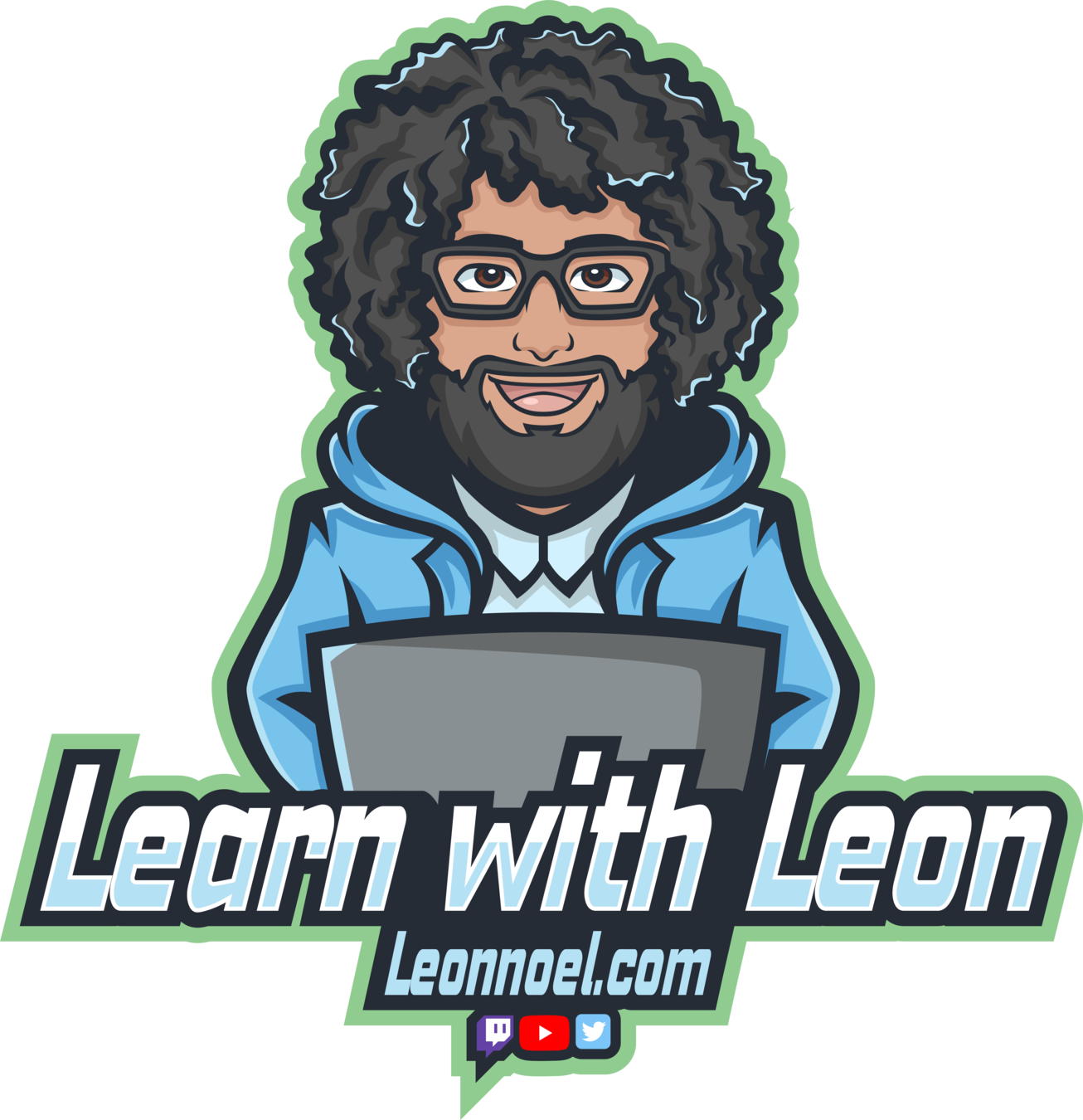
#100Devs
I like my love with a budget, I like my hugs with a scent
You smell like light, gas, water, electricity, rent
Agenda
-
Questions?
-
Let's Talk - #100Devs
-
Review - Functions && Loops
-
Review - Arrays
-
Learn - Objects
-
Learn - Basic APIs
-
Homework - Object City Yall
Questions
About last class or life
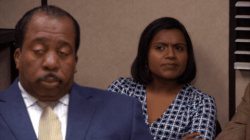
Checking In
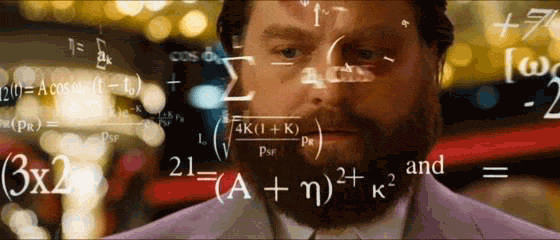
Like and Retweet the Tweet
!checkin
You Are Not Alone!
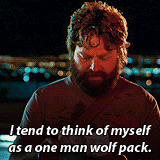
Trough Of Sorrow
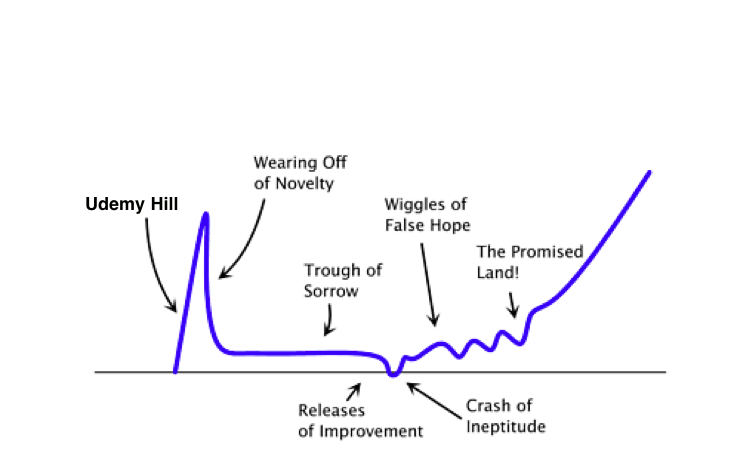
Health First
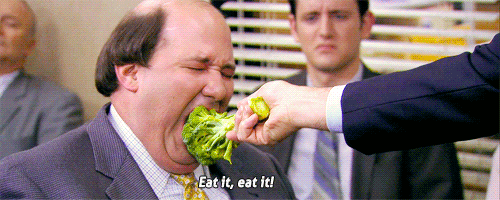
No Networking Until May
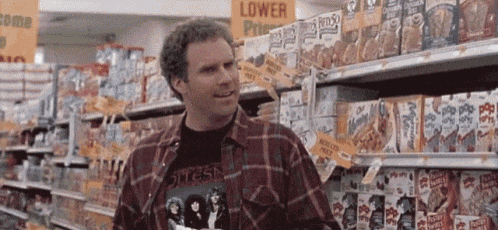
Client Deadline: May 3rd
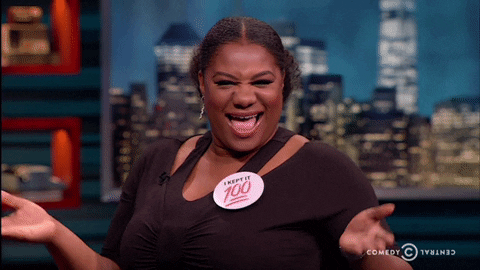
Client Alternatives
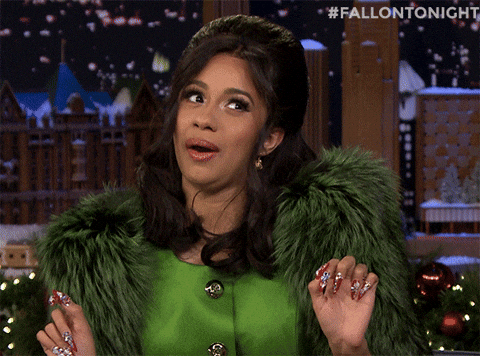
Grassroots Volunteer*
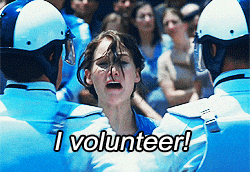
Free Software / Open Source
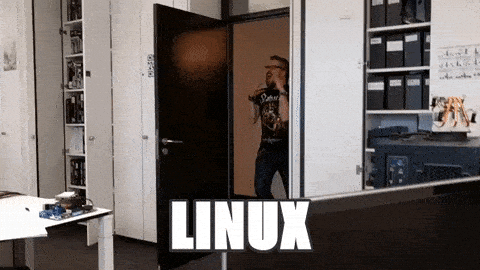
Live Portfolio & Resume Review
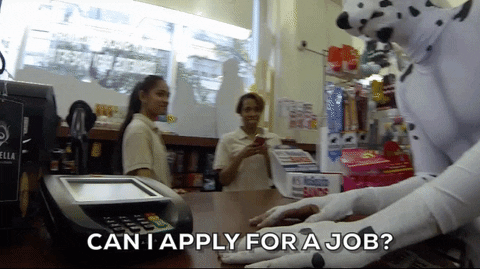
Monday
6:30pm EST
!youtube
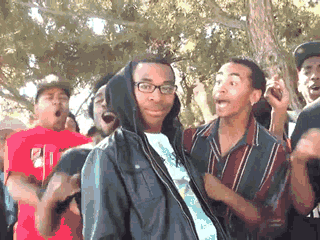
!newsletter
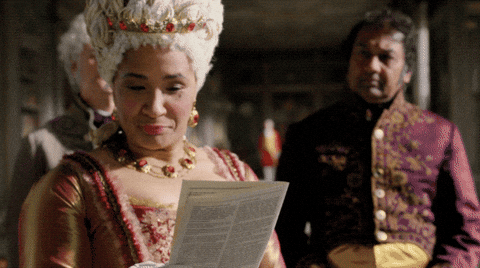
Welcome, helloitsrufio
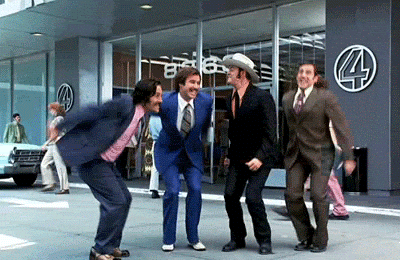
Tomorrow @ 6:30pm ET!
Microsoft
Sponsored Stream
Thursday Mar. 31st
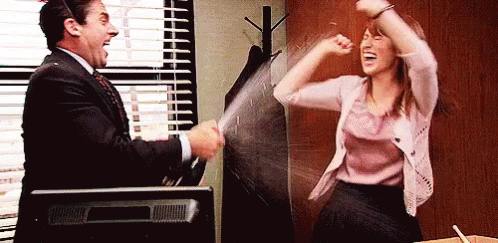
Thursday 6:30pm ET
Friday With Friends
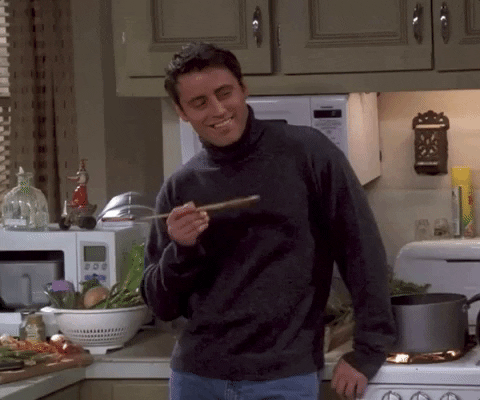
Friday 6pm ET on Discord!
/r/place
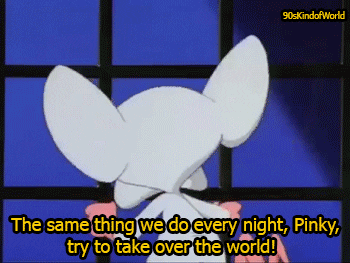
Saturday All Day

Office Hours
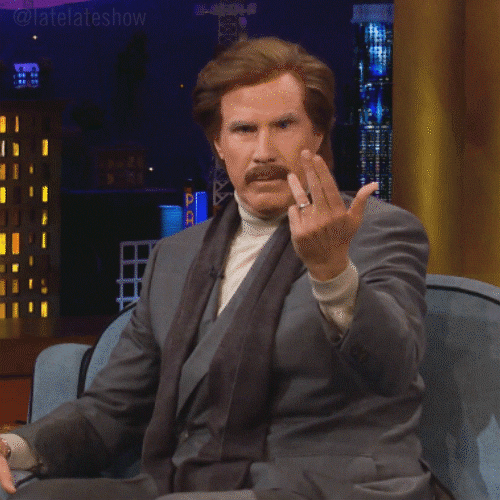
Sunday - 1pm ET
Mentor Monday
Portfolio / Resume Review Part 1
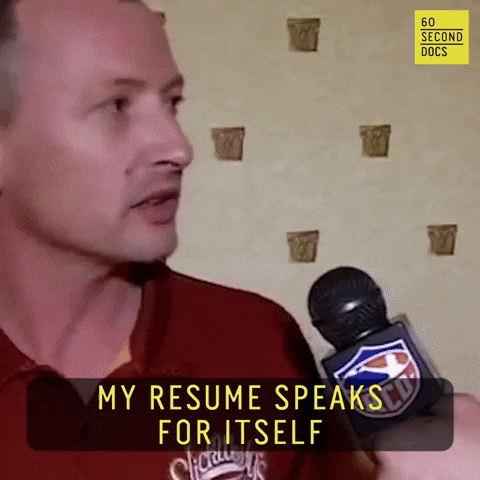
BEST WEEK EVER END?
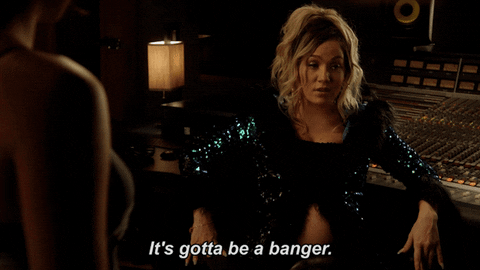
Tuesday 6:30pm ET
!Merch
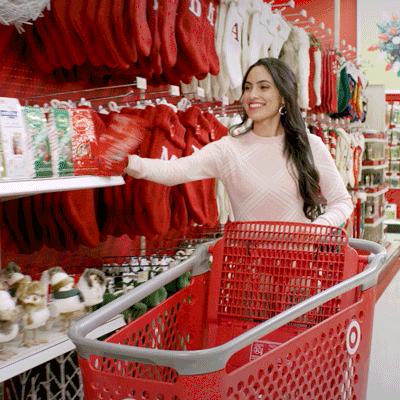
THANK YOU
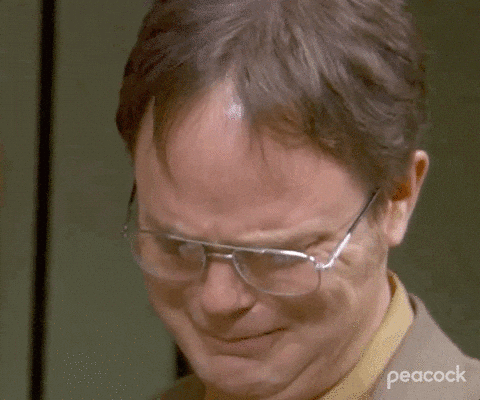
Programming
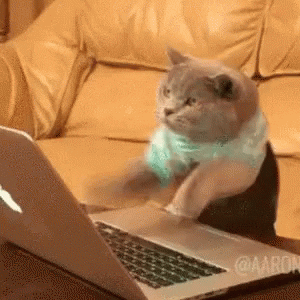
What is a program?
A program is a set of instructions that you write to tell a computer what to do
What is a programming?
Programming is the task of writing those instructions in a language that the computer can understand.
JAVASCRIPT
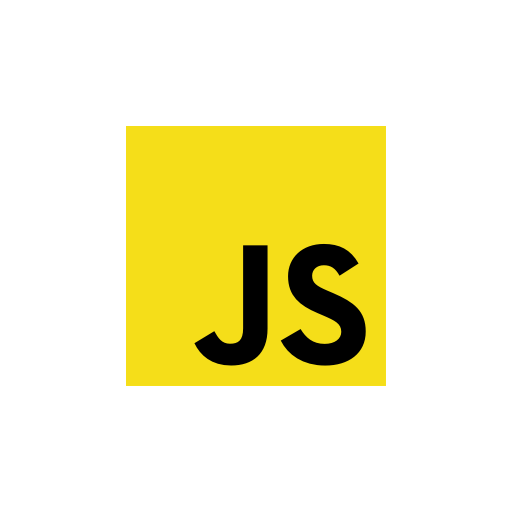
Variables
Declaration: let age
Assignment: age = 25
Both at the same time:
let age = 25
Conditional Syntax
if(condition is true) {
//Do this cool stuff
}else if(condition is true){
//Do this other cool stuff
}else{
//Default cool stuff
}
Multiple Conditions
if (name === "Leon" && status === "Ballin"){
//Wink at camera
}
Multiple Conditions
if (day === "Saturday" || day === "Sunday"){
//It is the weekend
}
Functions
What are functions?
Functions
-
Functions are simple sets of instructions!
-
Functions are reusable
-
Functions perform one action as a best practice
-
They form the basic "building blocks" of a program
Functions
function name(parameters){
//body
}
//call
name(arguments)
Functions
function yell(word){
alert(word)
}
yell("HELLO")
Loops
What are loops?
Loops
-
Repeat an action some number of times!
-
Three main types of loops in Javascript
-
For, while, and do while loops
-
Each type offers a different way to determine the start and end points of a loop
Loops - For
for (let i = 1; i < 5; i++) {
console.log(i)
}
Let's Code
Turbo Review
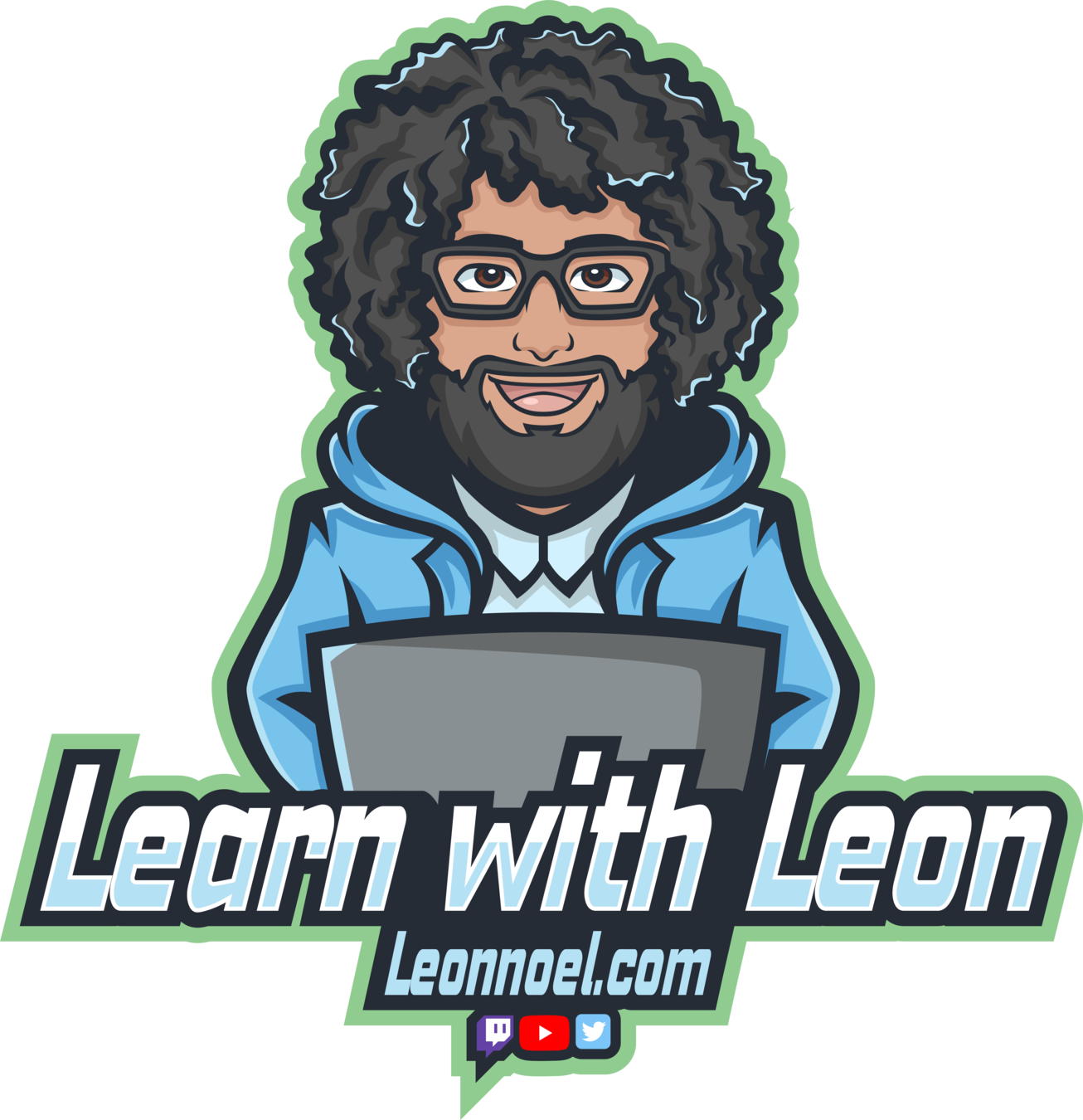
Arrays
What are arrays?
Toasters
Arrays
-
A data structure to store ordered collections!
-
Array elements are numbered starting with zero
-
Arrays have many methods to manage the order of elements
-
Can be created by a constructor or literal notation
Declaring Arrays
let newArr = new Array()
Array Constructor
Declaring Arrays
let newArr = []
Literal Notation
Declaring Arrays
newArr = ['Zebra',true,21]
Arrays are populated with elements
Elements can be of any type
Array Indexing
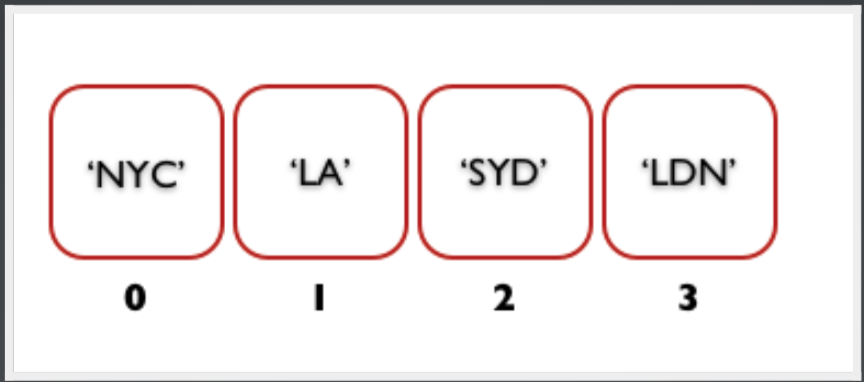
Array Indexing
newArr = ['Zebra',,true,21]
console.log( newArr[0] ) //Zebra
console.log( newArr[1] ) //undefined
console.log( newArr[2] ) //true
console.log( newArr[3] ) //21
Elements can be accessed by their index numbers
Array Indexing
newArr = ['Zebra',,true,21]
newArr[1] = 'Bob'
console.log( newArr )
// ['Zebra','Bob',true,21]
Elements can be updated by using the index at that position
Array Length
console.log( newArr.length ) //4
How do you determine how many elements are in your array?
Array Iteration
let bestColors = ['green','blue','yellow','black']
for(let i = 0; i < bestColors.length;i++){
console.log( bestColors[i] )
}
Iterates through an array passing in the value and index of each element
Array Iteration
let bestColors = ['green','blue','yellow','black']
bestColors.forEach((x,i)=> console.log(x))
Iterates through an array passing in the value and index of each element
Let's Code
Space Review
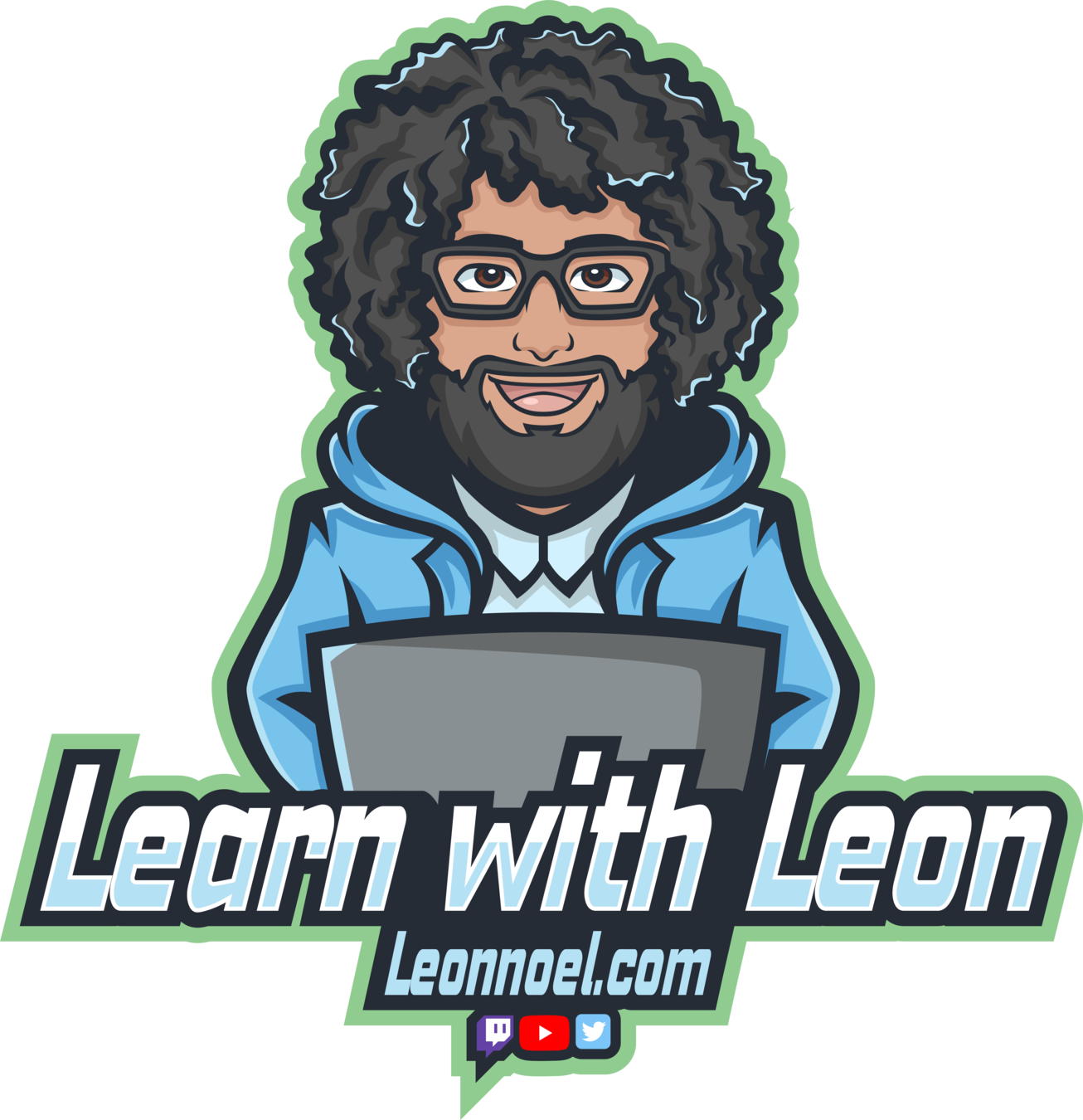
Objects
What are objects?
Objects
-
Objects are a collection of variables and functions!
-
Objects represent the attributes and behavior of something used in a program
-
Object variables are called properties and object functions are called methods
-
Objects store "keyed" collections
Think of a physical object
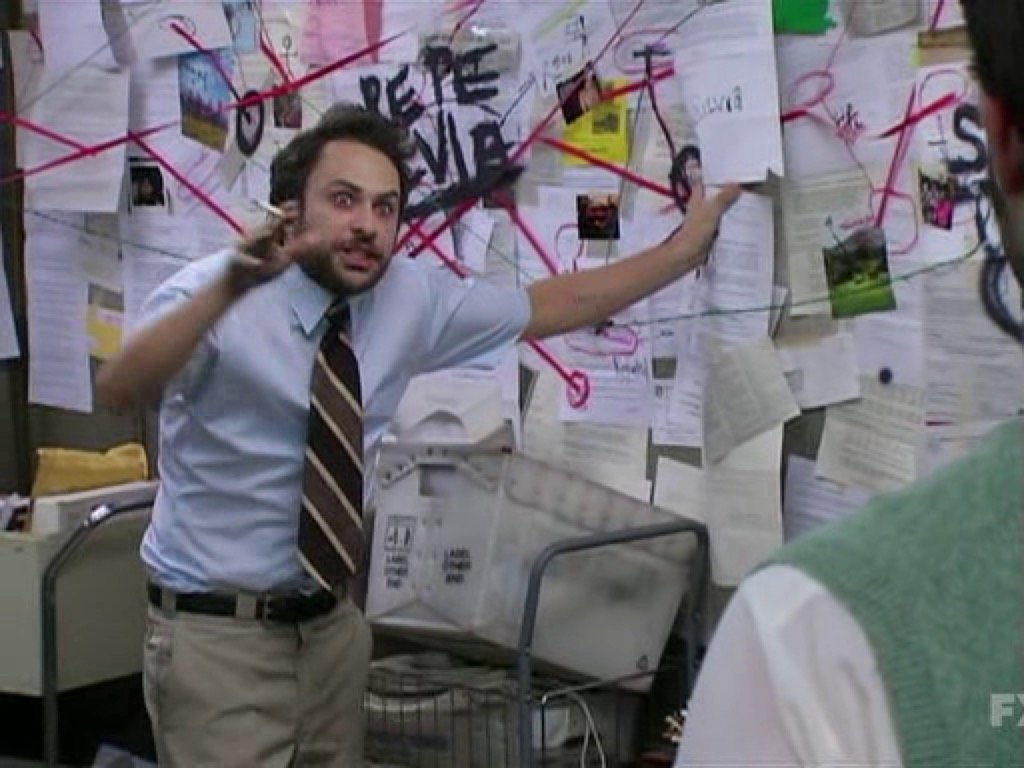
What are it's attributes and behaviors?
How about a stopwatch
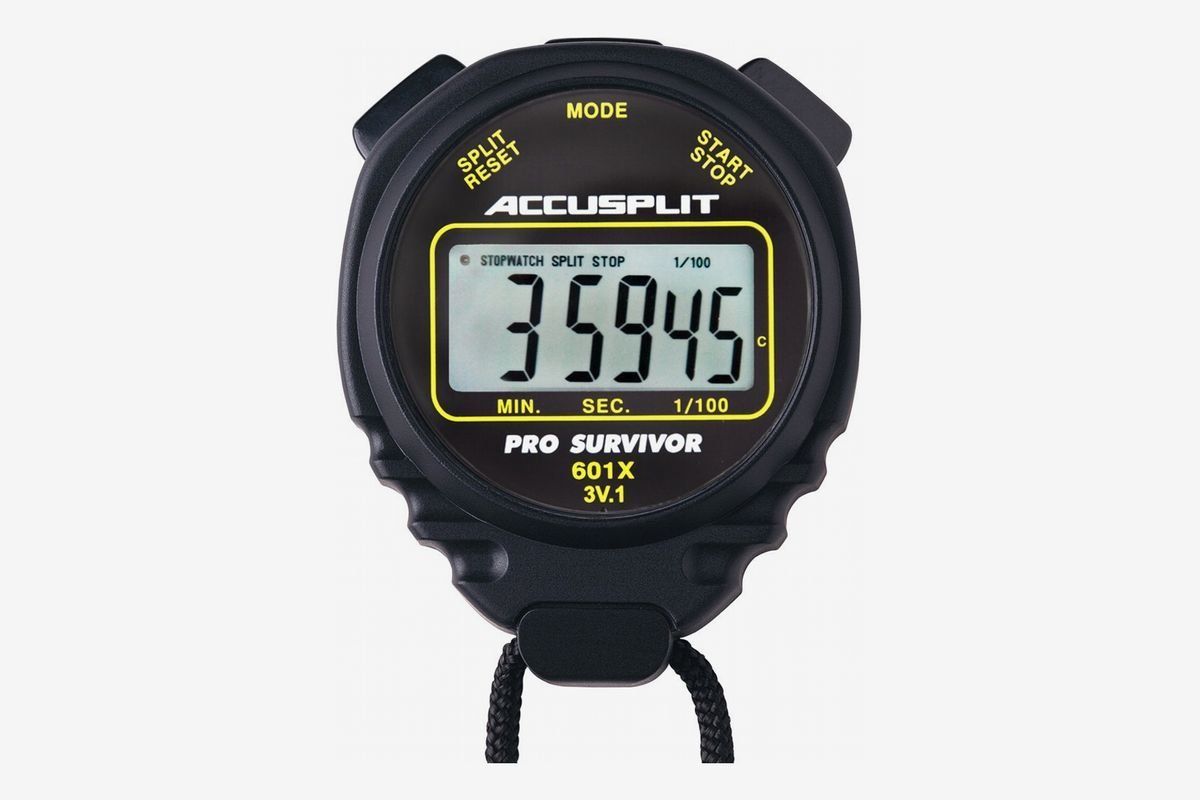
What are its attributes and behaviors?
Stopwatch Object
-
Properties (attributes):
Might contain a variable to represent hours, another to represent minutes, and another to represent seconds.
-
Methods (behaviors):
Might also contain functions that manipulate those values, such as "start ()" and "stop ()".
Stopwatch Object
let stopwatch = {}
stopwatch.currentTime = 12
stopwatch.tellTime = function(time){
console.log(`The current time is ${time}.`)
}
stopwatch.tellTime(stopwatch.currentTime)
Let's Code
Objects - Lost Galaxy
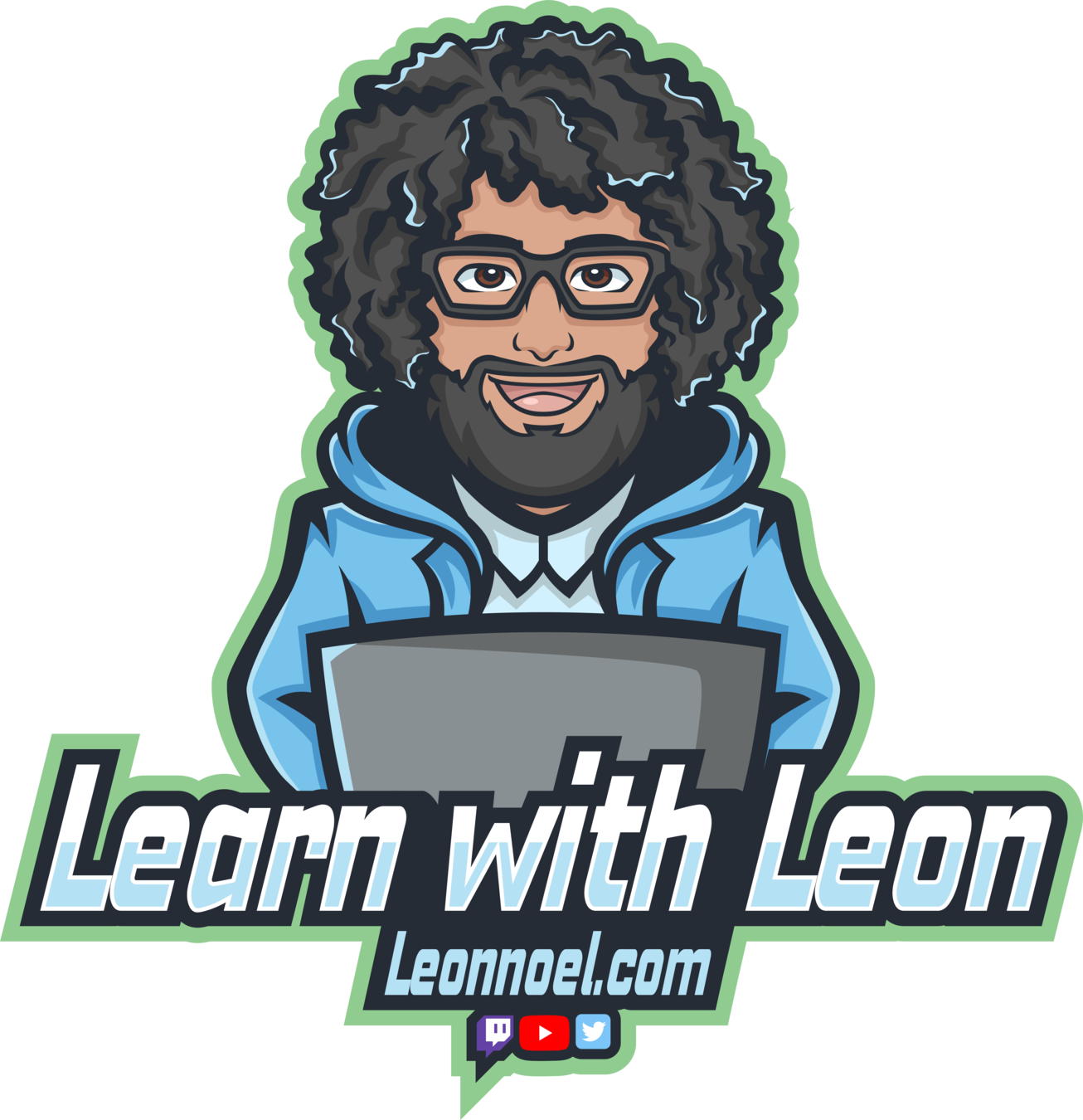
Objects
What if we want to make
a lot of objects?
How much money you got? How many problems you got? How many people done doubted you? Left you out to rot?
Car Factory?
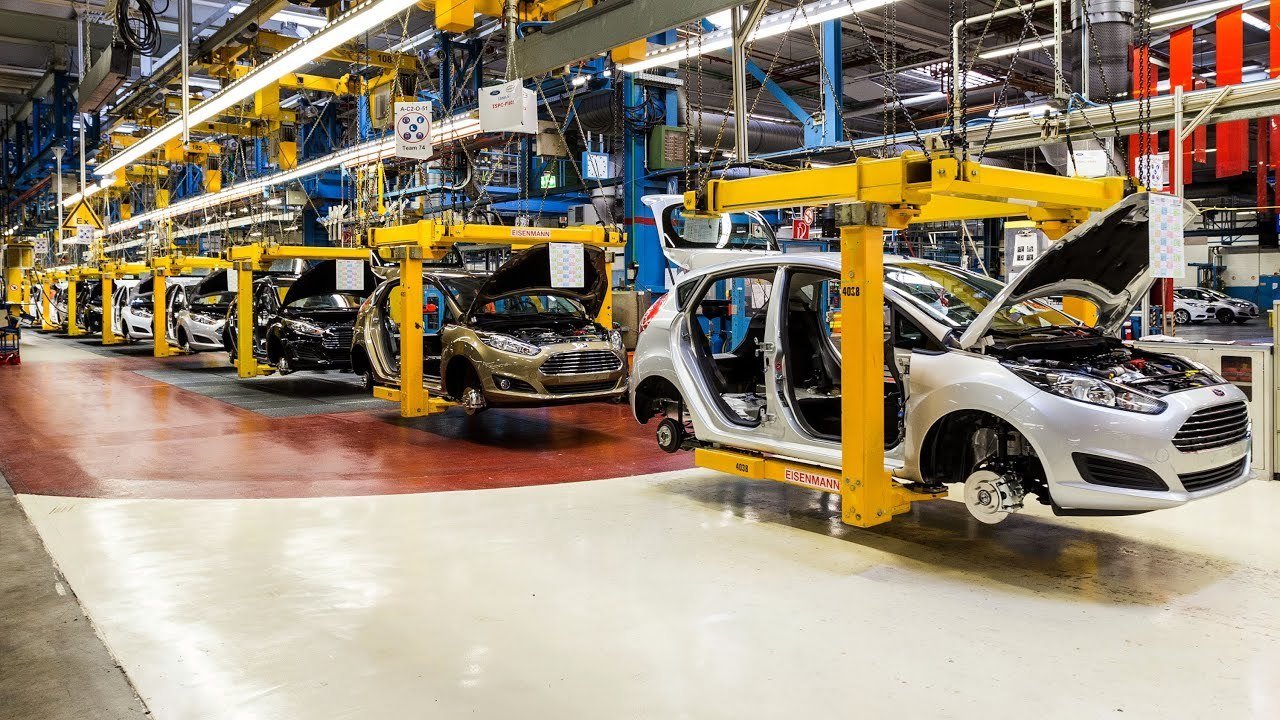
Constructors then Classes
Car Factory
Constructor
function MakeCar(carMake,carModel,carColor,numOfDoors){
this.make = carMake
this.model = carModel
this.color = carColor
this.doors = numOfDoors
this.honk = function(){
alert('BEEP BEEP FUCKER')
}
this.lock = function(){
alert(`Locked ${this.doors} doors!`)
}
}
let hondaCivic = new MakeCar('Honda','Civic','Silver', 4)
let teslaRoadster = new MakeCar('Tesla','Roadster', 'Red', 2)
Car Factory
We forgot enable bluetooth!
let teslaRoadster = new MakeCar('Tesla','Roadster', 'Red', 2)
console.log( teslaRoadster.bluetooth ) //undefined
MakeCar.prototype.bluetooth = true
console.log( teslaRoadster.bluetooth ) //true
A prototype is another object that is used as a fallback source of properties
Car Factory
Why does .toString() work?!?
let teslaRoadster = new MakeCar('Tesla','Roadster', 'Red', 2)
console.log( teslaRoadster.doors.toString() ) // "2" not 2
A prototype is another object that is used as a fallback source of properties
Let's Code
Objects - Tony Hawk Pro Skater
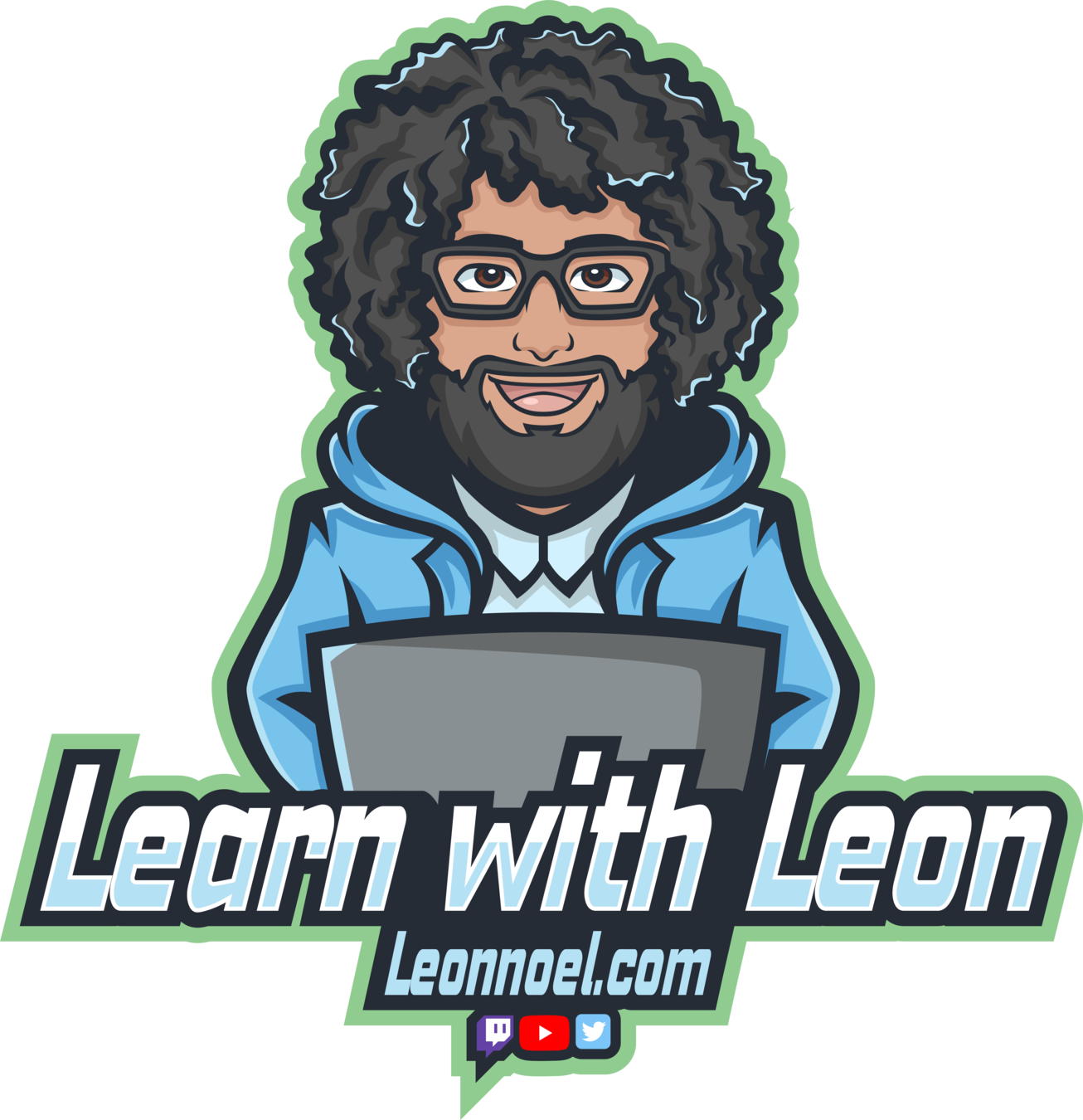
Car Factory
Look Ma! New syntax!
class MakeCar{
constructor(carMake,carModel,carColor,numOfDoors){
this.make = carMake
this.model = carModel
this.color = carColor
this.doors = numOfDoors
}
honk(){
alert('BEEP BEEP FUCKER')
}
lock(){
alert(`Locked ${this.doors} doors!`)
}
}
let hondaCivic = new MakeCar('Honda','Civic','Silver', 4)
let teslaRoadster = new MakeCar('Tesla','Roadster', 'Red', 2)
Classes are like templates for objects!
APIs
What are APIs?
APIs
-
A simple interface for some complex action!
-
Think of a restaurant menu! Remember those...
-
Lets one thing communicate with another thing without having to know how things are implemented.
APIs
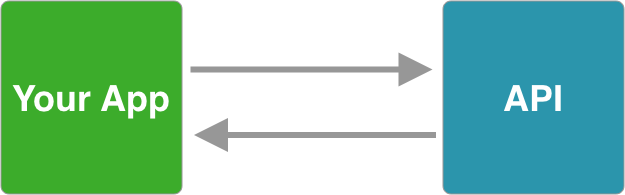
APIs
Fetch Fido, Fetch!
fetch("https://dog.ceo/api/breeds/image/random")
.then(res => res.json()) // parse response as JSON
.then(data => {
console.log(data)
})
.catch(err => {
console.log(`error ${err}`)
});
API returns a JSON object that we can use within our apps
Let's Code
DOG PHOTOS!
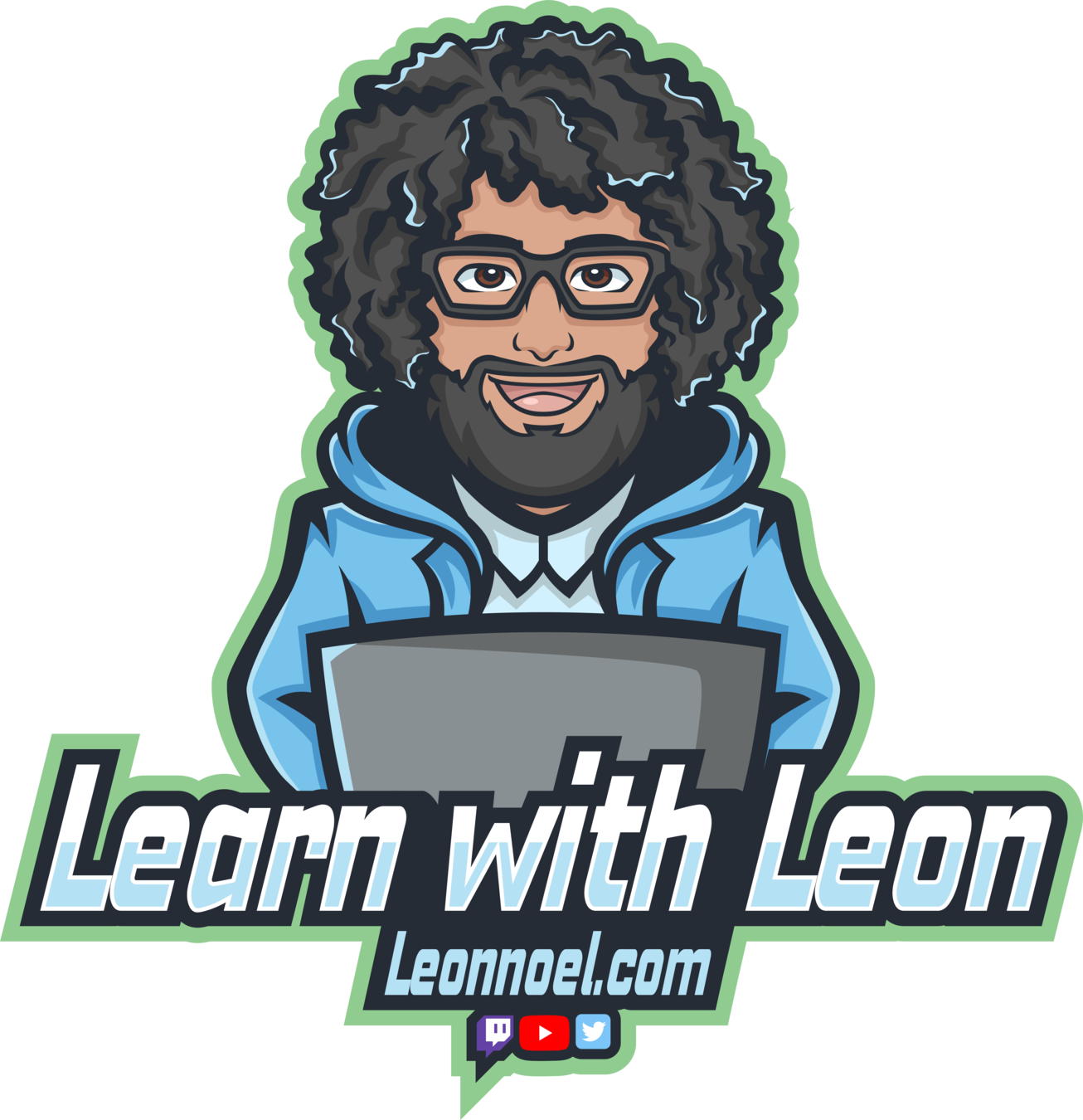
APIs
Stop trying to make Fetch happen!
fetch(url)
.then(res => res.json()) // parse response as JSON
.then(data => {
console.log(data)
})
.catch(err => {
console.log(`error ${err}`)
});
Some APIs need Query Parameters to return the correct data
const url = 'https://www.thecocktaildb.com/api/json/v1/1/search.php?s=margarita'
Let's Code
EVERYBODY! SHOTS! SHOTS! SHOTS!
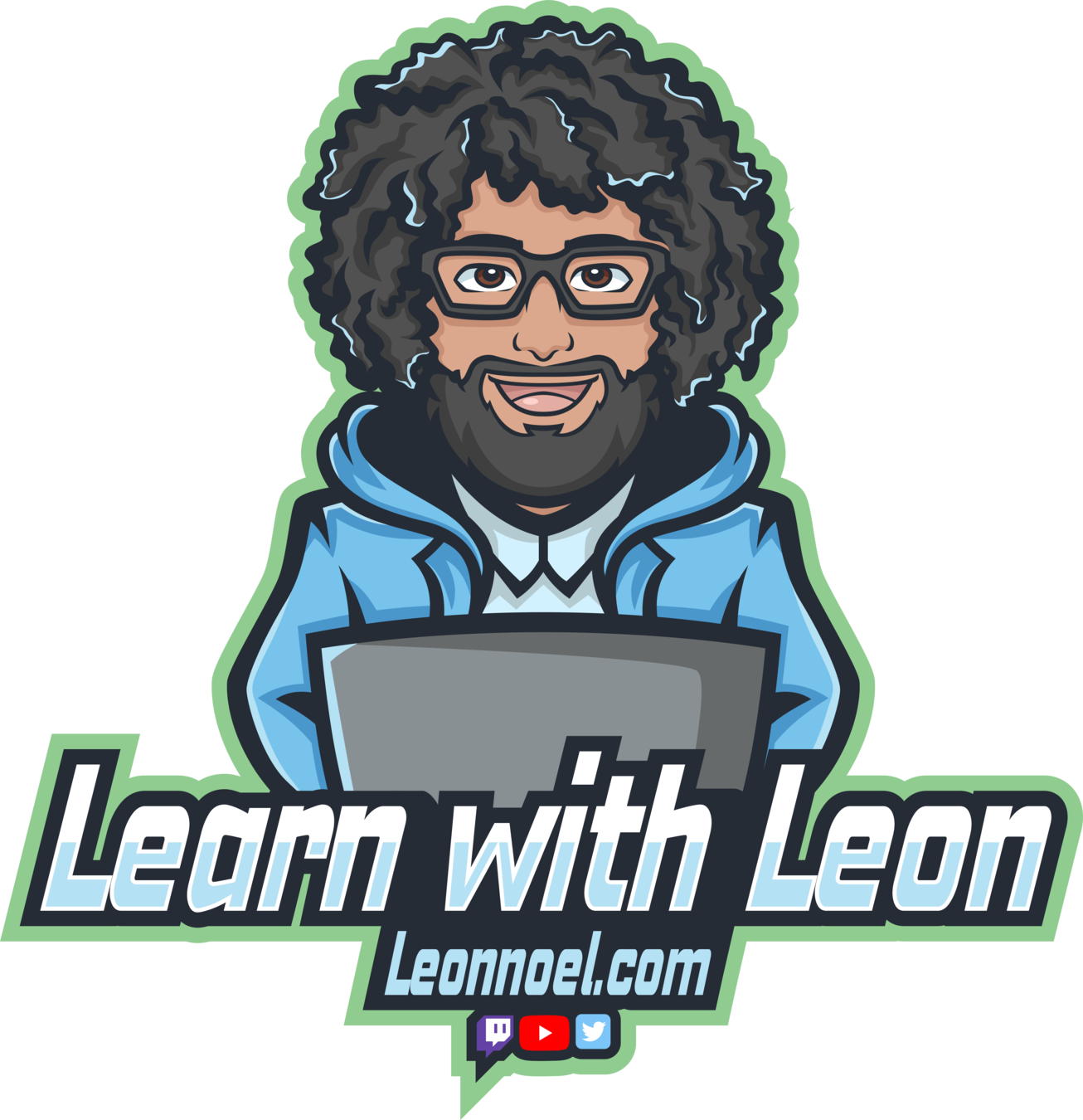
Let's Code
NASA PHOTOS
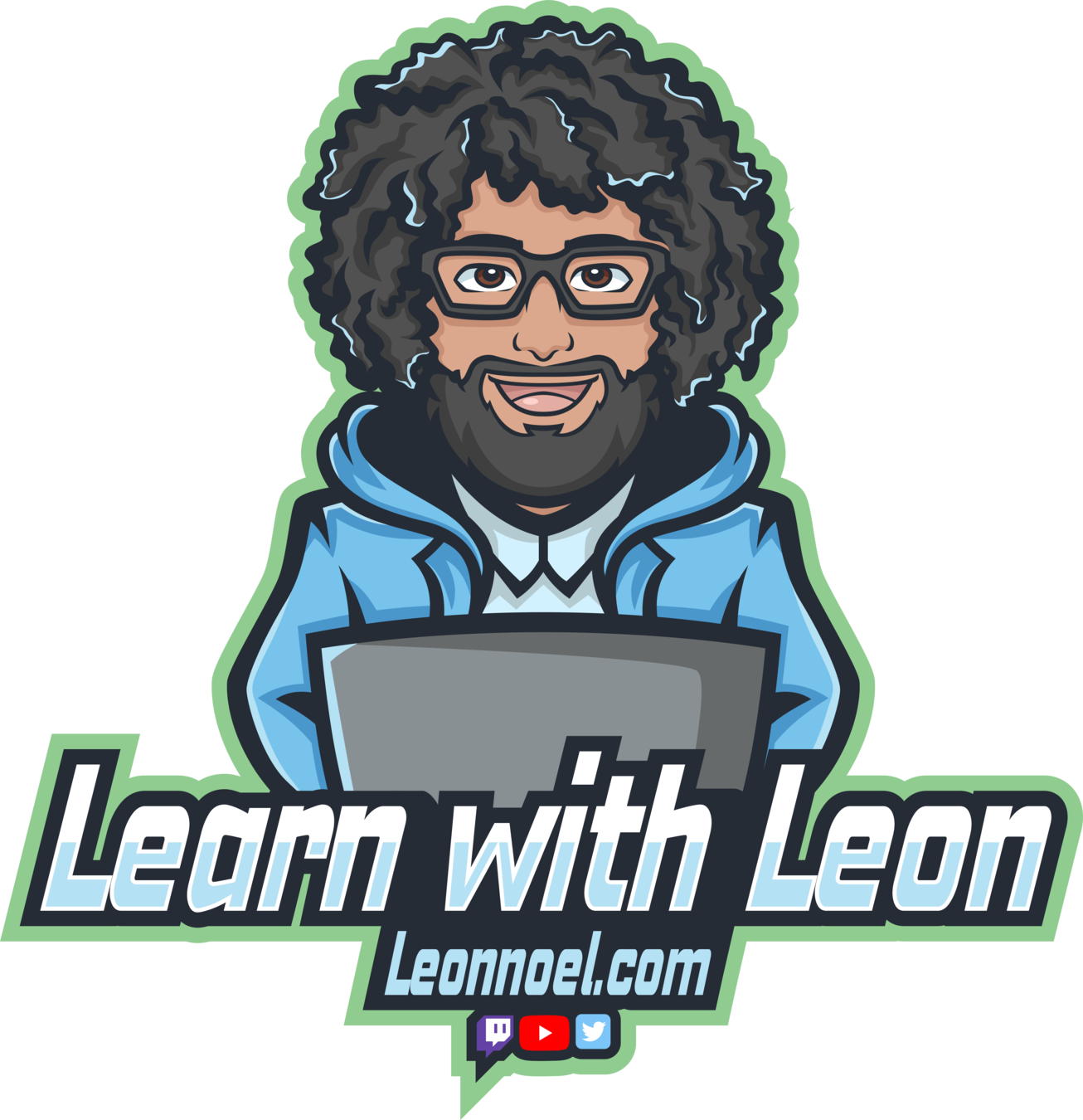
Homework
Read: Pillars of OOP - https://medium.com/@hamzzza.ahmed95/four-pillars-of-object-oriented-programming-oop-e8d7822aa219
Watch / Do: https://youtu.be/PFmuCDHHpwk
Watch: https://youtu.be/0fKg7e37bQE
Do: Four Codewars Fundamentals
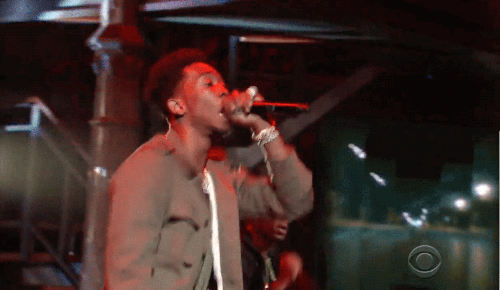
#100Devs - Javascript Objects Fun Time
By Leon Noel
#100Devs - Javascript Objects Fun Time
Class 23 of our Free Web Dev Bootcamp for folx affected by the pandemic. Join live T/Th 6:30pm ET leonnoel.com/twitch and ask questions here: leonnoel.com/discord
- 3,401