Javascript - OOP
Leon Noel
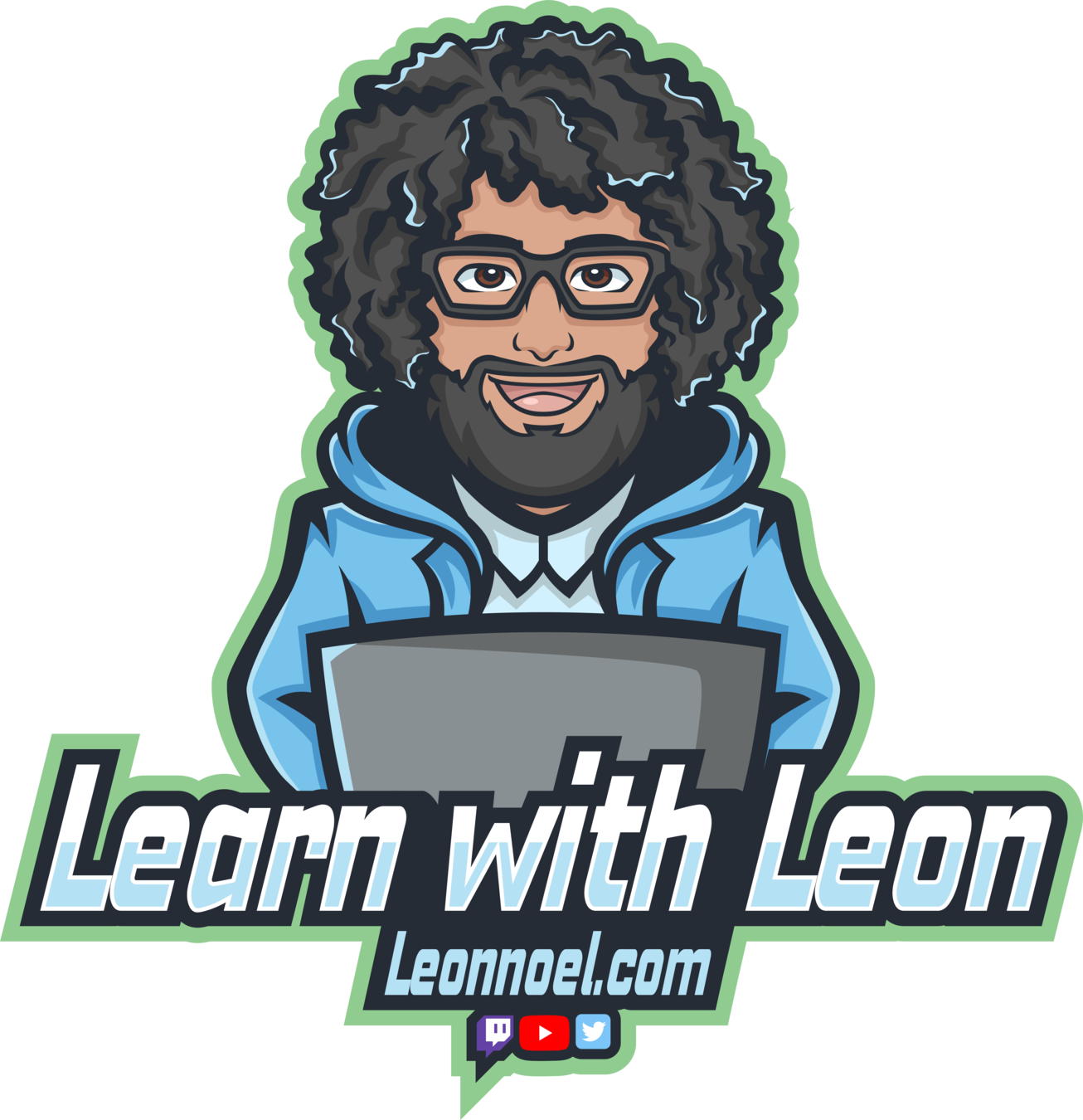
#100Devs
And I was thinking 'bout starting up my own school
A Montessori
And the hallway looking like a monastery, oh yes
I'm way up, I feel blessed
Agenda
-
Questions?
-
Let's Talk - #100Devs
-
Learn - Objects
-
Learn - Basic OOP Principles
-
Do - Work In Teams: Tic-tac-toe
-
Homework - Object City Yall
Questions
About last class or life
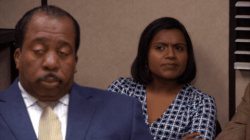
Checking In
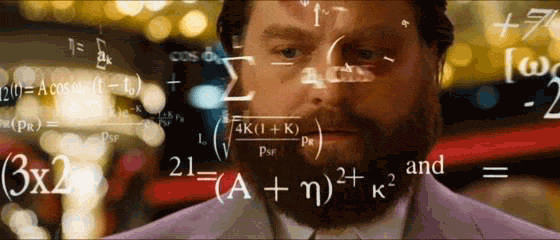
Like and Retweet the Tweet
!checkin
Trough Of Sorrow
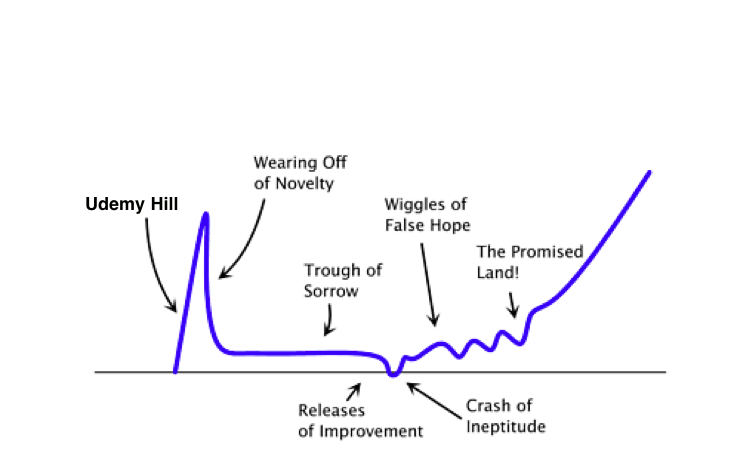
No Networking Until May
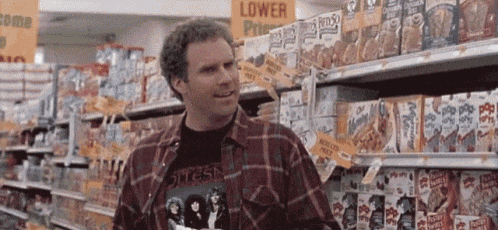
Client Deadline: March 5th
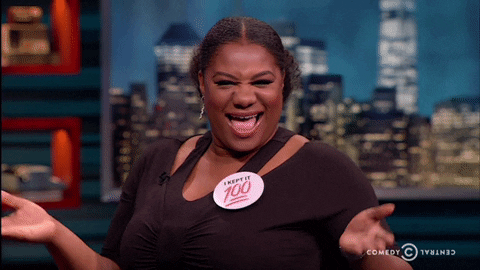
Client Alternatives
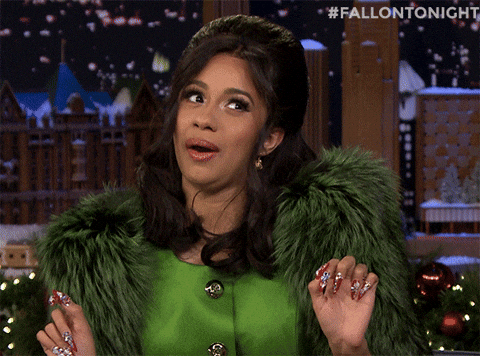
Grassroots Volunteer*
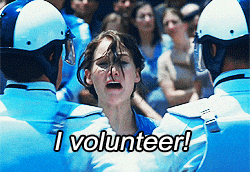
Free Software / Open Source
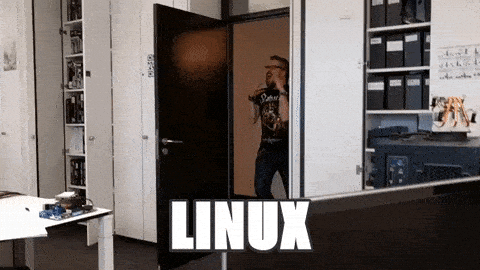
Live Crafting Your Story
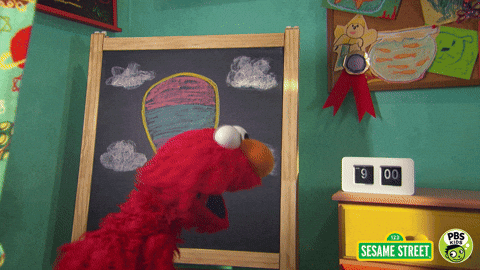
FRIDAY
6:30pm EST
OFFICE HOURS
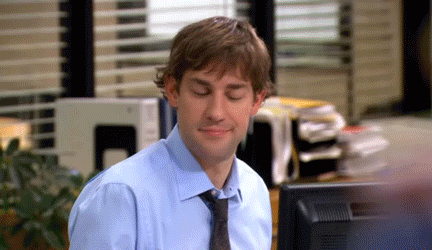
SUNDAY
1:00pm EST
Objects
What are objects?
USE
UNDERSTAND
BUILD
Finna Make Sum Nerds Angry
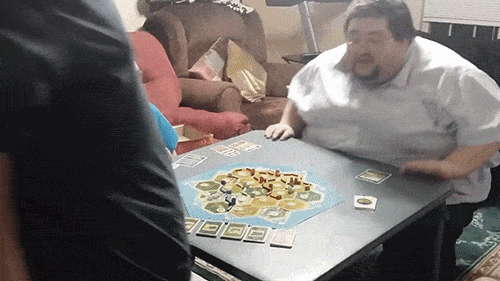
Objects
-
Objects are a collection of variables and functions!
-
Objects represent the attributes and behavior of something used in a program
-
Object variables are called properties and object functions are called methods
-
Objects store "keyed" collections
Think of a physical object
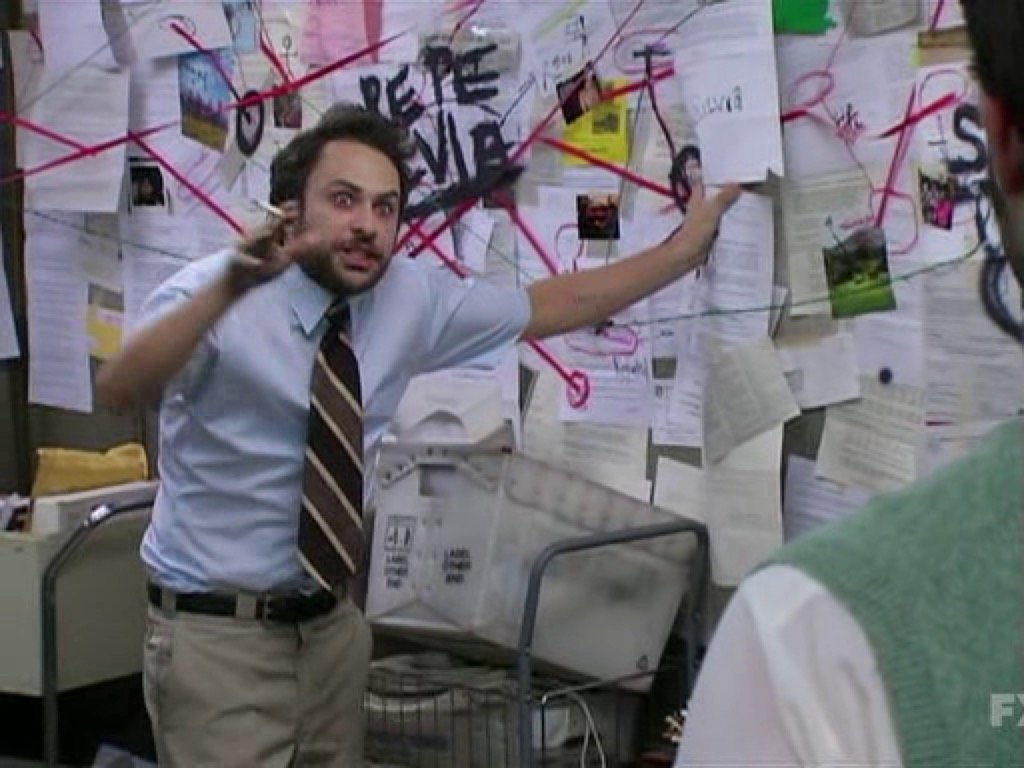
What are it's attributes and behaviors?
How about a stopwatch
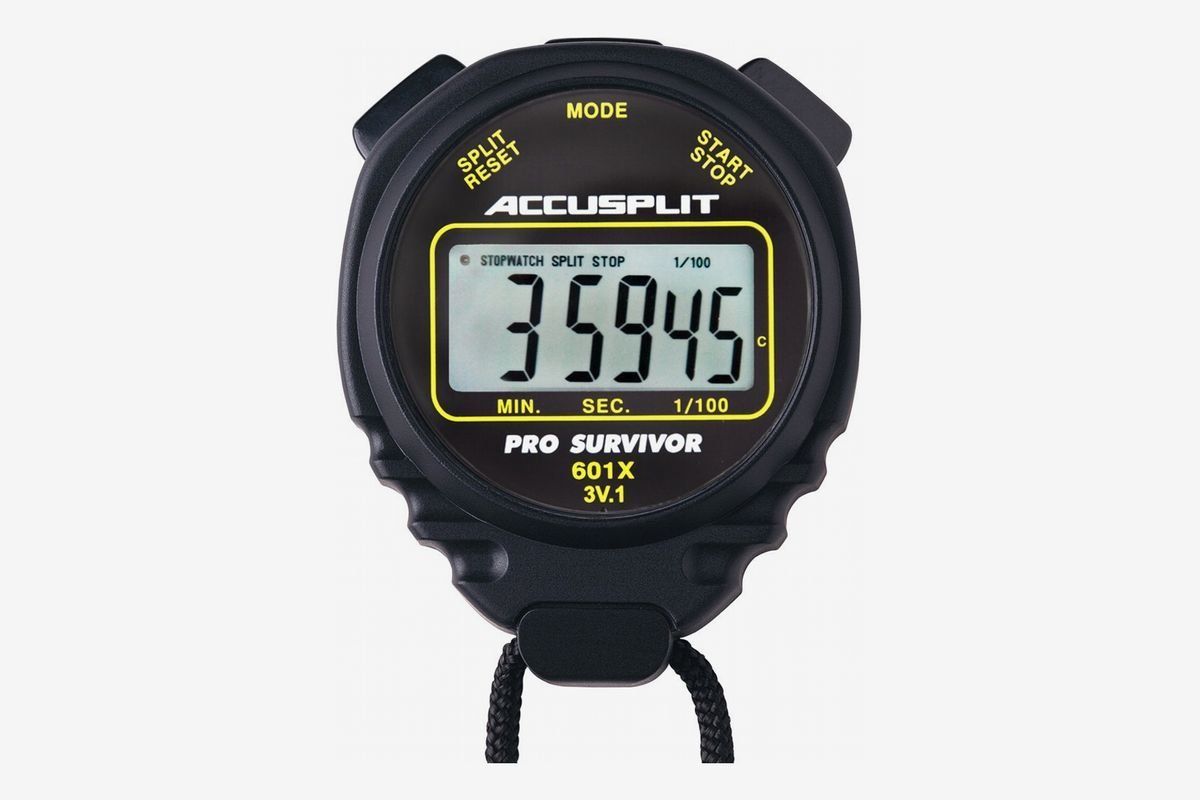
What are its attributes and behaviors?
Stopwatch Object
-
Properties (attributes):
Might contain a variable to represent hours, another to represent minutes, and another to represent seconds.
-
Methods (behaviors):
Might also contain functions that manipulate those values, such as "start ()" and "stop ()".
Stopwatch Object
let stopwatch = {}
stopwatch.currentTime = 12
stopwatch.tellTime = function(time){
console.log(`The current time is ${time}.`)
}
stopwatch.tellTime(stopwatch.currentTime)
Notation?
Objects
What if we want to make
a lot of objects?
How much money you got? How many problems you got? How many people done doubted you? Left you out to rot?
Car Factory?
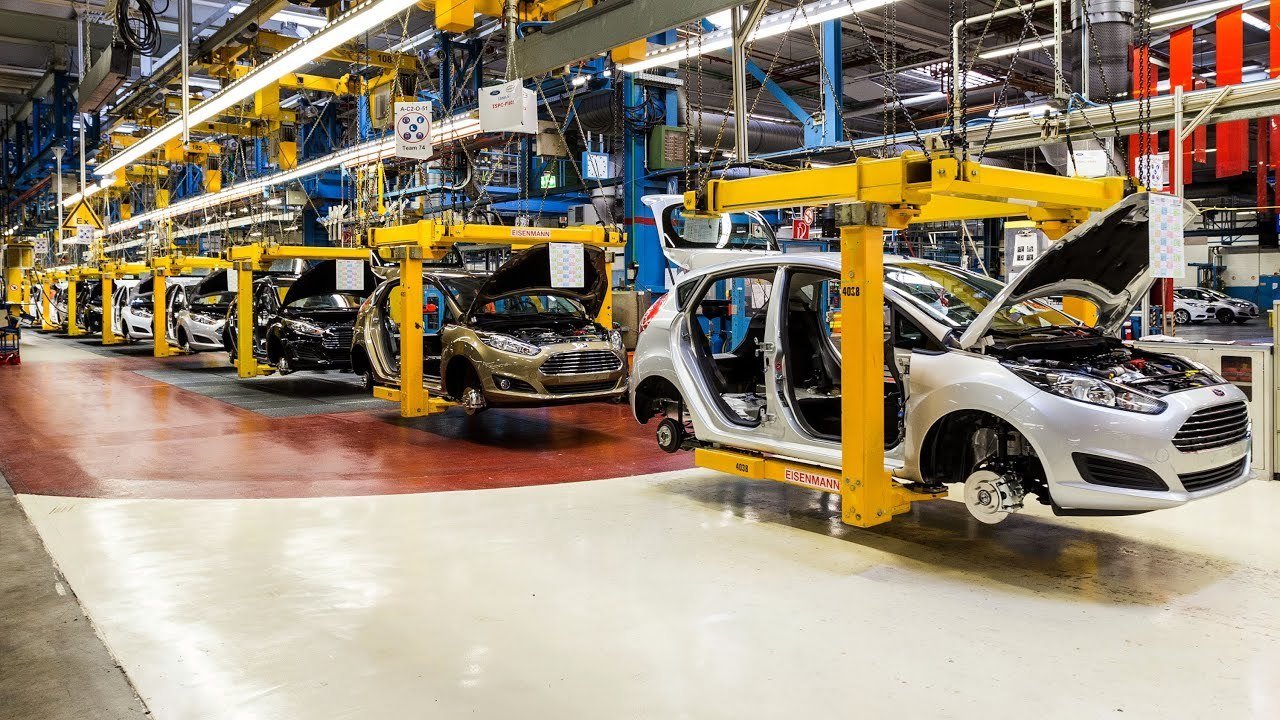
Constructors then Classes
Car Factory
Constructor
function MakeCar(carMake,carModel,carColor,numOfDoors){
this.make = carMake
this.model = carModel
this.color = carColor
this.doors = numOfDoors
this.honk = function(){
alert('BEEP BEEP FUCKER')
}
this.lock = function(){
alert(`Locked ${this.doors} doors!`)
}
}
let hondaCivic = new MakeCar('Honda','Civic','Silver', 4)
let teslaRoadster = new MakeCar('Tesla','Roadster', 'Red', 2)
Car Factory
We forgot enable bluetooth!
let teslaRoadster = new MakeCar('Tesla','Roadster', 'Red', 2)
console.log( teslaRoadster.bluetooth ) //undefined
MakeCar.prototype.bluetooth = true
console.log( teslaRoadster.bluetooth ) //true
A prototype is another object that is used as a fallback source of properties
Car Factory
Why does .toString() work?!?
let teslaRoadster = new MakeCar('Tesla','Roadster', 'Red', 2)
console.log( teslaRoadster.doors.toString() ) // "2" not 2
A prototype is another object that is used as a fallback source of properties
Car Factory
Look Ma! New syntax!
class MakeCar{
constructor(carMake,carModel,carColor,numOfDoors){
this.make = carMake
this.model = carModel
this.color = carColor
this.doors = numOfDoors
}
honk(){
alert('BEEP BEEP FUCKER')
}
lock(){
alert(`Locked ${this.doors} doors!`)
}
}
let hondaCivic = new MakeCar('Honda','Civic','Silver', 4)
let teslaRoadster = new MakeCar('Tesla','Roadster', 'Red', 2)
Classes are like templates for objects!
Let's Code
Objects - Espresso Machine
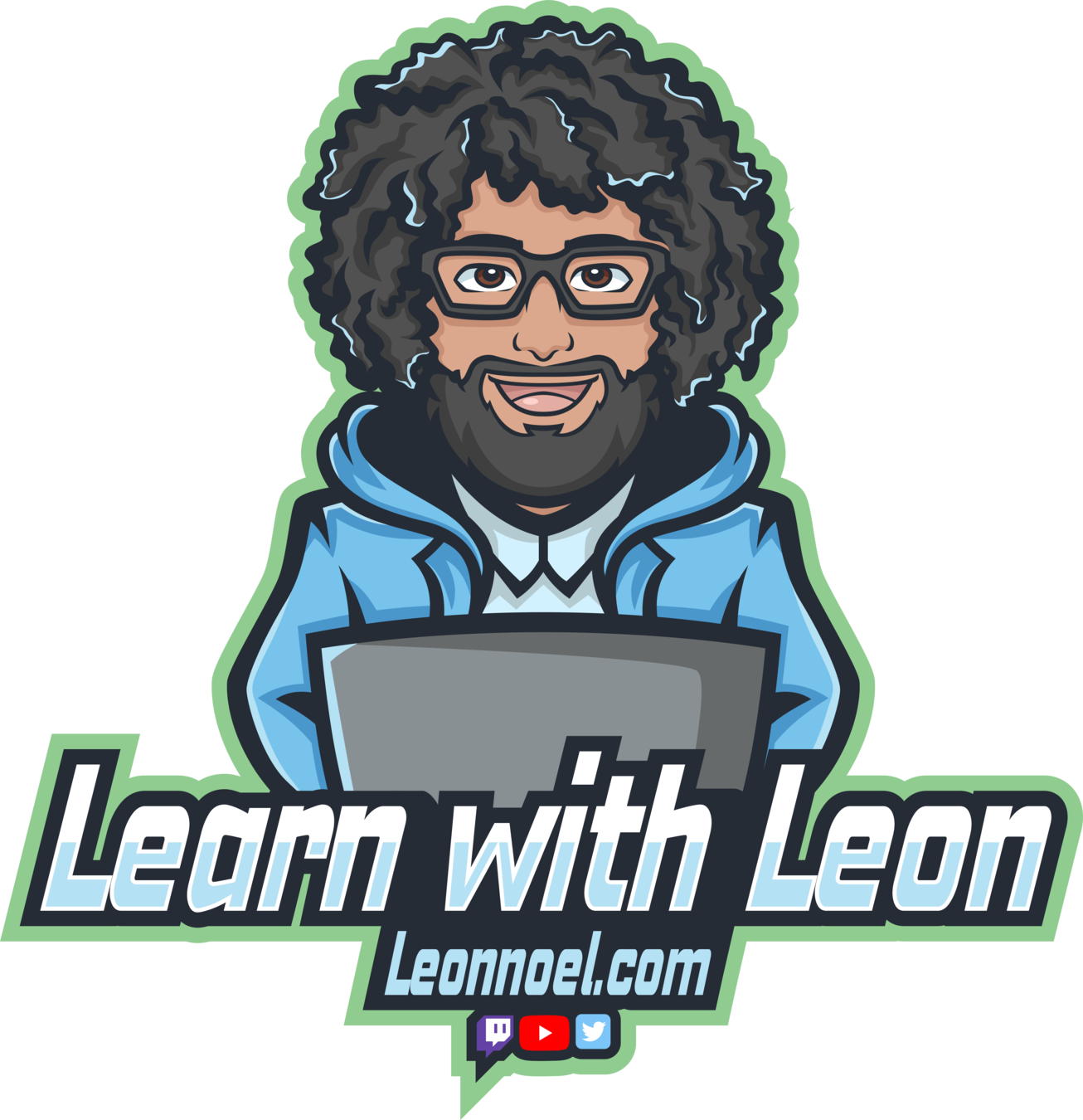
BUT WHY?
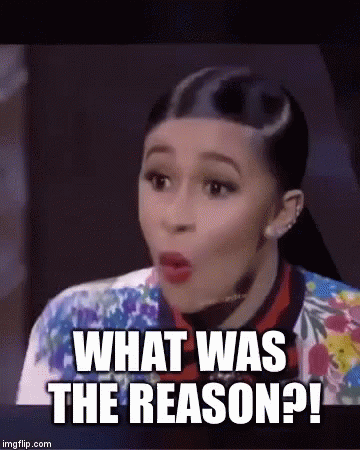
Why Use Objects?
Why Would We Need A Factory?
As our codebase gets larger and more folx join the team, can we keep our code organized?
Is it easy to add new features and functionality?
Can another developer look at my code and understand what is happening?
Can I make changes without losing sleep at night?
What if there was a system, a paradigm, a set of rules, an agreed upon way to structure our code that:
Made it easier to add new stuff
Made it easier to read through what was already coded
And made it so you were not afraid to make changes
Music & Light Warning - Next Slide
OOP BABY
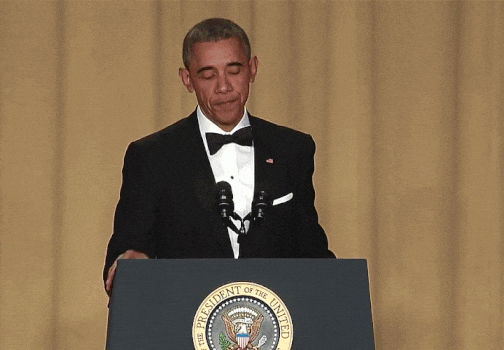
OBJECT ORIENTED PROGRAMING
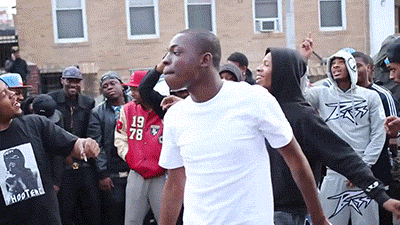
Let's See Some Code
let hourlyRate = 250
let hours = 160
let taxRate = .35
function calculateProfit(rate, numOfHours, taxes){
return rate * numOfHours * (1 - taxes)
}
let profit = calculateProfit(hourlyRate, hours, taxRate)
console.log(profit)
Is it easy to add new features and functionality?
let hourlyRate = 250
let hours = 160
let taxRate = .35
function calculateProfit(rate, numOfHours, taxes){
return rate * numOfHours * (1 - taxes)
}
function holdForTaxes(profitMade){
return hourlyRate * hours - profitMade
}
let profit = calculateProfit(hourlyRate, hours, taxRate)
let taxesHeld = holdForTaxes(profit)
console.log(profit)
console.log(taxesHeld)
Can another developer look at my code and understand what is happening?
let hourlyRate = 250
let hours = 160
let taxRate = .35
function calculateProfit(rate, numOfHours, taxes){
return rate * numOfHours * (1 - taxes)
}
function holdForTaxes(profitMade){
return hourlyRate * hours - profitMade
}
let profit = calculateProfit(hourlyRate, hours, taxRate)
let taxesHeld = holdForTaxes(profit)
console.log(profit)
console.log(taxesHeld)
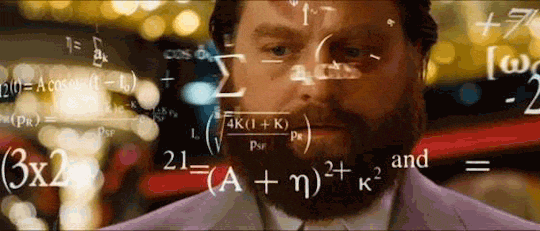
let hourlyRate = 250
let hours = 160
let taxRate = .35
function calculateProfit(rate, numOfHours, taxes){
return rate * numOfHours * (1 - taxes)
}
let profit = calculateProfit(hourlyRate, hours, taxRate)
console.log(profit)
Can I make changes without losing sleep at night?
let hourlyRate = 250
let hours = 160
let taxRate = .35
function calculateProfit(rate, numOfHours, taxes){
return rate * numOfHours * (1 - taxes)
}
function holdForTaxes(profitMade){
return hourlyRate * hours - profitMade
}
let profit = calculateProfit(hourlyRate, hours, taxRate)
let taxesHeld = holdForTaxes(profit)
console.log(profit)
console.log(taxesHeld)
DATA & FUNCTIONALITY
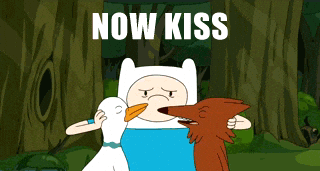
let hourlyRate = 250
let hours = 160
let taxRate = .35
DATA
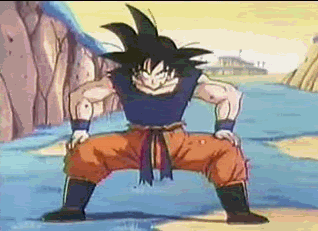
Functionality
function calculateProfit(rate, numOfHours, taxes){
return rate * numOfHours * (1 - taxes)
}
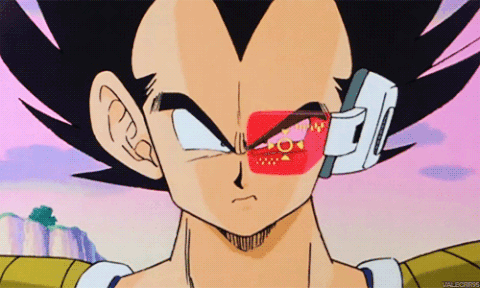
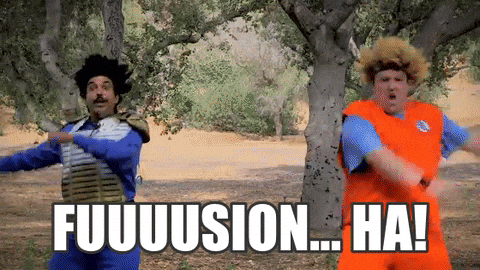
let seriousBusinessPerson = {
hourlyRate: 250,
hours: 160,
taxRate: .35,
calculateProfit: function() {
return this.hourlyRate * this.hours * (1 - this.taxRate)
}
}
Is it easy to add new features and functionality?
let seriousBusinessPerson = {
hourlyRate: 250,
hours: 160,
taxRate: .35,
calculateProfit: function() {
return this.hourlyRate * this.hours * (1 - this.taxRate)
}
}
let seriousBusinessPerson = {
hourlyRate: 250,
hours: 160,
taxRate: .35,
calculateProfit: function() {
return this.hourlyRate * this.hours * (1 - this.taxRate)
}
calculateTaxesHeld: function(){
return this.hourlyRate * this.hours - this.calculateProfit()
}
}
Can another developer look at my code and understand what is happening?
let seriousBusinessPerson = {
hourlyRate: 250,
hours: 160,
taxRate: .35,
calculateProfit: function() {
return this.hourlyRate * this.hours * (1 - this.taxRate)
}
}
Can I make changes without losing sleep at night?
let seriousBusinessPerson = {
hourlyRate: 250,
hours: 160,
taxRate: .40, //changed
calculateProfit: function() {
return this.hourlyRate * this.hours * (1 - this.taxRate)
}
}
This fusion of data and functionality into one object
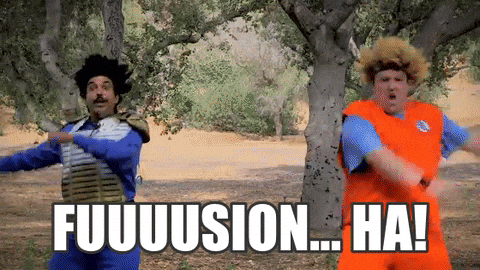
Music & Light Warning - Next Slide
Encapsulation Baby
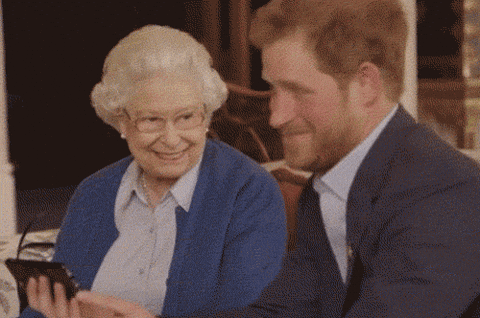
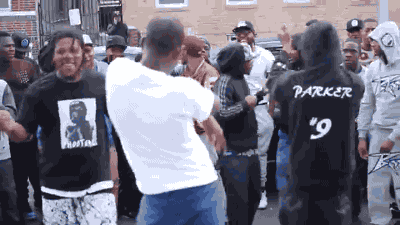
Encapsulation
The process of storing functions (methods) with their associated data (properties) - in one thing (object)*
*Nerds shaking violently
Is it easy to add new features and functionality?
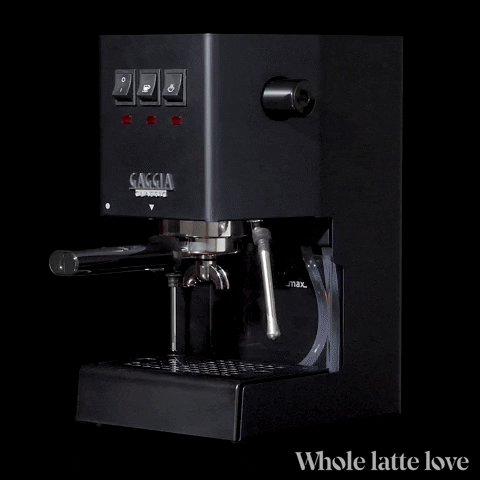
Heats water for espresso and steam for your milk
Steam wand sucks...
What should the engineers do?
Do they get rid of the water boiler?
probably not...
At first, do they even need to think about the water boiler?
So the water boiling is abstracted?
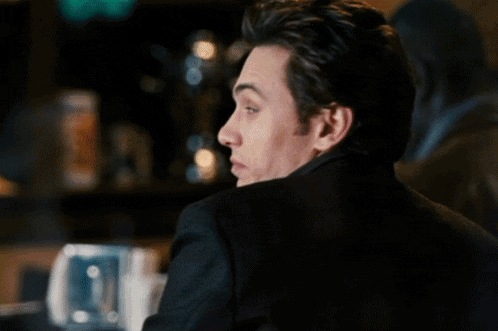
Let's look at some code
function AgencyContractor(hourlyRate, hours, taxRate){
this.hourlyRate = hourlyRate
this.hours = hours
this.taxRate = taxRate
this.calculateProfit = function() {
return this.hourlyRate * this.hours * (1 - this.taxRate)
}
this.invoiceClient = function(){
return `Your invoice total is ${this.hourlyRate * this.hours}`
}
}
let leon = new AgencyContractor(250,160,.35)
console.log( leon.invoiceClient() ) //40000
console.log( leon.hourlyRate ) //250
console.log( leon.calculateProfit() ) //26000
Ut Oh...
I PUT THIS CALCULATOR ON OUR AGENCY WEBSITE...
function AgencyContractor(hourlyRate, hours, taxRate){
this.hours = hours
this.taxRate = taxRate
let rate = hourlyRate
let calculateProfit = function() {
return rate * this.hours * (1 - this.taxRate)
}
this.invoiceClient = function(){
return `Your invoice total is ${rate * this.hours}`
}
}
let leon = new AgencyContractor(250,160,.35)
console.log( leon.invoiceClient() ) //40000
console.log( leon.hourlyRate ) //undefined
console.log( leon.calculateProfit() )
//Uncaught TypeError: leon.calculateProfit is not a function
Better...
*Nerds shaking violently
So now my client can still get their invoice, but not see my hourly rate
Water boiling was hidden from the steam wand
Hourly rate was hidden from the client
Complex or unnecessary details are hidden
This enables you to implement things without understanding or even thinking about all the hidden complexity
Smaller more manageable pieces of code
Do stuff once
Music & Light Warning - Next Slide
Abstraction Baby
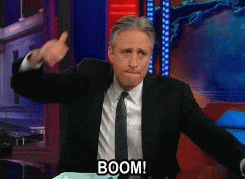
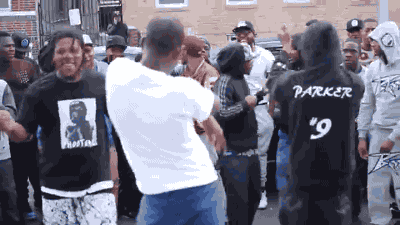
Abstraction
Hide details
and show essentials
SIMPLE, PREDICTABLE, MANAGEABLE
The Four Pillars
What if they actually made any sense...
Encapsulation
The process of storing functions (methods) with their associated data (properties) in one thing (object)
BUT WHY?
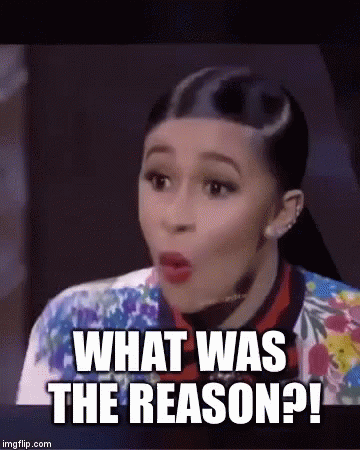
Made it easier to add new stuff
Made it easier to read through what was already coded
And made it so you were not afraid to make changes
Abstraction
Hide details
and show essentials
BUT WHY?
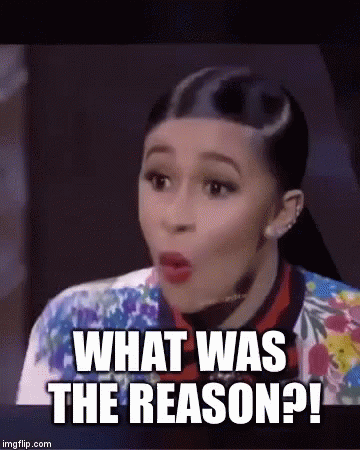
Smaller more manageable pieces of code
Helps you to split the complexity your software project into manageable parts
Inheritance
Polymophism
Next Class
Group Work
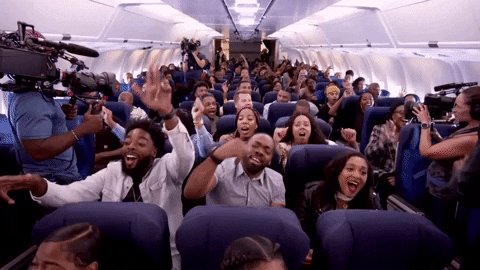
Right Of Passage
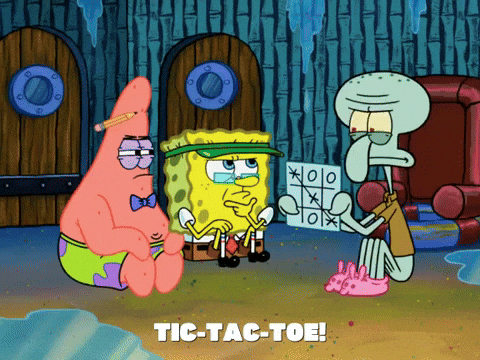
Get It To Work
Then:
Make it easier to add new stuff
Make it easier to read through what was already coded
And make it so you are not afraid to make changes
Homework
Watch / Do: https://youtu.be/PFmuCDHHpwk
Read / Do: JS Way Ch. 09
Push? Read / Do: Eloquent JS Ch. 06
Do: 7 Codewars
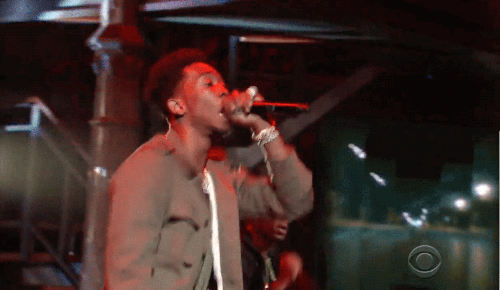
#100Devs - Javascript OOP (cohort 2)
By Leon Noel
#100Devs - Javascript OOP (cohort 2)
Class 30 of our Free Web Dev Bootcamp for folx affected by the pandemic. Join live T/Th 6:30pm ET leonnoel.com/twitch and ask questions here: leonnoel.com/discord
- 3,399