Node & Express
Leon Noel

#100Devs
I ain't know nothin' 'bout no Visa, I was in the park with the gang
Moms be feelin' bad, I try to tell her she is not to blame
No social security, couldn't get a license, but I still didn't complain
Agenda
Questions?
Let's Talk - #100Devs
Review - Event Loop
Learn - CRUD
Learn - Express
Learn - Build your own API
Homework - Build your own API
Questions
About last class or life

Checking In
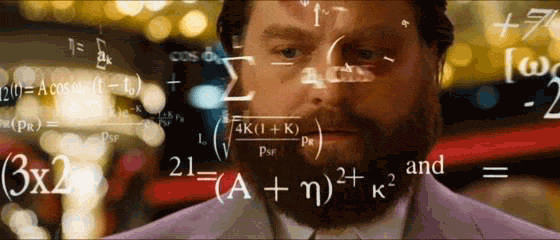
Like and Retweet the Tweet
!checkin
Live CAR Class
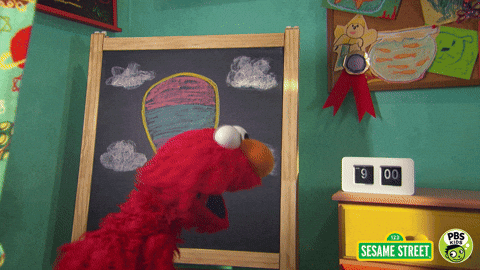
FRIDAY
6:00pm EST
And we are talking about the agency
Office Hours
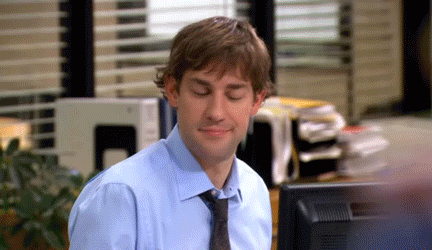
SUNDAY
1:00pm EST
Networking
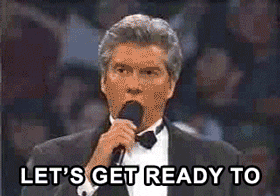
1 coffee chat this week
USE THE SHEET!
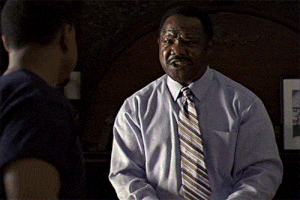
NOW WITH TWO TABS!: Google Sheet
Grab The Checklist
!checklist
PUSH EVERY DAY
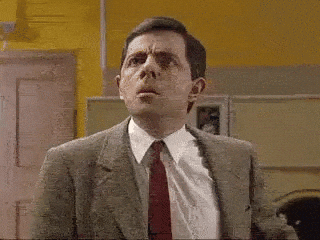
Submitting Work
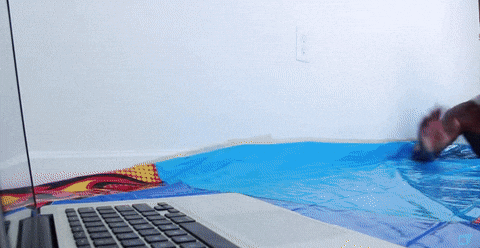
Submitting Work
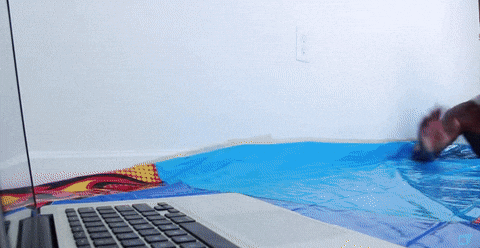
Homework
(should be done)
Spaced Repetition

Ali Abdaal: https://youtu.be/Z-zNHHpXoMM
Backend!
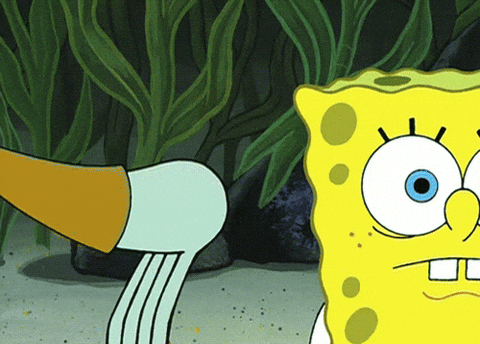
Butt first!
Javascript is
single-threaded
Synchronous aka processes
one operation at a time
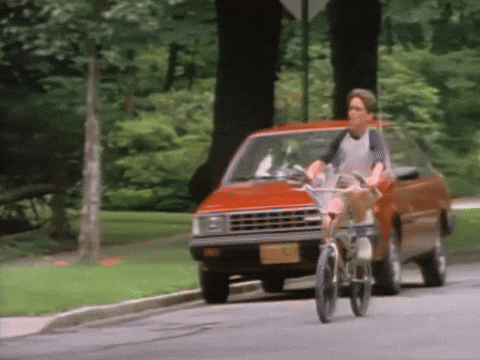
vs
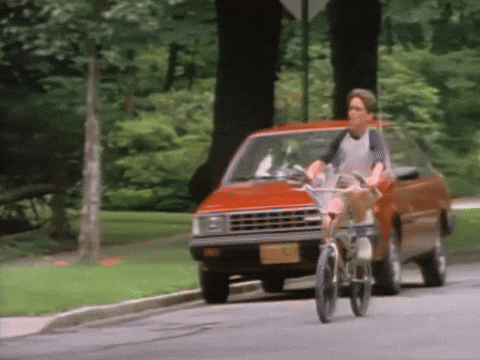
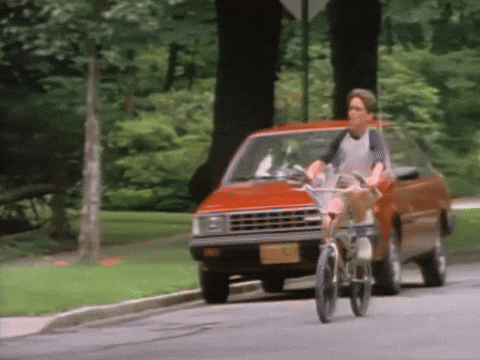
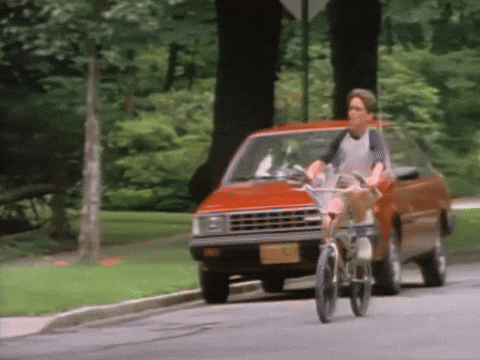
Things should block
THE ENVIRONMENT
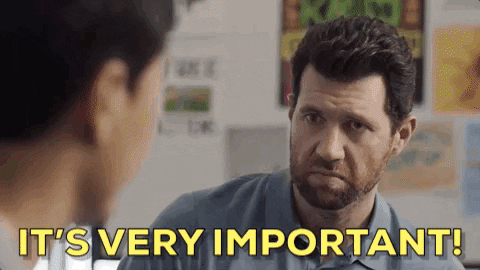
Not This
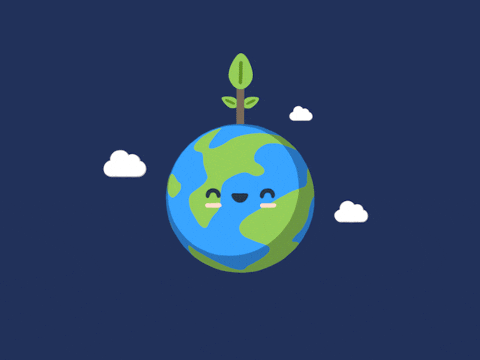
THIS
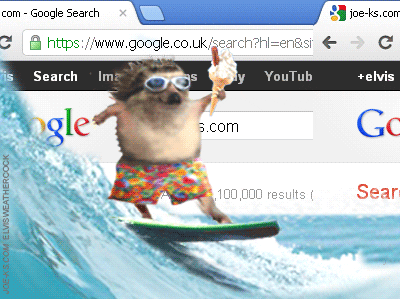
Our JS is running in
a browser
Browsers have a BUNCH of APIs we can use that are async and enable us to keeping looking a cute cat photos while those operations are being processed asynchronously
function houseOne(){
console.log('Paper delivered to house 1')
}
function houseTwo(){
setTimeout(() => console.log('Paper delivered to house 2'), 0)
}
function houseThree(){
console.log('Paper delivered to house 3')
}
houseOne()
houseTwo()
houseThree()
EVENT LOOP
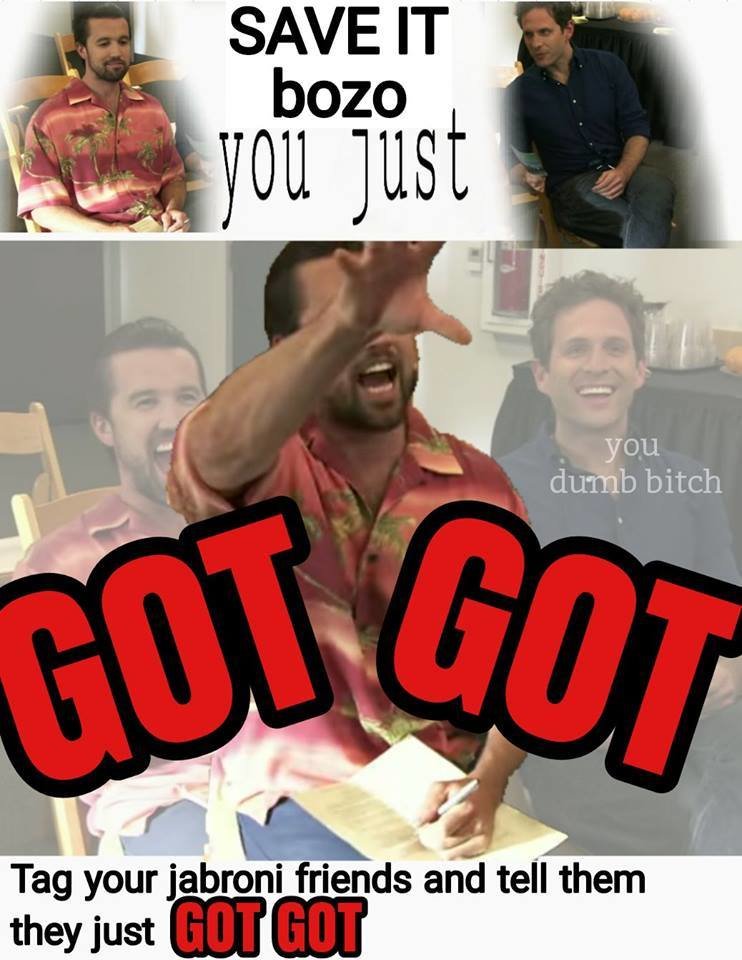
Time for some data structures
Queue

Like a real queue, the first element which was added to the list, will be the first element out.
This is called a FIFO (First In First Out) structure.
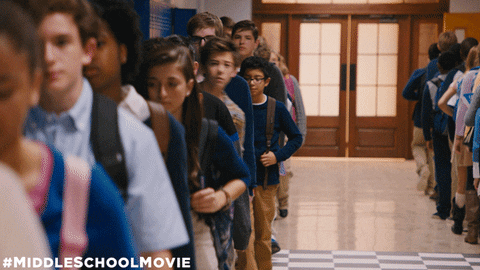
let queue = []
queue.push(2) // queue is now [2]
queue.push(5) // queue is now [2, 5]
let i = queue.shift() // queue is now [5]
alert(i) // displays 2
Queue Example
Stack
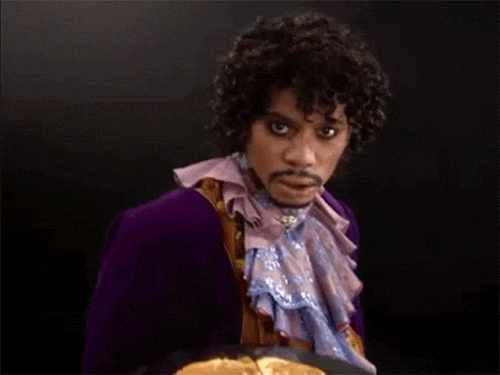
The first pancake made, is the last pancake served.
This is called a stack.
The first element which was added to the list, will be the last one out. This is called a LIFO (Last In First Out) structure.
let stack = []
stack.push(2) // stack is now [2]
stack.push(5) // stack is now [2, 5]
let = stack.pop() // stack is now [2]
alert(i) // displays 5
Stack Example
Back To Getting Got
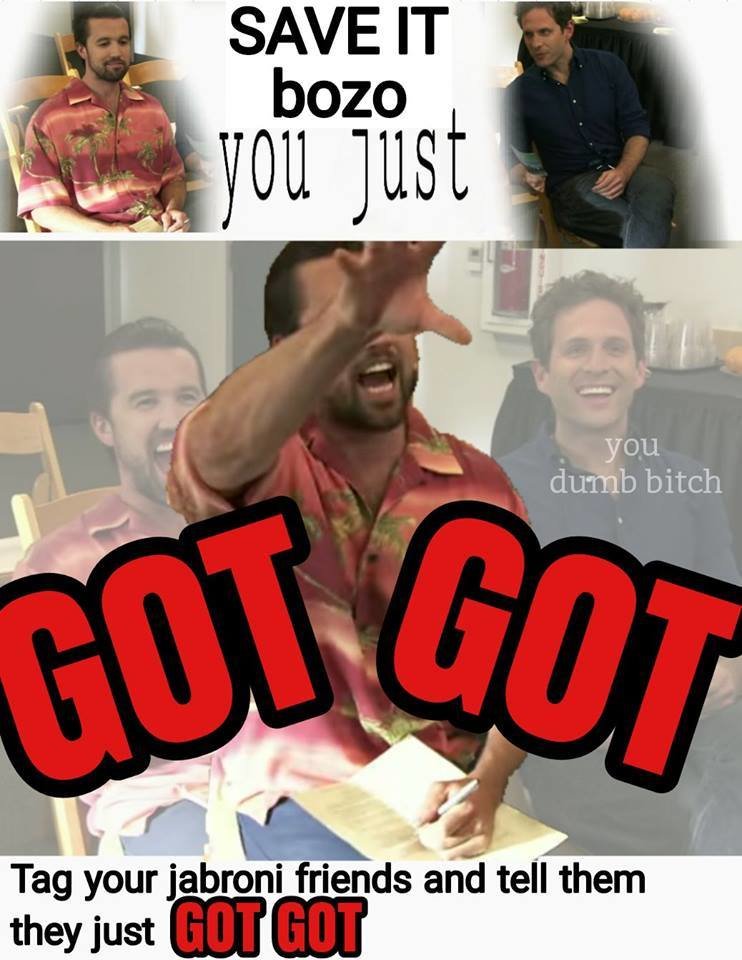
JS IS RUNNING IN THE BROWSER
V8 Engine
(Parse Code > Runnable Commands)
Window Runtime (Hosting Environment)
Gives Us Access To Web APIs
Passes stuff to Libevent (Event Loop)
The Event Loop monitors the Callback Queue and Job Queue and decides what needs to be pushed to the Call Stack.
Call Stack
Web APIs
Task Queue
(Also, a job queue)
console.log('Paper delivered to house 1')
setTimeout(() => {
console.log('Paper delivered to house 2'), 0)
}
console.log('Paper delivered to house 3')
Console
main()
Call Stack
Web APIs
Task Queue
(Also, a job queue)
console.log('Paper delivered to house 1')
setTimeout(() => {
console.log('Paper delivered to house 2'), 0)
}
console.log('Paper delivered to house 3')
Console
main()
log('house 1')
Call Stack
Web APIs
Task Queue
(Also, a job queue)
console.log('Paper delivered to house 1')
setTimeout(() => {
console.log('Paper delivered to house 2'), 0)
}
console.log('Paper delivered to house 3')
Console
main()
log('house 1')
Call Stack
Web APIs
Task Queue
(Also, a job queue)
console.log('Paper delivered to house 1')
setTimeout(() => {
console.log('Paper delivered to house 2'), 0)
}
console.log('Paper delivered to house 3')
Console
main()
log('house 1')
setTimeout()
Call Stack
Web APIs
Task Queue
(Also, a job queue)
console.log('Paper delivered to house 1')
setTimeout(() => {
console.log('Paper delivered to house 2'), 0)
}
console.log('Paper delivered to house 3')
Console
main()
log('house 1')
setTimeout()
Call Stack
Web APIs
Task Queue
(Also, a job queue)
console.log('Paper delivered to house 1')
setTimeout(() => {
console.log('Paper delivered to house 2'), 0)
}
console.log('Paper delivered to house 3')
Console
main()
log('house 1')
setTimeout() - cb or promise...
log('house 3')
Call Stack
Web APIs
Task Queue
(Also, a job queue)
console.log('Paper delivered to house 1')
setTimeout(() => {
console.log('Paper delivered to house 2'), 0)
}
console.log('Paper delivered to house 3')
Console
log('house 1')
setTimeout() - cb or promise...
log('house 3')
Call Stack
Web APIs
Task Queue
(Also, a job queue)
console.log('Paper delivered to house 1')
setTimeout(() => {
console.log('Paper delivered to house 2'), 0)
}
console.log('Paper delivered to house 3')
Console
cb / promise
log('house 1')
log('house 3')
Call Stack
Web APIs
Task Queue
(Also, a job queue)
console.log('Paper delivered to house 1')
setTimeout(() => {
console.log('Paper delivered to house 2'), 0)
}
console.log('Paper delivered to house 3')
Console
log('house 1')
log('house 3')
log('house 2')
Backend BABY
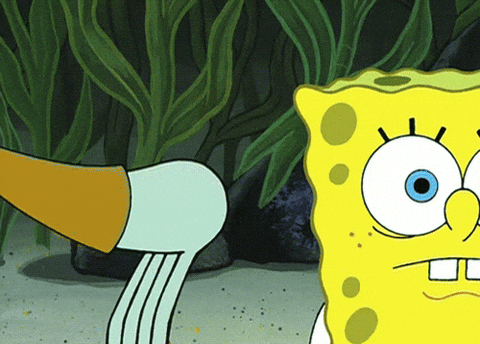
Does Javascript have access to the DOM natively (built in)?
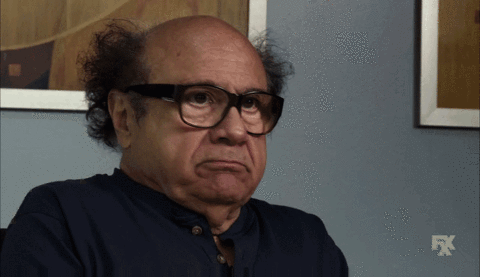
Javascript needed Web APIs to handle async and a bunch of stuff in the Browser
JS is a language that can only do what the hosting environment allows
What Do Servers Need?
Disk Access
(hardrive/ssd)
&&
Network Access
(internet, request / responses)
What if there was a hosting environment that allowed JS to have disk and network access
Music & Light Warning - Next Slide
NODE.js BABY
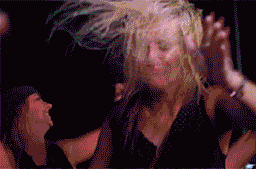
Node.js is a JavaScript runtime built on Chrome's V8 JavaScript engine.


The same shit that lets you run JS in the browser can now be used to run JS on Servers, Desktops, and elsewhere
Engine Vs. Compiler
And just like the browser's Web APIs Node come with a bunch of stuff
Built in Modules
(libraries or collections of functions)
HTTP (network access)
FS (file system access)
Access to millions of packages via NPM
(groupings of one or more custom modules)
Not Web APIs, but C/C++ APIs

sorry, don't remember the source
Let's Code
Simple Node Server

Just
HTTP & FS
const http = require('http')
const fs = require('fs')
http.createServer((req, res) => {
fs.readFile('demofile.html', (err, data) => {
res.writeHead(200, {'Content-Type': 'text/html'})
res.write(data)
res.end()
})
}).listen(8000)
Let's Look
More Complex Backend

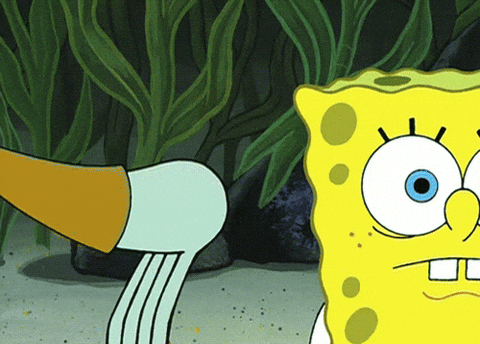
How could we clean this up?
Express
But First
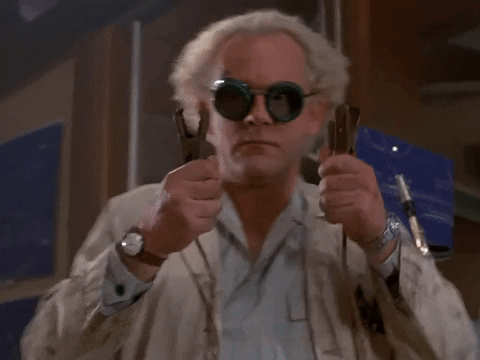
Blast To The Past
How Does The Internet Work
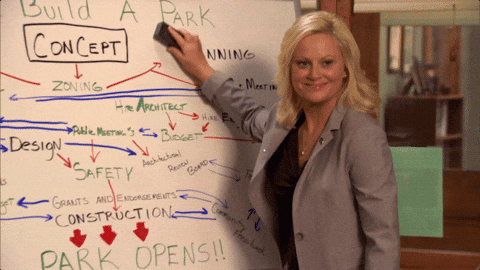
CRUD
Create (post) - Make something
Read (get) - Get Something
Update (put) - Change something
Delete (delete) - Remove something
What are some Create (post) requests?
What are some Read (get) requests?
What are some Update (put) requests?
What are some Delete (delete) requests?
Coffee Chat & Professional Review
Let's Build An App with Express
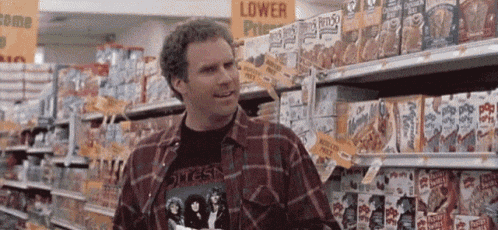
Express
Fast, unopinionated, minimalist web framework for Node.js
With a myriad of HTTP utility methods and middleware at your disposal, creating a robust API is quick and easy.

TONIGHT WE BUILD
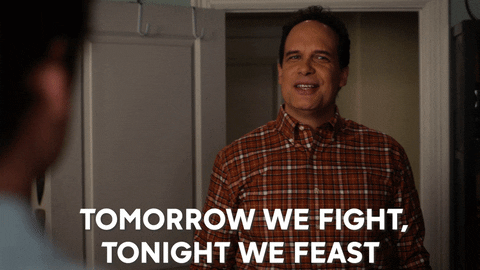
Key Steps
mkdir api-project
cd api-project
npm init
npm install express --save
Setting Up The Project
app.get('/', (req, res) => {
res.sendFile(__dirname + '/index.html')
})
Serving Up HTML
app.get('/api/savage', (request, response) => {
response.json(object)
})
Serving Up JSON
heroku login -i
heroku create simple-rap-api
echo "web: node server.js" > Procfile
git add .
git commit -m "changes"
git push heroku main
Push To Heroku

Homework
Do: Start prepping THE BANK
Do: Complete Your Professional Links
Create: Heroku, Mongo Atlas, and Postman Accounts
Read: Node.js and Express (Fullstack Open)
Do: Make Your Own API and Push To Heroku
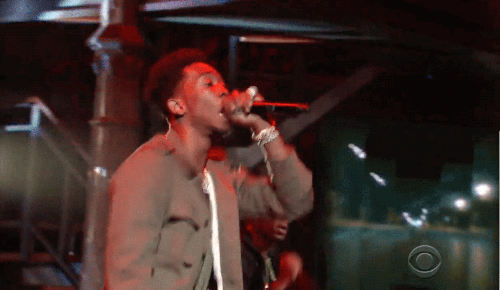
#100Devs - Node & Express
By Leon Noel
#100Devs - Node & Express
Class 38 of our Free Web Dev Bootcamp for folx affected by the pandemic. Join live T/Th 6:30pm ET leonnoel.com/twitch and ask questions here: leonnoel.com/discord
- 3,312